Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / PolicyFactory.cs / 1 / PolicyFactory.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // // Presharp uses the c# pragma mechanism to supress its warnings. // These are not recognised by the base compiler so we need to explictly // disable the following warnings. See http://winweb/cse/Tools/PREsharp/userguide/default.asp // for details. // #pragma warning disable 1634, 1691 // unknown message, unknown pragma namespace Microsoft.InfoCards { using System; using System.IO; using System.Xml; using System.Text; using System.Diagnostics; using System.ServiceModel; using System.Globalization; using System.Collections.Generic; using System.IdentityModel.Tokens; using System.IdentityModel.Selectors; using System.Collections.ObjectModel; using System.ServiceModel.Security.Tokens; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; internal enum PolicyType { PrimaryOnly, SecondaryOnly, Composite } // // This class contains the xml string forms of the incoming policy. It is used to manage the cases where we // are dealing with a composite of primary and secondary policies. // internal class ParsedPolicy { private string m_policyXmlOriginal; // Stores a copy of the original xml from which the policies were parsed. private string m_policyXmlPrimary; // Stores the raw policy xml for the primary policy. private string m_policyXmlSecondary; // Stores the raw policy xml for the secondary policy. private PolicyType m_policyType; // Stores whether both primary and secondary policy are present. public string PolicyXmlOriginal { get { return m_policyXmlOriginal; } } public string PolicyXmlPrimary { get { return m_policyXmlPrimary; } } public string PolicyXmlSecondary { get { return m_policyXmlSecondary; } } public PolicyType PolicyType { get { return m_policyType; } } public ParsedPolicy( string policyXmlOriginal, string policyXmlPrimary, string policyXmlSecondary ) { IDT.Assert( !String.IsNullOrEmpty( policyXmlOriginal ), "Original Policy Xml cannot be null or empty" ); m_policyXmlOriginal = policyXmlOriginal; m_policyXmlPrimary = policyXmlPrimary; m_policyXmlSecondary = policyXmlSecondary; if ( !String.IsNullOrEmpty( policyXmlPrimary ) && String.IsNullOrEmpty( policyXmlSecondary ) ) { m_policyType = PolicyType.PrimaryOnly; } else if( !String.IsNullOrEmpty( policyXmlPrimary ) && !String.IsNullOrEmpty( policyXmlSecondary ) ) { m_policyType = PolicyType.Composite; } else if( String.IsNullOrEmpty( policyXmlPrimary ) && !String.IsNullOrEmpty( policyXmlSecondary ) ) { m_policyType = PolicyType.SecondaryOnly; } else { IDT.Assert( false, "Both policies cannot be empty" ); } } } internal class PolicyFactory { private PolicyFactory() { } public static Policy CreatePolicyFromUnwrappedPolicyXml( string originalPolicyXml ) { // // wrap it in a root element to make it parseable // string rstPolicyXml = String.Format( CultureInfo.InvariantCulture, "<{0}:{1} xmlns:{0}='{2}'>{3}{0}:{1}>" , XmlNames.WSSecurityPolicyXmlSoap2005.Instance.DefaultPrefix , XmlNames.WSSecurityPolicyXmlSoap2005.Instance.RequestSecurityTokenTemplate , XmlNames.WSSecurityPolicyXmlSoap2005.Instance.Namespace , originalPolicyXml ); return new Policy( originalPolicyXml, rstPolicyXml ); } // // Used by CustomTokenProvider // public static InfoCardPolicy CreatePolicyForCustomTokenProvider( EndpointAddress immediateTokenRecipient, IssuedSecurityTokenParameters