Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Controls / SelectionChangedEventArgs.cs / 1 / SelectionChangedEventArgs.cs
using System.ComponentModel; using System.Collections; using System.Collections.Generic; using System.Windows.Threading; using System.Windows.Data; using System.Windows.Automation; using System.Windows.Automation.Provider; using MS.Utility; using System.Windows; using System; using System.Diagnostics; namespace System.Windows.Controls { ////// The delegate type for handling a selection changed event /// public delegate void SelectionChangedEventHandler( object sender, SelectionChangedEventArgs e); ////// The inputs to a selection changed event handler /// public class SelectionChangedEventArgs : RoutedEventArgs { #region Constructors ////// The constructor for selection changed args /// /// The event ID for the event about to fire -- should probably be Selector.SelectionChangedEvent /// The items that were unselected during this event /// The items that were selected during this event public SelectionChangedEventArgs( RoutedEvent id, IList removedItems, IList addedItems) { if (id == null) throw new ArgumentNullException("id"); if (removedItems == null) throw new ArgumentNullException("removedItems"); if (addedItems == null) throw new ArgumentNullException("addedItems"); RoutedEvent = id; _removedItems = new object[removedItems.Count]; removedItems.CopyTo(_removedItems, 0); _addedItems = new object[addedItems.Count]; addedItems.CopyTo(_addedItems, 0); } internal SelectionChangedEventArgs(IList removedItems, IList addedItems) : this(System.Windows.Controls.Primitives.Selector.SelectionChangedEvent, removedItems, addedItems) { } #endregion #region Public Properties ////// An IList containing the items that were unselected during this event /// public IList RemovedItems { get { return _removedItems; } } ////// An IList containing the items that were selected during this event /// public IList AddedItems { get { return _addedItems; } } #endregion #region Protected Methods ////// This method is used to perform the proper type casting in order to /// call the type-safe SelectionChangedEventHandler delegate for the SelectionChangedEvent event. /// /// The handler to invoke. /// The current object along the event's route. protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { SelectionChangedEventHandler handler = (SelectionChangedEventHandler)genericHandler; handler(genericTarget, this); } #endregion #region Data private object[] _addedItems; private object[] _removedItems; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.ComponentModel; using System.Collections; using System.Collections.Generic; using System.Windows.Threading; using System.Windows.Data; using System.Windows.Automation; using System.Windows.Automation.Provider; using MS.Utility; using System.Windows; using System; using System.Diagnostics; namespace System.Windows.Controls { ////// The delegate type for handling a selection changed event /// public delegate void SelectionChangedEventHandler( object sender, SelectionChangedEventArgs e); ////// The inputs to a selection changed event handler /// public class SelectionChangedEventArgs : RoutedEventArgs { #region Constructors ////// The constructor for selection changed args /// /// The event ID for the event about to fire -- should probably be Selector.SelectionChangedEvent /// The items that were unselected during this event /// The items that were selected during this event public SelectionChangedEventArgs( RoutedEvent id, IList removedItems, IList addedItems) { if (id == null) throw new ArgumentNullException("id"); if (removedItems == null) throw new ArgumentNullException("removedItems"); if (addedItems == null) throw new