Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Navigation / PageFunction.cs / 1 / PageFunction.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // PageFunctions are the "atom" of Structured Navigation, // this is the base element class from which the developer derives // from to enable returning results to a caller. // // History: // ??/10/01: michdav Created // 05/09/03: marka Moved over to WCP dir. Filled in comments and created generic based class // 04/29/04: sujalp Style enabled // 05/13/04: sujalp Moved most of the code to PageFunctionBase // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Controls; using MS.Internal.AppModel; namespace System.Windows.Navigation { ////// Abstract base class for the generic PageFunction class. /// public abstract class PageFunctionBase : Page { #region Constructors ////// PageFunctionBase constructor /// protected PageFunctionBase() { // Make a new GUID for this ID, set the parent ID to 0-0-0-0-0 PageFunctionId = Guid.NewGuid(); ParentPageFunctionId = Guid.Empty; } #endregion Constructors #region Public Properties ////// When set to true, the pagefunction with this property set and all child page functions will get removed from the journal. /// This allows easy building of "transactioned" UI. If a series of steps have been completed, and it doesn't make sense to hit the back button and submit that transaction again, /// setting this property enables those series of steps to be removed from the journal. /// ////// Examples abound of "transactioned UI" buying a book from an ecommerce application, selling stock, creating a user account. /// public bool RemoveFromJournal { get { return _fRemoveFromJournal; } set { _fRemoveFromJournal = value; } } #endregion Public Properties #region Protected Methods ////// This method is called when a PageFunction is first navigated to. /// It is not called when a Pagefunction resumes. /// ////// Typically a developer will write some initialization code in their Start method, /// or they may decide to invoke a child PageFunction. /// protected virtual void Start() { } /* ////// To be used by derived classes to raise strongly types return events. Must be overriden. /// // protected abstract void RaiseTypedReturnEvent(Delegate d, object returnEventArgs); */ #endregion Protected Methods #region Internal Methods // An internal method which is used to invoke the protected Start() method by // other parts of the PageFunction code base. internal void CallStart() { Start(); } ////// A Pagefunction calls this method to signal that it is completed and the ReturnEventArgs /// will be supplied to the listener on Return ( and that listener will be unsuspended). /// internal void _OnReturnUnTyped(object o) { if (_finish != null) { _finish(this, o); } } internal void _AddEventHandler(Delegate d) { // This is where the parent-child relationship is established. If // PageFunction A attaches one of its methods to PageFunction B's // Return event, then A must be B's parent. PageFunctionBase parent = d.Target as PageFunctionBase; if (parent != null) { ParentPageFunctionId = parent.PageFunctionId; } _returnHandler = Delegate.Combine(_returnHandler, d); } internal void _RemoveEventHandler(Delegate d) { _returnHandler = Delegate.Remove(_returnHandler, d); } internal void _DetachEvents() { _returnHandler = null; } // // Raise the event on the _returnHandler // internal void _OnFinish(object returnEventArgs) { RaiseTypedEventArgs args = new RaiseTypedEventArgs(_returnHandler, returnEventArgs); RaiseTypedEvent(this, args); //RaiseTypedReturnEvent(_returnHandler, returnEventArgs); } #endregion Internal Methods #region Internal Properties ////// This is the GUID for this PageFunction. It will be used as its childrens' /// ParentPageFunctionId. /// internal Guid PageFunctionId { get { return _pageFunctionId; } set { _pageFunctionId = value; } } ////// If this PageFunction is a child PageFunction, this will be the GUID of its /// parent PageFunction. If this is Guid.Empty, then the parent is NOT a /// PageFunction. /// internal Guid ParentPageFunctionId { get { return _parentPageFunctionId; } set { _parentPageFunctionId = value; } } internal Delegate _Return { get { return _returnHandler; } } ////// Whether the PF is being resumed after a child PF returns OR due to journal navigation /// (Go Back/Fwd) /// internal bool _Resume { get { return _resume; } set { _resume = value; } } internal ReturnEventSaver _Saver { get { return _saverInfo; } set { _saverInfo = value; } } #endregion Internal Properties #region Internal Events // // The FinishEventHandler is used to communicate between the NavigationService and the ending Pagefunction // internal FinishEventHandler FinishHandler { get { return _finish; } set { _finish = value; } } internal event EventToRaiseTypedEvent RaiseTypedEvent; #endregion #region Private Fields private Guid _pageFunctionId; private Guid _parentPageFunctionId; private bool _fRemoveFromJournal = true; // new default, to make PFs behave more like functions private bool _resume; private ReturnEventSaver _saverInfo; // keeps track of Parent caller's Return Event Info private FinishEventHandler _finish; private Delegate _returnHandler; // the delegate for the Return event #endregion Private Fields } internal delegate void EventToRaiseTypedEvent(PageFunctionBase sender, RaiseTypedEventArgs args); internal class RaiseTypedEventArgs : System.EventArgs { internal RaiseTypedEventArgs(Delegate d, object o) { D = d; O = o; } internal Delegate D; internal Object O; } ////// A callback handler used to receive a ReturnEventArgs of type T /// public delegate void ReturnEventHandler(object sender, ReturnEventArgs e); /// /// PageFunctions are the "atom" of Structured Navigation, /// this is the base element class from which the developer derives /// from to enable returning results to a caller. /// ////// Right now Pagefunctions are non-cls compliant owing to their use of generics. It is expected in the LH timeframe that /// all CLS languages will support generics. /// public class PageFunction: PageFunctionBase { #region Constructors /// /// Pagefunction constructor /// public PageFunction() { RaiseTypedEvent += new EventToRaiseTypedEvent(RaiseTypedReturnEvent); } #endregion Constructors #region Protected Methods ////// A Pagefunction calls this method to signal that it is completed and the ReturnEventArgs /// will be supplied to the listener on Return ( and that listener will be unsuspended). /// protected virtual void OnReturn(ReturnEventArgse) { _OnReturnUnTyped(e); } /// /// Used to raise a strongly typed return event. Sealed since nobody should have the need to override as /// all derived types of this generic type will automatically get the strongly typed version from this /// generic version. /// internal void RaiseTypedReturnEvent(PageFunctionBase b, RaiseTypedEventArgs args) { Delegate d = args.D; object returnEventArgs = args.O; if (d != null) { ReturnEventArgsra = returnEventArgs as ReturnEventArgs ; Debug.Assert((returnEventArgs == null) || (ra != null)); ReturnEventHandler eh = d as ReturnEventHandler ; Debug.Assert(eh != null); eh(this, ra); } } #endregion Protected Methods #region Public Events /// /// This is the event to which a caller will listen to get results returned. /// public event ReturnEventHandlerReturn { // We need to provide a way to surface out // the listeners to the event // So we override add/remove and keep track of it here. add { _AddEventHandler((Delegate)value); } remove { _RemoveEventHandler((Delegate)value); } } #endregion Public Events } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // PageFunctions are the "atom" of Structured Navigation, // this is the base element class from which the developer derives // from to enable returning results to a caller. // // History: // ??/10/01: michdav Created // 05/09/03: marka Moved over to WCP dir. Filled in comments and created generic based class // 04/29/04: sujalp Style enabled // 05/13/04: sujalp Moved most of the code to PageFunctionBase // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Controls; using MS.Internal.AppModel; namespace System.Windows.Navigation { ////// Abstract base class for the generic PageFunction class. /// public abstract class PageFunctionBase : Page { #region Constructors ////// PageFunctionBase constructor /// protected PageFunctionBase() { // Make a new GUID for this ID, set the parent ID to 0-0-0-0-0 PageFunctionId = Guid.NewGuid(); ParentPageFunctionId = Guid.Empty; } #endregion Constructors #region Public Properties ////// When set to true, the pagefunction with this property set and all child page functions will get removed from the journal. /// This allows easy building of "transactioned" UI. If a series