Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / WebControls / RadioButton.cs / 1 / RadioButton.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Web; using System.Web.UI; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; ////// [ Designer("System.Web.UI.Design.WebControls.CheckBoxDesigner, " + AssemblyRef.SystemDesign), SupportsEventValidation, ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class RadioButton : CheckBox, IPostBackDataHandler { #if SHIPPINGADAPTERS internal static readonly string AltSelectedText = "(o)"; internal static readonly string AltUnselectedText = "(_)"; #endif private string _uniqueGroupName = null; ///Constructs a radio button and defines its /// properties. ////// public RadioButton() { } ///Initializes a new instance of the ///class. /// [ DefaultValue(""), WebCategory("Behavior"), WebSysDescription(SR.RadioButton_GroupName), Themeable(false), ] public virtual string GroupName { get { string s = (string)ViewState["GroupName"]; return((s == null) ? String.Empty : s); } set { ViewState["GroupName"] = value; } } // Fully qualified GroupName for rendering purposes, to take care of conflicts // between different naming containers internal string UniqueGroupName { get { if (_uniqueGroupName == null) { // For radio buttons, we must make the groupname unique, but can't just use the // UniqueID because all buttons in a group must have the same name. So // we replace the last part of the UniqueID with the group Name. string name = GroupName; string uid = UniqueID; if (uid != null) { int lastColon = uid.LastIndexOf(IdSeparator); if (lastColon >= 0) { if (name.Length > 0) { name = uid.Substring(0, lastColon+1) + name; } else if (NamingContainer is RadioButtonList) { // If GroupName is not set we simply use the naming // container as the group name name = uid.Substring(0, lastColon); } } if (name.Length == 0) { name = uid; } } _uniqueGroupName = name; } return _uniqueGroupName; } } ///Gets or /// sets the name of the group that the radio button belongs to. ////// internal string ValueAttribute { get { string valueAttr = Attributes["value"]; if (valueAttr == null) { // VSWhidbey 146829. Always EnsureID so the valueAttribute will not change by // call to ClientID which happens during Render. EnsureID(); if (ID != null) valueAttr = ID; else valueAttr = UniqueID; } return valueAttr; } } ////// /// bool IPostBackDataHandler.LoadPostData(String postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } ///Method of IPostBackDataHandler interface to process posted data. /// RadioButton determines the posted radio group state. ////// /// protected override bool LoadPostData(String postDataKey, NameValueCollection postCollection) { string post = postCollection[UniqueGroupName]; bool valueChanged = false; if ((post != null) && post.Equals(ValueAttribute)) { ValidateEvent(UniqueGroupName, post); if (Checked == false) { Checked = true; // only fire change event for RadioButton that is being checked valueChanged = true; } } else { if (Checked == true) { Checked = false; } } return valueChanged; } ///Method of IPostBackDataHandler interface to process posted data. /// RadioButton determines the posted radio group state. ////// /// Raises when posted data for a control has changed. /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } ////// /// Raises when posted data for a control has changed. /// protected override void RaisePostDataChangedEvent() { if (AutoPostBack && !Page.IsPostBackEventControlRegistered) { // VSWhidbey 204824 Page.AutoPostBackControl = this; if (CausesValidation) { Page.Validate(ValidationGroup); } } OnCheckedChanged(EventArgs.Empty); } ////// /// This method is invoked just prior to