Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Configuration / RuleSettings.cs / 2 / RuleSettings.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.ComponentModel; using System.Web.Hosting; using System.Web.Util; using System.Web.Configuration; using System.Web.Management; using System.Web.Compilation; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class RuleSettings : ConfigurationElement { internal static int DEFAULT_MIN_INSTANCES = 1; internal static int DEFAULT_MAX_LIMIT = int.MaxValue; internal static TimeSpan DEFAULT_MIN_INTERVAL = TimeSpan.Zero; internal static string DEFAULT_CUSTOM_EVAL = null; private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propName = new ConfigurationProperty("name", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propEventName = new ConfigurationProperty("eventName", typeof(string), String.Empty, ConfigurationPropertyOptions.IsRequired); private static readonly ConfigurationProperty _propProvider = new ConfigurationProperty("provider", typeof(string), String.Empty, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProfile = new ConfigurationProperty("profile", typeof(string), String.Empty, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMinInstances = new ConfigurationProperty("minInstances", typeof(int), DEFAULT_MIN_INSTANCES, null, StdValidatorsAndConverters.NonZeroPositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxLimit = new ConfigurationProperty("maxLimit", typeof(int), DEFAULT_MAX_LIMIT, new InfiniteIntConverter(), StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMinInterval = new ConfigurationProperty("minInterval", typeof(TimeSpan), DEFAULT_MIN_INTERVAL, StdValidatorsAndConverters.InfiniteTimeSpanConverter, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCustom = new ConfigurationProperty("custom", typeof(string), String.Empty, ConfigurationPropertyOptions.None); static RuleSettings() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propName); _properties.Add(_propEventName); _properties.Add(_propProvider); _properties.Add(_propProfile); _properties.Add(_propMinInstances); _properties.Add(_propMaxLimit); _properties.Add(_propMinInterval); _properties.Add(_propCustom); } internal RuleSettings() { } public RuleSettings(String name, String eventName, String provider) : this() { Name = name; EventName = eventName; Provider = provider; } public RuleSettings(String name, String eventName, String provider, String profile, int minInstances, int maxLimit, TimeSpan minInterval) : this(name, eventName, provider) { Profile = profile; MinInstances = minInstances; MaxLimit = maxLimit; MinInterval = minInterval; } public RuleSettings(String name, String eventName, String provider, String profile, int minInstances, int maxLimit, TimeSpan minInterval, string custom) : this(name, eventName, provider) { Profile = profile; MinInstances = minInstances; MaxLimit = maxLimit; MinInterval = minInterval; Custom = custom; } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("name", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public String Name { get { return (string)base[_propName]; } set { base[_propName] = value; } } [ConfigurationProperty("eventName", IsRequired = true, DefaultValue = "")] public String EventName { get { return (string)base[_propEventName]; } set { base[_propEventName] = value; } } [ConfigurationProperty("custom", DefaultValue = "")] public String Custom { get { return (string)base[_propCustom]; } set { base[_propCustom] = value; } } [ConfigurationProperty("profile", DefaultValue = "")] public String Profile { get { return (string)base[_propProfile]; } set { base[_propProfile] = value; } } [ConfigurationProperty("provider", DefaultValue = "")] public String Provider { get { return (string)base[_propProvider]; } set { base[_propProvider] = value; } } [ConfigurationProperty("minInstances", DefaultValue = 1)] [IntegerValidator(MinValue = 1)] public int MinInstances { get { return (int)base[_propMinInstances]; } set { base[_propMinInstances] = value; } } [ConfigurationProperty("maxLimit", DefaultValue = int.MaxValue)] [TypeConverter(typeof(InfiniteIntConverter))] [IntegerValidator(MinValue = 0)] public int MaxLimit { get { return (int)base[_propMaxLimit]; } set { base[_propMaxLimit] = value; } } [ConfigurationProperty("minInterval", DefaultValue = "00:00:00")] [TypeConverter(typeof(InfiniteTimeSpanConverter))] public TimeSpan MinInterval { get { return (TimeSpan)base[_propMinInterval]; } set { base[_propMinInterval] = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.ComponentModel; using System.Web.Hosting; using System.Web.Util; using System.Web.Configuration; using System.Web.Management; using System.Web.Compilation; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class RuleSettings : ConfigurationElement { internal static int DEFAULT_MIN_INSTANCES = 1; internal static int DEFAULT_MAX_LIMIT = int.MaxValue; internal static TimeSpan DEFAULT_MIN_INTERVAL = TimeSpan.Zero; internal static string DEFAULT_CUSTOM_EVAL = null; private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propName = new ConfigurationProperty("name", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propEventName = new ConfigurationProperty("eventName", typeof(string), String.Empty, ConfigurationPropertyOptions.IsRequired); private static readonly ConfigurationProperty _propProvider = new ConfigurationProperty("provider", typeof(string), String.Empty, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProfile = new ConfigurationProperty("profile", typeof(string), String.Empty, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMinInstances = new ConfigurationProperty("minInstances", typeof(int), DEFAULT_MIN_INSTANCES, null, StdValidatorsAndConverters.NonZeroPositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMaxLimit = new ConfigurationProperty("maxLimit", typeof(int), DEFAULT_MAX_LIMIT, new InfiniteIntConverter(), StdValidatorsAndConverters.PositiveIntegerValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propMinInterval = new ConfigurationProperty("minInterval", typeof(TimeSpan), DEFAULT_MIN_INTERVAL, StdValidatorsAndConverters.InfiniteTimeSpanConverter, null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCustom = new ConfigurationProperty("custom", typeof(string), String.Empty, ConfigurationPropertyOptions.None); static RuleSettings() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propName); _properties.Add(_propEventName); _properties.Add(_propProvider); _properties.Add(_propProfile); _properties.Add(_propMinInstances); _properties.Add(_propMaxLimit); _properties.Add(_propMinInterval); _properties.Add(_propCustom); } internal RuleSettings() { } public RuleSettings(String name, String eventName, String provider) : this() { Name = name; EventName = eventName; Provider = provider; } public RuleSettings(String name, String eventName, String provider, String profile, int minInstances, int maxLimit, TimeSpan minInterval) : this(name, eventName, provider) { Profile = profile; MinInstances = minInstances; MaxLimit = maxLimit; MinInterval = minInterval; } public RuleSettings(String name, String eventName, String provider, String profile, int minInstances, int maxLimit, TimeSpan minInterval, string custom) : this(name, eventName, provider) { Profile = profile; MinInstances = minInstances; MaxLimit = maxLimit; MinInterval = minInterval; Custom = custom; } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("name", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public String Name { get { return (string)base[_propName]; } set { base[_propName] = value; } } [ConfigurationProperty("eventName", IsRequired = true, DefaultValue = "")] public