ArgumentNullException("addedItems"); RoutedEvent = id; _removedItems = new object[removedItems.Count]; removedItems.CopyTo(_removedItems, 0); _addedItems = new object[addedItems.Count]; addedItems.CopyTo(_addedItems, 0); } internal SelectionChangedEventArgs(IList removedItems, IList addedItems) : this(System.Windows.Controls.Primitives.Selector.SelectionChangedEvent, removedItems, addedItems) { } #endregion #region Public Properties ////// An IList containing the items that were unselected during this event /// public IList RemovedItems { get { return _removedItems; } } ////// An IList containing the items that were selected during this event /// public IList AddedItems { get { return _addedItems; } } #endregion #region Protected Methods ////// This method is used to perform the proper type casting in order to /// call the type-safe SelectionChangedEventHandler delegate for the SelectionChangedEvent event. /// /// The handler to invoke. /// The current object along the event's route. protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { SelectionChangedEventHandler handler = (SelectionChangedEventHandler)genericHandler; handler(genericTarget, this); } #endregion #region Data private object[] _addedItems; private object[] _removedItems; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
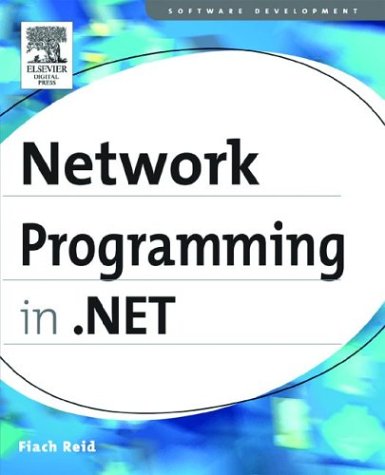
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InternalBufferOverflowException.cs
- AlternateView.cs
- AppDomainCompilerProxy.cs
- Matrix.cs
- WS2007FederationHttpBinding.cs
- TreeNodeBindingCollection.cs
- TypeUsageBuilder.cs
- Pair.cs
- WebPartConnectionsConnectVerb.cs
- Convert.cs
- DeferredTextReference.cs
- DesignerToolStripControlHost.cs
- DisableDpiAwarenessAttribute.cs
- DataErrorValidationRule.cs
- WebBrowserUriTypeConverter.cs
- AssemblyFilter.cs
- SmtpCommands.cs
- SqlDataAdapter.cs
- BamlRecords.cs
- SmiMetaDataProperty.cs
- XmlSchemaSimpleTypeRestriction.cs
- TableParagraph.cs
- ServiceBuildProvider.cs
- HttpResponseHeader.cs
- Int16KeyFrameCollection.cs
- UnSafeCharBuffer.cs
- StatusBarItem.cs
- PersonalizationAdministration.cs
- TdsParameterSetter.cs
- TypeConverterAttribute.cs
- SafeLocalAllocation.cs
- HtmlLink.cs
- Drawing.cs
- MetadataArtifactLoaderCompositeFile.cs
- TargetConverter.cs
- PolicyFactory.cs
- GifBitmapEncoder.cs
- ContainsRowNumberChecker.cs
- ProtocolReflector.cs
- Stopwatch.cs
- SchemaImporter.cs
- MsmqIntegrationOutputChannel.cs
- SmtpReplyReader.cs
- CustomCredentialPolicy.cs
- GroupBoxAutomationPeer.cs
- CheckBoxFlatAdapter.cs
- RequestCacheValidator.cs
- XamlTreeBuilder.cs
- CodeAttributeArgument.cs
- XmlSerializerVersionAttribute.cs
- DataRowChangeEvent.cs
- SplitterEvent.cs
- TargetInvocationException.cs
- RepeaterItemCollection.cs
- XmlQueryOutput.cs
- URL.cs
- HttpClientChannel.cs
- WindowsTooltip.cs
- TextProperties.cs
- PageAsyncTaskManager.cs
- BaseParaClient.cs
- SemanticKeyElement.cs
- TabControlAutomationPeer.cs
- DecoderBestFitFallback.cs
- ExpandedWrapper.cs
- DataMisalignedException.cs
- Timer.cs
- DataSvcMapFile.cs
- WhiteSpaceTrimStringConverter.cs
- DataGridTextBoxColumn.cs
- CellIdBoolean.cs
- XPathArrayIterator.cs
- WeakHashtable.cs
- WpfXamlMember.cs
- ToolStripPanel.cs
- FormDesigner.cs
- BlockUIContainer.cs
- EdmProviderManifest.cs
- BidOverLoads.cs
- CustomAttribute.cs
- XsltLibrary.cs
- DataGridItem.cs
- SqlNotificationEventArgs.cs
- XmlSignificantWhitespace.cs
- StaticFileHandler.cs
- UserMapPath.cs
- SystemFonts.cs
- ActivityInstance.cs
- DeploymentSection.cs
- Decoder.cs
- Rfc2898DeriveBytes.cs
- ReservationNotFoundException.cs
- ResourceWriter.cs
- _SSPISessionCache.cs
- ExceptionHelpers.cs
- AtomicFile.cs
- XhtmlStyleClass.cs
- ExtensionQuery.cs
- AttributeCollection.cs
- FixedSOMPageElement.cs