Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / Collections / Specialized / NameValueCollection.cs / 1305376 / NameValueCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Ordered String/String[] collection of name/value pairs with support for null key * Wraps NameObject collection * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Collections.Specialized { using Microsoft.Win32; using System.Collections; using System.Runtime.Serialization; using System.Text; using System.Globalization; ////// [Serializable()] public class NameValueCollection : NameObjectCollectionBase { private String[] _all; private String[] _allKeys; // // Constructors // ///Represents a sorted collection of associated ///keys and values that /// can be accessed either with the hash code of the key or with the index. /// public NameValueCollection() : base() { } ///Creates an empty ///with the default initial capacity /// and using the default case-insensitive hash code provider and the default /// case-insensitive comparer. /// public NameValueCollection(NameValueCollection col) : base( col != null? col.Comparer : null) { Add(col); } ///Copies the entries from the specified ///to a new with the same initial capacity as /// the number of entries copied and using the default case-insensitive hash code /// provider and the default case-insensitive comparer. /// #pragma warning disable 618 [Obsolete("Please use NameValueCollection(IEqualityComparer) instead.")] public NameValueCollection(IHashCodeProvider hashProvider, IComparer comparer) : base(hashProvider, comparer) { } #pragma warning restore 618 ///Creates an empty ///with the default initial capacity /// and using the specified case-insensitive hash code provider and the specified /// case-insensitive comparer. /// public NameValueCollection(int capacity) : base(capacity) { } public NameValueCollection(IEqualityComparer equalityComparer) : base( equalityComparer) { } public NameValueCollection(Int32 capacity, IEqualityComparer equalityComparer) : base(capacity, equalityComparer) { } ///Creates an empty ///with /// the specified initial capacity and using the default case-insensitive hash code /// provider and the default case-insensitive comparer. /// public NameValueCollection(int capacity, NameValueCollection col) : base(capacity, (col != null ? col.Comparer : null)) { if( col == null) { throw new ArgumentNullException("col"); } this.Comparer = col.Comparer; Add(col); } ///Copies the entries from the specified ///to a new with the specified initial capacity or the /// same initial capacity as the number of entries copied, whichever is greater, and /// using the default case-insensitive hash code provider and the default /// case-insensitive comparer. /// #pragma warning disable 618 [Obsolete("Please use NameValueCollection(Int32, IEqualityComparer) instead.")] public NameValueCollection(int capacity, IHashCodeProvider hashProvider, IComparer comparer) : base(capacity, hashProvider, comparer) { } #pragma warning restore 618 // Allow internal extenders to avoid creating the hashtable/arraylist. internal NameValueCollection(DBNull dummy) : base(dummy) { } // // Serialization support // ///Creates an empty ///with the specified initial capacity and /// using the specified case-insensitive hash code provider and the specified /// case-insensitive comparer. /// protected NameValueCollection(SerializationInfo info, StreamingContext context) : base(info, context) { } // // Helper methods // ///[To be supplied.] ////// protected void InvalidateCachedArrays() { _all = null; _allKeys = null; } private static String GetAsOneString(ArrayList list) { int n = (list != null) ? list.Count : 0; if (n == 1) { return (String)list[0]; } else if (n > 1) { StringBuilder s = new StringBuilder((String)list[0]); for (int i = 1; i < n; i++) { s.Append(','); s.Append((String)list[i]); } return s.ToString(); } else { return null; } } private static String[] GetAsStringArray(ArrayList list) { int n = (list != null) ? list.Count : 0; if (n == 0) return null; String [] array = new String[n]; list.CopyTo(0, array, 0, n); return array; } // // Misc public APIs // ///Resets the cached arrays of the collection to ///. /// public void Add(NameValueCollection c) { if( c == null) { throw new ArgumentNullException("c"); } InvalidateCachedArrays(); int n = c.Count; for (int i = 0; i < n; i++) { String key = c.GetKey(i); String[] values = c.GetValues(i); if (values != null) { for (int j = 0; j < values.Length; j++) Add(key, values[j]); } else { Add(key, null); } } } ///Copies the entries in the specified ///to the current . /// public virtual void Clear() { if (IsReadOnly) throw new NotSupportedException(SR.GetString(SR.CollectionReadOnly)); InvalidateCachedArrays(); BaseClear(); } public void CopyTo(Array dest, int index) { if (dest==null) { throw new ArgumentNullException("dest"); } if (dest.Rank != 1) { throw new ArgumentException(SR.GetString(SR.Arg_MultiRank)); } if (index < 0) { throw new ArgumentOutOfRangeException("index",SR.GetString(SR.IndexOutOfRange, index.ToString(CultureInfo.CurrentCulture)) ); } if (dest.Length - index < Count) { throw new ArgumentException(SR.GetString(SR.Arg_InsufficientSpace)); } int n = Count; if (_all == null) { _all = new String[n]; for (int i = 0; i < n; i++) { _all[i] = Get(i); dest.SetValue( _all[i], i + index); } } else { for (int i = 0; i < n; i++) { dest.SetValue( _all[i], i + index); } } } ///Invalidates the cached arrays and removes