issuedTokenParameters, ProtocolProfile profile ) { ParsedPolicy policy = CreateParsedPolicy( issuedTokenParameters.CreateRequestParameters( profile.MsgSecurityVersion, profile.TokenSerializer ) ); return new InfoCardPolicy( immediateTokenRecipient , issuedTokenParameters.IssuerAddress , policy , PolicyUsageContext.GetToken , null //there is no privacy URL , 0 //there is no privacy version , null //recipientIdentity will be populated later via SetRecipientInfo , false ); } // // Used by GetBrowserTokenRequest // // Remarks: // (a) If target xmlelement is null, which is not supposed to be, this will automatically throw. // (b) IssuerElement can be null. // public static InfoCardPolicy CreatePolicyForGetBrowserTokenRequest( CardSpacePolicyElement policyElement, Uri recipientPolicyNoticeLink, uint recipientPolicyNoticeVersion, RecipientIdentity recipientIdentity, PolicyUsageContext usageContext ) { EndpointAddress immediateTokenRecipient = null; if( !String.IsNullOrEmpty( policyElement.Target.OuterXml ) ) { immediateTokenRecipient = CreateEndpointAddressFromString( policyElement.Target.OuterXml ); } EndpointAddress issuer = null; if( null != policyElement.Issuer ) { if( !String.IsNullOrEmpty( policyElement.Issuer.OuterXml ) ) { issuer = CreateEndpointAddressFromString( policyElement.Issuer.OuterXml ); } } ParsedPolicy policy = CreateParsedPolicy( policyElement.Parameters ); // // Don't use policyElement.PolicyNoticeLink (and PolicyNoticeVersion) here because // we need to pass in the RECIPIENT's PolicyNoticeLink (and policyNoticeVersion). // return new InfoCardPolicy( immediateTokenRecipient , issuer , policy , usageContext , null != recipientPolicyNoticeLink ? recipientPolicyNoticeLink.ToString() : null , recipientPolicyNoticeVersion , recipientIdentity , false ); // we need the recipientIdentity here because we validate the recipient before we parse the policy } // // Used by GetBrowserTokenRequest // // Remarks: // (a) If target xmlelement is null, which is not supposed to be, this will automatically throw. // (b) IssuerElement can be null. // public static InfoCardPolicy CreatePolicyForIntermediateGetBrowserTokenRequest( CardSpacePolicyElement policyElement, Uri recipientPolicyNoticeLink, uint recipientPolicyNoticeVersion, RecipientIdentity recipientIdentity ) { EndpointAddress immediateTokenRecipient = null; if( !String.IsNullOrEmpty( policyElement.Target.OuterXml ) ) { immediateTokenRecipient = CreateEndpointAddressFromString( policyElement.Target.OuterXml ); } EndpointAddress issuer = null; if( null != policyElement.Issuer ) { if( !String.IsNullOrEmpty( policyElement.Issuer.OuterXml ) ) { issuer = CreateEndpointAddressFromString( policyElement.Issuer.OuterXml ); } } ParsedPolicy policy = CreateParsedPolicy( policyElement.Parameters ); // // Don't use policyElement.PolicyNoticeLink (and PolicyNoticeVersion) here because // we need to pass in the RECIPIENT's PolicyNoticeLink (and policyNoticeVersion). // return new InfoCardPolicy( immediateTokenRecipient , issuer , policy , PolicyUsageContext.Intermediate , null != recipientPolicyNoticeLink ? recipientPolicyNoticeLink.ToString() : null , recipientPolicyNoticeVersion , recipientIdentity , false ); // we need the recipientIdentity here because we validate the recipient before we parse the policy } // // Used by GetTokenRequest // public static InfoCardPolicy CreatePolicyForGetTokenRequest( BinaryReader