Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / ResourceSetExpression.cs / 1 / ResourceSetExpression.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Respresents a resource set in resource bound expression tree. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Reflection; ///ResourceSet Expression [DebuggerDisplay("ResourceSetExpression {Source}.{MemberExpression}")] internal class ResourceSetExpression : ResourceExpression { ////// The (static) type of the resources in this resource set. /// The resource type can differ from this.Type if this expression represents a transparent scope. /// For example, in TransparentScope{Category, Product}, the true element type is Product. /// private readonly Type resourceType; ///source expression private readonly Expression source; ///property member name private readonly Expression member; ///key predicate private DictionarykeyFilter; /// sequence query options private ListsequenceQueryOptions; /// Is Leaf Resource private bool leafResource; ///enclosing transparent scope private TransparentAccessors transparentScope; ////// Creates a ResourceSet expression /// /// the return type of the expression /// the source expression /// property member name /// the element type of the resource set /// expand paths for resource set /// custom query options for resourcse set internal ResourceSetExpression(Type type, Expression source, Expression memberExpression, Type resourceType, ListexpandPaths, Dictionary customQueryOptions) : base(source != null ? (ExpressionType)ResourceExpressionType.ResourceNavigationProperty : (ExpressionType)ResourceExpressionType.RootResourceSet, type, expandPaths, customQueryOptions) { this.source = source; this.member = memberExpression; this.resourceType = resourceType; this.sequenceQueryOptions = new List (); } /// /// Member for ResourceSet /// internal Expression MemberExpression { get { return this.member; } } ////// source /// internal Expression Source { get { return this.source; } } ////// Type of resources contained in this ResourceSet - it's element type. /// internal override Type ResourceType { get { return this.resourceType; } } ////// Is this ResourceSet enclosed in an anonymously-typed transparent scope produced by a SelectMany operation? /// Applies to navigation ResourceSets. /// internal bool HasTransparentScope { get { return this.transparentScope != null; } } ////// The property accesses required to reference this ResourceSet and its source ResourceSet if a transparent scope is present. /// May be null. Use internal TransparentAccessors TransparentScope { get { return this.transparentScope; } set { this.transparentScope = value; } } ///to test for the presence of a value. /// /// Has a key predicate restriction been applied to this ResourceSet? /// internal bool HasKeyPredicate { get { return this.keyFilter != null; } } ////// The property name/required value pairs that comprise the key predicate (if any) applied to this ResourceSet. /// May be null. Use internal Dictionaryto test for the presence of a value. /// KeyPredicate { get { return this.keyFilter; } set { this.keyFilter = value; } } /// /// A resource set produces at most 1 result if constrained by a key predicate /// internal override bool IsSingleton { get { return this.HasKeyPredicate; } } ////// Have sequence query options (filter, orderby, skip, take), expand paths, /// or custom query options been applied to this resource set? /// internal override bool HasQueryOptions { get { return this.sequenceQueryOptions.Count > 0 || this.ExpandPaths.Count > 0 || this.CustomQueryOptions.Count > 0; } } ////// Filter query option for ResourceSet /// internal FilterQueryOptionExpression Filter { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// OrderBy query option for ResourceSet /// internal OrderByQueryOptionExpression OrderBy { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// Skip query option for ResourceSet /// internal SkipQueryOptionExpression Skip { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// Take query option for ResourceSet /// internal TakeQueryOptionExpression Take { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// Gets sequence query options for ResourcSet /// internal IEnumerableSequenceQueryOptions { get { return this.sequenceQueryOptions.ToList(); } } internal bool HasSequenceQueryOptions { get { return