all entries /// from the ///. /// public bool HasKeys() { return InternalHasKeys(); } ///Gets a value indicating whether the ///contains entries whose keys are not . /// internal virtual bool InternalHasKeys() { return BaseHasKeys(); } // // Access by name // ///Allows derived classes to alter HasKeys(). ////// public virtual void Add(String name, String value) { if (IsReadOnly) throw new NotSupportedException(SR.GetString(SR.CollectionReadOnly)); InvalidateCachedArrays(); ArrayList values = (ArrayList)BaseGet(name); if (values == null) { // new key - add new key with single value values = new ArrayList(1); if (value != null) values.Add(value); BaseAdd(name, values); } else { // old key -- append value to the list of values if (value != null) values.Add(value); } } ///Adds an entry with the specified name and value into the /// ///. /// public virtual String Get(String name) { ArrayList values = (ArrayList)BaseGet(name); return GetAsOneString(values); } ///Gets the values associated with the specified key from the ///combined into one comma-separated list. /// public virtual String[] GetValues(String name) { ArrayList values = (ArrayList)BaseGet(name); return GetAsStringArray(values); } ///Gets the values associated with the specified key from the ///. /// public virtual void Set(String name, String value) { if (IsReadOnly) throw new NotSupportedException(SR.GetString(SR.CollectionReadOnly)); InvalidateCachedArrays(); ArrayList values = new ArrayList(1); values.Add(value); BaseSet(name, values); } ///Adds a value to an entry in the ///. /// public virtual void Remove(String name) { InvalidateCachedArrays(); BaseRemove(name); } ///Removes the entries with the specified key from the ///instance. /// public String this[String name] { get { return Get(name); } set { Set(name, value); } } // // Indexed access // ///Represents the entry with the specified key in the /// ///. /// public virtual String Get(int index) { ArrayList values = (ArrayList)BaseGet(index); return GetAsOneString(values); } ////// Gets the values at the specified index of the ///combined into one /// comma-separated list. /// public virtual String[] GetValues(int index) { ArrayList values = (ArrayList)BaseGet(index); return GetAsStringArray(values); } ///Gets the values at the specified index of the ///. /// public virtual String GetKey(int index) { return BaseGetKey(index); } ///Gets the key at the specified index of the ///. /// public String this[int index] { get { return Get(index); } } // // Access to keys and values as arrays // ///Represents the entry at the specified index of the ///. /// public virtual String[] AllKeys { get { if (_allKeys == null) _allKeys = BaseGetAllKeys(); return _allKeys; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Gets all the keys in the ///. // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Ordered String/String[] collection of name/value pairs with support for null key * Wraps NameObject collection * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Collections.Specialized { using Microsoft.Win32; using System.Collections; using System.Runtime.Serialization; using System.Text; using System.Globalization; ////// [Serializable()] public class NameValueCollection : NameObjectCollectionBase { private String[] _all; private String[] _allKeys; // // Constructors // ///Represents a sorted collection of associated ///keys and values that /// can be accessed either with the hash code of the key or with the index. /// public NameValueCollection() : base() { } ///Creates an empty ///with the default initial capacity /// and using the default case-insensitive hash code provider and the default /// case-insensitive comparer. /// public NameValueCollection(NameValueCollection col) : base( col != null? col.Comparer : null) { Add(col); } ///Copies the entries from the specified ///to a new with the same initial capacity as /// the number of entries copied and using the default case-insensitive hash code /// provider and the default case-insensitive comparer. /// #pragma warning disable 618 [Obsolete("Please use NameValueCollection(IEqualityComparer) instead.")] public NameValueCollection(IHashCodeProvider hashProvider, IComparer comparer) : base(hashProvider, comparer) { } #pragma warning restore 618 ///Creates an empty ///with the default initial capacity /// and using the specified case-insensitive hash code provider and the specified /// case-insensitive comparer. /// public NameValueCollection(int capacity) : base(capacity) { } public NameValueCollection(IEqualityComparer equalityComparer) : base( equalityComparer) { } public NameValueCollection(Int32 capacity, IEqualityComparer equalityComparer) : base(capacity, equalityComparer) { } ///Creates an empty ///with /// the specified initial capacity and using the default case-insensitive hash code /// provider and the default case-insensitive comparer. /// public NameValueCollection(int capacity, NameValueCollection col) : base(capacity, (col != null ? col.Comparer : null)) { if( col == null) { throw new ArgumentNullException("col"); } this.Comparer = col.Comparer; Add(col); } ///Copies the entries from the specified ///to a new with the specified initial capacity or the /// same initial capacity as the number of entries copied, whichever is greater, and /// using the default case-insensitive hash code provider and the default /// case-insensitive comparer. /// #pragma warning disable 618 [Obsolete("Please use