Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Controls / ItemsPanelTemplate.cs / 1 / ItemsPanelTemplate.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: ItemsPanelTemplate describes how ItemsPresenter creates the panel // that manages layout of containers for an ItemsControl. // // Specs: // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Controls; using System.Windows.Markup; using System.Diagnostics; namespace System.Windows.Controls { ////// ItemsPanelTemplate describes how ItemsPresenter creates the panel /// that manages layout of containers for an ItemsControl. /// public class ItemsPanelTemplate : FrameworkTemplate { #region Constructors //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- ////// ItemsPanelTemplate Constructor /// public ItemsPanelTemplate() { } ////// ItemsPanelTemplate Constructor /// public ItemsPanelTemplate(FrameworkElementFactory root) { VisualTree = root; } #endregion Constructors #region Public Properties //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #endregion PublicProperties #region Internal Properties //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- // // TargetType for ItemsPanelTemplate. This is override is // so FrameworkTemplate can see this property. // internal override Type TargetTypeInternal { get { return DefaultTargetType; } } // Subclasses must provide a way for the parser to directly set the // target type. For ItemsPanelTemplate, this is not allowed. internal override void SetTargetTypeInternal(Type targetType) { throw new InvalidOperationException(SR.Get(SRID.TemplateNotTargetType)); } // Target type of ItemsPanelTemplate is ItemsPresenter static internal Type DefaultTargetType { get { return typeof(ItemsPresenter); } } #endregion Internal Properties #region Internal Methods //------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- // // ProcessTemplateBeforeSeal // // This is used in the case of templates defined with FEFs. For templates // in Baml (the typical case), see the OnApply override. // // 1. Verify that // a. root element is a Panel // 2. Set IsItemsHost = true // internal override void ProcessTemplateBeforeSeal() { FrameworkElementFactory root; if( HasContent ) { // This is a Baml-style template // Validate the root type (it must be a Panel) if (!typeof(Panel).IsAssignableFrom(OptimizedTemplateContent.RootType)) throw new InvalidOperationException(SR.Get(SRID.ItemsPanelNotAPanel, OptimizedTemplateContent.RootType)); // Set the IsItemsHost property on the root, via the template tables BamlNamedElementStartRecord bamlNamedElementStartRecord = OptimizedTemplateContent.UnsharedContent as BamlNamedElementStartRecord; SharedDp sharedDp = new SharedDp( Panel.IsItemsHostProperty, true, bamlNamedElementStartRecord.RuntimeName ); OptimizedTemplateContent.SharedProperties.Add( sharedDp ); } else if ((root = this.VisualTree) != null) { // This is a FEF-style template if (!typeof(Panel).IsAssignableFrom(root.Type)) throw new InvalidOperationException(SR.Get(SRID.ItemsPanelNotAPanel, root.Type)); root.SetValue(Panel.IsItemsHostProperty, true); } } #endregion Internal Methods #region Protected Methods //------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------- ////// Validate against the following rules /// 1. Must have a non-null feTemplatedParent /// 2. A ItemsPanelTemplate must be applied to a ContentPresenter /// protected override void ValidateTemplatedParent(FrameworkElement templatedParent) { // Must have a non-null feTemplatedParent if (templatedParent == null) { throw new ArgumentNullException("templatedParent"); } // A ItemsPanelTemplate must be applied to an ItemsPresenter if (!(templatedParent is ItemsPresenter)) { throw new ArgumentException(SR.Get(SRID.TemplateTargetTypeMismatch, "ItemsPresenter", templatedParent.GetType().Name)); } } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: ItemsPanelTemplate describes how ItemsPresenter creates the panel // that manages layout of containers for an ItemsControl. // // Specs: // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Controls; using System.Windows.Markup; using System.Diagnostics; namespace System.Windows.Controls { ////// ItemsPanelTemplate describes how ItemsPresenter creates the panel /// that manages layout of containers for an ItemsControl. /// public class ItemsPanelTemplate : FrameworkTemplate { #region Constructors //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- ////// ItemsPanelTemplate Constructor /// public ItemsPanelTemplate() { } ////// ItemsPanelTemplate Constructor /// public ItemsPanelTemplate(FrameworkElementFactory root) { VisualTree = root; } #endregion Constructors #region Public Properties //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #endregion PublicProperties #region Internal Properties //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- // // TargetType for ItemsPanelTemplate. This is override is // so FrameworkTemplate can see this property. // internal override Type TargetTypeInternal { get { return DefaultTargetType; } } // Subclasses must provide a way for the parser to directly