of steps have been completed, and it doesn't make sense to hit the back button and submit that transaction again, /// setting this property enables those series of steps to be removed from the journal. /// ////// Examples abound of "transactioned UI" buying a book from an ecommerce application, selling stock, creating a user account. /// public bool RemoveFromJournal { get { return _fRemoveFromJournal; } set { _fRemoveFromJournal = value; } } #endregion Public Properties #region Protected Methods ////// This method is called when a PageFunction is first navigated to. /// It is not called when a Pagefunction resumes. /// ////// Typically a developer will write some initialization code in their Start method, /// or they may decide to invoke a child PageFunction. /// protected virtual void Start() { } /* ////// To be used by derived classes to raise strongly types return events. Must be overriden. /// // protected abstract void RaiseTypedReturnEvent(Delegate d, object returnEventArgs); */ #endregion Protected Methods #region Internal Methods // An internal method which is used to invoke the protected Start() method by // other parts of the PageFunction code base. internal void CallStart() { Start(); } ////// A Pagefunction calls this method to signal that it is completed and the ReturnEventArgs /// will be supplied to the listener on Return ( and that listener will be unsuspended). /// internal void _OnReturnUnTyped(object o) { if (_finish != null) { _finish(this, o); } } internal void _AddEventHandler(Delegate d) { // This is where the parent-child relationship is established. If // PageFunction A attaches one of its methods to PageFunction B's // Return event, then A must be B's parent. PageFunctionBase parent = d.Target as PageFunctionBase; if (parent != null) { ParentPageFunctionId = parent.PageFunctionId; } _returnHandler = Delegate.Combine(_returnHandler, d); } internal void _RemoveEventHandler(Delegate d) { _returnHandler = Delegate.Remove(_returnHandler, d); } internal void _DetachEvents() { _returnHandler = null; } // // Raise the event on the _returnHandler // internal void _OnFinish(object returnEventArgs) { RaiseTypedEventArgs args = new RaiseTypedEventArgs(_returnHandler, returnEventArgs); RaiseTypedEvent(this, args); //RaiseTypedReturnEvent(_returnHandler, returnEventArgs); } #endregion Internal Methods #region Internal Properties ////// This is the GUID for this PageFunction. It will be used as its childrens' /// ParentPageFunctionId. /// internal Guid PageFunctionId { get { return _pageFunctionId; } set { _pageFunctionId = value; } } ////// If this PageFunction is a child PageFunction, this will be the GUID of its /// parent PageFunction. If this is Guid.Empty, then the parent is NOT a /// PageFunction. /// internal Guid ParentPageFunctionId { get { return _parentPageFunctionId; } set { _parentPageFunctionId = value; } } internal Delegate _Return { get { return _returnHandler; } } ////// Whether the PF is being resumed after a child PF returns OR due to journal navigation /// (Go Back/Fwd) /// internal bool _Resume { get { return _resume; } set { _resume = value; } } internal ReturnEventSaver _Saver { get { return _saverInfo; } set { _saverInfo = value; } } #endregion Internal Properties #region Internal Events // // The FinishEventHandler is used to communicate between the NavigationService and the ending Pagefunction // internal FinishEventHandler FinishHandler { get { return _finish; } set { _finish = value; } } internal event EventToRaiseTypedEvent RaiseTypedEvent; #endregion #region Private Fields private Guid _pageFunctionId; private Guid _parentPageFunctionId; private bool _fRemoveFromJournal = true; // new default, to make PFs behave more like functions private bool _resume; private ReturnEventSaver _saverInfo; // keeps track of Parent caller's Return Event Info private FinishEventHandler _finish; private Delegate _returnHandler; // the delegate for the Return event #endregion Private Fields } internal delegate void EventToRaiseTypedEvent(PageFunctionBase sender, RaiseTypedEventArgs args); internal class RaiseTypedEventArgs : System.EventArgs { internal RaiseTypedEventArgs(Delegate d, object o) { D = d; O = o; } internal Delegate D; internal Object O; } ////// A callback handler used to receive a ReturnEventArgs of type T /// public delegate void ReturnEventHandler(object