Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / ToolboxBitmapAttribute.cs / 1305376 / ToolboxBitmapAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.IO; using Microsoft.Win32; using System.Runtime.InteropServices; using System.Globalization; using System.Runtime.Versioning; ////// /// ToolboxBitmapAttribute defines the images associated with /// a specified component. The component can offer a small /// and large image (large is optional). /// /// [AttributeUsage(AttributeTargets.Class)] public class ToolboxBitmapAttribute : Attribute { ////// /// The small image for this component /// private Image smallImage; ////// /// The large image for this component. /// private Image largeImage; ////// /// The default size of the large image. /// private static readonly Point largeDim = new Point(32, 32); ////// /// The default size of the large image. /// private static readonly Point smallDim = new Point(16, 16); ////// /// Constructs a new ToolboxBitmapAttribute. /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public ToolboxBitmapAttribute(string imageFile) : this(GetImageFromFile(imageFile, false), GetImageFromFile(imageFile, true)) { } ////// /// Constructs a new ToolboxBitmapAttribute. /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public ToolboxBitmapAttribute(Type t) : this(GetImageFromResource(t, null, false), GetImageFromResource(t, null, true)) { } ////// /// Constructs a new ToolboxBitmapAttribute. /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public ToolboxBitmapAttribute(Type t, string name) : this(GetImageFromResource(t, name, false), GetImageFromResource(t, name, true)) { } ////// /// Constructs a new ToolboxBitmapAttribute. /// private ToolboxBitmapAttribute(Image smallImage, Image largeImage) { this.smallImage = smallImage; this.largeImage = largeImage; } ////// /// public override bool Equals(object value) { if (value == this) { return true; } ToolboxBitmapAttribute attr = value as ToolboxBitmapAttribute; if (attr != null) { return attr.smallImage == smallImage && attr.largeImage == largeImage; } return false; } ///[To be supplied.] ////// /// public override int GetHashCode() { return base.GetHashCode(); } ///[To be supplied.] ////// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Image GetImage(object component) { return GetImage(component, true); } ///[To be supplied.] ////// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Image GetImage(object component, bool large) { if (component != null) { return GetImage(component.GetType(), large); } return null; } ///[To be supplied.] ////// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Image GetImage(Type type) { return GetImage(type, false); } ///[To be supplied.] ////// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Image GetImage(Type type, bool large) { return GetImage(type, null, large); } ///[To be supplied.] ////// /// [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public Image GetImage(Type type, string imgName, bool large) { if ((large && largeImage == null) || (!large && smallImage == null)) { Point largeDim = new Point(32, 32); Image img = null; if (large) { img = largeImage; } else { img = smallImage; } if (img == null) { img = GetImageFromResource(type, imgName, large); } //last resort for large images. if (large && largeImage == null && smallImage != null) { img = new Bitmap((Bitmap)smallImage, largeDim.X, largeDim.Y); } Bitmap b = img as Bitmap; if (b != null) { MakeBackgroundAlphaZero(b); } if (img == null) { img = DefaultComponent.GetImage(type, large); } if (large) { largeImage = img; } else { smallImage = img; } } Image toReturn = (large) ? largeImage : smallImage; if (this.Equals(Default)) { largeImage = null; smallImage = null; } return toReturn; } //helper to get the right icon from the given stream that represents an icon [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] private static Image GetIconFromStream(Stream stream, bool large) { if (stream == null) { return null; } Icon ico = new Icon(stream); Icon sizedico = new Icon(ico, large ? new Size(largeDim.X, largeDim.Y):new Size(smallDim.X, smallDim.Y)); return sizedico.ToBitmap(); } // Just forwards to Image.FromFile eating any non-critical exceptions that may result. [SuppressMessage("Microsoft.Design", "CA1031:DoNotCatchGeneralExceptionTypes")] [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] private static Image GetImageFromFile(string imageFile, bool large) { Image image = null; try { if (imageFile != null) { string ext = Path.GetExtension(imageFile); if (ext != null && string.Equals(ext, ".ico", StringComparison.OrdinalIgnoreCase)) { //ico files support both large and small, so we respec the large flag here. FileStream reader = System.IO.File.Open(imageFile, FileMode.Open); if (reader != null) { try { image = GetIconFromStream(reader, large); } finally { reader.Close(); } } } else if (!large) { //we only read small from non-ico files. image = Image.FromFile(imageFile); } } } catch (Exception e) { if (ClientUtils.IsCriticalException(e)) { throw; } Debug.Fail("Failed to load toolbox image '" + imageFile + "':\r\n" + e.ToString()); } return image; } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] static private Image GetBitmapFromResource(Type t, string bitmapname, bool large) { if (bitmapname == null) { return null; } Image img = null; // load the image from the manifest resources. // Stream stream = t.Module.Assembly.GetManifestResourceStream(t, bitmapname); if (stream != null) { Bitmap b = new Bitmap(stream); img = b; MakeBackgroundAlphaZero(b); if (large) { img = new Bitmap(b, largeDim.X, largeDim.Y); } } return img; } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] static private Image GetIconFromResource(Type t, string bitmapname, bool large) { if (bitmapname == null) { return null; } return GetIconFromStream(t.Module.Assembly.GetManifestResourceStream(t, bitmapname), large); } ///[To be supplied.] ////// /// [SuppressMessage("Microsoft.Design", "CA1031:DoNotCatchGeneralExceptionTypes")] [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] public static Image GetImageFromResource(Type t, string imageName, bool large) { Image img = null; try { string name = imageName; string iconname = null; string bmpname = null; string rawbmpname = null; // if we didn't get a name, use the class name // if (name == null) { name = t.FullName; int indexDot = name.LastIndexOf('.'); if (indexDot != -1) { name = name.Substring(indexDot + 1); } iconname = name + ".ico"; bmpname = name + ".bmp"; } else { if (String.Compare(Path.GetExtension(imageName), ".ico", true, CultureInfo.CurrentCulture) == 0) { iconname = name; } else if (String.Compare(Path.GetExtension(imageName), ".bmp", true, CultureInfo.CurrentCulture) == 0) { bmpname = name; } else { //we dont recognize the name as either bmp or ico. we need to try three things. //1. the name as a bitmap (back compat) //2. name+.bmp //3. name+.ico rawbmpname = name; bmpname = name + ".bmp"; iconname = name + ".ico"; } } img = GetBitmapFromResource(t, rawbmpname, large); if (img == null) { img = GetBitmapFromResource(t, bmpname, large); } if (img == null) { img = GetIconFromResource(t, iconname, large); } } catch (Exception e) { if (t == null) { Debug.Fail("Failed to load toolbox image for null type:\r\n" + e.ToString()); } else { Debug.Fail("Failed to load toolbox image for '" + t.FullName + "':\r\n" + e.ToString()); } } return img; } private static void MakeBackgroundAlphaZero(Bitmap img) { Color bottomLeft = img.GetPixel(0, img.Height - 1); img.MakeTransparent(); Color newBottomLeft = Color.FromArgb(0, bottomLeft); img.SetPixel(0, img.Height - 1, newBottomLeft); } ///[To be supplied.] ////// /// Default name is null /// [SuppressMessage("Microsoft.Security", "CA2104:DoNotDeclareReadOnlyMutableReferenceTypes")] public static readonly ToolboxBitmapAttribute Default = new ToolboxBitmapAttribute((Image)null, (Image)null); private static readonly ToolboxBitmapAttribute DefaultComponent; [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] static ToolboxBitmapAttribute() { //Fix for Dev10 560430. When we call Gdip.DummyFunction, JIT will make sure Gdip..cctor will be called before SafeNativeMethods.Gdip.DummyFunction(); Bitmap bitmap = null; Stream stream = typeof(ToolboxBitmapAttribute).Module.Assembly.GetManifestResourceStream(typeof(ToolboxBitmapAttribute), "DefaultComponent.bmp"); if (stream != null) { bitmap = new Bitmap(stream); MakeBackgroundAlphaZero(bitmap); } DefaultComponent = new ToolboxBitmapAttribute(bitmap, null); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
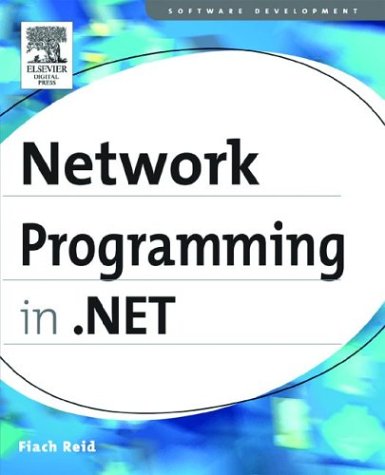
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigurationErrorsException.cs
- RoleManagerModule.cs
- ServiceEndpointAssociationProvider.cs
- EllipticalNodeOperations.cs
- TextOutput.cs
- WsdlServiceChannelBuilder.cs
- HatchBrush.cs
- DrawingGroup.cs
- GeometryConverter.cs
- TypeReference.cs
- Errors.cs
- ADConnectionHelper.cs
- TraceHandler.cs
- XmlSchemaComplexContentRestriction.cs
- ProcessManager.cs
- ClassHandlersStore.cs
- StateRuntime.cs
- SqlBuffer.cs
- NavigationService.cs
- SourceFileBuildProvider.cs
- TempFiles.cs
- AuthStoreRoleProvider.cs
- ParallelTimeline.cs
- ParamArrayAttribute.cs
- SafeProcessHandle.cs
- Ticks.cs
- TraceSection.cs
- JavaScriptString.cs
- MouseGestureValueSerializer.cs
- InputLanguageCollection.cs
- MessageSmuggler.cs
- ArrayExtension.cs
- ExceptionUtil.cs
- ChtmlTextWriter.cs
- UTF32Encoding.cs
- PerfCounters.cs
- HtmlUtf8RawTextWriter.cs
- TransactionOptions.cs
- ExpressionBuilder.cs
- DocumentPageView.cs
- CodeAccessPermission.cs
- CuspData.cs
- SafeBitVector32.cs
- XmlElement.cs
- IIS7UserPrincipal.cs
- ValidationManager.cs
- ReadOnlyCollection.cs
- XmlWriterTraceListener.cs
- FunctionMappingTranslator.cs
- FlatButtonAppearance.cs
- TypeConverterValueSerializer.cs
- Span.cs
- DelayedRegex.cs
- COSERVERINFO.cs
- GZipStream.cs
- FixedSchema.cs
- PublishLicense.cs
- DiagnosticsConfiguration.cs
- BasicViewGenerator.cs
- Nullable.cs
- XmlAtomicValue.cs
- SafeRegistryHandle.cs
- StorageFunctionMapping.cs
- TcpAppDomainProtocolHandler.cs
- IdentifierService.cs
- CodeDirectiveCollection.cs
- DocumentPageView.cs
- ClockGroup.cs
- ZipFileInfoCollection.cs
- URL.cs
- StorageSetMapping.cs
- ExpressionBuilderCollection.cs
- WebPartCloseVerb.cs
- EntityReference.cs
- Pointer.cs
- SqlConnectionFactory.cs
- AssociationSetMetadata.cs
- RowToFieldTransformer.cs
- ZoomPercentageConverter.cs
- ClientUriBehavior.cs
- HuffModule.cs
- HtmlEncodedRawTextWriter.cs
- DocumentViewer.cs
- ComponentCommands.cs
- StreamReader.cs
- StateBag.cs
- wgx_commands.cs
- SchemaNamespaceManager.cs
- UdpConstants.cs
- DiffuseMaterial.cs
- RelOps.cs
- ServiceModelInstallComponent.cs
- SecurityTokenContainer.cs
- ProxyElement.cs
- WorkflowExecutor.cs
- SelfIssuedAuthRSACryptoProvider.cs
- GeometryValueSerializer.cs
- MDIClient.cs
- UrlAuthFailureHandler.cs
- NotifyCollectionChangedEventArgs.cs