Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / CodeDOM / CodeNamespaceImportCollection.cs / 1 / CodeNamespaceImportCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom { using System.Diagnostics; using System; using System.Collections; using System.Runtime.InteropServices; using System.Globalization; ////// [ ClassInterface(ClassInterfaceType.AutoDispatch), ComVisible(true), Serializable, ] public class CodeNamespaceImportCollection : IList { private ArrayList data = new ArrayList(); private Hashtable keys = new Hashtable(StringComparer.OrdinalIgnoreCase); ////// Manages a collection of ///objects. /// /// public CodeNamespaceImport this[int index] { get { return ((CodeNamespaceImport)data[index]); } set { data[index] = value; SyncKeys(); } } ////// Indexer method that provides collection access. /// ////// public int Count { get { return data.Count; } } ////// Gets or sets the number of namespaces in the collection. /// ///bool IList.IsReadOnly { get { return false; } } /// bool IList.IsFixedSize { get { return false; } } /// /// public void Add(CodeNamespaceImport value) { if (!keys.ContainsKey(value.Namespace)) { keys[value.Namespace] = value; data.Add(value); } } ////// Adds a namespace import to the collection. /// ////// public void AddRange(CodeNamespaceImport[] value) { if (value == null) { throw new ArgumentNullException("value"); } foreach (CodeNamespaceImport c in value) { Add(c); } } ////// Adds a set of ///objects to the collection. /// /// public void Clear() { data.Clear(); keys.Clear(); } ////// Clears the collection of members. /// ////// private void SyncKeys() { keys = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach(CodeNamespaceImport c in this) { keys[c.Namespace] = c; } } ////// Makes the collection of keys synchronised with the data. /// ////// public IEnumerator GetEnumerator() { return data.GetEnumerator(); } ////// Gets an enumerator that enumerates the collection members. /// ///object IList.this[int index] { get { return this[index]; } set { this[index] = (CodeNamespaceImport)value; SyncKeys(); } } /// int ICollection.Count { get { return Count; } } /// bool ICollection.IsSynchronized { get { return false; } } /// object ICollection.SyncRoot { get { return null; } } /// void ICollection.CopyTo(Array array, int index) { data.CopyTo(array, index); } /// IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// int IList.Add(object value) { return data.Add((CodeNamespaceImport)value); } /// void IList.Clear() { Clear(); } /// bool IList.Contains(object value) { return data.Contains(value); } /// int IList.IndexOf(object value) { return data.IndexOf((CodeNamespaceImport)value); } /// void IList.Insert(int index, object value) { data.Insert(index, (CodeNamespaceImport)value); SyncKeys(); } /// void IList.Remove(object value) { data.Remove((CodeNamespaceImport)value); SyncKeys(); } /// void IList.RemoveAt(int index) { data.RemoveAt(index); SyncKeys(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom { using System.Diagnostics; using System; using System.Collections; using System.Runtime.InteropServices; using System.Globalization; ////// [ ClassInterface(ClassInterfaceType.AutoDispatch), ComVisible(true), Serializable, ] public class CodeNamespaceImportCollection : IList { private ArrayList data = new ArrayList(); private Hashtable keys = new Hashtable(StringComparer.OrdinalIgnoreCase); ////// Manages a collection of ///objects. /// /// public CodeNamespaceImport this[int index] { get { return ((CodeNamespaceImport)data[index]); } set { data[index] = value; SyncKeys(); } } ////// Indexer method that provides collection access. /// ////// public int Count { get { return data.Count; } } ////// Gets or sets the number of namespaces in the collection. /// ///bool IList.IsReadOnly { get { return false; } } /// bool IList.IsFixedSize { get { return false; } } /// /// public void Add(CodeNamespaceImport value) { if (!keys.ContainsKey(value.Namespace)) { keys[value.Namespace] = value; data.Add(value); } } ////// Adds a namespace import to the collection. /// ////// public void AddRange(CodeNamespaceImport[] value) { if (value == null) { throw new ArgumentNullException("value"); } foreach (CodeNamespaceImport c in value) { Add(c); } } ////// Adds a set of ///objects to the collection. /// /// public void Clear() { data.Clear(); keys.Clear(); } ////// Clears the collection of members. /// ////// private void SyncKeys() { keys = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach(CodeNamespaceImport c in this) { keys[c.Namespace] = c; } } ////// Makes the collection of keys synchronised with the data. /// ////// public IEnumerator GetEnumerator() { return data.GetEnumerator(); } ////// Gets an enumerator that enumerates the collection members. /// ///object IList.this[int index] { get { return this[index]; } set { this[index] = (CodeNamespaceImport)value; SyncKeys(); } } /// int ICollection.Count { get { return Count; } } /// bool ICollection.IsSynchronized { get { return false; } } /// object ICollection.SyncRoot { get { return null; } } /// void ICollection.CopyTo(Array array, int index) { data.CopyTo(array, index); } /// IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// int IList.Add(object value) { return data.Add((CodeNamespaceImport)value); } /// void IList.Clear() { Clear(); } /// bool IList.Contains(object value) { return data.Contains(value); } /// int IList.IndexOf(object value) { return data.IndexOf((CodeNamespaceImport)value); } /// void IList.Insert(int index, object value) { data.Insert(index, (CodeNamespaceImport)value); SyncKeys(); } /// void IList.Remove(object value) { data.Remove((CodeNamespaceImport)value); SyncKeys(); } /// void IList.RemoveAt(int index) { data.RemoveAt(index); SyncKeys(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
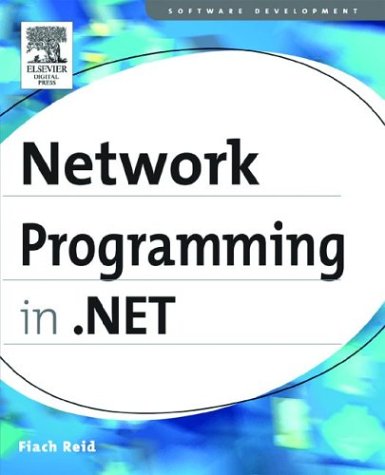
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StorageInfo.cs
- TableAdapterManagerMethodGenerator.cs
- DesignerLoader.cs
- Dynamic.cs
- EntityConnectionStringBuilder.cs
- RequestDescription.cs
- WindowsPrincipal.cs
- TextWriterEngine.cs
- RadioButtonPopupAdapter.cs
- Imaging.cs
- dataobject.cs
- Keyboard.cs
- BindingOperations.cs
- TagNameToTypeMapper.cs
- ContentTypeSettingDispatchMessageFormatter.cs
- DrawingImage.cs
- OleDbErrorCollection.cs
- Decorator.cs
- OptimisticConcurrencyException.cs
- MediaPlayer.cs
- InvalidCastException.cs
- _NTAuthentication.cs
- TranslateTransform3D.cs
- DataObject.cs
- InputScopeNameConverter.cs
- EpmSyndicationContentSerializer.cs
- ExtenderProviderService.cs
- PropertyGridView.cs
- CheckedPointers.cs
- AsymmetricSignatureFormatter.cs
- DataGridViewUtilities.cs
- Propagator.JoinPropagator.cs
- DataGridViewCell.cs
- _ListenerRequestStream.cs
- CryptoStream.cs
- TargetException.cs
- MessageEnumerator.cs
- NullableBoolConverter.cs
- StringKeyFrameCollection.cs
- SqlDependency.cs
- Utils.cs
- VarInfo.cs
- DrawingAttributeSerializer.cs
- RelationshipType.cs
- DeleteMemberBinder.cs
- TabRenderer.cs
- CategoryAttribute.cs
- SpanIndex.cs
- OrderingInfo.cs
- WebUtil.cs
- SoapReflectionImporter.cs
- EntitySetRetriever.cs
- Permission.cs
- ClientOptions.cs
- HtmlInputButton.cs
- EventBindingService.cs
- MimeAnyImporter.cs
- KnownTypeHelper.cs
- PageRanges.cs
- Int32AnimationUsingKeyFrames.cs
- ConnectionInterfaceCollection.cs
- StorageBasedPackageProperties.cs
- SchemaNames.cs
- DropShadowBitmapEffect.cs
- WindowsStatusBar.cs
- RuleSettingsCollection.cs
- dbdatarecord.cs
- ValidatorUtils.cs
- TimeEnumHelper.cs
- BufferBuilder.cs
- ResourceSet.cs
- _FtpDataStream.cs
- xsdvalidator.cs
- FrameworkReadOnlyPropertyMetadata.cs
- WmlSelectionListAdapter.cs
- TextBreakpoint.cs
- PublishLicense.cs
- SystemException.cs
- ParserHooks.cs
- URL.cs
- HasCopySemanticsAttribute.cs
- OledbConnectionStringbuilder.cs
- XmlException.cs
- ProxyHwnd.cs
- WebPartConnectionsCancelVerb.cs
- PersonalizationAdministration.cs
- GrammarBuilder.cs
- CompilerScopeManager.cs
- Quaternion.cs
- VirtualDirectoryMapping.cs
- WebEventCodes.cs
- TransactionManager.cs
- SafeNativeHandle.cs
- EmptyEnumerable.cs
- WindowsGraphics.cs
- BitmapEffectGeneralTransform.cs
- FlowNode.cs
- DiscoveryDocumentLinksPattern.cs
- VectorValueSerializer.cs
- TableItemPattern.cs