Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Automation / Peers / ContentElementAutomationPeer.cs / 1 / ContentElementAutomationPeer.cs
//---------------------------------------------------------------------------- // // File: ContentElementAutomationPeer.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Automation element for ContentElements // //--------------------------------------------------------------------------- using System; // Object using System.Collections.Generic; // Listusing System.Windows.Input; // AccessKeyManager using MS.Internal.PresentationCore; // SR namespace System.Windows.Automation.Peers { /// public class ContentElementAutomationPeer : AutomationPeer { /// public ContentElementAutomationPeer(ContentElement owner) { if (owner == null) { throw new ArgumentNullException("owner"); } _owner = owner; } /// public ContentElement Owner { get { return _owner; } } /// /// This static helper creates an AutomationPeer for the specified element and /// caches it - that means the created peer is going to live long and shadow the /// element for its lifetime. The peer will be used by Automation to proxy the element, and /// to fire events to the Automation when something happens with the element. /// The created peer is returned from this method and also from subsequent calls to this method /// and public static AutomationPeer CreatePeerForElement(ContentElement element) { if (element == null) { throw new ArgumentNullException("element"); } return element.CreateAutomationPeer(); } /// public static AutomationPeer FromElement(ContentElement element) { if (element == null) { throw new ArgumentNullException("element"); } return element.GetAutomationPeer(); } ///. The type of the peer is determined by the /// virtual callback. If FrameworkContentElement does not /// implement the callback, there will be no peer and this method will return 'null' (in other /// words, there is no such thing as a 'default peer'). /// /// override protected List/// GetChildrenCore() { return null; } /// override public object GetPattern(PatternInterface patternInterface) { return null; } /// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Custom; } ////// /// protected override string GetAutomationIdCore() { return AutomationProperties.GetAutomationId(_owner); } ////// /// protected override string GetNameCore() { return AutomationProperties.GetName(_owner); } ////// /// protected override string GetHelpTextCore() { return AutomationProperties.GetHelpText(_owner); } ////// /// override protected Rect GetBoundingRectangleCore() { return Rect.Empty; } ////// /// override protected bool IsOffscreenCore() { return true; } ////// /// override protected AutomationOrientation GetOrientationCore() { return AutomationOrientation.None; } ////// /// override protected string GetItemTypeCore() { return string.Empty; } ////// /// override protected string GetClassNameCore() { return string.Empty; } ////// /// override protected string GetItemStatusCore() { return string.Empty; } ////// /// override protected bool IsRequiredForFormCore() { return false; } ////// /// override protected bool IsKeyboardFocusableCore() { return Keyboard.IsFocusable(_owner); } ////// /// override protected bool HasKeyboardFocusCore() { return _owner.IsKeyboardFocused; } ////// /// override protected bool IsEnabledCore() { return _owner.IsEnabled; } ////// /// override protected bool IsPasswordCore() { return false; } ////// /// override protected bool IsContentElementCore() { return true; } ////// /// override protected bool IsControlElementCore() { return false; } ////// /// override protected AutomationPeer GetLabeledByCore() { return null; } ////// /// override protected string GetAcceleratorKeyCore() { return string.Empty; } ////// /// override protected string GetAccessKeyCore() { return AccessKeyManager.InternalGetAccessKeyCharacter(_owner); } ////// /// override protected Point GetClickablePointCore() { return new Point(double.NaN, double.NaN); } ////// /// override protected void SetFocusCore() { if (!_owner.Focus()) throw new InvalidOperationException(SR.Get(SRID.SetFocusFailed)); } private ContentElement _owner; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: ContentElementAutomationPeer.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Automation element for ContentElements // //--------------------------------------------------------------------------- using System; // Object using System.Collections.Generic; // List/// using System.Windows.Input; // AccessKeyManager using MS.Internal.PresentationCore; // SR namespace System.Windows.Automation.Peers { /// public class ContentElementAutomationPeer : AutomationPeer { /// public ContentElementAutomationPeer(ContentElement owner) { if (owner == null) { throw new ArgumentNullException("owner"); } _owner = owner; } /// public ContentElement Owner { get { return _owner; } } /// /// This static helper creates an AutomationPeer for the specified element and /// caches it - that means the created peer is going to live long and shadow the /// element for its lifetime. The peer will be used by Automation to proxy the element, and /// to fire events to the Automation when something happens with the element. /// The created peer is returned from this method and also from subsequent calls to this method /// and public static AutomationPeer