Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / ScrollPattern.cs / 1 / ScrollPattern.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for Scroll Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Represents UI elements that are expressing a value /// #if (INTERNAL_COMPILE) internal class ScrollPattern: BasePattern #else public class ScrollPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private ScrollPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Value used by SetSCrollPercent to indicate that no scrolling should take place in the specified direction public const double NoScroll = -1.0; ///Scroll pattern public static readonly AutomationPattern Pattern = ScrollPatternIdentifiers.Pattern; ///Property ID: HorizontalScrollPercent - Current horizontal scroll position public static readonly AutomationProperty HorizontalScrollPercentProperty = ScrollPatternIdentifiers.HorizontalScrollPercentProperty; ///Property ID: HorizontalViewSize - Minimum possible horizontal scroll position public static readonly AutomationProperty HorizontalViewSizeProperty = ScrollPatternIdentifiers.HorizontalViewSizeProperty; ///Property ID: VerticalScrollPercent - Current vertical scroll position public static readonly AutomationProperty VerticalScrollPercentProperty = ScrollPatternIdentifiers.VerticalScrollPercentProperty; ///Property ID: VerticalViewSize public static readonly AutomationProperty VerticalViewSizeProperty = ScrollPatternIdentifiers.VerticalViewSizeProperty; ///Property ID: HorizontallyScrollable public static readonly AutomationProperty HorizontallyScrollableProperty = ScrollPatternIdentifiers.HorizontallyScrollableProperty; ///Property ID: VerticallyScrollable public static readonly AutomationProperty VerticallyScrollableProperty = ScrollPatternIdentifiers.VerticallyScrollableProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ///Request to set the current horizontal and Vertical scroll position /// by percent (0-100). Passing in the value of "-1" will indicate that /// scrolling in that direction should be ignored. /// The ability to call this method and simultaneously scroll horizontally and /// vertically provides simple panning support. /// Amount to scroll by horizontally /// Amount to scroll by vertically /// ////// This API does not work inside the secure execution environment. /// public void SetScrollPercent( double horizontalPercent, double verticalPercent ) { UiaCoreApi.ScrollPattern_SetScrollPercent(_hPattern, horizontalPercent, verticalPercent); } ////// Request to scroll horizontally and vertically by the specified amount. /// The ability to call this method and simultaneously scroll horizontally /// and vertically provides simple panning support. If only horizontal or vertical percent /// needs to be changed the constant SetScrollPercentUnchanged can be used for /// either parameter and that axis wil be unchanged. /// /// amount to scroll by horizontally /// amount to scroll by vertically /// ////// This API does not work inside the secure execution environment. /// public void Scroll( ScrollAmount horizontalAmount, ScrollAmount verticalAmount ) { UiaCoreApi.ScrollPattern_Scroll(_hPattern, horizontalAmount, verticalAmount); } ////// /// Request to scroll horizontally by the specified amount /// /// Amount to scroll by /// ////// This API does not work inside the secure execution environment. /// public void ScrollHorizontal( ScrollAmount amount ) { UiaCoreApi.ScrollPattern_Scroll(_hPattern, amount, ScrollAmount.NoAmount); } ////// /// Request to scroll vertically by the specified amount /// /// Amount to scroll by /// ////// This API does not work inside the secure execution environment. /// public void ScrollVertical( ScrollAmount amount ) { UiaCoreApi.ScrollPattern_Scroll(_hPattern, ScrollAmount.NoAmount, amount); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// /// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public ScrollPatternInformation Cached { get { Misc.ValidateCached(_cached); return new ScrollPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public ScrollPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new ScrollPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods static internal object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new ScrollPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields SafePatternHandle _hPattern; bool _cached; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct ScrollPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal ScrollPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Get the current horizontal scroll position /// /// ////// This API does not work inside the secure execution environment. /// public double HorizontalScrollPercent { get { return (double)_el.GetPatternPropertyValue(HorizontalScrollPercentProperty, _useCache); } } ////// /// Get the current vertical scroll position /// /// ////// This API does not work inside the secure execution environment. /// public double VerticalScrollPercent { get { return (double)_el.GetPatternPropertyValue(VerticalScrollPercentProperty, _useCache); } } ////// /// Equal to the horizontal percentage of the entire control that is currently viewable. /// /// ////// This API does not work inside the secure execution environment. /// public double HorizontalViewSize { get { return (double)_el.GetPatternPropertyValue(HorizontalViewSizeProperty, _useCache); } } ////// /// Equal to the horizontal percentage of the entire control that is currently viewable. /// /// ////// This API does not work inside the secure execution environment. /// public double VerticalViewSize { get { return (double)_el.GetPatternPropertyValue(VerticalViewSizeProperty, _useCache); } } ////// /// True if control can scroll horizontally /// /// ////// This API does not work inside the secure execution environment. /// public bool HorizontallyScrollable { get { return (bool)_el.GetPatternPropertyValue(HorizontallyScrollableProperty, _useCache); } } ////// /// True if control can scroll vertically /// /// ////// This API does not work inside the secure execution environment. /// public bool VerticallyScrollable { get { return (bool)_el.GetPatternPropertyValue(VerticallyScrollableProperty, _useCache); } } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // ///// // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for Scroll Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Represents UI elements that are expressing a value /// #if (INTERNAL_COMPILE) internal class ScrollPattern: BasePattern #else public class ScrollPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private ScrollPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Value used by SetSCrollPercent to indicate that no scrolling should take place in the specified direction public const double NoScroll = -1.0; ///Scroll pattern public static readonly AutomationPattern Pattern = ScrollPatternIdentifiers.Pattern; ///Property ID: HorizontalScrollPercent - Current horizontal scroll position public static readonly AutomationProperty HorizontalScrollPercentProperty = ScrollPatternIdentifiers.HorizontalScrollPercentProperty; ///Property ID: HorizontalViewSize - Minimum possible horizontal scroll position public static readonly AutomationProperty HorizontalViewSizeProperty = ScrollPatternIdentifiers.HorizontalViewSizeProperty; ///Property ID: VerticalScrollPercent - Current vertical scroll position public static readonly AutomationProperty VerticalScrollPercentProperty = ScrollPatternIdentifiers.VerticalScrollPercentProperty; ///Property ID: VerticalViewSize public static readonly AutomationProperty VerticalViewSizeProperty = ScrollPatternIdentifiers.VerticalViewSizeProperty; ///Property ID: HorizontallyScrollable public static readonly AutomationProperty HorizontallyScrollableProperty = ScrollPatternIdentifiers.HorizontallyScrollableProperty; ///Property ID: VerticallyScrollable public static readonly AutomationProperty VerticallyScrollableProperty = ScrollPatternIdentifiers.VerticallyScrollableProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ///Request to set the current horizontal and Vertical scroll position /// by percent (0-100). Passing in the value of "-1" will indicate that /// scrolling in that direction should be ignored. /// The ability to call this method and simultaneously scroll horizontally and /// vertically provides simple panning support. /// Amount to scroll by horizontally /// Amount to scroll by vertically /// ////// This API does not work inside the secure execution environment. /// public void SetScrollPercent( double horizontalPercent, double verticalPercent ) { UiaCoreApi.ScrollPattern_SetScrollPercent(_hPattern, horizontalPercent, verticalPercent); } ////// Request to scroll horizontally and vertically by the specified amount. /// The ability to call this method and simultaneously scroll horizontally /// and vertically provides simple panning support. If only horizontal or vertical percent /// needs to be changed the constant SetScrollPercentUnchanged can be used for /// either parameter and that axis wil be unchanged. /// /// amount to scroll by horizontally /// amount to scroll by vertically /// ////// This API does not work inside the secure execution environment. /// public void Scroll( ScrollAmount horizontalAmount, ScrollAmount verticalAmount ) { UiaCoreApi.ScrollPattern_Scroll(_hPattern, horizontalAmount, verticalAmount); } ////// /// Request to scroll horizontally by the specified amount /// /// Amount to scroll by /// ////// This API does not work inside the secure execution environment. /// public void ScrollHorizontal( ScrollAmount amount ) { UiaCoreApi.ScrollPattern_Scroll(_hPattern, amount, ScrollAmount.NoAmount); } ////// /// Request to scroll vertically by the specified amount /// /// Amount to scroll by /// ////// This API does not work inside the secure execution environment. /// public void ScrollVertical( ScrollAmount amount ) { UiaCoreApi.ScrollPattern_Scroll(_hPattern, ScrollAmount.NoAmount, amount); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// /// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public ScrollPatternInformation Cached { get { Misc.ValidateCached(_cached); return new ScrollPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public ScrollPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new ScrollPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods static internal object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new ScrollPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields SafePatternHandle _hPattern; bool _cached; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct ScrollPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal ScrollPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Get the current horizontal scroll position /// /// ////// This API does not work inside the secure execution environment. /// public double HorizontalScrollPercent { get { return (double)_el.GetPatternPropertyValue(HorizontalScrollPercentProperty, _useCache); } } ////// /// Get the current vertical scroll position /// /// ////// This API does not work inside the secure execution environment. /// public double VerticalScrollPercent { get { return (double)_el.GetPatternPropertyValue(VerticalScrollPercentProperty, _useCache); } } ////// /// Equal to the horizontal percentage of the entire control that is currently viewable. /// /// ////// This API does not work inside the secure execution environment. /// public double HorizontalViewSize { get { return (double)_el.GetPatternPropertyValue(HorizontalViewSizeProperty, _useCache); } } ////// /// Equal to the horizontal percentage of the entire control that is currently viewable. /// /// ////// This API does not work inside the secure execution environment. /// public double VerticalViewSize { get { return (double)_el.GetPatternPropertyValue(VerticalViewSizeProperty, _useCache); } } ////// /// True if control can scroll horizontally /// /// ////// This API does not work inside the secure execution environment. /// public bool HorizontallyScrollable { get { return (bool)_el.GetPatternPropertyValue(HorizontallyScrollableProperty, _useCache); } } ////// /// True if control can scroll vertically /// /// ////// This API does not work inside the secure execution environment. /// public bool VerticallyScrollable { get { return (bool)_el.GetPatternPropertyValue(VerticallyScrollableProperty, _useCache); } } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
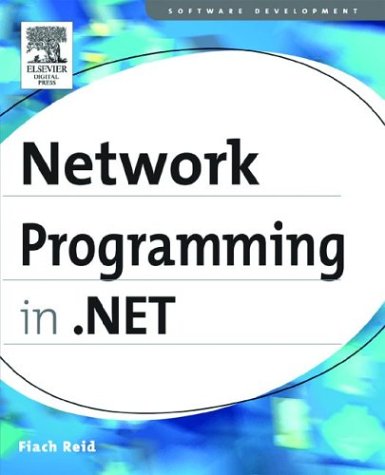
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TargetException.cs
- DataContractJsonSerializerOperationFormatter.cs
- MultiTrigger.cs
- HttpClientChannel.cs
- BindUriHelper.cs
- Utils.cs
- RequestUriProcessor.cs
- DrawItemEvent.cs
- SystemIPGlobalProperties.cs
- RootDesignerSerializerAttribute.cs
- DeflateInput.cs
- sqlstateclientmanager.cs
- BlobPersonalizationState.cs
- SelfSignedCertificate.cs
- _BasicClient.cs
- Stylesheet.cs
- Parameter.cs
- DependentList.cs
- CrossContextChannel.cs
- SQLRoleProvider.cs
- DataGridViewCell.cs
- CodeRemoveEventStatement.cs
- Span.cs
- HttpApplicationStateWrapper.cs
- FacetChecker.cs
- ObjectViewFactory.cs
- SystemWebCachingSectionGroup.cs
- WizardStepBase.cs
- ImageFormat.cs
- SQLMembershipProvider.cs
- AnimationStorage.cs
- HttpValueCollection.cs
- WindowsButton.cs
- InputReferenceExpression.cs
- MessageHeaderT.cs
- CodeCompileUnit.cs
- RelatedPropertyManager.cs
- ProfileManager.cs
- ViewBox.cs
- HttpRuntimeSection.cs
- FillRuleValidation.cs
- ToolStripDropTargetManager.cs
- SafeNativeMethods.cs
- RemoteArgument.cs
- DataMemberFieldEditor.cs
- Filter.cs
- StylusPointPropertyInfoDefaults.cs
- ListViewInsertEventArgs.cs
- basenumberconverter.cs
- ApplicationBuildProvider.cs
- PageStatePersister.cs
- EditorPart.cs
- SmiXetterAccessMap.cs
- followingquery.cs
- XmlElementAttribute.cs
- Model3D.cs
- HostVisual.cs
- StateChangeEvent.cs
- LoopExpression.cs
- ImageListImageEditor.cs
- DataSourceControl.cs
- AccessorTable.cs
- ColorConvertedBitmap.cs
- COM2ExtendedUITypeEditor.cs
- DataKeyCollection.cs
- EventsTab.cs
- EpmSyndicationContentDeSerializer.cs
- SystemWebCachingSectionGroup.cs
- RolePrincipal.cs
- ImportOptions.cs
- MultilineStringConverter.cs
- XmlDocumentType.cs
- TableLayoutSettingsTypeConverter.cs
- SingleAnimationBase.cs
- DetailsViewInsertedEventArgs.cs
- SettingsContext.cs
- AutomationIdentifier.cs
- BitmapData.cs
- FamilyMapCollection.cs
- ContainerFilterService.cs
- SqlStatistics.cs
- XamlFigureLengthSerializer.cs
- ArraySortHelper.cs
- ServiceModelStringsVersion1.cs
- DecimalAnimationUsingKeyFrames.cs
- PerfCounters.cs
- KeyboardNavigation.cs
- Model3DCollection.cs
- NonVisualControlAttribute.cs
- FrameworkElementAutomationPeer.cs
- BitmapCodecInfoInternal.cs
- QueryValue.cs
- SafeNativeMethodsCLR.cs
- CodeGeneratorOptions.cs
- PermissionSetEnumerator.cs
- SerializationHelper.cs
- ComponentCollection.cs
- TempEnvironment.cs
- handlecollector.cs
- Control.cs