Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlAnchor.cs / 3 / HtmlAnchor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlAnchor.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System.ComponentModel; using System; using System.Collections; using System.Web; using System.Web.UI; using System.Web.Util; using System.Security.Permissions; ////// [ DefaultEvent("ServerClick"), SupportsEventValidation, ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlAnchor : HtmlContainerControl, IPostBackEventHandler { private static readonly object EventServerClick = new object(); /* * Creates an intrinsic Html A control. */ ///The ////// class defines the methods, properties, and /// events for the HtmlAnchor control. /// This /// class /// allows programmatic access to the /// HTML <a> element on the server. /// public HtmlAnchor() : base("a") { } [ WebCategory("Behavior"), DefaultValue(true), ] public virtual bool CausesValidation { get { object b = ViewState["CausesValidation"]; return((b == null) ? true : (bool)b); } set { ViewState["CausesValidation"] = value; } } /* * Href property. */ ///Initializes a new instance of the ///class. /// [ WebCategory("Navigation"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), UrlProperty() ] public string HRef { get { string s = Attributes["href"]; return((s != null) ? s : String.Empty); } set { Attributes["href"] = MapStringAttributeToString(value); } } /* * Name of group this radio is in. */ ///Gets or sets the URL target of the link specified in the /// ////// server control. /// [ WebCategory("Navigation"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Name { get { string s = Attributes["name"]; return((s != null) ? s : String.Empty); } set { Attributes["name"] = MapStringAttributeToString(value); } } /* * Target window property. */ ///Gets or sets the bookmark name defined in the ////// server /// control. /// [ WebCategory("Navigation"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Target { get { string s = Attributes["target"]; return((s != null) ? s : String.Empty); } set { Attributes["target"] = MapStringAttributeToString(value); } } /* * Title property. */ ////// Gets or /// sets the target window or frame /// to load linked Web page content into. /// ////// [ WebCategory("Appearance"), Localizable(true), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Title { get { string s = Attributes["title"]; return((s != null) ? s : String.Empty); } set { Attributes["title"] = MapStringAttributeToString(value); } } [ WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.PostBackControl_ValidationGroup) ] public virtual string ValidationGroup { get { string s = (string)ViewState["ValidationGroup"]; return((s == null) ? string.Empty : s); } set { ViewState["ValidationGroup"] = value; } } ///Gets or sets the title that /// the browser displays when identifying linked content. ////// [ WebCategory("Action"), WebSysDescription(SR.HtmlControl_OnServerClick) ] public event EventHandler ServerClick { add { Events.AddHandler(EventServerClick, value); } remove { Events.RemoveHandler(EventServerClick, value); } } private PostBackOptions GetPostBackOptions() { PostBackOptions options = new PostBackOptions(this, string.Empty); options.RequiresJavaScriptProtocol = true; if (CausesValidation && Page.GetValidators(ValidationGroup).Count > 0) { options.PerformValidation = true; options.ValidationGroup = ValidationGroup; } return options; } ///Occurs on the server when a user clicks the ///control on the /// browser. protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null && Events[EventServerClick] != null) { Page.RegisterPostBackScript(); // if (CausesValidation && Page.GetValidators(ValidationGroup).Count > 0) { Page.RegisterWebFormsScript(); } } } /* * Override to generate postback code for onclick. */ /// /// /// protected override void RenderAttributes(HtmlTextWriter writer) { if (Events[EventServerClick] != null) { Attributes.Remove("href"); base.RenderAttributes(writer); PostBackOptions options = GetPostBackOptions(); Debug.Assert(options != null); string postBackEventReference = Page.ClientScript.GetPostBackEventReference(options, true); Debug.Assert(!string.IsNullOrEmpty(postBackEventReference)); writer.WriteAttribute("href", postBackEventReference, true); } else { PreProcessRelativeReferenceAttribute(writer, "href"); base.RenderAttributes(writer); } } /* * Method used to raise the OnServerClick event. */ ////// protected virtual void OnServerClick(EventArgs e) { EventHandler handler = (EventHandler)Events[EventServerClick]; if (handler != null) handler(this, e); } /* * Method of IPostBackEventHandler interface to raise events on post back. * Button fires an OnServerClick event. */ ///Raises the ////// event. /// /// void IPostBackEventHandler.RaisePostBackEvent(string eventArgument) { RaisePostBackEvent(eventArgument); } ////// /// protected virtual void RaisePostBackEvent(string eventArgument) { ValidateEvent(UniqueID, eventArgument); if (CausesValidation) { Page.Validate(ValidationGroup); } OnServerClick(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlAnchor.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System.ComponentModel; using System; using System.Collections; using System.Web; using System.Web.UI; using System.Web.Util; using System.Security.Permissions; ////// [ DefaultEvent("ServerClick"), SupportsEventValidation, ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlAnchor : HtmlContainerControl, IPostBackEventHandler { private static readonly object EventServerClick = new object(); /* * Creates an intrinsic Html A control. */ ///The ////// class defines the methods, properties, and /// events for the HtmlAnchor control. /// This /// class /// allows programmatic access to the /// HTML <a> element on the server. /// public HtmlAnchor() : base("a") { } [ WebCategory("Behavior"), DefaultValue(true), ] public virtual bool CausesValidation { get { object b = ViewState["CausesValidation"]; return((b == null) ? true : (bool)b); } set { ViewState["CausesValidation"] = value; } } /* * Href property. */ ///Initializes a new instance of the ///class. /// [ WebCategory("Navigation"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), UrlProperty() ] public string HRef { get { string s = Attributes["href"]; return((s != null) ? s : String.Empty); } set { Attributes["href"] = MapStringAttributeToString(value); } } /* * Name of group this radio is in. */ ///Gets or sets the URL target of the link specified in the /// ////// server control. /// [ WebCategory("Navigation"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Name { get { string s = Attributes["name"]; return((s != null) ? s : String.Empty); } set { Attributes["name"] = MapStringAttributeToString(value); } } /* * Target window property. */ ///Gets or sets the bookmark name defined in the ////// server /// control. /// [ WebCategory("Navigation"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Target { get { string s = Attributes["target"]; return((s != null) ? s : String.Empty); } set { Attributes["target"] = MapStringAttributeToString(value); } } /* * Title property. */ ////// Gets or /// sets the target window or frame /// to load linked Web page content into. /// ////// [ WebCategory("Appearance"), Localizable(true), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Title { get { string s = Attributes["title"]; return((s != null) ? s : String.Empty); } set { Attributes["title"] = MapStringAttributeToString(value); } } [ WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.PostBackControl_ValidationGroup) ] public virtual string ValidationGroup { get { string s = (string)ViewState["ValidationGroup"]; return((s == null) ? string.Empty : s); } set { ViewState["ValidationGroup"] = value; } } ///Gets or sets the title that /// the browser displays when identifying linked content. ////// [ WebCategory("Action"), WebSysDescription(SR.HtmlControl_OnServerClick) ] public event EventHandler ServerClick { add { Events.AddHandler(EventServerClick, value); } remove { Events.RemoveHandler(EventServerClick, value); } } private PostBackOptions GetPostBackOptions() { PostBackOptions options = new PostBackOptions(this, string.Empty); options.RequiresJavaScriptProtocol = true; if (CausesValidation && Page.GetValidators(ValidationGroup).Count > 