Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Net / System / Net / Mail / ContentDisposition.cs / 1 / ContentDisposition.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Mime { using System; using System.Collections; using System.Collections.Specialized; using System.IO; using System.Text; using System.Globalization; using System.Net.Mail; public class ContentDisposition { string dispositionType; TrackingStringDictionary parameters; bool isChanged; bool isPersisted; string disposition; public ContentDisposition() { isChanged = true; disposition = "attachment"; ParseValue(); } ////// ctor. /// /// Unparsed header value. public ContentDisposition(string disposition) { if (disposition == null) throw new ArgumentNullException("disposition"); isChanged = true; this.disposition = disposition; ParseValue(); } ////// Gets the disposition type of the content. /// public string DispositionType { get { return dispositionType; } set { if (value == null) { throw new ArgumentNullException("value"); } if (value == string.Empty) { throw new ArgumentException(SR.GetString(SR.net_emptystringset), "value"); } isChanged = true; dispositionType = value; } } public StringDictionary Parameters { get { if (parameters == null) { parameters = new TrackingStringDictionary(); } return parameters; } } ////// Gets the value of the Filename parameter. /// public string FileName { get { return Parameters["filename"]; } set { if (value == null || value == string.Empty) { Parameters.Remove("filename"); } else{ Parameters["filename"] = value; } } } ////// Gets the value of the Creation-Date parameter. /// public DateTime CreationDate { get { string dtValue = Parameters["creation-date"]; if (dtValue == null) return DateTime.MinValue; int i = 0; return MailBnfHelper.ReadDateTime(dtValue, ref i); } set { Parameters["creation-date"] = MailBnfHelper.GetDateTimeString(value, null); } } ////// Gets the value of the Modification-Date parameter. /// public DateTime ModificationDate { get { string dtValue = Parameters["modification-date"]; if (dtValue == null) return DateTime.MinValue; int i = 0; return MailBnfHelper.ReadDateTime(dtValue, ref i); } set { Parameters["modification-date"] = MailBnfHelper.GetDateTimeString(value, null); } } public bool Inline { get { return (dispositionType == DispositionTypeNames.Inline); } set { isChanged = true; if (value) { dispositionType = DispositionTypeNames.Inline; } else { dispositionType = DispositionTypeNames.Attachment; } } } ////// Gets the value of the Read-Date parameter. /// public DateTime ReadDate { get { string dtValue = Parameters["read-date"]; if (dtValue == null) return DateTime.MinValue; int i = 0; return MailBnfHelper.ReadDateTime(dtValue, ref i); } set { Parameters["read-date"] = MailBnfHelper.GetDateTimeString(value, null); } } ////// Gets the value of the Size parameter (-1 if unspecified). /// public long Size { get { string sizeValue = Parameters["size"]; if (sizeValue == null) return -1; else return long.Parse(sizeValue, CultureInfo.InvariantCulture); } set { Parameters["size"] = value.ToString(CultureInfo.InvariantCulture); } } internal void Set(string contentDisposition, HeaderCollection headers) { //we don't set ischanged because persistence was already handled //via the headers. disposition = contentDisposition; ParseValue(); headers.InternalSet(MailHeaderInfo.GetString(MailHeaderID.ContentDisposition), ToString()); isPersisted = true; } internal void PersistIfNeeded(HeaderCollection headers, bool forcePersist) { if (IsChanged || !isPersisted || forcePersist) { headers.InternalSet(MailHeaderInfo.GetString(MailHeaderID.ContentDisposition), ToString()); isPersisted = true; } } internal bool IsChanged { get { return (isChanged || parameters != null && parameters.IsChanged); } } public override string ToString() { if (disposition == null || isChanged || parameters != null && parameters.IsChanged) { StringBuilder builder = new StringBuilder(); builder.Append(dispositionType); foreach (string key in Parameters.Keys) { builder.Append("; "); builder.Append(key); builder.Append('='); MailBnfHelper.GetTokenOrQuotedString(parameters[key], builder); } disposition = builder.ToString(); isChanged = false; parameters.IsChanged = false; isPersisted = false; } return disposition; } public override bool Equals(object rparam) { if (rparam == null) { return false; } return (String.Compare(ToString(), rparam.ToString(), StringComparison.OrdinalIgnoreCase ) == 0); } public override int GetHashCode(){ return ToString().GetHashCode(); } void ParseValue() { int offset = 0; parameters = new TrackingStringDictionary(); Exception exception = null; try{ dispositionType = MailBnfHelper.ReadToken(disposition, ref offset, null); if(dispositionType == null || dispositionType.Length == 0){ exception = new FormatException(SR.GetString(SR.MailHeaderFieldInvalidCharacter)); } if (exception == null) { while (MailBnfHelper.SkipCFWS(disposition, ref offset)) { if (disposition[offset++] != ';') exception = new FormatException(SR.GetString(SR.MailHeaderFieldInvalidCharacter)); if (!MailBnfHelper.SkipCFWS(disposition, ref offset)) break; string paramAttribute = MailBnfHelper.ReadParameterAttribute(disposition, ref offset, null); string paramValue; if (disposition[offset++] != '='){ exception = new FormatException(SR.GetString(SR.MailHeaderFieldMalformedHeader)); break; } if (!MailBnfHelper.SkipCFWS(disposition, ref offset)) paramValue = string.Empty; else if (disposition[offset] == '"') paramValue = MailBnfHelper.ReadQuotedString(disposition, ref offset, null); else paramValue = MailBnfHelper.ReadToken(disposition, ref offset, null); if(paramAttribute == null || paramValue == null || paramAttribute.Length == 0 || paramValue.Length == 0){ exception = new FormatException(SR.GetString(SR.ContentDispositionInvalid)); break; } //validate date-time strings if(String.Compare(paramAttribute,"creation-date",StringComparison.OrdinalIgnoreCase) == 0 || String.Compare(paramAttribute,"modification-date",StringComparison.OrdinalIgnoreCase) == 0 || String.Compare(paramAttribute,"read-date",StringComparison.OrdinalIgnoreCase) == 0 ){ int i = 0; MailBnfHelper.ReadDateTime(paramValue, ref i); } parameters.Add(paramAttribute, paramValue); } } } catch(FormatException){ throw new FormatException(SR.GetString(SR.ContentDispositionInvalid)); } if (exception != null) { throw exception; } parameters.IsChanged = false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.Mime { using System; using System.Collections; using System.Collections.Specialized; using System.IO; using System.Text; using System.Globalization; using System.Net.Mail; public class ContentDisposition { string dispositionType; TrackingStringDictionary parameters; bool isChanged; bool isPersisted; string disposition; public ContentDisposition() { isChanged = true; disposition = "attachment"; ParseValue(); } ////// ctor. /// /// Unparsed header value. public ContentDisposition(string disposition) { if (disposition == null) throw new ArgumentNullException("disposition"); isChanged = true; this.disposition = disposition; ParseValue(); } ////// Gets the disposition type of the content. /// public string DispositionType { get { return dispositionType; } set { if (value == null) { throw new ArgumentNullException("value"); } if (value == string.Empty) { throw new ArgumentException(SR.GetString(SR.net_emptystringset), "value"); } isChanged = true; dispositionType = value; } } public StringDictionary Parameters { get { if (parameters == null) { parameters = new TrackingStringDictionary(); } return parameters; } } ////// Gets the value of the Filename