CreatePeerForElement(ContentElement element) { if (element == null) { throw new ArgumentNullException("element"); } return element.CreateAutomationPeer(); } /// public static AutomationPeer FromElement(ContentElement element) { if (element == null) { throw new ArgumentNullException("element"); } return element.GetAutomationPeer(); } ///. The type of the peer is determined by the /// virtual callback. If FrameworkContentElement does not /// implement the callback, there will be no peer and this method will return 'null' (in other /// words, there is no such thing as a 'default peer'). /// /// override protected List/// GetChildrenCore() { return null; } /// override public object GetPattern(PatternInterface patternInterface) { return null; } /// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Custom; } ////// /// protected override string GetAutomationIdCore() { return AutomationProperties.GetAutomationId(_owner); } ////// /// protected override string GetNameCore() { return AutomationProperties.GetName(_owner); } ////// /// protected override string GetHelpTextCore() { return AutomationProperties.GetHelpText(_owner); } ////// /// override protected Rect GetBoundingRectangleCore() { return Rect.Empty; } ////// /// override protected bool IsOffscreenCore() { return true; } ////// /// override protected AutomationOrientation GetOrientationCore() { return AutomationOrientation.None; } ////// /// override protected string GetItemTypeCore() { return string.Empty; } ////// /// override protected string GetClassNameCore() { return string.Empty; } ////// /// override protected string GetItemStatusCore() { return string.Empty; } ////// /// override protected bool IsRequiredForFormCore() { return false; } ////// /// override protected bool IsKeyboardFocusableCore() { return Keyboard.IsFocusable(_owner); } ////// /// override protected bool HasKeyboardFocusCore() { return _owner.IsKeyboardFocused; } ////// /// override protected bool IsEnabledCore() { return _owner.IsEnabled; } ////// /// override protected bool IsPasswordCore() { return false; } ////// /// override protected bool IsContentElementCore() { return true; } ////// /// override protected bool IsControlElementCore() { return false; } ////// /// override protected AutomationPeer GetLabeledByCore() { return null; } ////// /// override protected string GetAcceleratorKeyCore() { return string.Empty; } ////// /// override protected string GetAccessKeyCore() { return AccessKeyManager.InternalGetAccessKeyCharacter(_owner); } ////// /// override protected Point GetClickablePointCore() { return new Point(double.NaN, double.NaN); } ////// /// override protected void SetFocusCore() { if (!_owner.Focus()) throw new InvalidOperationException(SR.Get(SRID.SetFocusFailed)); } private ContentElement _owner; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
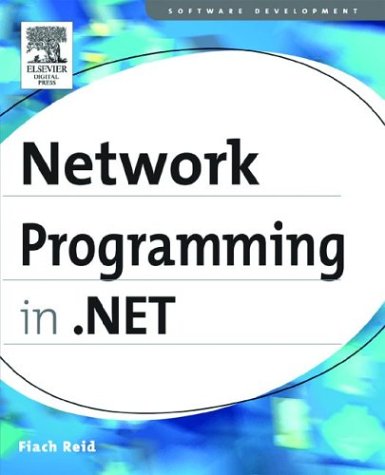
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PackageDigitalSignatureManager.cs
- DynamicResourceExtension.cs
- SQLMembershipProvider.cs
- SafeMILHandleMemoryPressure.cs
- HtmlLabelAdapter.cs
- DeploymentSectionCache.cs
- WpfKnownMember.cs
- Int32Rect.cs
- RepeatBehavior.cs
- DetailsViewUpdatedEventArgs.cs
- OdbcDataAdapter.cs
- _UriTypeConverter.cs
- ProfileSection.cs
- CompiledIdentityConstraint.cs
- SelectQueryOperator.cs
- FieldBuilder.cs
- Operand.cs
- PipelineComponent.cs
- Pair.cs
- QualifierSet.cs
- Stack.cs
- ArithmeticLiteral.cs
- DesignTimeHTMLTextWriter.cs
- mediapermission.cs
- ParameterEditorUserControl.cs
- ConfigurationCollectionAttribute.cs
- TypeLibraryHelper.cs
- ViewPort3D.cs
- RelOps.cs
- PropertyIDSet.cs
- DataGridViewCellValidatingEventArgs.cs
- AttributeData.cs
- UpdatePanelTrigger.cs
- FunctionQuery.cs
- CustomWebEventKey.cs
- XmlTextReaderImpl.cs
- MeasureData.cs
- WmlPhoneCallAdapter.cs
- HttpPostServerProtocol.cs
- StoreContentChangedEventArgs.cs
- DBSqlParserColumn.cs
- DataGridTablesFactory.cs
- CodeCompiler.cs
- TreeNodeStyle.cs
- HideDisabledControlAdapter.cs
- ShapeTypeface.cs
- DataGridRelationshipRow.cs
- VideoDrawing.cs
- ZipArchive.cs
- WindowsGraphicsCacheManager.cs
- SqlStatistics.cs
- CodeSnippetTypeMember.cs
- PointLight.cs
- DetailsViewDeletedEventArgs.cs
- XPathSelfQuery.cs
- ControlCodeDomSerializer.cs
- DesignSurfaceManager.cs
- VerificationAttribute.cs
- FormatterConverter.cs
- DataGridPageChangedEventArgs.cs
- DisplayInformation.cs
- PersonalizationProviderCollection.cs
- RestHandlerFactory.cs
- LayoutExceptionEventArgs.cs
- PtsHost.cs
- GroupBox.cs
- LogAppendAsyncResult.cs
- HttpFileCollection.cs
- UrlMappingsModule.cs
- ResourceDisplayNameAttribute.cs
- OrderedDictionary.cs
- Scripts.cs
- ParenthesizePropertyNameAttribute.cs
- WebSysDisplayNameAttribute.cs
- MdiWindowListStrip.cs
- ResponseBodyWriter.cs
- ApplicationCommands.cs
- AttachmentCollection.cs
- ToolStrip.cs
- XmlSchemaNotation.cs
- DoubleCollection.cs
- RectAnimationClockResource.cs
- CodeCatchClause.cs
- SmiEventSink.cs
- MetaForeignKeyColumn.cs
- EndpointPerformanceCounters.cs
- TextPatternIdentifiers.cs
- RefExpr.cs
- CompositeDataBoundControl.cs
- MostlySingletonList.cs
- RectKeyFrameCollection.cs
- SignatureSummaryDialog.cs
- DependencyObjectType.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- DynamicMethod.cs
- XmlReaderSettings.cs
- SchemaMapping.cs
- Set.cs
- CallbackValidator.cs
- _LazyAsyncResult.cs