Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Serialization / System / Xml / XmlBinaryReaderSession.cs / 1305376 / XmlBinaryReaderSession.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- using StringHandle = System.Int64; namespace System.Xml { using System.Xml; using System.Collections.Generic; using System.Runtime.Serialization; public class XmlBinaryReaderSession : IXmlDictionary { const int MaxArrayEntries = 2048; XmlDictionaryString[] strings; DictionarystringDict; public XmlBinaryReaderSession() { } public XmlDictionaryString Add(int id, string value) { if (id < 0) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException(SR.GetString(SR.XmlInvalidID))); if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); XmlDictionaryString xmlString; if (TryLookup(id, out xmlString)) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.XmlIDDefined))); xmlString = new XmlDictionaryString(this, value, id); if (id >= MaxArrayEntries) { if (stringDict == null) this.stringDict = new Dictionary (); this.stringDict.Add(id, xmlString); } else { if (strings == null) { strings = new XmlDictionaryString[Math.Max(id + 1, 16)]; } else if (id >= strings.Length) { XmlDictionaryString[] newStrings = new XmlDictionaryString[Math.Min(Math.Max(id + 1, strings.Length * 2), MaxArrayEntries)]; Array.Copy(strings, newStrings, strings.Length); strings = newStrings; } strings[id] = xmlString; } return xmlString; } public bool TryLookup(int key, out XmlDictionaryString result) { if (strings != null && key >= 0 && key < strings.Length) { result = strings[key]; return result != null; } else if (key >= MaxArrayEntries) { if (this.stringDict != null) return this.stringDict.TryGetValue(key, out result); } result = null; return false; } public bool TryLookup(string value, out XmlDictionaryString result) { if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); if (strings != null) { for (int i = 0; i < strings.Length; i++) { XmlDictionaryString s = strings[i]; if (s != null && s.Value == value) { result = s; return true; } } } if (this.stringDict != null) { foreach (XmlDictionaryString s in this.stringDict.Values) { if (s.Value == value) { result = s; return true; } } } result = null; return false; } public bool TryLookup(XmlDictionaryString value, out XmlDictionaryString result) { if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); if (value.Dictionary != this) { result = null; return false; } result = value; return true; } public void Clear() { if (strings != null) Array.Clear(strings, 0, strings.Length); if (this.stringDict != null) this.stringDict.Clear(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- using StringHandle = System.Int64; namespace System.Xml { using System.Xml; using System.Collections.Generic; using System.Runtime.Serialization; public class XmlBinaryReaderSession : IXmlDictionary { const int MaxArrayEntries = 2048; XmlDictionaryString[] strings; Dictionary stringDict; public XmlBinaryReaderSession() { } public XmlDictionaryString Add(int id, string value) { if (id < 0) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException(SR.GetString(SR.XmlInvalidID))); if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); XmlDictionaryString xmlString; if (TryLookup(id, out xmlString)) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.XmlIDDefined))); xmlString = new XmlDictionaryString(this, value, id); if (id >= MaxArrayEntries) { if (stringDict == null) this.stringDict = new Dictionary (); this.stringDict.Add(id, xmlString); } else { if (strings == null) { strings = new XmlDictionaryString[Math.Max(id + 1, 16)]; } else if (id >= strings.Length) { XmlDictionaryString[] newStrings = new XmlDictionaryString[Math.Min(Math.Max(id + 1, strings.Length * 2), MaxArrayEntries)]; Array.Copy(strings, newStrings, strings.Length); strings = newStrings; } strings[id] = xmlString; } return xmlString; } public bool TryLookup(int key, out XmlDictionaryString result) { if (strings != null && key >= 0 && key < strings.Length) { result = strings[key]; return result != null; } else if (key >= MaxArrayEntries) { if (this.stringDict != null) return this.stringDict.TryGetValue(key, out result); } result = null; return false; } public bool TryLookup(string value, out XmlDictionaryString result) { if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); if (strings != null) { for (int i = 0; i < strings.Length; i++) { XmlDictionaryString s = strings[i]; if (s != null && s.Value == value) { result = s; return true; } } } if (this.stringDict != null) { foreach (XmlDictionaryString s in this.stringDict.Values) { if (s.Value == value) { result = s; return true; } } } result = null; return false; } public bool TryLookup(XmlDictionaryString value, out XmlDictionaryString result) { if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); if (value.Dictionary != this) { result = null; return false; } result = value; return true; } public void Clear() { if (strings != null) Array.Clear(strings, 0, strings.Length); if (this.stringDict != null) this.stringDict.Clear(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
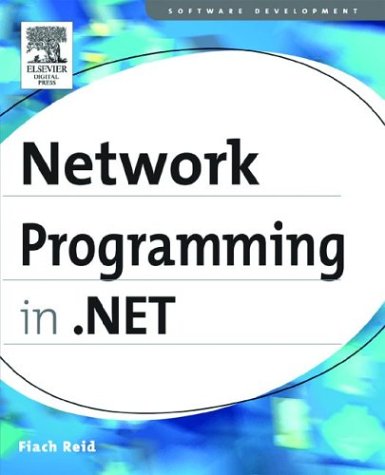
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIElementAutomationPeer.cs
- LambdaCompiler.Address.cs
- BasicExpressionVisitor.cs
- ControlBuilder.cs
- AcceleratedTokenProvider.cs
- BaseInfoTable.cs
- PinnedBufferMemoryStream.cs
- SelectionProcessor.cs
- SerialPinChanges.cs
- AccessControlEntry.cs
- TableLayoutPanelCellPosition.cs
- CodeLinePragma.cs
- NotSupportedException.cs
- FullTextLine.cs
- AspNetHostingPermission.cs
- KnownBoxes.cs
- TemplateBindingExtension.cs
- QuaternionRotation3D.cs
- SymLanguageType.cs
- ObjectFullSpanRewriter.cs
- DataGridViewRowPrePaintEventArgs.cs
- wmiprovider.cs
- Model3DGroup.cs
- PageBuildProvider.cs
- GenerateHelper.cs
- CombinedGeometry.cs
- SocketPermission.cs
- SymbolType.cs
- TimerEventSubscription.cs
- DomNameTable.cs
- EditorZoneBase.cs
- IApplicationTrustManager.cs
- RouteItem.cs
- ObjectComplexPropertyMapping.cs
- XmlEncoding.cs
- MessageFormatterConverter.cs
- WmfPlaceableFileHeader.cs
- MulticastOption.cs
- altserialization.cs
- ClientBuildManagerCallback.cs
- SiteMembershipCondition.cs
- EditingMode.cs
- TakeOrSkipQueryOperator.cs
- SafeTimerHandle.cs
- UnauthorizedAccessException.cs
- QueryStringParameter.cs
- FileIOPermission.cs
- ClipboardProcessor.cs
- DateTimeOffset.cs
- UriParserTemplates.cs
- NativeWindow.cs
- ToolStripDropDown.cs
- WizardDesigner.cs
- BreakSafeBase.cs
- BigInt.cs
- MemoryMappedViewAccessor.cs
- RadioButtonBaseAdapter.cs
- ScriptDescriptor.cs
- EntityDesignPluralizationHandler.cs
- ValidationHelper.cs
- StrokeRenderer.cs
- CornerRadiusConverter.cs
- Page.cs
- DataServiceCollectionOfT.cs
- IndexingContentUnit.cs
- ObjectItemLoadingSessionData.cs
- InstanceOwnerException.cs
- TextElementEnumerator.cs
- ContextMenu.cs
- DBSqlParserColumnCollection.cs
- WizardStepCollectionEditor.cs
- recordstate.cs
- SocketElement.cs
- URLIdentityPermission.cs
- relpropertyhelper.cs
- ListDictionary.cs
- BindStream.cs
- X509ChainElement.cs
- Span.cs
- DispatcherEventArgs.cs
- ProxyWebPartManager.cs
- DataRelationCollection.cs
- X509CertificateStore.cs
- SqlCommandBuilder.cs
- DeviceContext.cs
- SystemFonts.cs
- ThreadPool.cs
- TreeViewEvent.cs
- RSAPKCS1SignatureDeformatter.cs
- FontWeights.cs
- RijndaelManaged.cs
- XmlSchemaComplexContentRestriction.cs
- TextEditorCopyPaste.cs
- ProgressBarHighlightConverter.cs
- DynamicQueryableWrapper.cs
- DataFormats.cs
- StrongTypingException.cs
- XmlILConstructAnalyzer.cs
- BookmarkTable.cs
- SystemIcmpV6Statistics.cs