Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / Unit.cs / 1305376 / Unit.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Globalization; using System.ComponentModel; using System.ComponentModel.Design; using System.Security.Permissions; using System.Web.Util; [ TypeConverterAttribute(typeof(UnitConverter)) ] [Serializable] public struct Unit { ////// Specifies an empty unit. /// public static readonly Unit Empty = new Unit(); internal const int MaxValue = 32767; internal const int MinValue = -32768; private readonly UnitType type; private readonly double value; ////// public Unit(int value) { if ((value < MinValue) || (value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } this.value = value; this.type = UnitType.Pixel; } ///Initializes a new instance of the ///structure with the specified 32-bit signed integer as /// the unit value and as the (default) unit type. /// public Unit(double value) { if ((value < MinValue) || (value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } this.value = (int)value; this.type = UnitType.Pixel; } ///Initializes a new instance of the ///structure with the /// specified double-precision /// floating point number as the unit value and /// as the (default) unit type. /// public Unit(double value, UnitType type) { if ((value < MinValue) || (value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } if (type == UnitType.Pixel) { this.value = (int)value; } else { this.value = value; } this.type = type; } ///Initializes a new instance of the ///structure with the specified /// double-precision floating point number as the unit value and the specified /// as the unit type. /// public Unit(string value) : this(value, CultureInfo.CurrentCulture, UnitType.Pixel) { } ///Initializes a new instance of the ///structure with the specified text /// string that contains the unit value and unit type. If the unit type is not /// specified, the default is /// . /// public Unit(string value, CultureInfo culture) : this(value, culture, UnitType.Pixel) { } internal Unit(string value, CultureInfo culture, UnitType defaultType) { if (String.IsNullOrEmpty(value)) { this.value = 0; this.type = (UnitType)0; } else { if (culture == null) { culture = CultureInfo.CurrentCulture; } // This is invariant because it acts like an enum with a number together. // The enum part is invariant, but the number uses current culture. string trimLcase = value.Trim().ToLower(CultureInfo.InvariantCulture); int len = trimLcase.Length; int lastDigit = -1; for (int i = 0; i < len; i++) { char ch = trimLcase[i]; if (((ch < '0') || (ch > '9')) && (ch != '-') && (ch != '.') && (ch != ',')) break; lastDigit = i; } if (lastDigit == -1) { throw new FormatException(SR.GetString(SR.UnitParseNoDigits, value)); } if (lastDigit < len - 1) { type = (UnitType)GetTypeFromString(trimLcase.Substring(lastDigit+1).Trim()); } else { type = defaultType; } string numericPart = trimLcase.Substring(0, lastDigit+1); // Cannot use Double.FromString, because we don't use it in the ToString implementation try { TypeConverter converter = new SingleConverter(); this.value = (Single)converter.ConvertFromString(null, culture, numericPart); if (type == UnitType.Pixel) { this.value = (int)this.value; } } catch { throw new FormatException(SR.GetString(SR.UnitParseNumericPart, value, numericPart, type.ToString("G"))); } if ((this.value < MinValue) || (this.value > MaxValue)) { throw new ArgumentOutOfRangeException("value"); } } } ///[To be supplied.] ////// public bool IsEmpty { get { return type == (UnitType)0; } } ///Gets a value indicating whether the ///is empty. /// public UnitType Type { get { if (!IsEmpty) { return this.type; } else { return UnitType.Pixel; } } } ///Gets or sets the type of the ///. /// public double Value { get { return this.value; } } ///Gets the value of the ///. /// public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(type.GetHashCode(), value.GetHashCode()); } ///[To be supplied.] ////// public override bool Equals(object obj) { if (obj == null || !(obj is Unit)) { return false; } Unit u = (Unit)obj; // compare internal values to avoid "defaulting" in the case of "Empty" // if (u.type == type && u.value == value) { return true; } return false; } ///Compares this ///with the specified object. /// public static bool operator ==(Unit left, Unit right) { // compare internal values to avoid "defaulting" in the case of "Empty" // return (left.type == right.type && left.value == right.value); } ///Compares two units to find out if they have the same value and type. ////// public static bool operator !=(Unit left, Unit right) { // compare internal values to avoid "defaulting" in the case of "Empty" // return (left.type != right.type || left.value != right.value); } ///Compares two units to find out if they have different /// values and/or types. ////// Converts UnitType to persistence string. /// private static string GetStringFromType(UnitType type) { switch (type) { case UnitType.Pixel: return "px"; case UnitType.Point: return "pt"; case UnitType.Pica: return "pc"; case UnitType.Inch: return "in"; case UnitType.Mm: return "mm"; case UnitType.Cm: return "cm"; case UnitType.Percentage: return "%"; case UnitType.Em: return "em"; case UnitType.Ex: return "ex"; } return String.Empty; } ////// Converts persistence string to UnitType. /// private static UnitType GetTypeFromString(string value) { if (!String.IsNullOrEmpty(value)) { if (value.Equals("px")) { return UnitType.Pixel; } else if (value.Equals("pt")) { return UnitType.Point; } else if (value.Equals("%")) { return UnitType.Percentage; } else if (value.Equals("pc")) { return UnitType.Pica; } else if (value.Equals("in")) { return UnitType.Inch; } else if (value.Equals("mm")) { return UnitType.Mm; } else if (value.Equals("cm")) { return UnitType.Cm; } else if (value.Equals("em")) { return UnitType.Em; } else if (value.Equals("ex")) { return UnitType.Ex; } else { throw new ArgumentOutOfRangeException("value"); } } return UnitType.Pixel; } ////// public static Unit Parse(string s) { return new Unit(s, CultureInfo.CurrentCulture); } ///[To be supplied.] ////// public static Unit Parse(string s, CultureInfo culture) { return new Unit(s, culture); } ///[To be supplied.] ////// public static Unit Percentage(double n) { return new Unit(n,UnitType.Percentage); } ///Creates a ///of type from the specified 32-bit signed integer. /// public static Unit Pixel(int n) { return new Unit(n); } ///Creates a ///of type from the specified 32-bit signed integer. /// public static Unit Point(int n) { return new Unit(n,UnitType.Point); } ///Creates a ///of type from the /// specified 32-bit signed integer. /// /// public override string ToString() { return ToString((IFormatProvider)CultureInfo.CurrentCulture); } public string ToString(CultureInfo culture) { return ToString((IFormatProvider)culture); } public string ToString(IFormatProvider formatProvider) { if (IsEmpty) return String.Empty; // Double.ToString does not do the right thing, we get extra bits at the end string valuePart; if (type == UnitType.Pixel) { valuePart = ((int)value).ToString(formatProvider); } else { valuePart = ((float)value).ToString(formatProvider); } return valuePart + Unit.GetStringFromType(type); } ///Converts a ///to a . /// public static implicit operator Unit(int n) { return Unit.Pixel(n); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Implicitly creates a ///of type from the specified 32-bit unsigned integer.