String EventName { get { return (string)base[_propEventName]; } set { base[_propEventName] = value; } } [ConfigurationProperty("custom", DefaultValue = "")] public String Custom { get { return (string)base[_propCustom]; } set { base[_propCustom] = value; } } [ConfigurationProperty("profile", DefaultValue = "")] public String Profile { get { return (string)base[_propProfile]; } set { base[_propProfile] = value; } } [ConfigurationProperty("provider", DefaultValue = "")] public String Provider { get { return (string)base[_propProvider]; } set { base[_propProvider] = value; } } [ConfigurationProperty("minInstances", DefaultValue = 1)] [IntegerValidator(MinValue = 1)] public int MinInstances { get { return (int)base[_propMinInstances]; } set { base[_propMinInstances] = value; } } [ConfigurationProperty("maxLimit", DefaultValue = int.MaxValue)] [TypeConverter(typeof(InfiniteIntConverter))] [IntegerValidator(MinValue = 0)] public int MaxLimit { get { return (int)base[_propMaxLimit]; } set { base[_propMaxLimit] = value; } } [ConfigurationProperty("minInterval", DefaultValue = "00:00:00")] [TypeConverter(typeof(InfiniteTimeSpanConverter))] public TimeSpan MinInterval { get { return (TimeSpan)base[_propMinInterval]; } set { base[_propMinInterval] = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
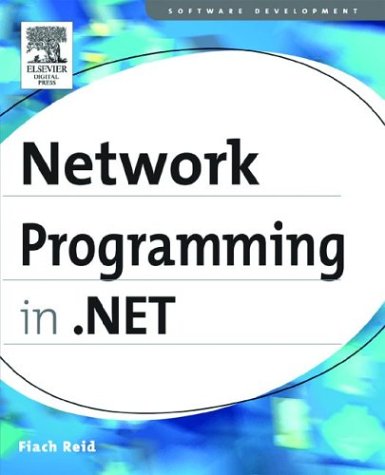
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ASCIIEncoding.cs
- InstanceKeyCompleteException.cs
- MergeLocalizationDirectives.cs
- FactoryId.cs
- ComponentCache.cs
- FontSource.cs
- CodeTypeReference.cs
- SmtpAuthenticationManager.cs
- UMPAttributes.cs
- QilInvoke.cs
- ChannelParameterCollection.cs
- dataSvcMapFileLoader.cs
- XmlSchema.cs
- SqlProviderServices.cs
- XmlArrayItemAttribute.cs
- HMACMD5.cs
- KoreanLunisolarCalendar.cs
- CodeSnippetStatement.cs
- HitTestWithPointDrawingContextWalker.cs
- UnmanagedBitmapWrapper.cs
- ExpressionLink.cs
- dbdatarecord.cs
- SqlDataSourceCommandParser.cs
- PerfCounterSection.cs
- ElementHost.cs
- StrokeNodeEnumerator.cs
- DataServiceProviderMethods.cs
- ObjectCloneHelper.cs
- SecurityCriticalDataForSet.cs
- _NativeSSPI.cs
- DocumentApplicationState.cs
- SchemaType.cs
- EncoderNLS.cs
- StandardRuntimeEnumValidatorAttribute.cs
- _AutoWebProxyScriptEngine.cs
- TheQuery.cs
- DescriptionAttribute.cs
- DesignerTransaction.cs
- TablePattern.cs
- X500Name.cs
- MenuItemStyleCollection.cs
- GPPOINTF.cs
- TextModifierScope.cs
- Select.cs
- SplayTreeNode.cs
- _CommandStream.cs
- TranslateTransform.cs
- SourceInterpreter.cs
- SubclassTypeValidatorAttribute.cs
- CodeBinaryOperatorExpression.cs
- Bitmap.cs
- UpdateManifestForBrowserApplication.cs
- ThreadAttributes.cs
- Tokenizer.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- _ServiceNameStore.cs
- ReadOnlyObservableCollection.cs
- RemotingAttributes.cs
- WebPartHelpVerb.cs
- StackOverflowException.cs
- AlphabeticalEnumConverter.cs
- Html32TextWriter.cs
- Vertex.cs
- ContainerSelectorActiveEvent.cs
- PipelineModuleStepContainer.cs
- HandlerFactoryCache.cs
- InputProcessorProfiles.cs
- ControlCodeDomSerializer.cs
- DragDeltaEventArgs.cs
- AppDomainFactory.cs
- XmlSchemaFacet.cs
- EventHandlersStore.cs
- _Semaphore.cs
- TransportContext.cs
- ColumnClickEvent.cs
- DebugView.cs
- SamlAuthorityBinding.cs
- TimeStampChecker.cs
- VisualStyleTypesAndProperties.cs
- NonClientArea.cs
- XmlSerializerNamespaces.cs
- LayoutManager.cs
- CroppedBitmap.cs
- DataGridViewElement.cs
- CommandDevice.cs
- XmlNamespaceDeclarationsAttribute.cs
- ModifiableIteratorCollection.cs
- SqlDataReader.cs
- LicenseException.cs
- SHA384.cs
- RenderDataDrawingContext.cs
- TextRange.cs
- PrintDocument.cs
- XmlUnspecifiedAttribute.cs
- SystemInfo.cs
- AddDataControlFieldDialog.cs
- XmlSchemaInferenceException.cs
- IconConverter.cs
- DataPagerField.cs
- SuppressIldasmAttribute.cs