Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / RepeatBehaviorConverter.cs / 1305600 / RepeatBehaviorConverter.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: RepeatBehaviorConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows.Media.Animation { ////// /// public sealed class RepeatBehaviorConverter : TypeConverter { #region Data private static char[] _iterationCharacter = new char[] { 'x' }; #endregion ////// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// ///public override object ConvertFrom( ITypeDescriptorContext td, CultureInfo cultureInfo, object value) { string stringValue = value as string; if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Forever") { return RepeatBehavior.Forever; } else if ( stringValue.Length > 0 && stringValue[stringValue.Length - 1] == _iterationCharacter[0]) { string stringDoubleValue = stringValue.TrimEnd(_iterationCharacter); double doubleValue = (double)TypeDescriptor.GetConverter(typeof(double)).ConvertFrom(td, cultureInfo, stringDoubleValue); return new RepeatBehavior(doubleValue); } } // The value is not Forever or an iteration count so it's either a TimeSpan // or we'll let the TimeSpanConverter raise the appropriate exception. TimeSpan timeSpanValue = (TimeSpan)TypeDescriptor.GetConverter(typeof(TimeSpan)).ConvertFrom(td, cultureInfo, stringValue); return new RepeatBehavior(timeSpanValue); } /// /// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for RepeatBehavior, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { if ( value is RepeatBehavior && destinationType != null) { RepeatBehavior repeatBehavior = (RepeatBehavior)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; if (repeatBehavior == RepeatBehavior.Forever) { mi = typeof(RepeatBehavior).GetProperty("Forever"); return new InstanceDescriptor(mi, null); } else if (repeatBehavior.HasCount) { mi = typeof(RepeatBehavior).GetConstructor(new Type[] { typeof(double) }); return new InstanceDescriptor(mi, new object[] { repeatBehavior.Count }); } else if (repeatBehavior.HasDuration) { mi = typeof(RepeatBehavior).GetConstructor(new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { repeatBehavior.Duration }); } else { Debug.Fail("Unknown type of RepeatBehavior passed to RepeatBehaviorConverter."); } } else if (destinationType == typeof(string)) { return repeatBehavior.InternalToString(null, cultureInfo); } } // We can't do the conversion, let the base class raise the // appropriate exception. return base.ConvertTo(context, cultureInfo, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
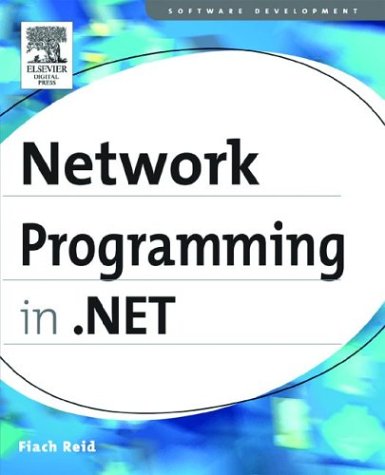
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BuildDependencySet.cs
- ClassGenerator.cs
- RepeatButton.cs
- Stylesheet.cs
- XsdDataContractExporter.cs
- HostingPreferredMapPath.cs
- AllowedAudienceUriElementCollection.cs
- columnmapkeybuilder.cs
- CompilationUtil.cs
- RegexCompiler.cs
- NativeMethods.cs
- ListenerElementsCollection.cs
- ZipPackage.cs
- WinFormsUtils.cs
- HtmlControlDesigner.cs
- ComplexTypeEmitter.cs
- HttpListenerContext.cs
- GroupByExpressionRewriter.cs
- OpenFileDialog.cs
- HostedHttpContext.cs
- NamespaceEmitter.cs
- PeerInputChannel.cs
- MenuItem.cs
- KeyValueConfigurationCollection.cs
- PropertyTabAttribute.cs
- AssemblyResourceLoader.cs
- Border.cs
- KeyFrames.cs
- WebPartConnectionsCancelVerb.cs
- SQLResource.cs
- ValidationUtility.cs
- BooleanFacetDescriptionElement.cs
- ParallelTimeline.cs
- DataGridViewTopLeftHeaderCell.cs
- EqualityComparer.cs
- TextTreeRootTextBlock.cs
- MessageSmuggler.cs
- InvalidEnumArgumentException.cs
- BulletedListEventArgs.cs
- CodeCastExpression.cs
- Bookmark.cs
- MeasureItemEvent.cs
- MouseDevice.cs
- TextAnchor.cs
- SqlServer2KCompatibilityAnnotation.cs
- GridItem.cs
- BindingExpression.cs
- ObfuscateAssemblyAttribute.cs
- XmlAttributeProperties.cs
- ToolStripDropDownMenu.cs
- DataControlLinkButton.cs
- WasEndpointConfigContainer.cs
- StringDictionaryCodeDomSerializer.cs
- PauseStoryboard.cs
- Codec.cs
- ManagementEventWatcher.cs
- BoundingRectTracker.cs
- _AutoWebProxyScriptHelper.cs
- StringValidator.cs
- UdpTransportSettings.cs
- RepeaterItemEventArgs.cs
- ListBoxItemWrapperAutomationPeer.cs
- HttpServerVarsCollection.cs
- DataSourceCache.cs
- BamlTreeMap.cs
- DrawListViewItemEventArgs.cs
- ExecutedRoutedEventArgs.cs
- FixedSOMTableRow.cs
- DateTimeUtil.cs
- VirtualPathProvider.cs
- ImageMap.cs
- FontStretch.cs
- FontFamily.cs
- TdsParserSessionPool.cs
- WSDualHttpBindingCollectionElement.cs
- DataControlFieldCell.cs
- DriveNotFoundException.cs
- MessageQueueException.cs
- connectionpool.cs
- ObjectQueryProvider.cs
- MenuItemBinding.cs
- DoubleLinkList.cs
- AccessDataSource.cs
- XmlCDATASection.cs
- TextWriterTraceListener.cs
- CodeCompileUnit.cs
- WorkflowServiceBuildProvider.cs
- AdornerHitTestResult.cs
- EditorZoneBase.cs
- ExeConfigurationFileMap.cs
- StylusLogic.cs
- TraceInternal.cs
- AdornerHitTestResult.cs
- TransactionException.cs
- _Rfc2616CacheValidators.cs
- ConfigurationManagerHelper.cs
- ManagementDateTime.cs
- BindToObject.cs
- SharedPersonalizationStateInfo.cs
- __Error.cs