this.SequenceQueryOptions.Count() > 0; } } /// /// Is ResourcSet a leaf resource? /// internal bool IsLeafResource { get { return this.leafResource; } set { this.leafResource = value; } } ////// Cast ResourceSetExpression to new type /// internal override ResourceExpression Cast(Type type) { ResourceSetExpression rse = new ResourceSetExpression(type, this.source, this.MemberExpression, TypeSystem.GetElementType(type), this.ExpandPaths.ToList(), this.CustomQueryOptions.ToDictionary(kvp => kvp.Key, kvp => kvp.Value)); rse.keyFilter = this.keyFilter; rse.sequenceQueryOptions = this.sequenceQueryOptions; rse.leafResource = this.leafResource; rse.transparentScope = this.transparentScope; return rse; } ////// Add query option to resource expression /// internal void AddSequenceQueryOption(QueryOptionExpression qoe) { Debug.Assert(qoe != null, "qoe != null"); QueryOptionExpression old = this.sequenceQueryOptions.Where(o => o.GetType() == qoe.GetType()).FirstOrDefault(); if (old != null) { qoe = qoe.ComposeMultipleSpecification(old); this.sequenceQueryOptions.Remove(old); } this.sequenceQueryOptions.Add(qoe); } ////// Instructs this resource set expression to use the input reference expression from /// The resource set expression from which to take the input reference. ///as it's /// own input reference, and to retarget the input reference from to this resource set expression. /// Used exclusively by internal void OverrideInputReference(ResourceSetExpression newInput) { Debug.Assert(newInput != null, "Original resource set cannot be null"); Debug.Assert(this.inputRef == null, "OverrideInputReference cannot be called if the target has already been referenced"); InputReferenceExpression inputRef = newInput.inputRef; if (inputRef != null) { this.inputRef = inputRef; inputRef.OverrideTarget(this); } } ///. /// Represents the property accesses required to access both this resource set and it's source /// resource/set (for navigations). This accesses are required to reference resource sets enclosed /// in transparent scopes introduced by use of SelectMany. /// [DebuggerDisplay("Accessor={Accessor}, SourceAccessor={SourceAccessor}")] internal class TransparentAccessors { ////// The property reference that must be applied to reference this resource set /// internal readonly string Accessor; ////// The property reference that must be applied to reference the source resource set. /// Note that this set's Accessor is NOT required to access the source set, but the /// source set MAY impose it's own Transparent Accessors /// internal readonly string SourceAccessor; ////// Constructs a new transparent scope with the specified set and source set accessors /// /// The name of the property required to access the resource set /// The name of the property required to access the resource set's source internal TransparentAccessors(string acc, string sourceAcc) { Debug.Assert(!string.IsNullOrEmpty(acc), "Set accessor cannot be null or empty"); Debug.Assert(!string.IsNullOrEmpty(sourceAcc), "Source accessor cannot be null or empty"); this.Accessor = acc; this.SourceAccessor = sourceAcc; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Respresents a resource set in resource bound expression tree. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Reflection; ///ResourceSet Expression [DebuggerDisplay("ResourceSetExpression {Source}.{MemberExpression}")] internal class ResourceSetExpression : ResourceExpression { ////// The (static) type of the resources in this resource set. /// The resource type can differ from this.Type if this expression represents a transparent scope. /// For example, in TransparentScope{Category, Product}, the true element type is Product. /// private readonly Type resourceType; ///source expression private readonly Expression source; ///property member name private readonly Expression member; ///key predicate private DictionarykeyFilter; /// sequence query options private ListsequenceQueryOptions; /// Is Leaf Resource private bool leafResource; ///enclosing transparent scope private TransparentAccessors transparentScope; ////// Creates a ResourceSet expression /// /// the return type of the expression /// the source expression /// property member name /// the element type of the resource set /// expand paths for resource set /// custom query options for resourcse set internal ResourceSetExpression(Type type, Expression