NameValueCollection(Int32, IEqualityComparer) instead.")] public NameValueCollection(int capacity, IHashCodeProvider hashProvider, IComparer comparer) : base(capacity, hashProvider, comparer) { } #pragma warning restore 618 // Allow internal extenders to avoid creating the hashtable/arraylist. internal NameValueCollection(DBNull dummy) : base(dummy) { } // // Serialization support // ///Creates an empty ///with the specified initial capacity and /// using the specified case-insensitive hash code provider and the specified /// case-insensitive comparer. /// protected NameValueCollection(SerializationInfo info, StreamingContext context) : base(info, context) { } // // Helper methods // ///[To be supplied.] ////// protected void InvalidateCachedArrays() { _all = null; _allKeys = null; } private static String GetAsOneString(ArrayList list) { int n = (list != null) ? list.Count : 0; if (n == 1) { return (String)list[0]; } else if (n > 1) { StringBuilder s = new StringBuilder((String)list[0]); for (int i = 1; i < n; i++) { s.Append(','); s.Append((String)list[i]); } return s.ToString(); } else { return null; } } private static String[] GetAsStringArray(ArrayList list) { int n = (list != null) ? list.Count : 0; if (n == 0) return null; String [] array = new String[n]; list.CopyTo(0, array, 0, n); return array; } // // Misc public APIs // ///Resets the cached arrays of the collection to ///. /// public void Add(NameValueCollection c) { if( c == null) { throw new ArgumentNullException("c"); } InvalidateCachedArrays(); int n = c.Count; for (int i = 0; i < n; i++) { String key = c.GetKey(i); String[] values = c.GetValues(i); if (values != null) { for (int j = 0; j < values.Length; j++) Add(key, values[j]); } else { Add(key, null); } } } ///Copies the entries in the specified ///to the current . /// public virtual void Clear() { if (IsReadOnly) throw new NotSupportedException(SR.GetString(SR.CollectionReadOnly)); InvalidateCachedArrays(); BaseClear(); } public void CopyTo(Array dest, int index) { if (dest==null) { throw new ArgumentNullException("dest"); } if (dest.Rank != 1) { throw new ArgumentException(SR.GetString(SR.Arg_MultiRank)); } if (index < 0) { throw new ArgumentOutOfRangeException("index",SR.GetString(SR.IndexOutOfRange, index.ToString(CultureInfo.CurrentCulture)) ); } if (dest.Length - index < Count) { throw new ArgumentException(SR.GetString(SR.Arg_InsufficientSpace)); } int n = Count; if (_all == null) { _all = new String[n]; for (int i = 0; i < n; i++) { _all[i] = Get(i); dest.SetValue( _all[i], i + index); } } else { for (int i = 0; i < n; i++) { dest.SetValue( _all[i], i + index); } } } ///Invalidates the cached arrays and removes all entries /// from the ///. /// public bool HasKeys() { return InternalHasKeys(); } ///Gets a value indicating whether the ///contains entries whose keys are not . /// internal virtual bool InternalHasKeys() { return BaseHasKeys(); } // // Access by name // ///Allows derived classes to alter HasKeys(). ////// public virtual void Add(String name, String value) { if (IsReadOnly) throw new NotSupportedException(SR.GetString(SR.CollectionReadOnly)); InvalidateCachedArrays(); ArrayList values = (ArrayList)BaseGet(name); if (values == null) { // new key - add new key with single value values = new ArrayList(1); if (value != null) values.Add(value); BaseAdd(name, values); } else { // old key -- append value to the list of values if (value != null) values.Add(value); } } ///Adds an entry with the specified name and value into the /// ///. /// public virtual String Get(String name) { ArrayList values = (ArrayList)BaseGet(name); return GetAsOneString(values); } ///Gets the values associated with the specified key from the ///combined into one comma-separated list. /// public virtual String[] GetValues(String name) { ArrayList values = (ArrayList)BaseGet(name); return GetAsStringArray(values); } ///Gets the values associated with the specified key from the ///. /// public virtual void Set(String name, String value) { if (IsReadOnly) throw new NotSupportedException(SR.GetString(SR.CollectionReadOnly)); InvalidateCachedArrays(); ArrayList values = new ArrayList(1); values.Add(value); BaseSet(name, values); } ///Adds a value to an entry in the ///. /// public virtual void Remove(String name) { InvalidateCachedArrays(); BaseRemove(name); } ///Removes the entries with the specified key from the ///instance. /// public String this[String name] { get { return Get(name); } set { Set(name, value); } } // // Indexed access // ///Represents the entry with the specified key in the /// ///. /// public virtual String Get(int index) { ArrayList values = (ArrayList)BaseGet(index); return GetAsOneString(values); } ////// Gets the values at the specified index of the ///combined into one /// comma-separated list. /// public virtual String[] GetValues(int index) { ArrayList values = (ArrayList)BaseGet(index); return GetAsStringArray(values); } ///Gets the values at the specified index of the ///. /// public virtual String GetKey(int index) { return BaseGetKey(index); } ///Gets the key at the specified index of the ///. /// public String this[int index] { get { return Get(index); } } // // Access to keys and values as arrays // ///Represents the entry at the specified index of the ///. /// public virtual String[] AllKeys { get { if (_allKeys == null) _allKeys = BaseGetAllKeys(); return _allKeys; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Gets all the keys in the ///.
