Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / Mail / HeaderCollection.cs / 1305376 / HeaderCollection.cs
using System; using System.Collections.Specialized; using System.Net.Mail; using System.Globalization; namespace System.Net.Mime { ////// Summary description for HeaderCollection. /// internal class HeaderCollection: NameValueCollection { MimeBasePart part = null; // default constructor // intentionally override the default comparer in the derived base class internal HeaderCollection() : base(StringComparer.OrdinalIgnoreCase) { } public override void Remove(string name) { if(Logging.On)Logging.PrintInfo(Logging.Web, this, "Remove", name); if (name == null) throw new ArgumentNullException("name"); if (name == string.Empty) throw new ArgumentException(SR.GetString(SR.net_emptystringcall,"name"), "name"); MailHeaderID id = MailHeaderInfo.GetID(name); if (id == MailHeaderID.ContentType && part != null) { part.ContentType = null; } else if (id == MailHeaderID.ContentDisposition && part is MimePart) { ((MimePart)part).ContentDisposition = null; } base.Remove(name); } public override string Get(string name) { if(Logging.On)Logging.PrintInfo(Logging.Web, this, "Get", name); if (name == null) throw new ArgumentNullException("name"); if (name == string.Empty) throw new ArgumentException(SR.GetString(SR.net_emptystringcall,"name"), "name"); MailHeaderID id = MailHeaderInfo.GetID(name); if (id == MailHeaderID.ContentType && part != null) { part.ContentType.PersistIfNeeded(this,false); } else if (id == MailHeaderID.ContentDisposition && part is MimePart) { ((MimePart)part).ContentDisposition.PersistIfNeeded(this, false); } return base.Get(name); } public override string[] GetValues(string name) { if(Logging.On)Logging.PrintInfo(Logging.Web, this, "Get", name); if (name == null) throw new ArgumentNullException("name"); if (name == string.Empty) throw new ArgumentException(SR.GetString(SR.net_emptystringcall,"name"), "name"); MailHeaderID id = MailHeaderInfo.GetID(name); if (id == MailHeaderID.ContentType && part != null) { part.ContentType.PersistIfNeeded(this,false); } else if (id == MailHeaderID.ContentDisposition && part is MimePart) { ((MimePart)part).ContentDisposition.PersistIfNeeded(this, false); } return base.GetValues(name); } internal void InternalRemove(string name){ base.Remove(name); } //set an existing header's value internal void InternalSet(string name, string value) { base.Set(name, value); } //add a new header and set its value internal void InternalAdd(string name, string value) { if (MailHeaderInfo.IsSingleton(name)) { base.Set(name, value); } else { base.Add(name, value); } } public override void Set(string name, string value) { if(Logging.On)Logging.PrintInfo(Logging.Web, this, "Set", name.ToString() + "=" + value.ToString()); if (name == null) throw new ArgumentNullException("name"); if (value == null) throw new ArgumentNullException("value"); if (name == string.Empty) throw new ArgumentException(SR.GetString(SR.net_emptystringcall,"name"), "name"); if (value == string.Empty) throw new ArgumentException(SR.GetString(SR.net_emptystringcall,"value"), "name"); if (!MimeBasePart.IsAscii(name,false)) { throw new FormatException(SR.GetString(SR.InvalidHeaderName)); } // normalize the case of well known headers name = MailHeaderInfo.NormalizeCase(name); MailHeaderID id = MailHeaderInfo.GetID(name); if (id == MailHeaderID.ContentType && part != null) { part.ContentType.Set(value.ToLower(CultureInfo.InvariantCulture), this); } else if (id == MailHeaderID.ContentDisposition && part is