Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / ManagedLibraries / Security / System / Security / Cryptography / Pkcs / Pkcs7Signer.cs / 1 / Pkcs7Signer.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Pkcs7Signer.cs // namespace System.Security.Cryptography.Pkcs { using System.Globalization; using System.Security.Cryptography; using System.Security.Cryptography.X509Certificates; [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CmsSigner { private SubjectIdentifierType m_signerIdentifierType; private X509Certificate2 m_certificate; private Oid m_digestAlgorithm; private CryptographicAttributeObjectCollection m_signedAttributes; private CryptographicAttributeObjectCollection m_unsignedAttributes; private X509Certificate2Collection m_certificates; private X509IncludeOption m_includeOption; private bool m_dummyCert; // // Constructors. // public CmsSigner () : this(SubjectIdentifierType.IssuerAndSerialNumber, null) {} public CmsSigner (SubjectIdentifierType signerIdentifierType) : this (signerIdentifierType, null) {} public CmsSigner (X509Certificate2 certificate) : this(SubjectIdentifierType.IssuerAndSerialNumber, certificate) {} public CmsSigner (CspParameters parameters) : this(SubjectIdentifierType.SubjectKeyIdentifier, PkcsUtils.CreateDummyCertificate(parameters)) { m_dummyCert = true; this.IncludeOption = X509IncludeOption.None; } public CmsSigner (SubjectIdentifierType signerIdentifierType, X509Certificate2 certificate) { switch (signerIdentifierType) { case SubjectIdentifierType.Unknown: this.SignerIdentifierType = SubjectIdentifierType.IssuerAndSerialNumber; this.IncludeOption = X509IncludeOption.ExcludeRoot; break; case SubjectIdentifierType.IssuerAndSerialNumber: this.SignerIdentifierType = signerIdentifierType; this.IncludeOption = X509IncludeOption.ExcludeRoot; break; case SubjectIdentifierType.SubjectKeyIdentifier: this.SignerIdentifierType = signerIdentifierType; this.IncludeOption = X509IncludeOption.ExcludeRoot; break; case SubjectIdentifierType.NoSignature: this.SignerIdentifierType = signerIdentifierType; this.IncludeOption = X509IncludeOption.None; break; default: this.SignerIdentifierType = SubjectIdentifierType.IssuerAndSerialNumber; this.IncludeOption = X509IncludeOption.ExcludeRoot; break; } this.Certificate = certificate; this.DigestAlgorithm = new Oid(CAPI.szOID_OIWSEC_sha1); m_signedAttributes = new CryptographicAttributeObjectCollection(); m_unsignedAttributes = new CryptographicAttributeObjectCollection(); m_certificates = new X509Certificate2Collection(); } // // Public APIs. // public SubjectIdentifierType SignerIdentifierType { get { return m_signerIdentifierType; } set { if (value != SubjectIdentifierType.IssuerAndSerialNumber && value != SubjectIdentifierType.SubjectKeyIdentifier && value != SubjectIdentifierType.NoSignature) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, SecurityResources.GetResourceString("Arg_EnumIllegalVal"), "value")); if (m_dummyCert && value != SubjectIdentifierType.SubjectKeyIdentifier) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, SecurityResources.GetResourceString("Arg_EnumIllegalVal"), "value")); m_signerIdentifierType = value; } } public X509Certificate2 Certificate { get { return m_certificate; } set { m_certificate = value; } } public Oid DigestAlgorithm { get { return m_digestAlgorithm; } set { m_digestAlgorithm = value; } } public