Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Diagnostics / Debugger.cs / 1 / Debugger.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // The Debugger class is a part of the System.Diagnostics package // and is used for communicating with a debugger. namespace System.Diagnostics { using System; using System.IO; using System.Collections; using System.Reflection; using System.Runtime.CompilerServices; using System.Security; using System.Security.Permissions; // No data, does not need to be marked with the serializable attribute [System.Runtime.InteropServices.ComVisible(true)] public sealed class Debugger { // Break causes a breakpoint to be signalled to an attached debugger. If no debugger // is attached, the user is asked if he wants to attach a debugger. If yes, then the // debugger is launched. public static void Break() { if (!IsDebuggerAttached()) { // Try and demand UnmanagedCodePermission. This is done in a try block because if this // fails we want to be able to silently eat the exception and just return so // that the call to Break does not possibly cause an unhandled exception. // The idea here is that partially trusted code shouldn't be able to launch a debugger // without the user going through Watson. try { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Demand(); } // If we enter this block, we do not have permission to break into the debugger // and so we just return. catch (SecurityException) { return; } } // Causing a break is now allowed. BreakInternal(); } static void BreakCanThrow() { if (!IsDebuggerAttached()) { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Demand(); } // Causing a break is now allowed. BreakInternal(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern void BreakInternal(); // Launch launches & attaches a debugger to the process. If a debugger is already attached, // nothing happens. // public static bool Launch() { if (IsDebuggerAttached()) return (true); // Try and demand UnmanagedCodePermission. This is done in a try block because if this // fails we want to be able to silently eat the exception and just return so // that the call to Break does not possibly cause an unhandled exception. // The idea here is that partially trusted code shouldn't be able to launch a debugger // without the user going through Watson. try { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Demand(); } // If we enter this block, we do not have permission to break into the debugger // and so we just return. catch (SecurityException) { return (false); } // Causing the debugger to launch is now allowed. return (LaunchInternal()); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern bool LaunchInternal(); // Returns whether or not a debugger is attached to the process. // public static bool IsAttached { get { return IsDebuggerAttached(); } } [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern bool IsDebuggerAttached(); // Constants representing the importance level of messages to be logged. // // An attached debugger can enable or disable which messages will // actually be reported to the user through the COM+ debugger // services API. This info is communicated to the runtime so only // desired events are actually reported to the debugger. // // Constant representing the default category public static readonly String DefaultCategory = null; // Posts a message for the attached debugger. If there is no // debugger attached, has no effect. The debugger may or may not // report the message depending on its settings. [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern void Log(int level, String category, String message); // Checks to see if an attached debugger has logging enabled // [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern bool IsLogging(); } }
Link Menu
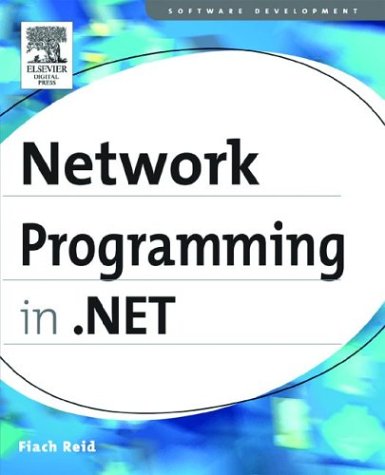
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebConfigurationManager.cs
- DesignerEventService.cs
- MenuCommand.cs
- SchemeSettingElement.cs
- WebPartUserCapability.cs
- PhysicalFontFamily.cs
- ScrollPattern.cs
- NameScope.cs
- DockProviderWrapper.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- __FastResourceComparer.cs
- DesignTimeTemplateParser.cs
- IPEndPoint.cs
- SamlSecurityToken.cs
- IDispatchConstantAttribute.cs
- CheckBoxFlatAdapter.cs
- SerializationEventsCache.cs
- Variant.cs
- ImageSourceConverter.cs
- DeferredReference.cs
- ToolStripButton.cs
- ChangeNode.cs
- ColumnResizeUndoUnit.cs
- AttachmentService.cs
- EntityDataSourceStatementEditor.cs
- QuotedPairReader.cs
- DataMemberConverter.cs
- FunctionNode.cs
- SetterBase.cs
- DecoderReplacementFallback.cs
- KeyGesture.cs
- oledbmetadatacollectionnames.cs
- PageTheme.cs
- dataprotectionpermissionattribute.cs
- MemberAccessException.cs
- SspiNegotiationTokenProviderState.cs
- PageParser.cs
- HtmlInputPassword.cs
- SQLCharsStorage.cs
- MetadataProperty.cs
- Renderer.cs
- SecurityException.cs
- RegisteredDisposeScript.cs
- TimelineCollection.cs
- ConfigXmlElement.cs
- AspCompat.cs
- RightsManagementEncryptionTransform.cs
- HttpProfileBase.cs
- SocketInformation.cs
- RepeatBehaviorConverter.cs
- PropertyInfoSet.cs
- ControlTemplate.cs
- HostSecurityManager.cs
- DbException.cs
- BufferedConnection.cs
- EffectiveValueEntry.cs
- TracePayload.cs
- EdmScalarPropertyAttribute.cs
- DoubleLink.cs
- Helpers.cs
- TransformedBitmap.cs
- TextView.cs
- JournalEntryStack.cs
- COM2Properties.cs
- DesignerCatalogPartChrome.cs
- MetadataCache.cs
- ItemType.cs
- PointConverter.cs
- BitmapEffectCollection.cs
- TemplateBuilder.cs
- BitStack.cs
- PkcsMisc.cs
- SrgsDocumentParser.cs
- XmlIgnoreAttribute.cs
- StatusBar.cs
- DataGridItemAutomationPeer.cs
- MergablePropertyAttribute.cs
- SmtpNtlmAuthenticationModule.cs
- PageFunction.cs
- X509Utils.cs
- IPCCacheManager.cs
- ScrollViewer.cs
- DrawingBrush.cs
- ScriptResourceHandler.cs
- SocketInformation.cs
- IODescriptionAttribute.cs
- Set.cs
- GregorianCalendar.cs
- EncodingTable.cs
- SamlNameIdentifierClaimResource.cs
- SqlWebEventProvider.cs
- ScrollData.cs
- ParsedAttributeCollection.cs
- EditorResources.cs
- MenuAutomationPeer.cs
- IsolatedStorage.cs
- Utils.cs
- HeaderedItemsControl.cs
- ResourceWriter.cs
- CssStyleCollection.cs