MimePart) { ((MimePart)part).ContentDisposition.Set(value.ToLower(CultureInfo.InvariantCulture), this); } else { base.Set(name, value); } } public override void Add(string name, string value) { if(Logging.On)Logging.PrintInfo(Logging.Web, this, "Add", name.ToString() + "=" + value.ToString()); if (name == null) throw new ArgumentNullException("name"); if (value == null) throw new ArgumentNullException("value"); if (name == string.Empty) throw new ArgumentException(SR.GetString(SR.net_emptystringcall,"name"), "name"); if (value == string.Empty) throw new ArgumentException(SR.GetString(SR.net_emptystringcall,"value"), "name"); MailBnfHelper.ValidateHeaderName(name); // normalize the case of well known headers name = MailHeaderInfo.NormalizeCase(name); MailHeaderID id = MailHeaderInfo.GetID(name); if(id == MailHeaderID.ContentType && part != null) { part.ContentType.Set(value.ToLower(CultureInfo.InvariantCulture), this); } else if (id == MailHeaderID.ContentDisposition && part is MimePart) { ((MimePart)part).ContentDisposition.Set(value.ToLower(CultureInfo.InvariantCulture), this); } else { InternalAdd(name, value); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
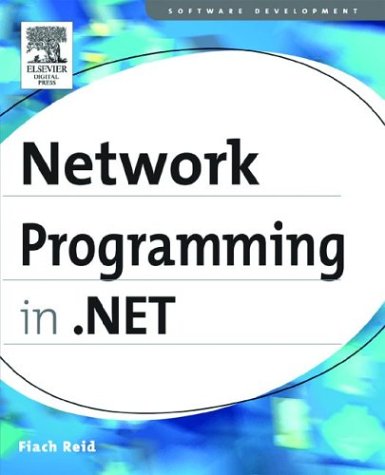
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileEnumerator.cs
- sqlnorm.cs
- TextHidden.cs
- ResourceContainerWrapper.cs
- ProjectionPathBuilder.cs
- ContentDefinition.cs
- EdmFunctions.cs
- MarkerProperties.cs
- listitem.cs
- ToolBarButtonDesigner.cs
- FileClassifier.cs
- DataContractSerializer.cs
- UseAttributeSetsAction.cs
- MouseEventArgs.cs
- MenuItemCollection.cs
- StructuredProperty.cs
- ShaperBuffers.cs
- HMACSHA512.cs
- TaskSchedulerException.cs
- XslAst.cs
- WpfPayload.cs
- OnOperation.cs
- TrackingQueryElement.cs
- IisTraceListener.cs
- Hashtable.cs
- PeerMessageDispatcher.cs
- ConcurrencyMode.cs
- wgx_render.cs
- FacetValueContainer.cs
- NonClientArea.cs
- HelpExampleGenerator.cs
- MasterPageCodeDomTreeGenerator.cs
- WorkflowInstance.cs
- Comparer.cs
- ActiveXHelper.cs
- DataObjectEventArgs.cs
- DiscoveryDocument.cs
- PrimitiveCodeDomSerializer.cs
- GPRECT.cs
- LineInfo.cs
- XmlQualifiedName.cs
- MouseCaptureWithinProperty.cs
- ScriptingRoleServiceSection.cs
- TextFormattingConverter.cs
- FixedTextSelectionProcessor.cs
- NameObjectCollectionBase.cs
- CompositionTarget.cs
- DataException.cs
- XamlPoint3DCollectionSerializer.cs
- ResetableIterator.cs
- DetailsViewPagerRow.cs
- ExtensionFile.cs
- Cursors.cs
- HttpConfigurationSystem.cs
- LocalValueEnumerator.cs
- TimeSpanSecondsConverter.cs
- QueryExpr.cs
- ListenerSingletonConnectionReader.cs
- StylusTip.cs
- PropertyInfoSet.cs
- DecoderReplacementFallback.cs
- ResourceCategoryAttribute.cs
- SqlNodeAnnotation.cs
- SchemaRegistration.cs
- xmlsaver.cs
- TemplateKey.cs
- FormsAuthenticationEventArgs.cs
- CurrentChangedEventManager.cs
- ConnectionManagementElementCollection.cs
- ExpressionBuilderCollection.cs
- MetadataCache.cs
- MessageQueue.cs
- Content.cs
- RegexTree.cs
- BinaryObjectWriter.cs
- MsmqOutputSessionChannel.cs
- ProfilePropertySettings.cs
- DetailsViewRowCollection.cs
- EventLog.cs
- Point3DCollection.cs
- ObjectList.cs
- base64Transforms.cs
- UriTemplateLiteralQueryValue.cs
- XmlDataFileEditor.cs
- Module.cs
- ApplicationActivator.cs
- WebBrowserUriTypeConverter.cs
- EditingCommands.cs
- CodeNamespaceImport.cs
- templategroup.cs
- HttpVersion.cs
- OperationAbortedException.cs
- DependsOnAttribute.cs
- Base64Encoder.cs
- UxThemeWrapper.cs
- NamedPermissionSet.cs
- ComboBoxRenderer.cs
- ClientConfigurationSystem.cs
- InvalidCommandTreeException.cs
- EpmSyndicationContentSerializer.cs