parameter. /// public string FileName { get { return Parameters["filename"]; } set { if (value == null || value == string.Empty) { Parameters.Remove("filename"); } else{ Parameters["filename"] = value; } } } ////// Gets the value of the Creation-Date parameter. /// public DateTime CreationDate { get { string dtValue = Parameters["creation-date"]; if (dtValue == null) return DateTime.MinValue; int i = 0; return MailBnfHelper.ReadDateTime(dtValue, ref i); } set { Parameters["creation-date"] = MailBnfHelper.GetDateTimeString(value, null); } } ////// Gets the value of the Modification-Date parameter. /// public DateTime ModificationDate { get { string dtValue = Parameters["modification-date"]; if (dtValue == null) return DateTime.MinValue; int i = 0; return MailBnfHelper.ReadDateTime(dtValue, ref i); } set { Parameters["modification-date"] = MailBnfHelper.GetDateTimeString(value, null); } } public bool Inline { get { return (dispositionType == DispositionTypeNames.Inline); } set { isChanged = true; if (value) { dispositionType = DispositionTypeNames.Inline; } else { dispositionType = DispositionTypeNames.Attachment; } } } ////// Gets the value of the Read-Date parameter. /// public DateTime ReadDate { get { string dtValue = Parameters["read-date"]; if (dtValue == null) return DateTime.MinValue; int i = 0; return MailBnfHelper.ReadDateTime(dtValue, ref i); } set { Parameters["read-date"] = MailBnfHelper.GetDateTimeString(value, null); } } ////// Gets the value of the Size parameter (-1 if unspecified). /// public long Size { get { string sizeValue = Parameters["size"]; if (sizeValue == null) return -1; else return long.Parse(sizeValue, CultureInfo.InvariantCulture); } set { Parameters["size"] = value.ToString(CultureInfo.InvariantCulture); } } internal void Set(string contentDisposition, HeaderCollection headers) { //we don't set ischanged because persistence was already handled //via the headers. disposition = contentDisposition; ParseValue(); headers.InternalSet(MailHeaderInfo.GetString(MailHeaderID.ContentDisposition), ToString()); isPersisted = true; } internal void PersistIfNeeded(HeaderCollection headers, bool forcePersist) { if (IsChanged || !isPersisted || forcePersist) { headers.InternalSet(MailHeaderInfo.GetString(MailHeaderID.ContentDisposition), ToString()); isPersisted = true; } } internal bool IsChanged { get { return (isChanged || parameters != null && parameters.IsChanged); } } public override string ToString() { if (disposition == null || isChanged || parameters != null && parameters.IsChanged) { StringBuilder builder = new StringBuilder(); builder.Append(dispositionType); foreach (string key in Parameters.Keys) { builder.Append("; "); builder.Append(key); builder.Append('='); MailBnfHelper.GetTokenOrQuotedString(parameters[key], builder); } disposition = builder.ToString(); isChanged = false; parameters.IsChanged = false; isPersisted = false; } return disposition; } public override bool Equals(object rparam) { if (rparam == null) { return false; } return (String.Compare(ToString(), rparam.ToString(), StringComparison.OrdinalIgnoreCase ) == 0); } public override int GetHashCode(){ return ToString().GetHashCode(); } void ParseValue() { int offset = 0; parameters = new TrackingStringDictionary(); Exception exception = null; try{ dispositionType = MailBnfHelper.ReadToken(disposition, ref offset, null); if(dispositionType == null || dispositionType.Length == 0){ exception = new FormatException(SR.GetString(SR.MailHeaderFieldInvalidCharacter)); } if (exception == null) { while (MailBnfHelper.SkipCFWS(disposition, ref offset)) { if (disposition[offset++] != ';') exception = new FormatException(SR.GetString(SR.MailHeaderFieldInvalidCharacter)); if (!MailBnfHelper.SkipCFWS(disposition, ref offset)) break; string paramAttribute = MailBnfHelper.ReadParameterAttribute(disposition, ref offset, null); string paramValue; if (disposition[offset++] != '='){ exception = new FormatException(SR.GetString(SR.MailHeaderFieldMalformedHeader)); break; } if (!MailBnfHelper.SkipCFWS(disposition, ref offset)) paramValue = string.Empty; else if (disposition[offset] == '"') paramValue = MailBnfHelper.ReadQuotedString(disposition, ref offset, null); else paramValue = MailBnfHelper.ReadToken(disposition, ref offset, null); if(paramAttribute == null || paramValue == null || paramAttribute.Length == 0 || paramValue.Length == 0){ exception = new FormatException(SR.GetString(SR.ContentDispositionInvalid)); break; } //validate date-time strings if(String.Compare(paramAttribute,"creation-date",StringComparison.OrdinalIgnoreCase) == 0 || String.Compare(paramAttribute,"modification-date",StringComparison.OrdinalIgnoreCase) == 0 || String.Compare(paramAttribute,"read-date",StringComparison.OrdinalIgnoreCase) == 0 ){ int i = 0; MailBnfHelper.ReadDateTime(paramValue, ref i); } parameters.Add(paramAttribute, paramValue); } } } catch(FormatException){ throw new FormatException(SR.GetString(SR.ContentDispositionInvalid)); } if (exception != null) { throw exception; } parameters.IsChanged = false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
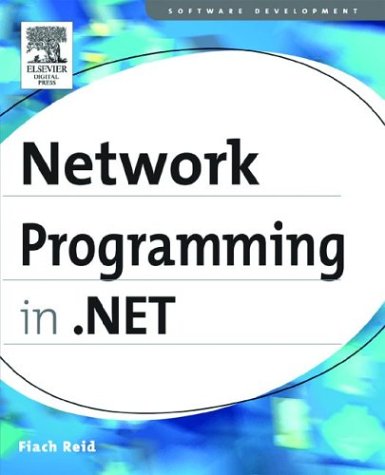
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolboxBitmapAttribute.cs
- SmtpFailedRecipientException.cs
- SqlWriter.cs
- SystemWebCachingSectionGroup.cs
- XamlWriter.cs
- XmlComplianceUtil.cs
- SafeEventLogReadHandle.cs
- DataListItemEventArgs.cs
- _Rfc2616CacheValidators.cs
- TreeNode.cs
- WrappedReader.cs
- SqlClientFactory.cs
- ClientScriptManagerWrapper.cs
- SecurityException.cs
- HttpDictionary.cs
- WindowsTab.cs
- SmtpNegotiateAuthenticationModule.cs
- KnownTypeAttribute.cs
- TextWriter.cs
- SrgsToken.cs
- CommentEmitter.cs
- BulletedListEventArgs.cs
- SmtpMail.cs
- RemoveFromCollection.cs
- RemotingException.cs
- AdRotator.cs
- WebPartDisplayModeCollection.cs
- PermissionSetTriple.cs
- Highlights.cs
- ProvideValueServiceProvider.cs
- DiagnosticsConfiguration.cs
- MemberJoinTreeNode.cs
- FileLogRecordEnumerator.cs
- InvalidateEvent.cs
- ValidationRule.cs
- WebZone.cs
- ButtonRenderer.cs
- StateChangeEvent.cs
- XmlNullResolver.cs
- XXXInfos.cs
- StackOverflowException.cs
- ActivityCodeDomSerializationManager.cs
- SerialErrors.cs
- StorageConditionPropertyMapping.cs
- UnsafeNativeMethods.cs
- BaseDataBoundControl.cs
- UIHelper.cs
- DomainLiteralReader.cs
- QualificationDataItem.cs
- InputLanguageCollection.cs
- XmlDataSourceView.cs
- DSACryptoServiceProvider.cs
- MailBnfHelper.cs
- SByteConverter.cs
- XsltOutput.cs
- Tile.cs
- InputDevice.cs
- CodeMemberEvent.cs
- StateMachineSubscription.cs
- SynchronizedDispatch.cs
- OrderedParallelQuery.cs
- Cursor.cs
- MasterPageCodeDomTreeGenerator.cs
- DriveInfo.cs
- SharedPerformanceCounter.cs
- ImageKeyConverter.cs
- StructuralCache.cs
- MDIWindowDialog.cs
- RecordManager.cs
- ToolStripItemDesigner.cs
- TextSelectionHelper.cs
- CookieParameter.cs
- ToolStripSeparator.cs
- EventLogEntry.cs
- MergeLocalizationDirectives.cs
- CollectionChangedEventManager.cs
- OpenTypeCommon.cs
- StickyNoteAnnotations.cs
- RemoteCryptoDecryptRequest.cs
- Size.cs
- SqlNodeAnnotations.cs
- SerializationFieldInfo.cs
- MachineKeyValidationConverter.cs
- PageContentAsyncResult.cs
- TableLayoutRowStyleCollection.cs
- WinEventHandler.cs
- DependencyPropertyKey.cs
- DisableDpiAwarenessAttribute.cs
- MachineKeySection.cs
- LogReserveAndAppendState.cs
- AncestorChangedEventArgs.cs
- TextRangeProviderWrapper.cs
- SamlAttribute.cs
- ReflectTypeDescriptionProvider.cs
- WindowsFormsSectionHandler.cs
- TabItem.cs
- PixelShader.cs
- EmptyEnumerator.cs
- TextEditor.cs
- ProtectedConfigurationSection.cs