source, Expression memberExpression, Type resourceType, ListexpandPaths, Dictionary customQueryOptions) : base(source != null ? (ExpressionType)ResourceExpressionType.ResourceNavigationProperty : (ExpressionType)ResourceExpressionType.RootResourceSet, type, expandPaths, customQueryOptions) { this.source = source; this.member = memberExpression; this.resourceType = resourceType; this.sequenceQueryOptions = new List (); } /// /// Member for ResourceSet /// internal Expression MemberExpression { get { return this.member; } } ////// source /// internal Expression Source { get { return this.source; } } ////// Type of resources contained in this ResourceSet - it's element type. /// internal override Type ResourceType { get { return this.resourceType; } } ////// Is this ResourceSet enclosed in an anonymously-typed transparent scope produced by a SelectMany operation? /// Applies to navigation ResourceSets. /// internal bool HasTransparentScope { get { return this.transparentScope != null; } } ////// The property accesses required to reference this ResourceSet and its source ResourceSet if a transparent scope is present. /// May be null. Use internal TransparentAccessors TransparentScope { get { return this.transparentScope; } set { this.transparentScope = value; } } ///to test for the presence of a value. /// /// Has a key predicate restriction been applied to this ResourceSet? /// internal bool HasKeyPredicate { get { return this.keyFilter != null; } } ////// The property name/required value pairs that comprise the key predicate (if any) applied to this ResourceSet. /// May be null. Use internal Dictionaryto test for the presence of a value. /// KeyPredicate { get { return this.keyFilter; } set { this.keyFilter = value; } } /// /// A resource set produces at most 1 result if constrained by a key predicate /// internal override bool IsSingleton { get { return this.HasKeyPredicate; } } ////// Have sequence query options (filter, orderby, skip, take), expand paths, /// or custom query options been applied to this resource set? /// internal override bool HasQueryOptions { get { return this.sequenceQueryOptions.Count > 0 || this.ExpandPaths.Count > 0 || this.CustomQueryOptions.Count > 0; } } ////// Filter query option for ResourceSet /// internal FilterQueryOptionExpression Filter { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// OrderBy query option for ResourceSet /// internal OrderByQueryOptionExpression OrderBy { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// Skip query option for ResourceSet /// internal SkipQueryOptionExpression Skip { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// Take query option for ResourceSet /// internal TakeQueryOptionExpression Take { get { return this.sequenceQueryOptions.OfType().SingleOrDefault(); } } /// /// Gets sequence query options for ResourcSet /// internal IEnumerableSequenceQueryOptions { get { return this.sequenceQueryOptions.ToList(); } } internal bool HasSequenceQueryOptions { get { return this.SequenceQueryOptions.Count() > 0; } } /// /// Is ResourcSet a leaf resource? /// internal bool IsLeafResource { get { return this.leafResource; } set { this.leafResource = value; } } ////// Cast ResourceSetExpression to new type /// internal override ResourceExpression Cast(Type type) { ResourceSetExpression rse = new ResourceSetExpression(type, this.source, this.MemberExpression, TypeSystem.GetElementType(type), this.ExpandPaths.ToList(), this.CustomQueryOptions.ToDictionary(kvp => kvp.Key, kvp => kvp.Value)); rse.keyFilter = this.keyFilter; rse.sequenceQueryOptions = this.sequenceQueryOptions; rse.leafResource = this.leafResource; rse.transparentScope = this.transparentScope; return rse; } ////// Add query option to resource expression /// internal void AddSequenceQueryOption(QueryOptionExpression qoe) { Debug.Assert(qoe != null, "qoe != null"); QueryOptionExpression old = this.sequenceQueryOptions.Where(o => o.GetType() == qoe.GetType()).FirstOrDefault(); if (old != null) { qoe = qoe.ComposeMultipleSpecification(old); this.sequenceQueryOptions.Remove(old); } this.sequenceQueryOptions.Add(qoe); } ////// Instructs this resource set expression to use the input reference expression from /// The resource set expression from which to take the input reference. ///as it's /// own input reference, and to retarget the input reference from to this resource