Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / Synthesis / TextWriterEngine.cs / 1 / TextWriterEngine.cs
//// Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // // History: // 3/15/2005 [....] Created //---------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.Speech.Synthesis; using System.Speech.Synthesis.TtsEngine; using System.Text; using System.Xml; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. #pragma warning disable 56524 // The _xmlWriter member is not created in this module and should not be disposed namespace System.Speech.Internal.Synthesis { internal class TextWriterEngine : ISsmlParser { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal TextWriterEngine (XmlTextWriter writer, CultureInfo culture) { _writer = writer; _culture = culture; } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods public object ProcessSpeak (string sVersion, string baseUri, CultureInfo culture, ListextraNamespace) { if (!string.IsNullOrEmpty (baseUri)) { throw new ArgumentException (SR.Get (SRID.InvalidSpeakAttribute, "baseUri", "speak"), "baseUri"); } bool fNewCulture = culture != null && !culture.Equals (_culture); if (fNewCulture || !string.IsNullOrEmpty (_pexmlPrefix) || extraNamespace.Count > 0) { _writer.WriteStartElement ("voice"); // Always add the culture info as the voice element cannot not be empty (namespaces declaration don't count) _writer.WriteAttributeString ("xml", "lang", null, culture != null ? culture.Name : _culture.Name); // write all the additionnal namespace foreach (SsmlXmlAttribute ns in extraNamespace) { _writer.WriteAttributeString ("xmlns", ns._name, ns._ns, ns._value); } // If the prompt builder is used with to add prompt engine data, add the namespace if (!string.IsNullOrEmpty (_pexmlPrefix)) { _writer.WriteAttributeString ("xmlns", _pexmlPrefix, null, xmlNamespacePrompt); } _closeSpeak = true; } return null; } public void ProcessText (string text, object voice, ref FragmentState fragmentState, int position, bool fIgnore) { _writer.WriteString (text); } public void ProcessAudio (object voice, string uri, string baseUri, bool fIgnore) { _writer.WriteStartElement ("audio"); _writer.WriteAttributeString ("src", uri); } public void ProcessBreak (object voice, ref FragmentState fragmentState, EmphasisBreak eBreak, int time, bool fIgnore) { _writer.WriteStartElement ("break"); if (time > 0 && eBreak == EmphasisBreak.None) { _writer.WriteAttributeString ("time", time.ToString (CultureInfo.InvariantCulture) + "ms"); } else { string value = null; switch (eBreak) { case EmphasisBreak.None: value = "none"; break; case EmphasisBreak.ExtraWeak: value = "x-weak"; break; case EmphasisBreak.Weak: value = "weak"; break; case EmphasisBreak.Medium: value = "medium"; break; case EmphasisBreak.Strong: value = "strong"; break; case EmphasisBreak.ExtraStrong: value = "x-strong"; break; } if (!string.IsNullOrEmpty (value)) { _writer.WriteAttributeString ("strength", value); } } } public void ProcessDesc (CultureInfo culture) { _writer.WriteStartElement ("desc"); if (culture != null) { _writer.WriteAttributeString ("xml", "lang", null, culture.Name); } } public void ProcessEmphasis (bool noLevel, EmphasisWord word) { _writer.WriteStartElement ("emphasis"); if (word != EmphasisWord.Default) { _writer.WriteAttributeString ("level", word.ToString ().ToLowerInvariant ()); } } public void ProcessMark (object voice, ref FragmentState fragmentState, string name, bool fIgnore) { _writer.WriteStartElement ("mark"); _writer.WriteAttributeString ("name", name); } public object ProcessTextBlock (bool isParagraph, object voice, ref FragmentState fragmentState, CultureInfo culture, bool newCulture, VoiceGender gender, VoiceAge age) { _writer.WriteStartElement (isParagraph ? "p" : "s"); if (culture != null) { _writer.WriteAttributeString ("xml", "lang", null, culture.Name); } return null; } public void EndProcessTextBlock (bool isParagraph) { } public void ProcessPhoneme (ref FragmentState fragmentState, AlphabetType alphabet, string ph, char [] phoneIds) { _writer.WriteStartElement ("phoneme"); if (alphabet != AlphabetType.Ipa) { _writer.WriteAttributeString ("alphabet", alphabet == AlphabetType.Sapi ? "x-microsoft-sapi" : "x-microsoft-ups"); System.Diagnostics.Debug.Assert (alphabet == AlphabetType.Ups || alphabet == AlphabetType.Sapi); } _writer.WriteAttributeString ("ph", ph); } public void ProcessProsody (string pitch, string range, string rate, string volume, string duration, string points) { _writer.WriteStartElement ("prosody"); if (!string.IsNullOrEmpty (range)) { _writer.WriteAttributeString ("range", range); } if (!string.IsNullOrEmpty (rate)) { _writer.WriteAttributeString ("rate", rate); } if (!string.IsNullOrEmpty (volume)) { _writer.WriteAttributeString ("volume", volume); } if (!string.IsNullOrEmpty (duration)) { _writer.WriteAttributeString ("duration", duration); } if (!string.IsNullOrEmpty (points)) { _writer.WriteAttributeString ("range", points); } } public void ProcessSayAs (string interpretAs, string format, string detail) { _writer.WriteStartElement ("say-as"); _writer.WriteAttributeString ("interpret-as", interpretAs); if (!string.IsNullOrEmpty (format)) { _writer.WriteAttributeString ("format", format); } if (!string.IsNullOrEmpty (detail)) { _writer.WriteAttributeString ("detail", detail); } } public void ProcessSub (string alias, object voice, ref FragmentState fragmentState, int position, bool fIgnore) { _writer.WriteStartElement ("sub"); _writer.WriteAttributeString ("alias", alias); } public object ProcessVoice (string name, CultureInfo culture, VoiceGender gender, VoiceAge age, int variant, bool fNewCulture, List extraNamespace) { _writer.WriteStartElement ("voice"); if (!string.IsNullOrEmpty (name)) { _writer.WriteAttributeString ("name", name); } if (fNewCulture && culture != null) { _writer.WriteAttributeString ("xml", "lang", null, culture.Name); } if (gender != VoiceGender.NotSet) { _writer.WriteAttributeString ("gender", gender.ToString ().ToLowerInvariant ()); } if (age != VoiceAge.NotSet) { _writer.WriteAttributeString ("age", ((int) age).ToString (CultureInfo.InvariantCulture)); } if (variant > 0) { _writer.WriteAttributeString ("variant", (variant).ToString (CultureInfo.InvariantCulture)); } // write all the additionnal namespace if (extraNamespace != null) { foreach (SsmlXmlAttribute ns in extraNamespace) { _writer.WriteAttributeString ("xmlns", ns._name, ns._ns, ns._value); } } return null; } public void ProcessLexicon (Uri uri, string type) { _writer.WriteStartElement ("lexicon"); _writer.WriteAttributeString ("uri", uri.ToString ()); if (!string.IsNullOrEmpty (type)) { _writer.WriteAttributeString ("type", type); } } public void EndElement () { _writer.WriteEndElement (); } public void EndSpeakElement () { if (_closeSpeak) { _writer.WriteEndElement (); } } public void ProcessUnknownElement (object voice, ref FragmentState fragmentState, XmlReader reader) { _writer.WriteNode (reader, false); } public void StartProcessUnknownAttributes (object voice, ref FragmentState fragmentState, string sElement, List extraAttributes) { // write all the additionnal namespace foreach (SsmlXmlAttribute attribute in extraAttributes) { _writer.WriteAttributeString (attribute._prefix, attribute._name, attribute._ns, attribute._value); } } public void EndProcessUnknownAttributes (object voice, ref FragmentState fragmentState, string sElement, List extraAttributes) { } #region Prompt Engine public void ContainsPexml (string pexmlPrefix) { _pexmlPrefix = pexmlPrefix; } //this is not a public API!!! #pragma warning disable 56507 private bool ProcessPromptEngine (string element, params KeyValuePair [] attributes) { _writer.WriteStartElement (_pexmlPrefix, element, xmlNamespacePrompt); if (attributes != null) { foreach (KeyValuePair kp in attributes) { if (kp.Value != null) { _writer.WriteAttributeString (kp.Key, kp.Value); } } } return true; } #pragma warning restore 56507 public bool BeginPromptEngineOutput (object voice) { return ProcessPromptEngine ("prompt_output"); } public bool ProcessPromptEngineDatabase (object voice, string fname, string delta, string idset) { return ProcessPromptEngine ("database", new KeyValuePair [] { new KeyValuePair ("fname", fname), new KeyValuePair ("delta", delta), new KeyValuePair ("idset", idset) } ); } public bool ProcessPromptEngineDiv (object voice) { return ProcessPromptEngine ("div"); } public bool ProcessPromptEngineId (object voice, string id) { return ProcessPromptEngine ("id", new KeyValuePair [] { new KeyValuePair ("id", id) }); } public bool BeginPromptEngineTts (object voice) { return ProcessPromptEngine ("tts"); } public void EndPromptEngineTts (object voice) { } public bool BeginPromptEngineWithTag (object voice, string tag) { return ProcessPromptEngine ("withtag", new KeyValuePair [] { new KeyValuePair ("tag", tag) }); } public void EndPromptEngineWithTag (object voice, string tag) { } public bool BeginPromptEngineRule (object voice, string name) { return ProcessPromptEngine ("rule", new KeyValuePair [] { new KeyValuePair ("name", name) }); } public void EndPromptEngineRule (object voice, string name) { } public void EndPromptEngineOutput (object voice) { } #endregion #endregion //******************************************************************* // // Internal Properties // //******************************************************************** #region Internal Properties public string Ssml { get { return null; } } #endregion //******************************************************************** // // Private Fields // //******************************************************************* #region Private Fields private XmlTextWriter _writer; private CultureInfo _culture; private bool _closeSpeak; private string _pexmlPrefix; private const string xmlNamespacePrompt = "http://schemas.microsoft.com/Speech/2003/03/PromptEngine"; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
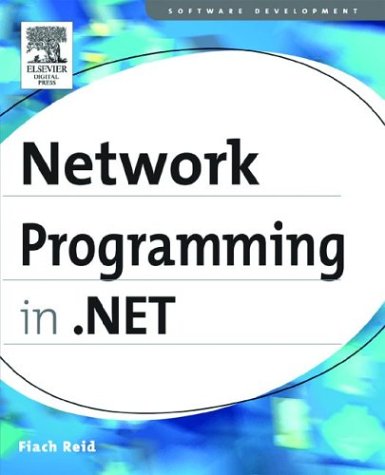
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Pointer.cs
- ProgressBar.cs
- SQLBinary.cs
- DocumentScope.cs
- HttpSessionStateWrapper.cs
- _SecureChannel.cs
- AutomationPatternInfo.cs
- GroupLabel.cs
- Slider.cs
- DataGridViewCellEventArgs.cs
- CollectionViewSource.cs
- DataPager.cs
- RewritingPass.cs
- CornerRadius.cs
- ProgressBar.cs
- CodeTypeParameterCollection.cs
- PackageRelationshipSelector.cs
- CopyOfAction.cs
- VisualTreeUtils.cs
- WeakReferenceList.cs
- ConfigurationManagerInternalFactory.cs
- LocalizedNameDescriptionPair.cs
- Compress.cs
- Environment.cs
- JoinSymbol.cs
- FixedFlowMap.cs
- XmlIterators.cs
- XmlCDATASection.cs
- ProtocolViolationException.cs
- TypeUtils.cs
- UriTemplateCompoundPathSegment.cs
- CellLabel.cs
- NonNullItemCollection.cs
- Enum.cs
- BuildProviderAppliesToAttribute.cs
- SingleObjectCollection.cs
- StateMachineWorkflowDesigner.cs
- SaveLedgerEntryRequest.cs
- SessionEndingCancelEventArgs.cs
- HelloOperationCD1AsyncResult.cs
- RegexCaptureCollection.cs
- ApplicationActivator.cs
- ShapingWorkspace.cs
- Margins.cs
- OdbcCommandBuilder.cs
- SymLanguageType.cs
- PasswordRecovery.cs
- ColumnBinding.cs
- TagPrefixInfo.cs
- IconConverter.cs
- StreamGeometry.cs
- DataSourceProvider.cs
- ResourceDefaultValueAttribute.cs
- ExpressionLexer.cs
- Form.cs
- TypeElementCollection.cs
- MetadataAssemblyHelper.cs
- SqlXmlStorage.cs
- MenuTracker.cs
- GiveFeedbackEventArgs.cs
- RtfNavigator.cs
- SystemNetworkInterface.cs
- EmptyStringExpandableObjectConverter.cs
- MethodCallConverter.cs
- Application.cs
- WebConfigurationHost.cs
- DataGridViewRowsRemovedEventArgs.cs
- Metafile.cs
- DataGridColumn.cs
- BitmapPalette.cs
- TimeIntervalCollection.cs
- ListControl.cs
- SortKey.cs
- DataGridPageChangedEventArgs.cs
- DataTableNameHandler.cs
- SqlEnums.cs
- ObjectDataSourceFilteringEventArgs.cs
- Debug.cs
- TreeNodeCollectionEditor.cs
- WebExceptionStatus.cs
- ProfileManager.cs
- NonDualMessageSecurityOverHttp.cs
- TabControlDesigner.cs
- TreeNode.cs
- EntityDataSourceState.cs
- VectorAnimationBase.cs
- _HTTPDateParse.cs
- CodeAttributeDeclarationCollection.cs
- WebException.cs
- DataGridDesigner.cs
- ContentElementAutomationPeer.cs
- TemplateField.cs
- PagePropertiesChangingEventArgs.cs
- HttpDigestClientCredential.cs
- ActivityCodeDomSerializer.cs
- SystemNetworkInterface.cs
- ParameterDataSourceExpression.cs
- InternalConfigConfigurationFactory.cs
- CompositeDataBoundControl.cs
- AttachedPropertyInfo.cs