Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / HelpProvider.cs / 1 / HelpProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System; using System.ComponentModel; using System.ComponentModel.Design; using Microsoft.Win32; using Hashtable = System.Collections.Hashtable; using System.Windows.Forms; using System.Windows.Forms.Design; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; ////// /// [ ProvideProperty("HelpString", typeof(Control)), ProvideProperty("HelpKeyword", typeof(Control)), ProvideProperty("HelpNavigator", typeof(Control)), ProvideProperty("ShowHelp", typeof(Control)), ToolboxItemFilter("System.Windows.Forms"), SRDescription(SR.DescriptionHelpProvider) ] public class HelpProvider : Component, IExtenderProvider { private string helpNamespace = null; private Hashtable helpStrings = new Hashtable(); private Hashtable showHelp = new Hashtable(); private Hashtable boundControls = new Hashtable(); private Hashtable keywords = new Hashtable(); private Hashtable navigators = new Hashtable(); private object userData; ////// Provides pop-up or online Help for controls. /// ////// /// public HelpProvider() { } ////// Initializes a new instance of the ///class. /// /// /// [ Localizable(true), DefaultValue(null), Editor("System.Windows.Forms.Design.HelpNamespaceEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), SRDescription(SR.HelpProviderHelpNamespaceDescr) ] public virtual string HelpNamespace { get { return helpNamespace; } set { this.helpNamespace = value; } } ////// Gets or sets a string indicating the name of the Help /// file associated with this ///object. /// [ SRCategory(SR.CatData), Localizable(false), Bindable(true), SRDescription(SR.ControlTagDescr), DefaultValue(null), TypeConverter(typeof(StringConverter)), ] public object Tag { get { return userData; } set { userData = value; } } /// /// /// public virtual bool CanExtend(object target) { return(target is Control); } ////// Determines if the help provider can offer it's extender properties /// to the specified target object. /// ////// /// [ DefaultValue(null), Localizable(true), SRDescription(SR.HelpProviderHelpKeywordDescr) ] public virtual string GetHelpKeyword(Control ctl) { return(string)keywords[ctl]; } ////// Retrieves the Help Keyword displayed when the /// user invokes Help for the specified control. /// ////// /// [ DefaultValue(HelpNavigator.AssociateIndex), Localizable(true), SRDescription(SR.HelpProviderNavigatorDescr) ] public virtual HelpNavigator GetHelpNavigator(Control ctl) { object nav = navigators[ctl]; return (nav == null) ? HelpNavigator.AssociateIndex : (HelpNavigator)nav; } ////// Retrieves the contents of the pop-up help window for the specified /// control. /// ////// /// [ DefaultValue(null), Localizable(true), SRDescription(SR.HelpProviderHelpStringDescr) ] public virtual string GetHelpString(Control ctl) { return(string)helpStrings[ctl]; } ////// Retrieves the contents of the pop-up help window for the specified /// control. /// ////// /// [ Localizable(true), SRDescription(SR.HelpProviderShowHelpDescr) ] public virtual bool GetShowHelp(Control ctl) { object b = showHelp[ctl]; if (b == null) { return false; } else { return(Boolean) b; } } ////// Retrieves a value indicating whether Help displays for /// the specified control. /// ////// /// Handles the help event for any bound controls. /// ///private void OnControlHelp(object sender, HelpEventArgs hevent) { Control ctl = (Control)sender; string helpString = GetHelpString(ctl); string keyword = GetHelpKeyword(ctl); HelpNavigator