Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / Base64Encoder.cs / 1305376 / Base64Encoder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Text; using System.Diagnostics; namespace System.Xml { internal abstract class Base64Encoder { byte[] leftOverBytes; int leftOverBytesCount; char[] charsLine; internal const int Base64LineSize = 76; internal const int LineSizeInBytes = Base64LineSize/4*3; internal Base64Encoder() { charsLine = new char[Base64LineSize]; } internal abstract void WriteChars( char[] chars, int index, int count ); internal void Encode( byte[] buffer, int index, int count ) { if ( buffer == null ) { throw new ArgumentNullException( "buffer" ); } if ( index < 0 ) { throw new ArgumentOutOfRangeException( "index" ); } if ( count < 0 ) { throw new ArgumentOutOfRangeException( "count" ); } if ( count > buffer.Length - index ) { throw new ArgumentOutOfRangeException( "count" ); } // encode left-over buffer if( leftOverBytesCount > 0 ) { int i = leftOverBytesCount; while ( i < 3 && count > 0 ) { leftOverBytes[i++] = buffer[index++]; count--; } // the total number of buffer we have is less than 3 -> return if ( count == 0 && i < 3 ) { leftOverBytesCount = i; return; } // encode the left-over buffer and write out int leftOverChars = Convert.ToBase64CharArray( leftOverBytes, 0, 3, charsLine, 0 ); WriteChars( charsLine, 0, leftOverChars ); } // store new left-over buffer leftOverBytesCount = count % 3; if ( leftOverBytesCount > 0 ) { count -= leftOverBytesCount; if ( leftOverBytes == null ) { leftOverBytes = new byte[3]; } for( int i = 0; i < leftOverBytesCount; i++ ) { leftOverBytes[i] = buffer[ index + count + i ]; } } // encode buffer in 76 character long chunks int endIndex = index + count; int chunkSize = LineSizeInBytes; while( index < endIndex ) { if ( index + chunkSize > endIndex ) { chunkSize = endIndex - index; } int charCount = Convert.ToBase64CharArray( buffer, index, chunkSize, charsLine, 0 ); WriteChars( charsLine, 0, charCount ); index += chunkSize; } } internal void Flush() { if ( leftOverBytesCount > 0 ) { int leftOverChars = Convert.ToBase64CharArray( leftOverBytes, 0, leftOverBytesCount, charsLine, 0 ); WriteChars( charsLine, 0, leftOverChars ); leftOverBytesCount = 0; } } } internal class XmlRawWriterBase64Encoder : Base64Encoder { XmlRawWriter rawWriter; internal XmlRawWriterBase64Encoder( XmlRawWriter rawWriter ) { this.rawWriter = rawWriter; } internal override void WriteChars( char[] chars, int index, int count ) { rawWriter.WriteRaw( chars, index, count ); } } #if !SILVERLIGHT internal class XmlTextWriterBase64Encoder : Base64Encoder { XmlTextEncoder xmlTextEncoder; internal XmlTextWriterBase64Encoder( XmlTextEncoder xmlTextEncoder ) { this.xmlTextEncoder = xmlTextEncoder; } internal override void WriteChars( char[] chars, int index, int count ) { xmlTextEncoder.WriteRaw( chars, index, count ); } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Text; using System.Diagnostics; namespace System.Xml { internal abstract class Base64Encoder { byte[] leftOverBytes; int leftOverBytesCount; char[] charsLine; internal const int Base64LineSize = 76; internal const int LineSizeInBytes = Base64LineSize/4*3; internal Base64Encoder() { charsLine = new char[Base64LineSize]; } internal abstract void WriteChars( char[] chars, int index, int count ); internal void Encode( byte[] buffer, int index, int count ) { if ( buffer == null ) { throw new ArgumentNullException( "buffer" ); } if ( index < 0 ) { throw new ArgumentOutOfRangeException( "index" ); } if ( count < 0 ) { throw new ArgumentOutOfRangeException( "count" ); } if ( count > buffer.Length - index ) { throw new ArgumentOutOfRangeException( "count" ); } // encode left-over buffer if( leftOverBytesCount > 0 ) { int i = leftOverBytesCount; while ( i < 3 && count > 0 ) { leftOverBytes[i++] = buffer[index++]; count--; } // the total number of buffer we have is less than 3 -> return if ( count == 0 && i < 3 ) { leftOverBytesCount = i; return; } // encode the left-over buffer and write out int leftOverChars = Convert.ToBase64CharArray( leftOverBytes, 0, 3, charsLine, 0 ); WriteChars( charsLine, 0, leftOverChars ); } // store new left-over buffer leftOverBytesCount = count % 3; if ( leftOverBytesCount > 0 ) { count -= leftOverBytesCount; if ( leftOverBytes == null ) { leftOverBytes = new byte[3]; } for( int i = 0; i < leftOverBytesCount; i++ ) { leftOverBytes[i] = buffer[ index + count + i ]; } } // encode buffer in 76 character long chunks int endIndex = index + count; int chunkSize = LineSizeInBytes; while( index < endIndex ) { if ( index + chunkSize > endIndex ) { chunkSize = endIndex - index; } int charCount = Convert.ToBase64CharArray( buffer, index, chunkSize, charsLine, 0 ); WriteChars( charsLine, 0, charCount ); index += chunkSize; } } internal void Flush() { if ( leftOverBytesCount > 0 ) { int leftOverChars = Convert.ToBase64CharArray( leftOverBytes, 0, leftOverBytesCount, charsLine, 0 ); WriteChars( charsLine, 0, leftOverChars ); leftOverBytesCount = 0; } } } internal class XmlRawWriterBase64Encoder : Base64Encoder { XmlRawWriter rawWriter; internal XmlRawWriterBase64Encoder( XmlRawWriter rawWriter ) { this.rawWriter = rawWriter; } internal override void WriteChars( char[] chars, int index, int count ) { rawWriter.WriteRaw( chars, index, count ); } } #if !SILVERLIGHT internal class XmlTextWriterBase64Encoder : Base64Encoder { XmlTextEncoder xmlTextEncoder; internal XmlTextWriterBase64Encoder( XmlTextEncoder xmlTextEncoder ) { this.xmlTextEncoder = xmlTextEncoder; } internal override void WriteChars( char[] chars, int index, int count ) { xmlTextEncoder.WriteRaw( chars, index, count ); } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
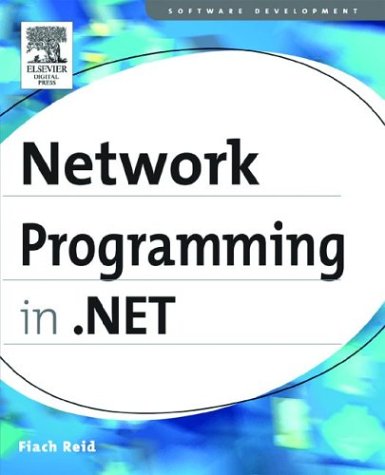
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlQualifiedName.cs
- MatrixIndependentAnimationStorage.cs
- DrawingGroup.cs
- SecurityResources.cs
- WebPartCollection.cs
- TextRunProperties.cs
- Variant.cs
- SortDescription.cs
- ApplicationInfo.cs
- DesignerVerbCollection.cs
- EFAssociationProvider.cs
- ServiceInfo.cs
- MatrixUtil.cs
- DESCryptoServiceProvider.cs
- WebPartMenuStyle.cs
- ZipIOExtraFieldPaddingElement.cs
- RequestBringIntoViewEventArgs.cs
- ObfuscateAssemblyAttribute.cs
- InfoCardArgumentException.cs
- ListControl.cs
- Thickness.cs
- FileSystemInfo.cs
- FragmentNavigationEventArgs.cs
- PrintDialogException.cs
- Point4DValueSerializer.cs
- NativeObjectSecurity.cs
- Rijndael.cs
- Path.cs
- TypeForwardedToAttribute.cs
- PropertyToken.cs
- remotingproxy.cs
- Wizard.cs
- AttributeConverter.cs
- ContainerUtilities.cs
- OracleCommandSet.cs
- FunctionNode.cs
- HandledEventArgs.cs
- Viewport3DAutomationPeer.cs
- DataServiceResponse.cs
- OpenTypeLayoutCache.cs
- FontWeights.cs
- ComponentDesigner.cs
- TrackPoint.cs
- Pair.cs
- MouseActionValueSerializer.cs
- RootBrowserWindow.cs
- querybuilder.cs
- TreeView.cs
- SynchronizingStream.cs
- SmiTypedGetterSetter.cs
- FrameworkReadOnlyPropertyMetadata.cs
- AmbientProperties.cs
- DataGridCell.cs
- CopyAttributesAction.cs
- Util.cs
- HashCodeCombiner.cs
- PageAsyncTask.cs
- TextProviderWrapper.cs
- NoResizeSelectionBorderGlyph.cs
- MemberListBinding.cs
- SqlUDTStorage.cs
- DataTransferEventArgs.cs
- EntityDataSourceDesignerHelper.cs
- Fault.cs
- httpstaticobjectscollection.cs
- DataPagerField.cs
- UnsafeNetInfoNativeMethods.cs
- ClaimTypes.cs
- MsmqProcessProtocolHandler.cs
- InvalidCastException.cs
- SQLByte.cs
- LogReserveAndAppendState.cs
- AssociationTypeEmitter.cs
- ObjectDataSource.cs
- StorageTypeMapping.cs
- XMLSchema.cs
- PropertyPushdownHelper.cs
- HebrewCalendar.cs
- ColumnHeader.cs
- ScrollBar.cs
- DesignDataSource.cs
- DbDeleteCommandTree.cs
- InfiniteTimeSpanConverter.cs
- TextModifier.cs
- Canvas.cs
- FullTextState.cs
- StorageEntityContainerMapping.cs
- DocumentPageTextView.cs
- XDRSchema.cs
- XmlHierarchicalDataSourceView.cs
- _IPv6Address.cs
- GifBitmapEncoder.cs
- UniqueIdentifierService.cs
- WorkflowServiceBuildProvider.cs
- ErrorWrapper.cs
- ProvideValueServiceProvider.cs
- ListManagerBindingsCollection.cs
- ClaimSet.cs
- TiffBitmapDecoder.cs
- CheckPair.cs