reader, string recipientXml, string issuerXml, string policyXml, bool isManaged ) { if( !String.IsNullOrEmpty( policyXml ) ) { // // We need to convert the incoming raw policy XML string into a DOM so we can manipulate it. // // // wrap it in a root element to make it parseable // string wrappedPolicyXml = String.Format( CultureInfo.InvariantCulture, "<{0}:{1} xmlns:{0}='{2}'>{3}{0}:{1}>" , XmlNames.WSSecurityPolicyXmlSoap2005.Instance.DefaultPrefix , XmlNames.WSSecurityPolicyXmlSoap2005.Instance.RequestSecurityTokenTemplate , XmlNames.WSSecurityPolicyXmlSoap2005.Instance.Namespace , policyXml ); XmlDocument policyElements = new XmlDocument(); policyElements.LoadXml( wrappedPolicyXml ); Collectionelements = new Collection (); foreach( XmlElement element in policyElements.DocumentElement.ChildNodes ) { elements.Add( element ); } ParsedPolicy policy = CreateParsedPolicy( elements ); return new InfoCardPolicy( !String.IsNullOrEmpty( recipientXml ) ? CreateEndpointAddressFromString( recipientXml ) : null , !String.IsNullOrEmpty( issuerXml ) ? CreateEndpointAddressFromString( issuerXml ) : null , policy , PolicyUsageContext.GetToken , null //privacy URL will be populated later as this is NOT true , 0 //privacy version will be populated later , null , isManaged ); //recipientIdentity will be populated later via SetRecipientInfo } else { // // policyXml is null. We should only receive a null policy Xml if the isManaged flag is set. // if( !isManaged ) { throw IDT.ThrowHelperError( new PolicyValidationException( SR.GetString( SR.InvalidPolicySpecified ) ) ); } return new InfoCardPolicy( !String.IsNullOrEmpty( recipientXml ) ? CreateEndpointAddressFromString( recipientXml ) : null , !String.IsNullOrEmpty( issuerXml ) ? CreateEndpointAddressFromString( issuerXml ) : null , null , PolicyUsageContext.GetToken , null //privacy URL will be populated later as this is NOT true , 0 //privacy version will be populated later , null , isManaged ); //recipientIdentity will be populated later via SetRecipientInfo } } private static ParsedPolicy CreateParsedPolicy( IEnumerable elements ) { try { XmlElement secondaryParams = null; StringBuilder primaryPolicyBuilder = new StringBuilder(); // Used to concatenate the strings belonging to the primary policy. StringBuilder originalPolicyBuilder = new StringBuilder(); // Used to concatenate all strings inside the Xml elements foreach( XmlElement element in elements ) { originalPolicyBuilder.Append( element.OuterXml ); if( element.LocalName == XmlNames.WSTrustOasis2007.c_SecondaryParameters && element.NamespaceURI == XmlNames.WSTrustOasis2007.c_Namespace ) { // // Found a SecondaryParameters element. Set this element aside to use later // on in the formation of the composite policy. // secondaryParams = element; } else { primaryPolicyBuilder.Append( element.OuterXml ); } } if( null != secondaryParams ) { // // We have a valid SecondaryParameters element. // return new ParsedPolicy( originalPolicyBuilder.ToString(), primaryPolicyBuilder.ToString(), secondaryParams.InnerXml ); } else { return new ParsedPolicy( originalPolicyBuilder.ToString(), primaryPolicyBuilder.ToString(), null ); } } catch( XmlException xe ) { throw IDT.ThrowHelperError( new PolicyValidationException( SR.GetString( SR.InvalidPolicySpecified ), xe ) ); } } // // Summary: // Takes a fragment of xml and tries to deserialize it into an EndpointAddress. // // Parameters: // fragment - A fragment of Xml to deserialize into an EndpointAddress. // private static EndpointAddress CreateEndpointAddressFromString( string fragment ) { try { using( XmlReader reader = LoadXmlIntoReader( fragment.Trim() ) ) { // // We ignore the reader.MoveToElement return, because the // constructor of EndpointAddress will throw an exception // for us. // reader.MoveToElement(); EndpointAddress epr; epr = EndpointAddress.ReadFrom( XmlDictionaryReader.CreateDictionaryReader( reader ) ); return epr; } } catch( Exception e ) { if( IDT.IsFatal( e ) ) { throw; } throw IDT.ThrowHelperError( new PolicyValidationException( SR.GetString( SR.ServiceInvalidEprInPolicy ), e ) ); } } // // Summary: // Takes a fragment of xml in the form of a string and returns an XmlReader. // // Parameters: // fragment - A fragment of Xml to load into an XmlReader. // private static XmlReader LoadXmlIntoReader( string fragment ) { return InfoCardSchemas.CreateReader( fragment ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
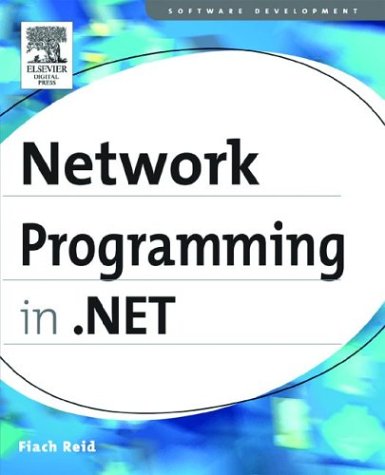
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpHandlerActionCollection.cs
- BmpBitmapEncoder.cs
- GridViewCancelEditEventArgs.cs
- ETagAttribute.cs
- XpsFont.cs
- RandomNumberGenerator.cs
- PolicyDesigner.cs
- AtomServiceDocumentSerializer.cs
- SoapHttpTransportImporter.cs
- UrlPropertyAttribute.cs
- SaveFileDialog.cs
- ActiveXContainer.cs
- TriggerActionCollection.cs
- NameValuePair.cs
- XAMLParseException.cs
- DecodeHelper.cs
- COM2ComponentEditor.cs
- webproxy.cs
- Bitmap.cs
- UnsafeMethods.cs
- HttpProxyCredentialType.cs
- RepeatBehaviorConverter.cs
- XmlNodeList.cs
- CompoundFileStreamReference.cs
- XsltLibrary.cs
- UnionExpr.cs
- PartialList.cs
- PerformanceCounterLib.cs
- TrustLevel.cs
- __FastResourceComparer.cs
- ProtocolsSection.cs
- InfoCardUIAgent.cs
- WindowsScrollBarBits.cs
- Control.cs
- KnownTypesHelper.cs
- GorillaCodec.cs
- translator.cs
- ValidationRuleCollection.cs
- PersonalizablePropertyEntry.cs
- XslException.cs
- RepeaterCommandEventArgs.cs
- DetailsViewDeletedEventArgs.cs
- CreateRefExpr.cs
- WizardForm.cs
- PersistencePipeline.cs
- MappingException.cs
- MatrixStack.cs
- UIElement3D.cs
- DescendantBaseQuery.cs
- FilterEventArgs.cs
- MouseBinding.cs
- ErrorFormatterPage.cs
- WithParamAction.cs
- CustomCategoryAttribute.cs
- XmlNotation.cs
- DBSchemaRow.cs
- ServiceRouteHandler.cs
- Highlights.cs
- SQLBytes.cs
- Rect3DConverter.cs
- WpfKnownTypeInvoker.cs
- SqlDataSourceFilteringEventArgs.cs
- QilSortKey.cs
- StreamGeometry.cs
- Size3DConverter.cs
- DrawingCollection.cs
- TreeViewEvent.cs
- DeclaredTypeValidator.cs
- ComponentChangedEvent.cs
- Cursor.cs
- SafeBitVector32.cs
- DataSourceXmlSerializer.cs
- SQlBooleanStorage.cs
- ChangeProcessor.cs
- MarkupWriter.cs
- PagedDataSource.cs
- PrincipalPermission.cs
- ActivityStateQuery.cs
- x509utils.cs
- CompModHelpers.cs
- OdbcStatementHandle.cs
- FormViewPagerRow.cs
- COMException.cs
- CapacityStreamGeometryContext.cs
- StsCommunicationException.cs
- MenuItemStyleCollection.cs
- Win32.cs
- NumericPagerField.cs
- DataIdProcessor.cs
- ImageIndexConverter.cs
- RayMeshGeometry3DHitTestResult.cs
- ResourceExpressionEditorSheet.cs
- ReflectPropertyDescriptor.cs
- altserialization.cs
- StylusCaptureWithinProperty.cs
- ExpressionList.cs
- HostTimeoutsElement.cs
- InkCanvasSelectionAdorner.cs
- XmlSchemaObjectTable.cs
- ListDictionaryInternal.cs