navigator = GetHelpNavigator(ctl); bool show = GetShowHelp(ctl); if (!show) { return; } // If the mouse was down, we first try whats this help // if (Control.MouseButtons != MouseButtons.None && helpString != null) { Debug.WriteLineIf(Help.WindowsFormsHelpTrace.TraceVerbose, "HelpProvider:: Mouse down w/ helpstring"); if (helpString.Length > 0) { Help.ShowPopup(ctl, helpString, hevent.MousePos); hevent.Handled = true; } } // If we have a help file, and help keyword we try F1 help next // if (!hevent.Handled && helpNamespace != null) { Debug.WriteLineIf(Help.WindowsFormsHelpTrace.TraceVerbose, "HelpProvider:: F1 help"); if (keyword != null) { if (keyword.Length > 0) { Help.ShowHelp(ctl, helpNamespace, navigator, keyword); hevent.Handled = true; } } if (!hevent.Handled) { Help.ShowHelp(ctl, helpNamespace, navigator); hevent.Handled = true; } } // So at this point we don't have a help keyword, so try to display // the whats this help // if (!hevent.Handled && helpString != null) { Debug.WriteLineIf(Help.WindowsFormsHelpTrace.TraceVerbose, "HelpProvider:: back to helpstring"); if (helpString.Length > 0) { Help.ShowPopup(ctl, helpString, hevent.MousePos); hevent.Handled = true; } } // As a last resort, just popup the contents page of the help file... // if (!hevent.Handled && helpNamespace != null) { Debug.WriteLineIf(Help.WindowsFormsHelpTrace.TraceVerbose, "HelpProvider:: contents"); Help.ShowHelp(ctl, helpNamespace); hevent.Handled = true; } } /// /// /// Handles the help event for any bound controls. /// ///private void OnQueryAccessibilityHelp(object sender, QueryAccessibilityHelpEventArgs e) { Control ctl = (Control)sender; e.HelpString = GetHelpString(ctl); e.HelpKeyword = GetHelpKeyword(ctl); e.HelpNamespace = HelpNamespace; } /// /// /// public virtual void SetHelpString(Control ctl, string helpString) { helpStrings[ctl] = helpString; if (helpString != null) { if (helpString.Length > 0) { SetShowHelp(ctl, true); } } UpdateEventBinding(ctl); } ////// Specifies /// a Help string associated with a control. /// ////// /// public virtual void SetHelpKeyword(Control ctl, string keyword) { keywords[ctl] = keyword; if (keyword != null) { if (keyword.Length > 0) { SetShowHelp(ctl, true); } } UpdateEventBinding(ctl); } ////// Specifies the Help keyword to display when /// the user invokes Help for a control. /// ////// /// public virtual void SetHelpNavigator(Control ctl, HelpNavigator navigator) { //valid values are 0x80000001 to 0x80000007 if (!ClientUtils.IsEnumValid(navigator, (int)navigator, (int)HelpNavigator.Topic, (int)HelpNavigator.TopicId)){ //validate the HelpNavigator enum throw new InvalidEnumArgumentException("navigator", (int)navigator, typeof(HelpNavigator)); } navigators[ctl] = navigator; SetShowHelp(ctl, true); UpdateEventBinding(ctl); } ////// Specifies the Help keyword to display when /// the user invokes Help for a control. /// ////// /// public virtual void SetShowHelp(Control ctl, bool value) { showHelp[ ctl] = value ; UpdateEventBinding(ctl); } ////// Specifies whether Help is displayed for a given control. /// ////// /// Used by the designer /// ///internal virtual bool ShouldSerializeShowHelp(Control ctl) { return showHelp.ContainsKey(ctl); } /// /// /// Used by the designer /// ///public virtual void ResetShowHelp(Control ctl) { showHelp.Remove(ctl); } /// /// /// Binds/unbinds event handlers to ctl /// ///private void UpdateEventBinding(Control ctl) { if (GetShowHelp(ctl) && !boundControls.ContainsKey(ctl)) { ctl.HelpRequested += new HelpEventHandler(this.OnControlHelp); ctl.QueryAccessibilityHelp += new QueryAccessibilityHelpEventHandler(this.OnQueryAccessibilityHelp); boundControls[ctl] = ctl; } else if (!GetShowHelp(ctl) && boundControls.ContainsKey(ctl)) { ctl.HelpRequested -= new HelpEventHandler(this.OnControlHelp); ctl.QueryAccessibilityHelp -= new QueryAccessibilityHelpEventHandler(this.OnQueryAccessibilityHelp); boundControls.Remove(ctl); } } /// /// /// Returns a string representation for this control. /// ///public override string ToString() { string s = base.ToString(); return s + ", HelpNamespace: " + HelpNamespace; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System; using System.ComponentModel; using System.ComponentModel.Design; using Microsoft.Win32; using Hashtable = System.Collections.Hashtable; using System.Windows.Forms; using System.Windows.Forms.Design; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; ////// /// [ ProvideProperty("HelpString", typeof(Control)), ProvideProperty("HelpKeyword", typeof(Control)), ProvideProperty("HelpNavigator", typeof(Control)), ProvideProperty("ShowHelp", typeof(Control)), ToolboxItemFilter("System.Windows.Forms"), SRDescription(SR.DescriptionHelpProvider) ] public class HelpProvider : Component, IExtenderProvider { private string helpNamespace = null; private Hashtable helpStrings = new Hashtable(); private Hashtable showHelp = new Hashtable(); private Hashtable boundControls = new Hashtable(); private Hashtable keywords = new Hashtable(); private Hashtable navigators = new Hashtable(); private object userData; ////// Provides pop-up or online Help for controls. /// ////// /// public HelpProvider() { } ////// Initializes a new instance of the ///class. /// /// /// [ Localizable(true), DefaultValue(null), Editor("System.Windows.Forms.Design.HelpNamespaceEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), SRDescription(SR.HelpProviderHelpNamespaceDescr) ] public virtual string HelpNamespace { get { return helpNamespace; } set { this.helpNamespace = value; } } ////// Gets or sets a string indicating the name of the Help /// file associated with this ///object. /// [ SRCategory(SR.CatData), Localizable(false), Bindable(true), SRDescription(SR.ControlTagDescr), DefaultValue(null), TypeConverter(typeof(StringConverter)), ] public object Tag { get { return userData; } set { userData = value; } } /// /// /// public virtual bool CanExtend(object target) { return(target is Control); } ////// Determines if the help provider can offer it's extender properties /// to the specified target object. /// ////// /// [ DefaultValue(null), Localizable(true), SRDescription(SR.HelpProviderHelpKeywordDescr) ] public virtual string GetHelpKeyword(Control ctl) { return(string)keywords[ctl]; } ////// Retrieves the Help Keyword displayed when the /// user invokes Help for the specified control. /// ////// /// [ DefaultValue(HelpNavigator.AssociateIndex), Localizable(true), SRDescription(SR.HelpProviderNavigatorDescr) ] public virtual HelpNavigator GetHelpNavigator(Control ctl) { object nav = navigators[ctl]; return (nav == null) ? HelpNavigator.AssociateIndex : (HelpNavigator)nav; } ////// Retrieves the contents of the pop-up help window for the specified /// control. /// ////// /// [ DefaultValue(null), Localizable(true), SRDescription(SR.HelpProviderHelpStringDescr) ] public virtual string GetHelpString(Control ctl) { return(string)helpStrings[ctl]; } ////// Retrieves the contents of the pop-up help window for the specified /// control. /// ////// /// [ Localizable(true), SRDescription(SR.HelpProviderShowHelpDescr) ] public