Link Menu
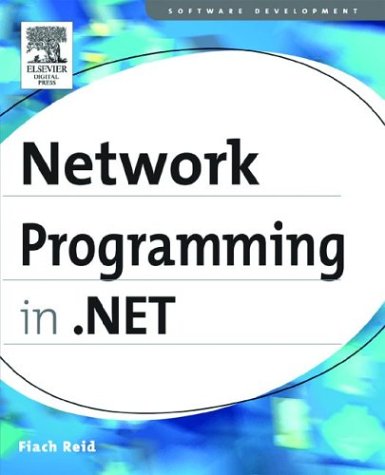
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinaryWriter.cs
- ServiceOperation.cs
- ICollection.cs
- Configuration.cs
- SmiMetaData.cs
- MLangCodePageEncoding.cs
- RTTypeWrapper.cs
- ResourcePart.cs
- Range.cs
- EmptyEnumerable.cs
- LinkArea.cs
- EditorPartChrome.cs
- SHA1CryptoServiceProvider.cs
- CompiledXpathExpr.cs
- ScriptingWebServicesSectionGroup.cs
- ProviderUtil.cs
- DeclaredTypeValidatorAttribute.cs
- XmlDataSourceView.cs
- OleDbParameter.cs
- XmlTextAttribute.cs
- basevalidator.cs
- WindowsIdentity.cs
- SamlAuthorizationDecisionClaimResource.cs
- PropertyValueChangedEvent.cs
- MessageQueueException.cs
- AnnotationResource.cs
- ExtendedProtectionPolicyElement.cs
- ISessionStateStore.cs
- XmlDataSourceView.cs
- IProvider.cs
- FormsAuthenticationConfiguration.cs
- ListViewSortEventArgs.cs
- DecimalStorage.cs
- WinInet.cs
- ActivityCollectionMarkupSerializer.cs
- CompatibleComparer.cs
- QueryStringParameter.cs
- GradientStop.cs
- EntityDataSourceContextDisposingEventArgs.cs
- ComboBoxRenderer.cs
- XmlResolver.cs
- GenericAuthenticationEventArgs.cs
- XmlAttribute.cs
- VisualProxy.cs
- MinimizableAttributeTypeConverter.cs
- User.cs
- ObjectDataSourceChooseMethodsPanel.cs
- DataObjectAttribute.cs
- DataGridViewHitTestInfo.cs
- HttpClientChannel.cs
- BrushValueSerializer.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- ConfigurationStrings.cs
- ConfigXmlElement.cs
- QilDataSource.cs
- Invariant.cs
- RSAPKCS1KeyExchangeFormatter.cs
- TreeNode.cs
- DataServiceRequestOfT.cs
- CustomWebEventKey.cs
- ProfileBuildProvider.cs
- IndexerNameAttribute.cs
- TypeListConverter.cs
- hwndwrapper.cs
- Utils.cs
- FormViewCommandEventArgs.cs
- SingleBodyParameterMessageFormatter.cs
- XmlComment.cs
- DynamicMethod.cs
- RuntimeArgumentHandle.cs
- MbpInfo.cs
- XPathNavigatorKeyComparer.cs
- ZipIOBlockManager.cs
- ProfileSettings.cs
- ByteKeyFrameCollection.cs
- XmlUrlResolver.cs
- InternalConfigEventArgs.cs
- IDataContractSurrogate.cs
- DataError.cs
- FileUtil.cs
- TableLayoutSettings.cs
- CustomAttributeSerializer.cs
- DataChangedEventManager.cs
- _TimerThread.cs
- Composition.cs
- UIElement3D.cs
- SqlConnectionFactory.cs
- InstalledFontCollection.cs
- PrintDocument.cs
- CommandValueSerializer.cs
- CommandLibraryHelper.cs
- CancelEventArgs.cs
- UrlMappingsModule.cs
- Attributes.cs
- XmlResolver.cs
- ConnectionStringSettings.cs
- WebEvents.cs
- _ConnectionGroup.cs
- DynamicObject.cs
- EdmComplexTypeAttribute.cs