0) { options.PerformValidation = true; options.ValidationGroup = ValidationGroup; } return options; } ///Occurs on the server when a user clicks the ///control on the /// browser. protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null && Events[EventServerClick] != null) { Page.RegisterPostBackScript(); // if (CausesValidation && Page.GetValidators(ValidationGroup).Count > 0) { Page.RegisterWebFormsScript(); } } } /* * Override to generate postback code for onclick. */ /// /// /// protected override void RenderAttributes(HtmlTextWriter writer) { if (Events[EventServerClick] != null) { Attributes.Remove("href"); base.RenderAttributes(writer); PostBackOptions options = GetPostBackOptions(); Debug.Assert(options != null); string postBackEventReference = Page.ClientScript.GetPostBackEventReference(options, true); Debug.Assert(!string.IsNullOrEmpty(postBackEventReference)); writer.WriteAttribute("href", postBackEventReference, true); } else { PreProcessRelativeReferenceAttribute(writer, "href"); base.RenderAttributes(writer); } } /* * Method used to raise the OnServerClick event. */ ////// protected virtual void OnServerClick(EventArgs e) { EventHandler handler = (EventHandler)Events[EventServerClick]; if (handler != null) handler(this, e); } /* * Method of IPostBackEventHandler interface to raise events on post back. * Button fires an OnServerClick event. */ ///Raises the ////// event. /// /// void IPostBackEventHandler.RaisePostBackEvent(string eventArgument) { RaisePostBackEvent(eventArgument); } ////// /// protected virtual void RaisePostBackEvent(string eventArgument) { ValidateEvent(UniqueID, eventArgument); if (CausesValidation) { Page.Validate(ValidationGroup); } OnServerClick(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
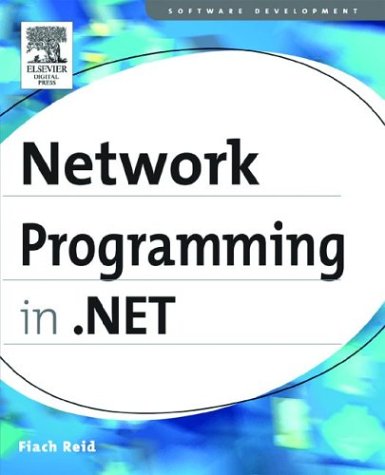
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputBinder.cs
- XsdBuilder.cs
- DataChangedEventManager.cs
- XmlObjectSerializerContext.cs
- DataControlFieldCollection.cs
- SystemColors.cs
- SqlConnectionPoolProviderInfo.cs
- Context.cs
- DataGridRow.cs
- ProxyManager.cs
- TrustLevelCollection.cs
- PropertyNames.cs
- DbException.cs
- ResourceSetExpression.cs
- DocumentManager.cs
- ColumnClickEvent.cs
- AssemblyBuilder.cs
- BaseParser.cs
- XmlElement.cs
- ArgumentsParser.cs
- XmlSchemaSequence.cs
- DeviceFilterEditorDialog.cs
- SHA256Managed.cs
- Atom10FormatterFactory.cs
- FormsAuthenticationUser.cs
- SharedPersonalizationStateInfo.cs
- BinaryUtilClasses.cs
- FindCriteriaElement.cs
- PropertyGridCommands.cs
- ReadOnlyDictionary.cs
- SecurityPermission.cs
- CursorConverter.cs
- LeaseManager.cs
- DrawingContextDrawingContextWalker.cs
- DescendentsWalkerBase.cs
- TCPClient.cs
- DragDrop.cs
- SqlCacheDependency.cs
- CodeNamespaceImportCollection.cs
- EntityModelSchemaGenerator.cs
- ActivityInstanceMap.cs
- PreviewKeyDownEventArgs.cs
- HealthMonitoringSection.cs
- ValidatedControlConverter.cs
- SqlCommandBuilder.cs
- ImageMap.cs
- TextElementEnumerator.cs
- WebPartEditorCancelVerb.cs
- SparseMemoryStream.cs
- ZipIOFileItemStream.cs
- FixedPageProcessor.cs
- ObjectStorage.cs
- Mapping.cs
- WorkItem.cs
- UserControl.cs
- InternalControlCollection.cs
- GeometryModel3D.cs
- CustomAttributeBuilder.cs
- PrimarySelectionGlyph.cs
- PagerSettings.cs
- TemplateKeyConverter.cs
- Button.cs
- activationcontext.cs
- CultureInfo.cs
- ISAPIRuntime.cs
- ObjectPersistData.cs
- Rect3D.cs
- RubberbandSelector.cs
- _FixedSizeReader.cs
- SqlDataSourceTableQuery.cs
- Authorization.cs
- ElementHostAutomationPeer.cs
- CommentEmitter.cs
- TransactionBridgeSection.cs
- PersonalizableTypeEntry.cs
- Application.cs
- SmtpNetworkElement.cs
- ViewEvent.cs
- ClientBuildManagerCallback.cs
- ValueConversionAttribute.cs
- SlotInfo.cs
- ApplicationTrust.cs
- ObjectDataSourceStatusEventArgs.cs
- GridViewAutoFormat.cs
- AddInAdapter.cs
- BindingsCollection.cs
- Dictionary.cs
- RelatedCurrencyManager.cs
- COM2PropertyDescriptor.cs
- QueueProcessor.cs
- TrackingLocationCollection.cs
- CatchBlock.cs
- COM2PictureConverter.cs
- SafeNativeMemoryHandle.cs
- ToolStripDropDownButton.cs
- RevocationPoint.cs
- TextTreeFixupNode.cs
- Pair.cs
- WebResponse.cs
- WebPartVerb.cs