set expression. /// Used exclusively by internal void OverrideInputReference(ResourceSetExpression newInput) { Debug.Assert(newInput != null, "Original resource set cannot be null"); Debug.Assert(this.inputRef == null, "OverrideInputReference cannot be called if the target has already been referenced"); InputReferenceExpression inputRef = newInput.inputRef; if (inputRef != null) { this.inputRef = inputRef; inputRef.OverrideTarget(this); } } ///. /// Represents the property accesses required to access both this resource set and it's source /// resource/set (for navigations). This accesses are required to reference resource sets enclosed /// in transparent scopes introduced by use of SelectMany. /// [DebuggerDisplay("Accessor={Accessor}, SourceAccessor={SourceAccessor}")] internal class TransparentAccessors { ////// The property reference that must be applied to reference this resource set /// internal readonly string Accessor; ////// The property reference that must be applied to reference the source resource set. /// Note that this set's Accessor is NOT required to access the source set, but the /// source set MAY impose it's own Transparent Accessors /// internal readonly string SourceAccessor; ////// Constructs a new transparent scope with the specified set and source set accessors /// /// The name of the property required to access the resource set /// The name of the property required to access the resource set's source internal TransparentAccessors(string acc, string sourceAcc) { Debug.Assert(!string.IsNullOrEmpty(acc), "Set accessor cannot be null or empty"); Debug.Assert(!string.IsNullOrEmpty(sourceAcc), "Source accessor cannot be null or empty"); this.Accessor = acc; this.SourceAccessor = sourceAcc; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
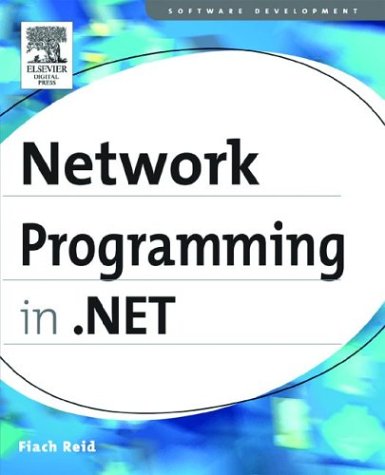
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SetterBaseCollection.cs
- backend.cs
- HiddenFieldPageStatePersister.cs
- WebPartTransformerCollection.cs
- DataControlLinkButton.cs
- CodeNamespaceImportCollection.cs
- CompositeScriptReferenceEventArgs.cs
- Journaling.cs
- MultilineStringConverter.cs
- RadioButtonDesigner.cs
- VariableQuery.cs
- HttpPostProtocolReflector.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- SurrogateDataContract.cs
- OneToOneMappingSerializer.cs
- AnnotationHighlightLayer.cs
- Wizard.cs
- Pair.cs
- EntityReference.cs
- DbDataSourceEnumerator.cs
- OutputChannel.cs
- Effect.cs
- WebPart.cs
- KeyboardNavigation.cs
- XmlEncoding.cs
- Compensate.cs
- IncrementalReadDecoders.cs
- SiteMapNodeItemEventArgs.cs
- FunctionImportElement.cs
- MouseCaptureWithinProperty.cs
- XmlReflectionMember.cs
- AnchoredBlock.cs
- UserControlCodeDomTreeGenerator.cs
- ReliableSessionBindingElement.cs
- ObjectManager.cs
- AdPostCacheSubstitution.cs
- counter.cs
- ReferencedType.cs
- InlinedAggregationOperatorEnumerator.cs
- ProcessRequestAsyncResult.cs
- DesignerCommandSet.cs
- XmlILModule.cs
- CollectionViewSource.cs
- ProvidersHelper.cs
- OleDbPropertySetGuid.cs
- Int32AnimationUsingKeyFrames.cs
- PropertyExpression.cs
- CodeExpressionCollection.cs
- DataSourceSelectArguments.cs
- IntMinMaxAggregationOperator.cs
- PlainXmlDeserializer.cs
- HuffCodec.cs
- dtdvalidator.cs
- DetailsViewUpdatedEventArgs.cs
- TemplateBamlTreeBuilder.cs
- MaterialCollection.cs
- WorkItem.cs
- OdbcErrorCollection.cs
- NetNamedPipeSecurityElement.cs
- StrongName.cs
- TargetControlTypeCache.cs
- ServiceDebugBehavior.cs
- RangeBaseAutomationPeer.cs
- Ipv6Element.cs
- DocumentViewerHelper.cs
- CryptographicAttribute.cs
- LayoutUtils.cs
- ByteKeyFrameCollection.cs
- FrameworkElement.cs
- PackUriHelper.cs
- XmlSerializerOperationGenerator.cs
- QilGenerator.cs
- ToolStripItemCollection.cs
- AllMembershipCondition.cs
- StylusPointPropertyInfo.cs
- NativeMethods.cs
- XmlBinaryReaderSession.cs
- CustomMenuItemCollection.cs
- FileStream.cs
- SoapEnumAttribute.cs
- OuterGlowBitmapEffect.cs
- DbgUtil.cs
- AnimationException.cs
- ConfigXmlDocument.cs
- XamlReaderHelper.cs
- SQLConvert.cs
- SafeBuffer.cs
- SessionStateUtil.cs
- ZipIOCentralDirectoryFileHeader.cs
- linebase.cs
- PagerStyle.cs
- ScriptModule.cs
- ButtonBaseAdapter.cs
- XPathAncestorQuery.cs
- ComponentResourceManager.cs
- UnmanagedMemoryStreamWrapper.cs
- SetterBase.cs
- TemplateBindingExtensionConverter.cs
- ProgressBarHighlightConverter.cs
- MsmqChannelFactory.cs