sender, ReturnEventArgs e); /// /// PageFunctions are the "atom" of Structured Navigation, /// this is the base element class from which the developer derives /// from to enable returning results to a caller. /// ////// Right now Pagefunctions are non-cls compliant owing to their use of generics. It is expected in the LH timeframe that /// all CLS languages will support generics. /// public class PageFunction: PageFunctionBase { #region Constructors /// /// Pagefunction constructor /// public PageFunction() { RaiseTypedEvent += new EventToRaiseTypedEvent(RaiseTypedReturnEvent); } #endregion Constructors #region Protected Methods ////// A Pagefunction calls this method to signal that it is completed and the ReturnEventArgs /// will be supplied to the listener on Return ( and that listener will be unsuspended). /// protected virtual void OnReturn(ReturnEventArgse) { _OnReturnUnTyped(e); } /// /// Used to raise a strongly typed return event. Sealed since nobody should have the need to override as /// all derived types of this generic type will automatically get the strongly typed version from this /// generic version. /// internal void RaiseTypedReturnEvent(PageFunctionBase b, RaiseTypedEventArgs args) { Delegate d = args.D; object returnEventArgs = args.O; if (d != null) { ReturnEventArgsra = returnEventArgs as ReturnEventArgs ; Debug.Assert((returnEventArgs == null) || (ra != null)); ReturnEventHandler eh = d as ReturnEventHandler ; Debug.Assert(eh != null); eh(this, ra); } } #endregion Protected Methods #region Public Events /// /// This is the event to which a caller will listen to get results returned. /// public event ReturnEventHandlerReturn { // We need to provide a way to surface out // the listeners to the event // So we override add/remove and keep track of it here. add { _AddEventHandler((Delegate)value); } remove { _RemoveEventHandler((Delegate)value); } } #endregion Public Events } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
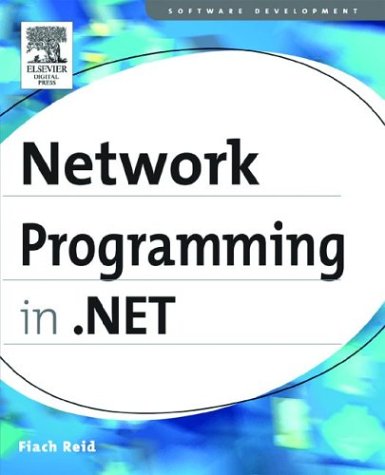
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CqlWriter.cs
- ResourceProviderFactory.cs
- BrowsableAttribute.cs
- EncoderReplacementFallback.cs
- validationstate.cs
- BuildProvider.cs
- ConcurrentDictionary.cs
- EnumUnknown.cs
- TypeReference.cs
- WebPartEditVerb.cs
- DataError.cs
- DateTimeConverter2.cs
- XmlILCommand.cs
- _NegoState.cs
- VariableAction.cs
- Native.cs
- BufferBuilder.cs
- ResourceIDHelper.cs
- FragmentQuery.cs
- ISessionStateStore.cs
- DataGridViewCellPaintingEventArgs.cs
- ComponentChangedEvent.cs
- RepeaterItemCollection.cs
- HttpWebRequest.cs
- StringExpressionSet.cs
- TextLineResult.cs
- LineBreakRecord.cs
- DBAsyncResult.cs
- StringValidatorAttribute.cs
- ConfigurationSchemaErrors.cs
- MailDefinition.cs
- ReadOnlyDictionary.cs
- XmlNamespaceManager.cs
- StrokeIntersection.cs
- PackageStore.cs
- Button.cs
- PropertyEmitterBase.cs
- CodeCompiler.cs
- LocalFileSettingsProvider.cs
- PassportAuthenticationEventArgs.cs
- DiscreteKeyFrames.cs
- SoapElementAttribute.cs
- IsolatedStorage.cs
- X509ThumbprintKeyIdentifierClause.cs
- TdsValueSetter.cs
- EntityReference.cs
- CanonicalXml.cs
- ThemeInfoAttribute.cs
- BuildDependencySet.cs
- BuildManagerHost.cs
- _HeaderInfoTable.cs
- MouseEventArgs.cs
- SafeEventLogWriteHandle.cs
- ClientTargetSection.cs
- SimpleBitVector32.cs
- ProxyWebPart.cs
- HttpWriter.cs
- QueryExpression.cs
- GridViewColumnHeader.cs
- TextReturnReader.cs
- StringDictionaryCodeDomSerializer.cs
- RectConverter.cs
- ExpressionNode.cs
- UserUseLicenseDictionaryLoader.cs
- SessionStateSection.cs
- HttpDebugHandler.cs
- BitmapCache.cs
- CapabilitiesRule.cs
- WebBrowserProgressChangedEventHandler.cs
- CodeIndexerExpression.cs
- RuntimeConfig.cs
- ConnectionStringsExpressionEditor.cs
- SlipBehavior.cs
- CloudCollection.cs
- PersonalizableAttribute.cs
- AnimationLayer.cs
- XpsColorContext.cs
- PageTheme.cs
- CodeGenerator.cs
- XmlReturnReader.cs
- CollectionViewProxy.cs
- ConfigXmlComment.cs
- ToolBarDesigner.cs
- ECDsa.cs
- ValueProviderWrapper.cs
- ResponseStream.cs
- CodeExpressionStatement.cs
- GenerateHelper.cs
- TextureBrush.cs
- SupportingTokenParameters.cs
- CodeIdentifier.cs
- OdbcErrorCollection.cs
- CachedCompositeFamily.cs
- LeaseManager.cs
- ParseNumbers.cs
- EventDescriptor.cs
- TransformedBitmap.cs
- InsufficientMemoryException.cs
- FontFamily.cs
- SynchronousChannel.cs