rendering. /// Register client script for handling postback if onChangeHandler is set. /// protected internal override void OnPreRender(EventArgs e) { // must call CheckBox PreRender base.OnPreRender(e); if (Page != null && !Checked && Enabled) { Page.RegisterRequiresPostBack(this); } } internal override void RenderInputTag(HtmlTextWriter writer, string clientID, string onClick) { writer.AddAttribute(HtmlTextWriterAttribute.Id, clientID); writer.AddAttribute(HtmlTextWriterAttribute.Type, "radio"); writer.AddAttribute(HtmlTextWriterAttribute.Name, UniqueGroupName); writer.AddAttribute(HtmlTextWriterAttribute.Value, ValueAttribute); if (Page != null) { Page.ClientScript.RegisterForEventValidation(UniqueGroupName, ValueAttribute); } if (Checked) writer.AddAttribute(HtmlTextWriterAttribute.Checked, "checked"); // ASURT 119141: Render disabled attribute on the INPUT tag (instead of the SPAN) so the checkbox actually gets disabled when Enabled=false if (!IsEnabled) { writer.AddAttribute(HtmlTextWriterAttribute.Disabled, "disabled"); } // We don't do autopostback if the radio button has been selected. // This is to make it consistent that it only posts back if its // state has been changed. Also, it avoids the problem of missing // validation since the data changed event would not be fired if the // selected radio button was posting back. if (AutoPostBack && !Checked && Page != null) { PostBackOptions options = new PostBackOptions(this, String.Empty); // ASURT 98368 // Need to merge the autopostback script with the user script if (CausesValidation) { options.PerformValidation = true; options.ValidationGroup = ValidationGroup; } if (Page.Form != null) { options.AutoPostBack = true; } onClick = Util.MergeScript(onClick, Page.ClientScript.GetPostBackEventReference(options)); writer.AddAttribute(HtmlTextWriterAttribute.Onclick, onClick); if (EnableLegacyRendering) { writer.AddAttribute("language", "javascript", false); } } else { if (onClick != null) { writer.AddAttribute(HtmlTextWriterAttribute.Onclick, onClick); } } string s = AccessKey; if (s.Length > 0) writer.AddAttribute(HtmlTextWriterAttribute.Accesskey, s); int i = TabIndex; if (i != 0) { writer.AddAttribute(HtmlTextWriterAttribute.Tabindex, i.ToString(NumberFormatInfo.InvariantInfo)); } if (_inputAttributes != null && _inputAttributes.Count != 0) { _inputAttributes.AddAttributes(writer); } writer.RenderBeginTag(HtmlTextWriterTag.Input); writer.RenderEndTag(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Web; using System.Web.UI; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Globalization; using System.Security.Permissions; ////// [ Designer("System.Web.UI.Design.WebControls.CheckBoxDesigner, " + AssemblyRef.SystemDesign), SupportsEventValidation, ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class RadioButton : CheckBox, IPostBackDataHandler { #if SHIPPINGADAPTERS internal static readonly string AltSelectedText = "(o)"; internal static readonly string AltUnselectedText = "(_)"; #endif private string _uniqueGroupName = null; ///Constructs a radio button and defines its /// properties. ////// public RadioButton() { } ///Initializes a new instance of the ///class. /// [ DefaultValue(""), WebCategory("Behavior"), WebSysDescription(SR.RadioButton_GroupName), Themeable(false), ] public virtual string GroupName { get { string s = (string)ViewState["GroupName"]; return((s == null) ? String.Empty : s); } set { ViewState["GroupName"] = value; } } // Fully qualified GroupName for rendering purposes, to take care of conflicts // between different naming containers internal string UniqueGroupName { get { if (_uniqueGroupName == null) { // For radio buttons, we must make the groupname