Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / GDI / TextRenderer.cs / 1 / TextRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Internal; using System; using System.Drawing; using System.Windows.Forms.Internal; using System.Diagnostics; ////// /// public sealed class TextRenderer { //cannot instantiate private TextRenderer() { } ////// This class provides API for drawing GDI text. /// ///public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText( text, wf, bounds, foreColor, GetIntTextFormatFlags( flags ) ); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText(text, wf, bounds, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } private static IntTextFormatFlags GetIntTextFormatFlags(TextFormatFlags flags) { if( ((uint)flags & WindowsGraphics.GdiUnsupportedFlagMask) == 0 ) { return (IntTextFormatFlags) flags; } // Clear TextRenderer custom flags. IntTextFormatFlags windowsGraphicsSupportedFlags = (IntTextFormatFlags) ( ((uint)flags) & ~WindowsGraphics.GdiUnsupportedFlagMask ); return windowsGraphicsSupportedFlags; } /// MeasureText wrappers. public static Size MeasureText(string text, Font font ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf); } } public static Size MeasureText(string text, Font font, Size proposedSize ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, WindowsGraphicsCacheManager.GetWindowsFont(font), proposedSize); } } public static Size MeasureText(string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } public static Size MeasureText(IDeviceContext dc, string text, Font font) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf, proposedSize); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using (WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper(dc, flags)) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wgr.WindowsGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } } internal static Color DisabledTextColor(Color backColor) { //Theme specs -- if the backcolor is darker than Control, we use // ControlPaint.Dark(backcolor). Otherwise we use ControlDark. // see VS#357226 Color disabledTextForeColor = SystemColors.ControlDark; if (ControlPaint.IsDarker(backColor, SystemColors.Control)) { disabledTextForeColor = ControlPaint.Dark(backColor); } return disabledTextForeColor; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Internal; using System; using System.Drawing; using System.Windows.Forms.Internal; using System.Diagnostics; ////// /// public sealed class TextRenderer { //cannot instantiate private TextRenderer() { } ////// This class provides API for drawing GDI text. /// ///public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText( text, wf, bounds, foreColor, GetIntTextFormatFlags( flags ) ); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText(text, wf, bounds, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } private static IntTextFormatFlags GetIntTextFormatFlags(TextFormatFlags flags) { if( ((uint)flags & WindowsGraphics.GdiUnsupportedFlagMask) == 0 ) { return (IntTextFormatFlags) flags; } // Clear TextRenderer custom flags. IntTextFormatFlags windowsGraphicsSupportedFlags = (IntTextFormatFlags) ( ((uint)flags) & ~WindowsGraphics.GdiUnsupportedFlagMask ); return windowsGraphicsSupportedFlags; } /// MeasureText wrappers. public static Size MeasureText(string text, Font font ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf); } } public static Size MeasureText(string text, Font font, Size proposedSize ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, WindowsGraphicsCacheManager.GetWindowsFont(font), proposedSize); } } public static Size MeasureText(string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } public static Size MeasureText(IDeviceContext dc, string text, Font font) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf, proposedSize); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using (WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper(dc, flags)) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wgr.WindowsGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } } internal static Color DisabledTextColor(Color backColor) { //Theme specs -- if the backcolor is darker than Control, we use // ControlPaint.Dark(backcolor). Otherwise we use ControlDark. // see VS#357226 Color disabledTextForeColor = SystemColors.ControlDark; if (ControlPaint.IsDarker(backColor, SystemColors.Control)) { disabledTextForeColor = ControlPaint.Dark(backColor); } return disabledTextForeColor; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
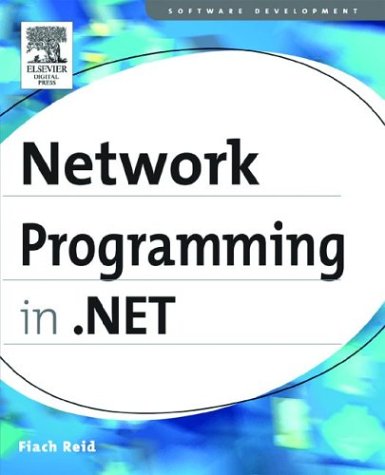
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OdbcPermission.cs
- infer.cs
- OutputCacheSection.cs
- ConnectionPointCookie.cs
- TimeSpanMinutesConverter.cs
- InfoCardSchemas.cs
- ValueTypeFixupInfo.cs
- LineServicesRun.cs
- PerfProviderCollection.cs
- ErrorWebPart.cs
- ListViewUpdatedEventArgs.cs
- ScrollProperties.cs
- LicFileLicenseProvider.cs
- Char.cs
- TypeGeneratedEventArgs.cs
- DataGridViewAccessibleObject.cs
- ColumnReorderedEventArgs.cs
- DropSource.cs
- WeakReference.cs
- RootBrowserWindowAutomationPeer.cs
- OleDbParameterCollection.cs
- DataGridViewRowCancelEventArgs.cs
- XmlTextReaderImpl.cs
- NameNode.cs
- EdgeModeValidation.cs
- RichTextBoxAutomationPeer.cs
- NestPullup.cs
- SByteConverter.cs
- WebPartAddingEventArgs.cs
- DbgCompiler.cs
- CellNormalizer.cs
- SafeRegistryKey.cs
- UInt64Storage.cs
- GrammarBuilderWildcard.cs
- PkcsMisc.cs
- RangeExpression.cs
- VirtualPathUtility.cs
- PeerResolver.cs
- ToolBar.cs
- Parser.cs
- DbProviderFactories.cs
- StreamGeometry.cs
- ISAPIRuntime.cs
- PtsHost.cs
- QuaternionConverter.cs
- AudienceUriMode.cs
- EditingCoordinator.cs
- InputScopeConverter.cs
- SecurityCriticalDataForSet.cs
- xml.cs
- UnsafeNativeMethods.cs
- SmtpFailedRecipientException.cs
- SecurityResources.cs
- PropertyGridEditorPart.cs
- NamespaceEmitter.cs
- DetailsViewDeleteEventArgs.cs
- WebErrorHandler.cs
- base64Transforms.cs
- ToolStripArrowRenderEventArgs.cs
- IntegerCollectionEditor.cs
- SystemKeyConverter.cs
- TextBoxBase.cs
- ScrollBarRenderer.cs
- Zone.cs
- MasterPage.cs
- Attachment.cs
- CursorConverter.cs
- GenericAuthenticationEventArgs.cs
- LOSFormatter.cs
- ValidatingPropertiesEventArgs.cs
- SmtpFailedRecipientException.cs
- LicenseManager.cs
- SerializerProvider.cs
- DataPointer.cs
- IPPacketInformation.cs
- ProviderManager.cs
- RtType.cs
- PropertyTabChangedEvent.cs
- XmlSerializerNamespaces.cs
- CollaborationHelperFunctions.cs
- WpfPayload.cs
- QilLiteral.cs
- PropertyIdentifier.cs
- OutKeywords.cs
- BehaviorEditorPart.cs
- LogPolicy.cs
- AssociatedControlConverter.cs
- ValueExpressions.cs
- Verify.cs
- XomlCompilerResults.cs
- CompositeScriptReference.cs
- PluralizationService.cs
- TCPClient.cs
- XmlSchemaAny.cs
- SqlInfoMessageEvent.cs
- categoryentry.cs
- StateManagedCollection.cs
- DataGridViewColumnEventArgs.cs
- Run.cs
- SliderAutomationPeer.cs