Link Menu
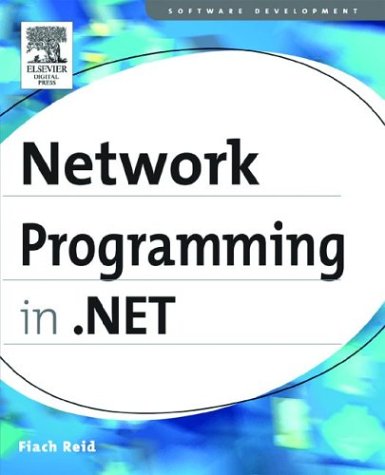
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TrackingAnnotationCollection.cs
- FrameworkReadOnlyPropertyMetadata.cs
- ToolStripItemGlyph.cs
- EventListener.cs
- ControlTemplate.cs
- DocComment.cs
- RuntimeHandles.cs
- BuildResultCache.cs
- JpegBitmapEncoder.cs
- SystemIPGlobalProperties.cs
- ClientConfigurationHost.cs
- XPathNode.cs
- SQLInt64Storage.cs
- ComponentGlyph.cs
- TrackingMemoryStream.cs
- DataGrid.cs
- XmlIlTypeHelper.cs
- WeakReferenceList.cs
- RecordBuilder.cs
- EventProxy.cs
- GridEntryCollection.cs
- ConnectionPoint.cs
- XamlSerializerUtil.cs
- DynamicValidatorEventArgs.cs
- DataGridViewCellCancelEventArgs.cs
- NewArray.cs
- TextDecorations.cs
- TypeContext.cs
- DesignerWidgets.cs
- ForEachAction.cs
- SqlRowUpdatingEvent.cs
- Cell.cs
- ListViewUpdateEventArgs.cs
- IssuedTokenParametersElement.cs
- NativeMethods.cs
- TransactionState.cs
- DataGridColumn.cs
- Speller.cs
- InvokeDelegate.cs
- KeyGestureConverter.cs
- SingleKeyFrameCollection.cs
- RoleService.cs
- SettingsAttributes.cs
- PropertyPathWorker.cs
- StartUpEventArgs.cs
- smtpconnection.cs
- CodeTypeDeclarationCollection.cs
- HistoryEventArgs.cs
- LoadedOrUnloadedOperation.cs
- CalendarDay.cs
- ListViewPagedDataSource.cs
- UInt32Converter.cs
- ObjectSecurityT.cs
- StylusPointPropertyInfo.cs
- SystemNetworkInterface.cs
- DiscoveryServiceExtension.cs
- SqlFlattener.cs
- NavigationPropertyEmitter.cs
- ExpressionWriter.cs
- ConfigurationStrings.cs
- RedistVersionInfo.cs
- MsmqMessageProperty.cs
- EntitySetBase.cs
- CompoundFileReference.cs
- SpeechSynthesizer.cs
- NavigationProgressEventArgs.cs
- tibetanshape.cs
- ObfuscationAttribute.cs
- querybuilder.cs
- SortDescription.cs
- GraphicsContext.cs
- SecurityDescriptor.cs
- ListDataHelper.cs
- DataGridViewComboBoxEditingControl.cs
- ProjectionCamera.cs
- BoundingRectTracker.cs
- SingleTagSectionHandler.cs
- PopupControlService.cs
- DateRangeEvent.cs
- QueryStoreStatusRequest.cs
- XmlAttribute.cs
- NativeMethods.cs
- CodeAttributeDeclarationCollection.cs
- updateconfighost.cs
- BitmapCodecInfoInternal.cs
- WsdlInspector.cs
- columnmapfactory.cs
- BaseParaClient.cs
- SerialReceived.cs
- ObjectAnimationBase.cs
- BitmapEncoder.cs
- DbLambda.cs
- XsltContext.cs
- SeekStoryboard.cs
- objectquery_tresulttype.cs
- WebPartDeleteVerb.cs
- EventHandlingScope.cs
- RbTree.cs
- SystemFonts.cs
- basecomparevalidator.cs