unique, but can't just use the // UniqueID because all buttons in a group must have the same name. So // we replace the last part of the UniqueID with the group Name. string name = GroupName; string uid = UniqueID; if (uid != null) { int lastColon = uid.LastIndexOf(IdSeparator); if (lastColon >= 0) { if (name.Length > 0) { name = uid.Substring(0, lastColon+1) + name; } else if (NamingContainer is RadioButtonList) { // If GroupName is not set we simply use the naming // container as the group name name = uid.Substring(0, lastColon); } } if (name.Length == 0) { name = uid; } } _uniqueGroupName = name; } return _uniqueGroupName; } } ///Gets or /// sets the name of the group that the radio button belongs to. ////// internal string ValueAttribute { get { string valueAttr = Attributes["value"]; if (valueAttr == null) { // VSWhidbey 146829. Always EnsureID so the valueAttribute will not change by // call to ClientID which happens during Render. EnsureID(); if (ID != null) valueAttr = ID; else valueAttr = UniqueID; } return valueAttr; } } ////// /// bool IPostBackDataHandler.LoadPostData(String postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } ///Method of IPostBackDataHandler interface to process posted data. /// RadioButton determines the posted radio group state. ////// /// protected override bool LoadPostData(String postDataKey, NameValueCollection postCollection) { string post = postCollection[UniqueGroupName]; bool valueChanged = false; if ((post != null) && post.Equals(ValueAttribute)) { ValidateEvent(UniqueGroupName, post); if (Checked == false) { Checked = true; // only fire change event for RadioButton that is being checked valueChanged = true; } } else { if (Checked == true) { Checked = false; } } return valueChanged; } ///Method of IPostBackDataHandler interface to process posted data. /// RadioButton determines the posted radio group state. ////// /// Raises when posted data for a control has changed. /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } ////// /// Raises when posted data for a control has changed. /// protected override void RaisePostDataChangedEvent() { if (AutoPostBack && !Page.IsPostBackEventControlRegistered) { // VSWhidbey 204824 Page.AutoPostBackControl = this; if (CausesValidation) { Page.Validate(ValidationGroup); } } OnCheckedChanged(EventArgs.Empty); } ////// /// This method is invoked just prior to rendering. /// Register client script for handling postback if onChangeHandler is set. /// protected internal override void OnPreRender(EventArgs e) { // must call CheckBox PreRender base.OnPreRender(e); if (Page != null && !Checked && Enabled) { Page.RegisterRequiresPostBack(this); } } internal override void RenderInputTag(HtmlTextWriter writer, string clientID, string onClick) { writer.AddAttribute(HtmlTextWriterAttribute.Id, clientID); writer.AddAttribute(HtmlTextWriterAttribute.Type, "radio"); writer.AddAttribute(HtmlTextWriterAttribute.Name, UniqueGroupName); writer.AddAttribute(HtmlTextWriterAttribute.Value, ValueAttribute); if (Page != null) { Page.ClientScript.RegisterForEventValidation(UniqueGroupName, ValueAttribute); } if (Checked) writer.AddAttribute(HtmlTextWriterAttribute.Checked, "checked"); // ASURT 119141: Render disabled attribute on the INPUT tag (instead of the SPAN) so the checkbox actually gets disabled when Enabled=false if (!IsEnabled) { writer.AddAttribute(HtmlTextWriterAttribute.Disabled, "disabled"); } // We don't do autopostback if the radio button has been selected. // This is to make it consistent that it only posts back if its // state has been changed. Also, it avoids the problem of missing // validation since the data changed event would not be fired if the // selected radio button was posting back. if (AutoPostBack && !Checked && Page != null) { PostBackOptions options = new PostBackOptions(this, String.Empty); // ASURT 98368 // Need to merge the autopostback script with the user script if (CausesValidation) { options.PerformValidation = true; options.ValidationGroup = ValidationGroup; } if (Page.Form != null) { options.AutoPostBack = true; } onClick = Util.MergeScript(onClick, Page.ClientScript.GetPostBackEventReference(options)); writer.AddAttribute(HtmlTextWriterAttribute.Onclick, onClick); if (EnableLegacyRendering) { writer.AddAttribute("language", "javascript", false); } } else { if (onClick != null) { writer.AddAttribute(HtmlTextWriterAttribute.Onclick, onClick); } } string s = AccessKey; if (s.Length > 0) writer.AddAttribute(HtmlTextWriterAttribute.Accesskey, s); int i = TabIndex; if (i != 0) { writer.AddAttribute(HtmlTextWriterAttribute.Tabindex, i.ToString(NumberFormatInfo.InvariantInfo)); } if (_inputAttributes != null && _inputAttributes.Count != 0) { _inputAttributes.AddAttributes(writer); } writer.RenderBeginTag(HtmlTextWriterTag.Input); writer.RenderEndTag(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
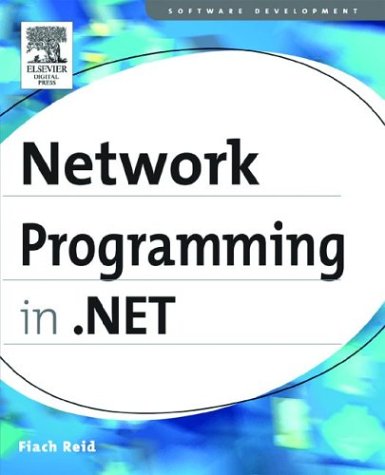
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Int32AnimationUsingKeyFrames.cs
- DependencyProperty.cs
- SHA384.cs
- OleDbConnectionFactory.cs
- StaticSiteMapProvider.cs
- SinglePageViewer.cs
- ValidationRuleCollection.cs
- RoutedUICommand.cs
- MultipleViewProviderWrapper.cs
- PrintDialogException.cs
- TdsRecordBufferSetter.cs
- ToggleProviderWrapper.cs
- MLangCodePageEncoding.cs
- ModelPropertyImpl.cs
- OutputCacheSection.cs
- HtmlTitle.cs
- PolyLineSegment.cs
- StrokeFIndices.cs
- EventLogPermissionEntry.cs
- ValueTable.cs
- AppLevelCompilationSectionCache.cs
- Bits.cs
- DesignTable.cs
- WriteableOnDemandStream.cs
- BCLDebug.cs
- PlainXmlWriter.cs
- ValidationPropertyAttribute.cs
- CodeCommentStatement.cs
- ProgressBarAutomationPeer.cs
- coordinatorscratchpad.cs
- CompiledQuery.cs
- basenumberconverter.cs
- EntityDataReader.cs
- StylusButtonCollection.cs
- AlternateViewCollection.cs
- ListBindingConverter.cs
- StrokeRenderer.cs
- EntityParameter.cs
- ComEventsInfo.cs
- Converter.cs
- WinFormsComponentEditor.cs
- TraceContext.cs
- GridViewSortEventArgs.cs
- ContravarianceAdapter.cs
- HttpCapabilitiesEvaluator.cs
- CollectionMarkupSerializer.cs
- ChannelAcceptor.cs
- RuleAction.cs
- _CommandStream.cs
- invalidudtexception.cs
- PropertyRef.cs
- ToolStripItemClickedEventArgs.cs
- OleDbFactory.cs
- WebServiceTypeData.cs
- PropertiesTab.cs
- InstancePersistenceContext.cs
- _emptywebproxy.cs
- EventKeyword.cs
- ConditionalAttribute.cs
- TextReader.cs
- GradientBrush.cs
- ControlParser.cs
- RectValueSerializer.cs
- SetterBaseCollection.cs
- WebConfigurationHost.cs
- EntityDataSourceStatementEditor.cs
- ReflectPropertyDescriptor.cs
- ScrollData.cs
- ExtendedPropertyDescriptor.cs
- Graphics.cs
- OpenTypeLayoutCache.cs
- DbMetaDataCollectionNames.cs
- XhtmlMobileTextWriter.cs
- OracleParameterCollection.cs
- WindowsListViewItemCheckBox.cs
- ImageListStreamer.cs
- RC2CryptoServiceProvider.cs
- ContainerVisual.cs
- ScriptResourceHandler.cs
- WithParamAction.cs
- ObjectList.cs
- BitmapData.cs
- ToolStripSplitStackLayout.cs
- WindowAutomationPeer.cs
- ActivationServices.cs
- DefinitionUpdate.cs
- VScrollProperties.cs
- PreviewPrintController.cs
- KerberosSecurityTokenAuthenticator.cs
- SQLMembershipProvider.cs
- XPathItem.cs
- MethodBuilderInstantiation.cs
- TemplatedWizardStep.cs
- XpsPartBase.cs
- ResourcePermissionBaseEntry.cs
- ListViewCommandEventArgs.cs
- TextBoxAutomationPeer.cs
- ImmComposition.cs
- Tuple.cs
- PropertyGrid.cs