set the // target type. For ItemsPanelTemplate, this is not allowed. internal override void SetTargetTypeInternal(Type targetType) { throw new InvalidOperationException(SR.Get(SRID.TemplateNotTargetType)); } // Target type of ItemsPanelTemplate is ItemsPresenter static internal Type DefaultTargetType { get { return typeof(ItemsPresenter); } } #endregion Internal Properties #region Internal Methods //------------------------------------------------------------------- // // Internal Methods // //-------------------------------------------------------------------- // // ProcessTemplateBeforeSeal // // This is used in the case of templates defined with FEFs. For templates // in Baml (the typical case), see the OnApply override. // // 1. Verify that // a. root element is a Panel // 2. Set IsItemsHost = true // internal override void ProcessTemplateBeforeSeal() { FrameworkElementFactory root; if( HasContent ) { // This is a Baml-style template // Validate the root type (it must be a Panel) if (!typeof(Panel).IsAssignableFrom(OptimizedTemplateContent.RootType)) throw new InvalidOperationException(SR.Get(SRID.ItemsPanelNotAPanel, OptimizedTemplateContent.RootType)); // Set the IsItemsHost property on the root, via the template tables BamlNamedElementStartRecord bamlNamedElementStartRecord = OptimizedTemplateContent.UnsharedContent as BamlNamedElementStartRecord; SharedDp sharedDp = new SharedDp( Panel.IsItemsHostProperty, true, bamlNamedElementStartRecord.RuntimeName ); OptimizedTemplateContent.SharedProperties.Add( sharedDp ); } else if ((root = this.VisualTree) != null) { // This is a FEF-style template if (!typeof(Panel).IsAssignableFrom(root.Type)) throw new InvalidOperationException(SR.Get(SRID.ItemsPanelNotAPanel, root.Type)); root.SetValue(Panel.IsItemsHostProperty, true); } } #endregion Internal Methods #region Protected Methods //------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------- ////// Validate against the following rules /// 1. Must have a non-null feTemplatedParent /// 2. A ItemsPanelTemplate must be applied to a ContentPresenter /// protected override void ValidateTemplatedParent(FrameworkElement templatedParent) { // Must have a non-null feTemplatedParent if (templatedParent == null) { throw new ArgumentNullException("templatedParent"); } // A ItemsPanelTemplate must be applied to an ItemsPresenter if (!(templatedParent is ItemsPresenter)) { throw new ArgumentException(SR.Get(SRID.TemplateTargetTypeMismatch, "ItemsPresenter", templatedParent.GetType().Name)); } } #endregion Protected Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
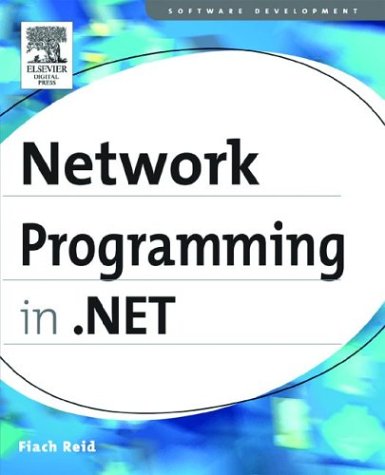
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- JobStaple.cs
- ServiceDocument.cs
- Decoder.cs
- WindowsScrollBar.cs
- QuaternionAnimation.cs
- DataSourceView.cs
- DataContractSet.cs
- ColorAnimationUsingKeyFrames.cs
- Filter.cs
- TextRunCache.cs
- DetailsView.cs
- MappingSource.cs
- AutomationPatternInfo.cs
- DbConnectionInternal.cs
- StylusButtonEventArgs.cs
- HtmlLiteralTextAdapter.cs
- StackBuilderSink.cs
- DiscoveryServerProtocol.cs
- _CommandStream.cs
- XappLauncher.cs
- StateMachineExecutionState.cs
- NumberFormatter.cs
- RemoteWebConfigurationHostStream.cs
- StringExpressionSet.cs
- TransactionProxy.cs
- XmlQueryOutput.cs
- RouteData.cs
- RowCache.cs
- WebPartActionVerb.cs
- PageCache.cs
- PrimitiveSchema.cs
- RouteValueExpressionBuilder.cs
- EasingFunctionBase.cs
- HatchBrush.cs
- XmlSchemaRedefine.cs
- WorkflowEnvironment.cs
- SecurityHelper.cs
- DataServices.cs
- InternalDuplexBindingElement.cs
- XmlStreamStore.cs
- COAUTHIDENTITY.cs
- TimeSpanValidatorAttribute.cs
- PersistenceProviderBehavior.cs
- DefaultProxySection.cs
- MissingSatelliteAssemblyException.cs
- DisposableCollectionWrapper.cs
- ComponentSerializationService.cs
- ZipIOExtraFieldElement.cs
- WorkflowInstanceAbortedRecord.cs
- DataObjectCopyingEventArgs.cs
- TabRenderer.cs
- CachedTypeface.cs
- ListCollectionView.cs
- CheckBoxList.cs
- CodePropertyReferenceExpression.cs
- EntityDataSourceUtil.cs
- MenuBase.cs
- CharacterHit.cs
- ParagraphVisual.cs
- PointF.cs
- SyndicationItemFormatter.cs
- AppDomainAttributes.cs
- RelOps.cs
- ReachFixedDocumentSerializer.cs
- ParagraphResult.cs
- XmlObjectSerializerWriteContext.cs
- Attribute.cs
- CodeAttributeDeclarationCollection.cs
- ControlAdapter.cs
- _SSPIWrapper.cs
- SchemaObjectWriter.cs
- GifBitmapDecoder.cs
- SqlTypeSystemProvider.cs
- RegexMatch.cs
- TemplateBindingExpression.cs
- TrackBarDesigner.cs
- SQLDouble.cs
- WorkflowViewManager.cs
- codemethodreferenceexpression.cs
- SupportsEventValidationAttribute.cs
- SelectionRangeConverter.cs
- SchemaEntity.cs
- Graph.cs
- WebPartHeaderCloseVerb.cs
- Color.cs
- ComAdminWrapper.cs
- DataGridViewLinkCell.cs
- PolyBezierSegment.cs
- ConnectionInterfaceCollection.cs
- StaticContext.cs
- Token.cs
- streamingZipPartStream.cs
- HttpHeaderCollection.cs
- ManualResetEvent.cs
- BackgroundFormatInfo.cs
- ReadOnlyNameValueCollection.cs
- XmlReflectionImporter.cs
- RuntimeConfigurationRecord.cs
- XmlArrayItemAttribute.cs
- SystemResourceKey.cs