Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / ManipulationInertiaStartingEventArgs.cs / 1305600 / ManipulationInertiaStartingEventArgs.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Windows; using System.Windows.Input.Manipulations; using System.Windows.Media; using MS.Internal; namespace System.Windows.Input { ////// Provides information about the start of the inertia phase of the manipulation. /// public sealed class ManipulationInertiaStartingEventArgs : InputEventArgs { ////// Instantiates a new instance of this class. /// internal ManipulationInertiaStartingEventArgs( ManipulationDevice manipulationDevice, int timestamp, IInputElement manipulationContainer, Point origin, ManipulationVelocities initialVelocities, bool isInInertia) : base(manipulationDevice, timestamp) { if (initialVelocities == null) { throw new ArgumentNullException("initialVelocities"); } RoutedEvent = Manipulation.ManipulationInertiaStartingEvent; ManipulationContainer = manipulationContainer; ManipulationOrigin = origin; InitialVelocities = initialVelocities; _isInInertia = isInInertia; } ////// Invokes a handler of this event. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { if (genericHandler == null) { throw new ArgumentNullException("genericHandler"); } if (genericTarget == null) { throw new ArgumentNullException("genericTarget"); } if (RoutedEvent == Manipulation.ManipulationInertiaStartingEvent) { ((EventHandler)genericHandler)(genericTarget, this); } else { base.InvokeEventHandler(genericHandler, genericTarget); } } /// /// Defines the coordinate space of the other properties. /// public IInputElement ManipulationContainer { get; private set; } ////// Returns the value of the origin. /// public Point ManipulationOrigin { get; set; } ////// Returns the default, calculated velocities of the current manipulation. /// ////// These values can be used to populate the initial velocity properties in /// the various behaviors that can be set. /// public ManipulationVelocities InitialVelocities { get; private set; } ////// The desired behavior for how position changes due to inertia. /// public InertiaTranslationBehavior TranslationBehavior { get { if (!IsBehaviorSet(Behaviors.Translation)) { _translationBehavior = new InertiaTranslationBehavior(InitialVelocities.LinearVelocity); SetBehavior(Behaviors.Translation); } return _translationBehavior; } set { _translationBehavior = value; } } ////// The desired behavior for how the angle changes due to inertia. /// public InertiaRotationBehavior RotationBehavior { get { if (!IsBehaviorSet(Behaviors.Rotation)) { _rotationBehavior = new InertiaRotationBehavior(InitialVelocities.AngularVelocity); SetBehavior(Behaviors.Rotation); } return _rotationBehavior; } set { _rotationBehavior = value; } } ////// The desired behavior for how the size changes due to inertia. /// public InertiaExpansionBehavior ExpansionBehavior { get { if (!IsBehaviorSet(Behaviors.Expansion)) { _expansionBehavior = new InertiaExpansionBehavior(InitialVelocities.ExpansionVelocity); SetBehavior(Behaviors.Expansion); } return _expansionBehavior; } set { _expansionBehavior = value; } } ////// Method to cancel the Manipulation /// ///A bool indicating the success of Cancel public bool Cancel() { if (!_isInInertia) { RequestedCancel = true; return true; } return false; } ////// A handler Requested to cancel the Manipulation /// internal bool RequestedCancel { get; private set; } ////// The Manipulators for this manipulation. /// public IEnumerableManipulators { get { if (_manipulators == null) { _manipulators = ((ManipulationDevice)Device).GetManipulatorsReadOnly(); } return _manipulators; } } /// /// Supplies additional parameters for calculating the inertia. /// /// The new parameter. [Browsable(false)] public void SetInertiaParameter(InertiaParameters2D parameter) { if (parameter == null) { throw new ArgumentNullException("parameter"); } if (_inertiaParameters == null) { _inertiaParameters = new List(); } _inertiaParameters.Add(parameter); } internal bool CanBeginInertia() { if (_inertiaParameters != null && _inertiaParameters.Count > 0) { return true; } if (_translationBehavior != null && _translationBehavior.CanUseForInertia()) { return true; } if (_rotationBehavior != null && _rotationBehavior.CanUseForInertia()) { return true; } if (_expansionBehavior != null && _expansionBehavior.CanUseForInertia()) { return true; } return false; } internal void ApplyParameters(InertiaProcessor2D processor) { processor.InitialOriginX = (float)ManipulationOrigin.X; processor.InitialOriginY = (float)ManipulationOrigin.Y; ManipulationVelocities velocities = InitialVelocities; InertiaTranslationBehavior.ApplyParameters(_translationBehavior, processor, velocities.LinearVelocity); InertiaRotationBehavior.ApplyParameters(_rotationBehavior, processor, velocities.AngularVelocity); InertiaExpansionBehavior.ApplyParameters(_expansionBehavior, processor, velocities.ExpansionVelocity); if (_inertiaParameters != null) { for (int i = 0, count = _inertiaParameters.Count; i < count; i++) { processor.SetParameters(_inertiaParameters[i]); } } } private bool IsBehaviorSet(Behaviors behavior) { return ((_behaviors & behavior) == behavior); } private void SetBehavior(Behaviors behavior) { _behaviors |= behavior; } private List _inertiaParameters; private InertiaTranslationBehavior _translationBehavior; private InertiaRotationBehavior _rotationBehavior; private InertiaExpansionBehavior _expansionBehavior; private Behaviors _behaviors = Behaviors.None; private bool _isInInertia = false; // This is true when it is a second level inertia (inertia due to inertia). private IEnumerable _manipulators; [Flags] private enum Behaviors { None = 0, Translation = 0x00000001, Rotation = 0x00000002, Expansion = 0x00000004 } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
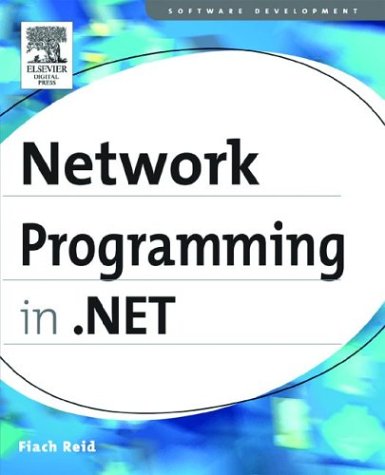
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringDictionary.cs
- PackWebResponse.cs
- SqlXml.cs
- ServiceParser.cs
- ServiceManagerHandle.cs
- ContextStaticAttribute.cs
- HtmlTextArea.cs
- BuildProvider.cs
- ListenerConstants.cs
- MethodInfo.cs
- Dispatcher.cs
- DiscreteKeyFrames.cs
- NativeMethodsCLR.cs
- InfoCardArgumentException.cs
- SecurityHeaderTokenResolver.cs
- DataGridColumn.cs
- BamlRecordHelper.cs
- GeneratedContractType.cs
- Accessible.cs
- Maps.cs
- ObjectDataSourceMethodEventArgs.cs
- StringConverter.cs
- CaseInsensitiveOrdinalStringComparer.cs
- SafeRightsManagementSessionHandle.cs
- LongPath.cs
- AggregatePushdown.cs
- GridItemCollection.cs
- AutoGeneratedField.cs
- CodeTypeOfExpression.cs
- FormsAuthenticationModule.cs
- OdbcConnection.cs
- FormatterServices.cs
- DomNameTable.cs
- InertiaExpansionBehavior.cs
- Type.cs
- CreatingCookieEventArgs.cs
- PrimitiveSchema.cs
- FormViewModeEventArgs.cs
- ObjectCloneHelper.cs
- UmAlQuraCalendar.cs
- _ScatterGatherBuffers.cs
- EditingCommands.cs
- ListItemParagraph.cs
- ToolStrip.cs
- TaiwanLunisolarCalendar.cs
- DiagnosticEventProvider.cs
- Rule.cs
- DiscardableAttribute.cs
- StateBag.cs
- KeyBinding.cs
- Point3DAnimation.cs
- TextChange.cs
- LocationReferenceEnvironment.cs
- WindowsGraphics.cs
- UnsafeNativeMethods.cs
- HandlerFactoryWrapper.cs
- ErrorRuntimeConfig.cs
- pingexception.cs
- TypedElement.cs
- SpeechSeg.cs
- ExpressionCopier.cs
- _TransmitFileOverlappedAsyncResult.cs
- SpeechAudioFormatInfo.cs
- Object.cs
- _NegoStream.cs
- EntityDataSourceContainerNameConverter.cs
- CommandLibraryHelper.cs
- EventlogProvider.cs
- EntryIndex.cs
- ProjectionPruner.cs
- DesignTimeValidationFeature.cs
- TreeNodeSelectionProcessor.cs
- CodeDelegateCreateExpression.cs
- TagPrefixInfo.cs
- ArgumentException.cs
- ImageCollectionCodeDomSerializer.cs
- PersonalizationEntry.cs
- GridSplitterAutomationPeer.cs
- LogicalTreeHelper.cs
- ScrollChrome.cs
- LoadedOrUnloadedOperation.cs
- GlyphsSerializer.cs
- CFStream.cs
- Helpers.cs
- WebRequestModuleElementCollection.cs
- ProcessThread.cs
- MetadataStore.cs
- ClonableStack.cs
- HandlerBase.cs
- RtfFormatStack.cs
- TdsParameterSetter.cs
- NullableIntSumAggregationOperator.cs
- SID.cs
- HttpConfigurationSystem.cs
- ArraySegment.cs
- List.cs
- SpellerHighlightLayer.cs
- ObjectAnimationUsingKeyFrames.cs
- TcpDuplicateContext.cs
- SoapSchemaExporter.cs