Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / CodeDOM / Compiler / CompilerResults.cs / 1 / CompilerResults.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.CodeDom; using System.Reflection; using System.Collections; using System.Collections.Specialized; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters.Binary; using System.IO; ////// [Serializable()] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] public class CompilerResults { private CompilerErrorCollection errors = new CompilerErrorCollection(); private StringCollection output = new StringCollection(); private Assembly compiledAssembly; private string pathToAssembly; private int nativeCompilerReturnValue; private TempFileCollection tempFiles; private Evidence evidence; ////// Represents the results /// of compilation from the compiler. /// ////// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] public CompilerResults(TempFileCollection tempFiles) { this.tempFiles = tempFiles; } ////// Initializes a new instance of ////// that uses the specified /// temporary files. /// /// public TempFileCollection TempFiles { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return tempFiles; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { tempFiles = value; } } ////// Gets or sets the temporary files to use. /// ////// public Evidence Evidence { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { Evidence e = null; if (evidence != null) e = CloneEvidence(evidence); return e; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [SecurityPermissionAttribute( SecurityAction.Demand, ControlEvidence = true )] set { if (value != null) evidence = CloneEvidence(value); else evidence = null; } } ////// Set the evidence for partially trusted scenarios. /// ////// public Assembly CompiledAssembly { [SecurityPermissionAttribute(SecurityAction.Assert, Flags=SecurityPermissionFlag.ControlEvidence)] get { if (compiledAssembly == null && pathToAssembly != null) { AssemblyName assemName = new AssemblyName(); assemName.CodeBase = pathToAssembly; compiledAssembly = Assembly.Load(assemName,evidence); } return compiledAssembly; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { compiledAssembly = value; } } ////// The compiled assembly. /// ////// public CompilerErrorCollection Errors { get { return errors; } } ////// Gets or sets the collection of compiler errors. /// ////// public StringCollection Output { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return output; } } ////// Gets or sets the compiler output messages. /// ////// public string PathToAssembly { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return pathToAssembly; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { pathToAssembly = value; } } ////// Gets or sets the path to the assembly. /// ////// public int NativeCompilerReturnValue { get { return nativeCompilerReturnValue; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { nativeCompilerReturnValue = value; } } internal static Evidence CloneEvidence(Evidence ev) { new PermissionSet( PermissionState.Unrestricted ).Assert(); MemoryStream stream = new MemoryStream(); BinaryFormatter formatter = new BinaryFormatter(); formatter.Serialize( stream, ev ); stream.Position = 0; return (Evidence)formatter.Deserialize( stream ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Gets or sets the compiler's return value. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.CodeDom; using System.Reflection; using System.Collections; using System.Collections.Specialized; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters.Binary; using System.IO; ////// [Serializable()] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] public class CompilerResults { private CompilerErrorCollection errors = new CompilerErrorCollection(); private StringCollection output = new StringCollection(); private Assembly compiledAssembly; private string pathToAssembly; private int nativeCompilerReturnValue; private TempFileCollection tempFiles; private Evidence evidence; ////// Represents the results /// of compilation from the compiler. /// ////// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] public CompilerResults(TempFileCollection tempFiles) { this.tempFiles = tempFiles; } ////// Initializes a new instance of ////// that uses the specified /// temporary files. /// /// public TempFileCollection TempFiles { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return tempFiles; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { tempFiles = value; } } ////// Gets or sets the temporary files to use. /// ////// public Evidence Evidence { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { Evidence e = null; if (evidence != null) e = CloneEvidence(evidence); return e; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [SecurityPermissionAttribute( SecurityAction.Demand, ControlEvidence = true )] set { if (value != null) evidence = CloneEvidence(value); else evidence = null; } } ////// Set the evidence for partially trusted scenarios. /// ////// public Assembly CompiledAssembly { [SecurityPermissionAttribute(SecurityAction.Assert, Flags=SecurityPermissionFlag.ControlEvidence)] get { if (compiledAssembly == null && pathToAssembly != null) { AssemblyName assemName = new AssemblyName(); assemName.CodeBase = pathToAssembly; compiledAssembly = Assembly.Load(assemName,evidence); } return compiledAssembly; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { compiledAssembly = value; } } ////// The compiled assembly. /// ////// public CompilerErrorCollection Errors { get { return errors; } } ////// Gets or sets the collection of compiler errors. /// ////// public StringCollection Output { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return output; } } ////// Gets or sets the compiler output messages. /// ////// public string PathToAssembly { [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] get { return pathToAssembly; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { pathToAssembly = value; } } ////// Gets or sets the path to the assembly. /// ////// public int NativeCompilerReturnValue { get { return nativeCompilerReturnValue; } [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] set { nativeCompilerReturnValue = value; } } internal static Evidence CloneEvidence(Evidence ev) { new PermissionSet( PermissionState.Unrestricted ).Assert(); MemoryStream stream = new MemoryStream(); BinaryFormatter formatter = new BinaryFormatter(); formatter.Serialize( stream, ev ); stream.Position = 0; return (Evidence)formatter.Deserialize( stream ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Gets or sets the compiler's return value. /// ///
Link Menu
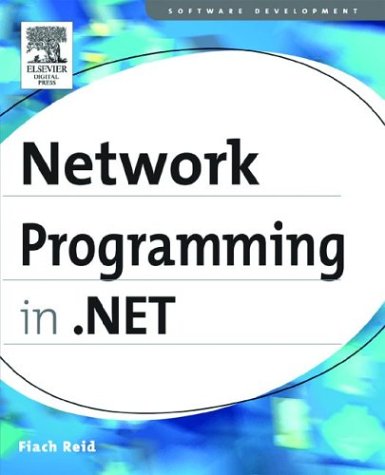
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HyperLinkColumn.cs
- DataGridViewComboBoxColumn.cs
- SqlFormatter.cs
- TextViewSelectionProcessor.cs
- SqlMethodTransformer.cs
- HttpPostProtocolReflector.cs
- CurrencyWrapper.cs
- XmlSchemaProviderAttribute.cs
- CookielessData.cs
- XmlNodeChangedEventManager.cs
- TextEndOfLine.cs
- ExpressionVisitor.cs
- GestureRecognitionResult.cs
- DataServiceExpressionVisitor.cs
- DrawingGroupDrawingContext.cs
- PropertyIDSet.cs
- FilterEventArgs.cs
- GuidelineSet.cs
- CommandEventArgs.cs
- DataSourceControl.cs
- SslStream.cs
- GetCertificateRequest.cs
- XmlSchemaAttributeGroupRef.cs
- MemberRelationshipService.cs
- DataSetMappper.cs
- DescendentsWalkerBase.cs
- FloatSumAggregationOperator.cs
- EndpointDispatcher.cs
- HyperLinkField.cs
- CompressEmulationStream.cs
- SimpleType.cs
- BamlRecords.cs
- InputLanguageEventArgs.cs
- AnnotationObservableCollection.cs
- FrameworkElementAutomationPeer.cs
- ActivitySurrogate.cs
- SpotLight.cs
- SchemaEntity.cs
- ObjectDataSource.cs
- ChtmlSelectionListAdapter.cs
- mongolianshape.cs
- MustUnderstandBehavior.cs
- DataServiceException.cs
- TextBoxBase.cs
- TerminatorSinks.cs
- ProcessProtocolHandler.cs
- BuildProviderUtils.cs
- GroupItemAutomationPeer.cs
- SerialPort.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- UnsafeNativeMethodsMilCoreApi.cs
- CellIdBoolean.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- TransferRequestHandler.cs
- MenuItem.cs
- ProfileProvider.cs
- RegexCapture.cs
- BinaryUtilClasses.cs
- ComponentEvent.cs
- NonNullItemCollection.cs
- ToolboxCategory.cs
- WebPartCatalogCloseVerb.cs
- KeyInterop.cs
- DiscoveryClientBindingElement.cs
- DelegateInArgument.cs
- UnsafeNativeMethods.cs
- __TransparentProxy.cs
- EventData.cs
- Peer.cs
- DataGridViewButtonColumn.cs
- EventsTab.cs
- RowCache.cs
- SliderAutomationPeer.cs
- StringValidatorAttribute.cs
- FrameworkTextComposition.cs
- WindowsIdentity.cs
- DataGridViewRowHeaderCell.cs
- PictureBox.cs
- HostedNamedPipeTransportManager.cs
- DuplicateDetector.cs
- ContentType.cs
- FormatConvertedBitmap.cs
- ApplicationContext.cs
- IsolatedStorageSecurityState.cs
- DrawingContextDrawingContextWalker.cs
- UndoManager.cs
- InstanceOwnerException.cs
- HttpStaticObjectsCollectionWrapper.cs
- WebPartEditorCancelVerb.cs
- MediaContextNotificationWindow.cs
- ConstructorBuilder.cs
- MarshalDirectiveException.cs
- NameTable.cs
- LinearKeyFrames.cs
- SystemColors.cs
- CustomTypeDescriptor.cs
- MaskedTextBox.cs
- SystemPens.cs
- ResourcePool.cs
- CompiledQuery.cs