virtual bool GetShowHelp(Control ctl) { object b = showHelp[ctl]; if (b == null) { return false; } else { return(Boolean) b; } } ////// Retrieves a value indicating whether Help displays for /// the specified control. /// ////// /// Handles the help event for any bound controls. /// ///private void OnControlHelp(object sender, HelpEventArgs hevent) { Control ctl = (Control)sender; string helpString = GetHelpString(ctl); string keyword = GetHelpKeyword(ctl); HelpNavigator navigator = GetHelpNavigator(ctl); bool show = GetShowHelp(ctl); if (!show) { return; } // If the mouse was down, we first try whats this help // if (Control.MouseButtons != MouseButtons.None && helpString != null) { Debug.WriteLineIf(Help.WindowsFormsHelpTrace.TraceVerbose, "HelpProvider:: Mouse down w/ helpstring"); if (helpString.Length > 0) { Help.ShowPopup(ctl, helpString, hevent.MousePos); hevent.Handled = true; } } // If we have a help file, and help keyword we try F1 help next // if (!hevent.Handled && helpNamespace != null) { Debug.WriteLineIf(Help.WindowsFormsHelpTrace.TraceVerbose, "HelpProvider:: F1 help"); if (keyword != null) { if (keyword.Length > 0) { Help.ShowHelp(ctl, helpNamespace, navigator, keyword); hevent.Handled = true; } } if (!hevent.Handled) { Help.ShowHelp(ctl, helpNamespace, navigator); hevent.Handled = true; } } // So at this point we don't have a help keyword, so try to display // the whats this help // if (!hevent.Handled && helpString != null) { Debug.WriteLineIf(Help.WindowsFormsHelpTrace.TraceVerbose, "HelpProvider:: back to helpstring"); if (helpString.Length > 0) { Help.ShowPopup(ctl, helpString, hevent.MousePos); hevent.Handled = true; } } // As a last resort, just popup the contents page of the help file... // if (!hevent.Handled && helpNamespace != null) { Debug.WriteLineIf(Help.WindowsFormsHelpTrace.TraceVerbose, "HelpProvider:: contents"); Help.ShowHelp(ctl, helpNamespace); hevent.Handled = true; } } /// /// /// Handles the help event for any bound controls. /// ///private void OnQueryAccessibilityHelp(object sender, QueryAccessibilityHelpEventArgs e) { Control ctl = (Control)sender; e.HelpString = GetHelpString(ctl); e.HelpKeyword = GetHelpKeyword(ctl); e.HelpNamespace = HelpNamespace; } /// /// /// public virtual void SetHelpString(Control ctl, string helpString) { helpStrings[ctl] = helpString; if (helpString != null) { if (helpString.Length > 0) { SetShowHelp(ctl, true); } } UpdateEventBinding(ctl); } ////// Specifies /// a Help string associated with a control. /// ////// /// public virtual void SetHelpKeyword(Control ctl, string keyword) { keywords[ctl] = keyword; if (keyword != null) { if (keyword.Length > 0) { SetShowHelp(ctl, true); } } UpdateEventBinding(ctl); } ////// Specifies the Help keyword to display when /// the user invokes Help for a control. /// ////// /// public virtual void SetHelpNavigator(Control ctl, HelpNavigator navigator) { //valid values are 0x80000001 to 0x80000007 if (!ClientUtils.IsEnumValid(navigator, (int)navigator, (int)HelpNavigator.Topic, (int)HelpNavigator.TopicId)){ //validate the HelpNavigator enum throw new InvalidEnumArgumentException("navigator", (int)navigator, typeof(HelpNavigator)); } navigators[ctl] = navigator; SetShowHelp(ctl, true); UpdateEventBinding(ctl); } ////// Specifies the Help keyword to display when /// the user invokes Help for a control. /// ////// /// public virtual void SetShowHelp(Control ctl, bool value) { showHelp[ ctl] = value ; UpdateEventBinding(ctl); } ////// Specifies whether Help is displayed for a given control. /// ////// /// Used