Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Configuration / FormsAuthenticationConfiguration.cs / 3 / FormsAuthenticationConfiguration.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /***************************************************************************** From machine.config******************************************************************************/ namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class FormsAuthenticationConfiguration : ConfigurationElement { private static readonly ConfigurationElementProperty s_elemProperty = new ConfigurationElementProperty(new CallbackValidator(typeof(FormsAuthenticationConfiguration), Validate)); private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propCredentials = new ConfigurationProperty("credentials", typeof(FormsAuthenticationCredentials), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propName = new ConfigurationProperty("name", typeof(string), ".ASPXAUTH", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propLoginUrl = new ConfigurationProperty("loginUrl", typeof(string), "login.aspx", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDefaultUrl = new ConfigurationProperty("defaultUrl", typeof(string), "default.aspx", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProtection = new ConfigurationProperty("protection", typeof(FormsProtectionEnum), FormsProtectionEnum.All, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propTimeout = new ConfigurationProperty("timeout", typeof(TimeSpan), TimeSpan.FromMinutes(30.0), StdValidatorsAndConverters.TimeSpanMinutesConverter, new TimeSpanValidator(TimeSpan.FromMinutes(1), TimeSpan.MaxValue), ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propPath = new ConfigurationProperty("path", typeof(string), "/", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propRequireSSL = new ConfigurationProperty("requireSSL", typeof(bool), false, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propSlidingExpiration = new ConfigurationProperty("slidingExpiration", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propCookieless = new ConfigurationProperty("cookieless", typeof(HttpCookieMode), HttpCookieMode.UseDeviceProfile, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDomain = new ConfigurationProperty("domain", typeof(string), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propEnableCrossAppRedirects = new ConfigurationProperty("enableCrossAppRedirects", typeof(bool), false, ConfigurationPropertyOptions.None); static FormsAuthenticationConfiguration() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propCredentials); _properties.Add(_propName); _properties.Add(_propLoginUrl); _properties.Add(_propDefaultUrl); _properties.Add(_propProtection); _properties.Add(_propTimeout); _properties.Add(_propPath); _properties.Add(_propRequireSSL); _properties.Add(_propSlidingExpiration); _properties.Add(_propCookieless); _properties.Add(_propDomain); _properties.Add(_propEnableCrossAppRedirects); } public FormsAuthenticationConfiguration() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("credentials")] public FormsAuthenticationCredentials Credentials { get { return (FormsAuthenticationCredentials)base[_propCredentials]; } } [ConfigurationProperty("name", DefaultValue = ".ASPXAUTH")] [StringValidator(MinLength = 1)] public string Name { get { return (string)base[_propName]; } set { if (String.IsNullOrEmpty(value)) { base[_propName] = _propName.DefaultValue; } else { base[_propName] = value; } } } [ConfigurationProperty("loginUrl", DefaultValue = "login.aspx")] [StringValidator(MinLength = 1)] public string LoginUrl { get { return (string)base[_propLoginUrl]; } set { if (String.IsNullOrEmpty(value)) { base[_propLoginUrl] = _propLoginUrl.DefaultValue; } else { base[_propLoginUrl] = value; } } } [ConfigurationProperty("defaultUrl", DefaultValue = "default.aspx")] [StringValidator(MinLength = 1)] public string DefaultUrl { get { return (string)base[_propDefaultUrl]; } set { if (String.IsNullOrEmpty(value)) { base[_propDefaultUrl] = _propDefaultUrl.DefaultValue; } else { base[_propDefaultUrl] = value; } } } [ConfigurationProperty("protection", DefaultValue = FormsProtectionEnum.All)] public FormsProtectionEnum Protection { get { return (FormsProtectionEnum)base[_propProtection]; } set { base[_propProtection] = value; } } [ConfigurationProperty("timeout", DefaultValue = "00:30:00")] [TimeSpanValidator(MinValueString="00:01:00", MaxValueString=TimeSpanValidatorAttribute.TimeSpanMaxValue)] [TypeConverter(typeof(TimeSpanMinutesConverter))] public TimeSpan Timeout { get { return (TimeSpan)base[_propTimeout]; } set { base[_propTimeout] = value; } } [ConfigurationProperty("path", DefaultValue = "/")] [StringValidator(MinLength = 1)] public string Path { get { return (string)base[_propPath]; } set { if (String.IsNullOrEmpty(value)) { base[_propPath] = _propPath.DefaultValue; } else { base[_propPath] = value; } } } [ConfigurationProperty("requireSSL", DefaultValue = false)] public bool RequireSSL { get { return (bool)base[_propRequireSSL]; } set { base[_propRequireSSL] = value; } } [ConfigurationProperty("slidingExpiration", DefaultValue = true)] public bool SlidingExpiration { get { return (bool)base[_propSlidingExpiration]; } set { base[_propSlidingExpiration] = value; } } [ConfigurationProperty("enableCrossAppRedirects", DefaultValue = false)] public bool EnableCrossAppRedirects { get { return (bool)base[_propEnableCrossAppRedirects]; } set { base[_propEnableCrossAppRedirects] = value; } } [ConfigurationProperty("cookieless", DefaultValue = HttpCookieMode.UseDeviceProfile)] public HttpCookieMode Cookieless { get { return (HttpCookieMode)base[_propCookieless]; } set { base[_propCookieless] = value; } } [ConfigurationProperty("domain", DefaultValue = "")] public string Domain { get { return (string)base[_propDomain]; } set { base[_propDomain] = value; } } protected override ConfigurationElementProperty ElementProperty { get { return s_elemProperty; } } private static void Validate(object value) { if (value == null) { throw new ArgumentNullException("forms"); } FormsAuthenticationConfiguration elem = (FormsAuthenticationConfiguration)value; if (StringUtil.StringStartsWith(elem.LoginUrl, "\\\\") || (elem.LoginUrl.Length > 1 && elem.LoginUrl[1] == ':')) { throw new ConfigurationErrorsException(SR.GetString(SR.Auth_bad_url), elem.ElementInformation.Properties["loginUrl"].Source, elem.ElementInformation.Properties["loginUrl"].LineNumber); } if (StringUtil.StringStartsWith(elem.DefaultUrl, "\\\\") || (elem.DefaultUrl.Length > 1 && elem.DefaultUrl[1] == ':')) { throw new ConfigurationErrorsException(SR.GetString(SR.Auth_bad_url), elem.ElementInformation.Properties["defaultUrl"].Source, elem.ElementInformation.Properties["defaultUrl"].LineNumber); } } } // class FormsAuthenticationConfiguration }
Link Menu
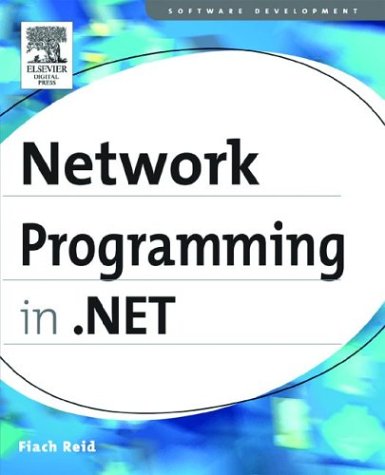
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlQueryContext.cs
- Substitution.cs
- XmlDocumentSerializer.cs
- WizardPanel.cs
- GrammarBuilderPhrase.cs
- UpdatePanelTrigger.cs
- XamlStackWriter.cs
- TextRenderer.cs
- PlainXmlDeserializer.cs
- DesignerActionPanel.cs
- ColorInterpolationModeValidation.cs
- ListSortDescriptionCollection.cs
- SynchronizedDispatch.cs
- CodeLabeledStatement.cs
- CodeTryCatchFinallyStatement.cs
- RelationshipType.cs
- Vector3dCollection.cs
- RenamedEventArgs.cs
- TimeoutException.cs
- FrameworkContentElement.cs
- infer.cs
- RenamedEventArgs.cs
- StateWorkerRequest.cs
- XmlSchemaGroup.cs
- BindingValueChangedEventArgs.cs
- InvalidPipelineStoreException.cs
- GenericArgumentsUpdater.cs
- HWStack.cs
- HttpPostedFileBase.cs
- BaseTemplateCodeDomTreeGenerator.cs
- Marshal.cs
- WindowsSolidBrush.cs
- VarRefManager.cs
- ListControlConvertEventArgs.cs
- SizeFConverter.cs
- SwitchElementsCollection.cs
- ToolStripDropTargetManager.cs
- SparseMemoryStream.cs
- SqlDataReader.cs
- BindingElement.cs
- MemberCollection.cs
- PartitionResolver.cs
- GridViewCancelEditEventArgs.cs
- PropertyDescriptorGridEntry.cs
- TypeExtension.cs
- SmtpNetworkElement.cs
- ToolStripComboBox.cs
- TemplateBindingExtensionConverter.cs
- StatusCommandUI.cs
- DoubleLinkListEnumerator.cs
- XsltArgumentList.cs
- NativeCppClassAttribute.cs
- CharEntityEncoderFallback.cs
- PackageRelationship.cs
- ParameterElement.cs
- CodeRemoveEventStatement.cs
- InfoCardUIAgent.cs
- AudioBase.cs
- SelectionRange.cs
- PrintController.cs
- ConfigurationStrings.cs
- DataSourceSerializationException.cs
- PlaceHolder.cs
- ScaleTransform.cs
- TextServicesCompartmentContext.cs
- Cell.cs
- SHA1Managed.cs
- SQLChars.cs
- XmlNamespaceMappingCollection.cs
- SiteMapDataSource.cs
- DrawingContextDrawingContextWalker.cs
- UInt64Converter.cs
- ReflectEventDescriptor.cs
- ConcurrentStack.cs
- TreeViewItemAutomationPeer.cs
- ColumnResult.cs
- EnumValAlphaComparer.cs
- BaseTemplateBuildProvider.cs
- PopOutPanel.cs
- SplashScreenNativeMethods.cs
- PeerNameRegistration.cs
- ContainerControl.cs
- CertificateElement.cs
- BuildResultCache.cs
- ButtonField.cs
- WebBodyFormatMessageProperty.cs
- BlockingCollection.cs
- TransformValueSerializer.cs
- SystemNetworkInterface.cs
- recordstate.cs
- PointF.cs
- X509CertificateChain.cs
- UntypedNullExpression.cs
- AvTrace.cs
- OleDbConnectionFactory.cs
- EventlogProvider.cs
- Rect3D.cs
- Util.cs
- SelectionRange.cs
- TraceInternal.cs