Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Controls / GroupStyle.cs / 2 / GroupStyle.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Description of UI for grouping. // // See spec at http://avalon/connecteddata/Specs/Grouping.mht // //--------------------------------------------------------------------------- using System.ComponentModel; // [DefaultValue] using System.Windows.Data; // CollectionViewGroup namespace System.Windows.Controls { ////// The GroupStyle describes how to display the items in a GroupCollection, /// such as the collection obtained from CollectionViewGroup.Items. /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // cannot be read & localized as string public class GroupStyle : INotifyPropertyChanged { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Initializes a new instance of GroupStyle. /// public GroupStyle() { _panel = DefaultGroupPanel; } static GroupStyle() { ItemsPanelTemplate template = new ItemsPanelTemplate(new FrameworkElementFactory(typeof(StackPanel))); template.Seal(); DefaultGroupPanel = template; s_DefaultGroupStyle = new GroupStyle(); } #endregion Constructors #region INotifyPropertyChanged ////// This event is raised when a property of the group style has changed. /// event PropertyChangedEventHandler INotifyPropertyChanged.PropertyChanged { add { PropertyChanged += value; } remove { PropertyChanged -= value; } } ////// PropertyChanged event (per protected virtual event PropertyChangedEventHandler PropertyChanged; ///). /// /// A subclass can call this method to raise the PropertyChanged event. /// protected virtual void OnPropertyChanged(PropertyChangedEventArgs e) { if (PropertyChanged != null) { PropertyChanged(this, e); } } #endregion INotifyPropertyChanged #region Public Properties //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// A template that creates the panel used to layout the items. /// public ItemsPanelTemplate Panel { get { return _panel; } set { _panel = value; OnPropertyChanged("Panel"); } } ////// ContainerStyle is the style that is applied to the GroupItem generated /// for each item. /// [DefaultValue(null)] public Style ContainerStyle { get { return _containerStyle; } set { _containerStyle = value; OnPropertyChanged("ContainerStyle"); } } ////// ContainerStyleSelector allows the app writer to provide custom style selection logic /// for a style to apply to each generated GroupItem. /// [DefaultValue(null)] public StyleSelector ContainerStyleSelector { get { return _containerStyleSelector; } set { _containerStyleSelector = value; OnPropertyChanged("ContainerStyleSelector"); } } ////// HeaderTemplate is the template used to display the group header. /// [DefaultValue(null)] public DataTemplate HeaderTemplate { get { return _headerTemplate; } set { _headerTemplate = value; OnPropertyChanged("HeaderTemplate"); } } ////// HeaderTemplateSelector allows the app writer to provide custom selection logic /// for a template used to display the group header. /// [DefaultValue(null)] public DataTemplateSelector HeaderTemplateSelector { get { return _headerTemplateSelector; } set { _headerTemplateSelector = value; OnPropertyChanged("HeaderTemplateSelector"); } } ////// HeaderStringFormat is the format used to display the header content as a string. /// This arises only when no template is available. /// [DefaultValue(null)] public String HeaderStringFormat { get { return _headerStringFormat; } set { _headerStringFormat = value; OnPropertyChanged("HeaderStringFormat"); } } ////// HidesIfEmpty allows the app writer to indicate whether items corresponding /// to empty groups should be displayed. /// [DefaultValue(false)] public bool HidesIfEmpty { get { return _hidesIfEmpty; } set { _hidesIfEmpty = value; OnPropertyChanged("HidesIfEmpty"); } } ////// AlternationCount controls the range of values assigned to the /// ItemsControl.AlternationIndex property on containers generated /// for this level of grouping. [DefaultValue(0)] public int AlternationCount { get { return _alternationCount; } set { _alternationCount = value; _isAlternationCountSet = true; OnPropertyChanged("AlternationCount"); } } /// The default panel template. public static readonly ItemsPanelTemplate DefaultGroupPanel; ///The default GroupStyle. public static GroupStyle Default { get { return s_DefaultGroupStyle; } } #endregion Public Properties #region Private Properties private void OnPropertyChanged(string propertyName) { OnPropertyChanged(new PropertyChangedEventArgs(propertyName)); } internal bool IsAlternationCountSet { get { return _isAlternationCountSet; } } #endregion Private Properties #region Private Fields //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ ItemsPanelTemplate _panel; Style _containerStyle; StyleSelector _containerStyleSelector; DataTemplate _headerTemplate; DataTemplateSelector _headerTemplateSelector; string _headerStringFormat; bool _hidesIfEmpty; bool _isAlternationCountSet; int _alternationCount; static GroupStyle s_DefaultGroupStyle; #endregion Private Fields } ////// A delegate to select the group style as a function of the /// parent group and its level. /// public delegate GroupStyle GroupStyleSelector(CollectionViewGroup group, int level); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Description of UI for grouping. // // See spec at http://avalon/connecteddata/Specs/Grouping.mht // //--------------------------------------------------------------------------- using System.ComponentModel; // [DefaultValue] using System.Windows.Data; // CollectionViewGroup namespace System.Windows.Controls { ////// The GroupStyle describes how to display the items in a GroupCollection, /// such as the collection obtained from CollectionViewGroup.Items. /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // cannot be read & localized as string public class GroupStyle : INotifyPropertyChanged { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// Initializes a new instance of GroupStyle. /// public GroupStyle() { _panel = DefaultGroupPanel; } static GroupStyle() { ItemsPanelTemplate template = new ItemsPanelTemplate(new FrameworkElementFactory(typeof(StackPanel))); template.Seal(); DefaultGroupPanel = template; s_DefaultGroupStyle = new GroupStyle(); } #endregion Constructors #region INotifyPropertyChanged ////// This event is raised when a property of the group style has changed. /// event PropertyChangedEventHandler INotifyPropertyChanged.PropertyChanged { add { PropertyChanged += value; } remove { PropertyChanged -= value; } } ////// PropertyChanged event (per protected virtual event PropertyChangedEventHandler PropertyChanged; ///). /// /// A subclass can call this method to raise the PropertyChanged event. /// protected virtual void OnPropertyChanged(PropertyChangedEventArgs e) { if (PropertyChanged != null) { PropertyChanged(this, e); } } #endregion INotifyPropertyChanged #region Public Properties //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ////// A template that creates the panel used to layout the items. /// public ItemsPanelTemplate Panel { get { return _panel; } set { _panel = value; OnPropertyChanged("Panel"); } } ////// ContainerStyle is the style that is applied to the GroupItem generated /// for each item. /// [DefaultValue(null)] public Style ContainerStyle { get { return _containerStyle; } set { _containerStyle = value; OnPropertyChanged("ContainerStyle"); } } ////// ContainerStyleSelector allows the app writer to provide custom style selection logic /// for a style to apply to each generated GroupItem. /// [DefaultValue(null)] public StyleSelector ContainerStyleSelector { get { return _containerStyleSelector; } set { _containerStyleSelector = value; OnPropertyChanged("ContainerStyleSelector"); } } ////// HeaderTemplate is the template used to display the group header. /// [DefaultValue(null)] public DataTemplate HeaderTemplate { get { return _headerTemplate; } set { _headerTemplate = value; OnPropertyChanged("HeaderTemplate"); } } ////// HeaderTemplateSelector allows the app writer to provide custom selection logic /// for a template used to display the group header. /// [DefaultValue(null)] public DataTemplateSelector HeaderTemplateSelector { get { return _headerTemplateSelector; } set { _headerTemplateSelector = value; OnPropertyChanged("HeaderTemplateSelector"); } } ////// HeaderStringFormat is the format used to display the header content as a string. /// This arises only when no template is available. /// [DefaultValue(null)] public String HeaderStringFormat { get { return _headerStringFormat; } set { _headerStringFormat = value; OnPropertyChanged("HeaderStringFormat"); } } ////// HidesIfEmpty allows the app writer to indicate whether items corresponding /// to empty groups should be displayed. /// [DefaultValue(false)] public bool HidesIfEmpty { get { return _hidesIfEmpty; } set { _hidesIfEmpty = value; OnPropertyChanged("HidesIfEmpty"); } } ////// AlternationCount controls the range of values assigned to the /// ItemsControl.AlternationIndex property on containers generated /// for this level of grouping. [DefaultValue(0)] public int AlternationCount { get { return _alternationCount; } set { _alternationCount = value; _isAlternationCountSet = true; OnPropertyChanged("AlternationCount"); } } /// The default panel template. public static readonly ItemsPanelTemplate DefaultGroupPanel; ///The default GroupStyle. public static GroupStyle Default { get { return s_DefaultGroupStyle; } } #endregion Public Properties #region Private Properties private void OnPropertyChanged(string propertyName) { OnPropertyChanged(new PropertyChangedEventArgs(propertyName)); } internal bool IsAlternationCountSet { get { return _isAlternationCountSet; } } #endregion Private Properties #region Private Fields //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ ItemsPanelTemplate _panel; Style _containerStyle; StyleSelector _containerStyleSelector; DataTemplate _headerTemplate; DataTemplateSelector _headerTemplateSelector; string _headerStringFormat; bool _hidesIfEmpty; bool _isAlternationCountSet; int _alternationCount; static GroupStyle s_DefaultGroupStyle; #endregion Private Fields } ////// A delegate to select the group style as a function of the /// parent group and its level. /// public delegate GroupStyle GroupStyleSelector(CollectionViewGroup group, int level); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
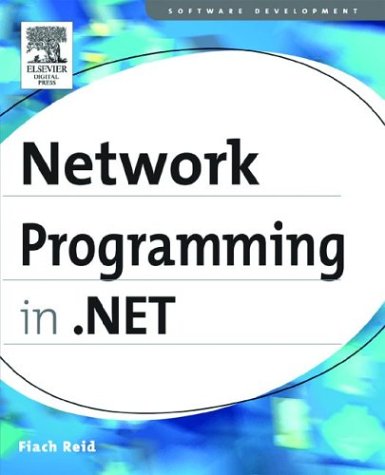
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LogLogRecordHeader.cs
- InfocardChannelParameter.cs
- HitTestWithGeometryDrawingContextWalker.cs
- DialogBaseForm.cs
- _AcceptOverlappedAsyncResult.cs
- FixedLineResult.cs
- SQLChars.cs
- RelationshipFixer.cs
- IisTraceListener.cs
- XPathDocumentNavigator.cs
- ProfileModule.cs
- EventLog.cs
- SQLGuidStorage.cs
- ScrollItemProviderWrapper.cs
- TreeViewHitTestInfo.cs
- ImageClickEventArgs.cs
- Confirm.cs
- EntityModelSchemaGenerator.cs
- LoginNameDesigner.cs
- RenderCapability.cs
- TypedReference.cs
- DataGridViewRowConverter.cs
- DataGridRelationshipRow.cs
- InvokeWebService.cs
- Rotation3DAnimationBase.cs
- AnimationException.cs
- AnnotationResourceCollection.cs
- TabItem.cs
- AttributeSetAction.cs
- DatePicker.cs
- TextEditor.cs
- WindowsFormsHostAutomationPeer.cs
- SerialPinChanges.cs
- ListParaClient.cs
- AggregateException.cs
- XsdCachingReader.cs
- ProfileManager.cs
- GradientStop.cs
- OleDbPermission.cs
- _LocalDataStoreMgr.cs
- WhitespaceRuleReader.cs
- XmlSchemaValidationException.cs
- CodeDomSerializationProvider.cs
- BoundPropertyEntry.cs
- Message.cs
- XsdValidatingReader.cs
- HttpResponseInternalWrapper.cs
- DeclarativeCatalogPart.cs
- TypeInitializationException.cs
- XmlKeywords.cs
- TextDecoration.cs
- FactoryRecord.cs
- compensatingcollection.cs
- ChangeInterceptorAttribute.cs
- Visual3D.cs
- ThrowHelper.cs
- DataGridViewRowsAddedEventArgs.cs
- HttpCapabilitiesBase.cs
- ListViewItemMouseHoverEvent.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- HttpHostedTransportConfiguration.cs
- InstanceKey.cs
- WpfWebRequestHelper.cs
- AuthenticationService.cs
- SchemaNotation.cs
- AmbientLight.cs
- LayoutSettings.cs
- SamlAction.cs
- UnsafeNativeMethods.cs
- ProviderConnectionPoint.cs
- ClaimSet.cs
- CoordinationService.cs
- DataGridColumnEventArgs.cs
- SqlRowUpdatingEvent.cs
- CryptoHandle.cs
- DataObject.cs
- WindowsProgressbar.cs
- NameTable.cs
- DeadCharTextComposition.cs
- StylusShape.cs
- FontWeights.cs
- InputScopeAttribute.cs
- RoleManagerModule.cs
- ColumnBinding.cs
- GuidelineCollection.cs
- XmlDocumentSchema.cs
- COM2Enum.cs
- LogicalExpr.cs
- OlePropertyStructs.cs
- FlatButtonAppearance.cs
- ProtocolElement.cs
- DecimalStorage.cs
- EventDescriptorCollection.cs
- ClientBuildManager.cs
- Comparer.cs
- OneToOneMappingSerializer.cs
- ExceptionUtil.cs
- CodeNamespaceImportCollection.cs
- TextRangeEditTables.cs
- DiscoveryInnerClientAdhoc11.cs