by the designer /// ///internal virtual bool ShouldSerializeShowHelp(Control ctl) { return showHelp.ContainsKey(ctl); } /// /// /// Used by the designer /// ///public virtual void ResetShowHelp(Control ctl) { showHelp.Remove(ctl); } /// /// /// Binds/unbinds event handlers to ctl /// ///private void UpdateEventBinding(Control ctl) { if (GetShowHelp(ctl) && !boundControls.ContainsKey(ctl)) { ctl.HelpRequested += new HelpEventHandler(this.OnControlHelp); ctl.QueryAccessibilityHelp += new QueryAccessibilityHelpEventHandler(this.OnQueryAccessibilityHelp); boundControls[ctl] = ctl; } else if (!GetShowHelp(ctl) && boundControls.ContainsKey(ctl)) { ctl.HelpRequested -= new HelpEventHandler(this.OnControlHelp); ctl.QueryAccessibilityHelp -= new QueryAccessibilityHelpEventHandler(this.OnQueryAccessibilityHelp); boundControls.Remove(ctl); } } /// /// /// Returns a string representation for this control. /// ///public override string ToString() { string s = base.ToString(); return s + ", HelpNamespace: " + HelpNamespace; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
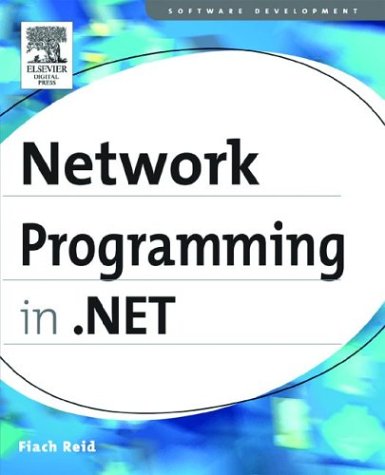
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProviderSettingsCollection.cs
- SamlAssertionDirectKeyIdentifierClause.cs
- DefaultTextStoreTextComposition.cs
- ListSortDescriptionCollection.cs
- ExtendedTransformFactory.cs
- BuildTopDownAttribute.cs
- ApplicationCommands.cs
- DiagnosticsConfiguration.cs
- PathData.cs
- JavaScriptObjectDeserializer.cs
- CallbackHandler.cs
- ParentUndoUnit.cs
- DigitShape.cs
- SoapIgnoreAttribute.cs
- GenericPrincipal.cs
- DataSvcMapFile.cs
- AbandonedMutexException.cs
- DependencyPropertyAttribute.cs
- SocketException.cs
- DataAdapter.cs
- WebBrowserHelper.cs
- MaskDescriptor.cs
- CqlBlock.cs
- PageCodeDomTreeGenerator.cs
- CodeGotoStatement.cs
- ChineseLunisolarCalendar.cs
- SimpleApplicationHost.cs
- OciEnlistContext.cs
- contentDescriptor.cs
- CodeSnippetStatement.cs
- TreePrinter.cs
- FilterQuery.cs
- UnionCodeGroup.cs
- Floater.cs
- CodeVariableDeclarationStatement.cs
- X509SecurityToken.cs
- ExpressionsCollectionEditor.cs
- bidPrivateBase.cs
- XmlSchemaValidator.cs
- WorkflowTraceTransfer.cs
- GraphicsState.cs
- FatalException.cs
- ExpressionVisitor.cs
- Single.cs
- SHA384CryptoServiceProvider.cs
- CacheMemory.cs
- AttributeSetAction.cs
- TabPage.cs
- PackageProperties.cs
- Zone.cs
- ExecutionContext.cs
- PerfCounterSection.cs
- TypeDescriptionProviderAttribute.cs
- WindowsIdentity.cs
- RSAPKCS1KeyExchangeFormatter.cs
- EventMap.cs
- Subset.cs
- ImageBrush.cs
- CellConstantDomain.cs
- HwndSourceKeyboardInputSite.cs
- GuidelineSet.cs
- PublisherIdentityPermission.cs
- MaskedTextBoxTextEditorDropDown.cs
- EditorZone.cs
- DecoderExceptionFallback.cs
- BaseResourcesBuildProvider.cs
- EventLogEntryCollection.cs
- TablePatternIdentifiers.cs
- ChangePasswordDesigner.cs
- Drawing.cs
- Light.cs
- SqlResolver.cs
- UInt16Converter.cs
- TextSpan.cs
- SaveCardRequest.cs
- EncoderParameter.cs
- QueryConverter.cs
- PassportAuthenticationModule.cs
- IncrementalReadDecoders.cs
- PostBackOptions.cs
- ScrollViewerAutomationPeer.cs
- PeerMaintainer.cs
- XmlNodeList.cs
- PageEventArgs.cs
- InvalidOperationException.cs
- ButtonFieldBase.cs
- ResolvedKeyFrameEntry.cs
- ImageAnimator.cs
- ComNativeDescriptor.cs
- TypeSchema.cs
- HtmlPanelAdapter.cs
- _AutoWebProxyScriptHelper.cs
- listitem.cs
- LinkedResourceCollection.cs
- HealthMonitoringSectionHelper.cs
- TraceHandler.cs
- StringFormat.cs
- RelationshipEnd.cs
- RecipientInfo.cs
- UpdateManifestForBrowserApplication.cs