CryptographicAttributeObjectCollection SignedAttributes { get { return m_signedAttributes; } } public CryptographicAttributeObjectCollection UnsignedAttributes { get { return m_unsignedAttributes; } } public X509Certificate2Collection Certificates { get { return m_certificates; } } public X509IncludeOption IncludeOption { get { return m_includeOption; } set { if (value < X509IncludeOption.None || value > X509IncludeOption.WholeChain) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, SecurityResources.GetResourceString("Arg_EnumIllegalVal"), "value")); m_includeOption = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Pkcs7Signer.cs // namespace System.Security.Cryptography.Pkcs { using System.Globalization; using System.Security.Cryptography; using System.Security.Cryptography.X509Certificates; [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CmsSigner { private SubjectIdentifierType m_signerIdentifierType; private X509Certificate2 m_certificate; private Oid m_digestAlgorithm; private CryptographicAttributeObjectCollection m_signedAttributes; private CryptographicAttributeObjectCollection m_unsignedAttributes; private X509Certificate2Collection m_certificates; private X509IncludeOption m_includeOption; private bool m_dummyCert; // // Constructors. // public CmsSigner () : this(SubjectIdentifierType.IssuerAndSerialNumber, null) {} public CmsSigner (SubjectIdentifierType signerIdentifierType) : this (signerIdentifierType, null) {} public CmsSigner (X509Certificate2 certificate) : this(SubjectIdentifierType.IssuerAndSerialNumber, certificate) {} public CmsSigner (CspParameters parameters) : this(SubjectIdentifierType.SubjectKeyIdentifier, PkcsUtils.CreateDummyCertificate(parameters)) { m_dummyCert = true; this.IncludeOption = X509IncludeOption.None; } public CmsSigner (SubjectIdentifierType signerIdentifierType, X509Certificate2 certificate) { switch (signerIdentifierType) { case SubjectIdentifierType.Unknown: this.SignerIdentifierType = SubjectIdentifierType.IssuerAndSerialNumber; this.IncludeOption = X509IncludeOption.ExcludeRoot; break; case SubjectIdentifierType.IssuerAndSerialNumber: this.SignerIdentifierType = signerIdentifierType; this.IncludeOption = X509IncludeOption.ExcludeRoot; break; case SubjectIdentifierType.SubjectKeyIdentifier: this.SignerIdentifierType = signerIdentifierType; this.IncludeOption = X509IncludeOption.ExcludeRoot; break; case SubjectIdentifierType.NoSignature: this.SignerIdentifierType = signerIdentifierType; this.IncludeOption = X509IncludeOption.None; break; default: this.SignerIdentifierType = SubjectIdentifierType.IssuerAndSerialNumber; this.IncludeOption = X509IncludeOption.ExcludeRoot; break; } this.Certificate = certificate; this.DigestAlgorithm = new Oid(CAPI.szOID_OIWSEC_sha1); m_signedAttributes = new CryptographicAttributeObjectCollection(); m_unsignedAttributes = new CryptographicAttributeObjectCollection(); m_certificates = new X509Certificate2Collection(); } // // Public APIs. // public SubjectIdentifierType SignerIdentifierType { get { return m_signerIdentifierType; } set { if (value != SubjectIdentifierType.IssuerAndSerialNumber && value != SubjectIdentifierType.SubjectKeyIdentifier && value != SubjectIdentifierType.NoSignature) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, SecurityResources.GetResourceString("Arg_EnumIllegalVal"), "value")); if (m_dummyCert && value != SubjectIdentifierType.SubjectKeyIdentifier) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, SecurityResources.GetResourceString("Arg_EnumIllegalVal"), "value")); m_signerIdentifierType = value; } } public X509Certificate2 Certificate { get { return m_certificate; } set { m_certificate = value; } } public Oid DigestAlgorithm { get { return m_digestAlgorithm; } set { m_digestAlgorithm = value; } } public CryptographicAttributeObjectCollection SignedAttributes { get { return m_signedAttributes; } } public CryptographicAttributeObjectCollection UnsignedAttributes { get { return m_unsignedAttributes; } } public X509Certificate2Collection Certificates { get { return m_certificates; } } public X509IncludeOption IncludeOption { get { return m_includeOption; } set { if (value < X509IncludeOption.None || value > X509IncludeOption.WholeChain) throw new ArgumentException(String.Format(CultureInfo.CurrentCulture, SecurityResources.GetResourceString("Arg_EnumIllegalVal"), "value")); m_includeOption = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
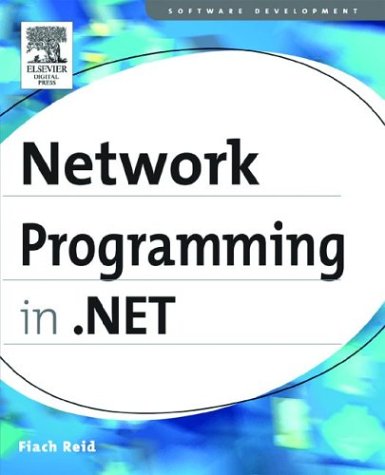
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NumberFormatInfo.cs
- SqlGenericUtil.cs
- Message.cs
- NullExtension.cs
- MsmqInputChannel.cs
- IUnknownConstantAttribute.cs
- Vector.cs
- GetLedgerEntryForRecipientRequest.cs
- ManualResetEvent.cs
- DbConnectionPool.cs
- TryExpression.cs
- ClientSession.cs
- RouteData.cs
- Viewport3DVisual.cs
- TextTreeFixupNode.cs
- PingReply.cs
- CardSpaceException.cs
- GenerateHelper.cs
- KeyConverter.cs
- ColorConvertedBitmapExtension.cs
- SchemaMapping.cs
- FileLoadException.cs
- TokenDescriptor.cs
- ConnectionPointCookie.cs
- ResourceType.cs
- XmlRawWriterWrapper.cs
- SystemDiagnosticsSection.cs
- Latin1Encoding.cs
- XmlCountingReader.cs
- XamlStyleSerializer.cs
- SettingsAttributes.cs
- DotAtomReader.cs
- BinaryNode.cs
- SafeRegistryHandle.cs
- StringInfo.cs
- HelpProvider.cs
- WorkflowQueueInfo.cs
- StructuredCompositeActivityDesigner.cs
- Pair.cs
- XslAst.cs
- ContentHostHelper.cs
- DbLambda.cs
- SystemKeyConverter.cs
- Image.cs
- TraceSection.cs
- DiscriminatorMap.cs
- Point3D.cs
- StylusPointCollection.cs
- WindowsSecurityTokenAuthenticator.cs
- ListMarkerLine.cs
- Int64AnimationBase.cs
- BindingMemberInfo.cs
- SetMemberBinder.cs
- Exceptions.cs
- AstTree.cs
- DialogResultConverter.cs
- PropertyChangingEventArgs.cs
- DataFieldConverter.cs
- PrimitiveCodeDomSerializer.cs
- RelationshipWrapper.cs
- ReadOnlyAttribute.cs
- OdbcCommandBuilder.cs
- RequestNavigateEventArgs.cs
- CrossAppDomainChannel.cs
- BitmapEffectInputData.cs
- CatalogZoneAutoFormat.cs
- BamlVersionHeader.cs
- SizeF.cs
- XamlFilter.cs
- QueueProcessor.cs
- ProfilePropertySettingsCollection.cs
- MetadataUtilsSmi.cs
- WorkflowQueue.cs
- Style.cs
- SystemColors.cs
- GregorianCalendar.cs
- UIAgentRequest.cs
- PropertyDescriptorComparer.cs
- XmlDictionaryString.cs
- UIAgentAsyncParams.cs
- MoveSizeWinEventHandler.cs
- XmlSchemaSimpleType.cs
- clipboard.cs
- BuildProviderAppliesToAttribute.cs
- FaultCode.cs
- ReturnValue.cs
- SupportingTokenSecurityTokenResolver.cs
- TraceContext.cs
- Column.cs
- ExceptionHelpers.cs
- SmiRequestExecutor.cs
- WmfPlaceableFileHeader.cs
- FixUpCollection.cs
- GridItemProviderWrapper.cs
- HyperLink.cs
- ListViewTableCell.cs
- RootDesignerSerializerAttribute.cs
- X509Chain.cs
- ProcessHostMapPath.cs
- TabletDeviceInfo.cs