Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Compiler / TypeSystem / EventInfo.cs / 1305376 / EventInfo.cs
namespace System.Workflow.ComponentModel.Compiler { using System; using System.CodeDom; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Globalization; using System.Reflection; #region DesignTimeEventInfo internal sealed class DesignTimeEventInfo: EventInfo { #region Members and Constructors private string name; private DesignTimeMethodInfo addMethod = null; private DesignTimeMethodInfo removeMethod = null; private Attribute[] attributes = null; private MemberAttributes memberAttributes; private DesignTimeType declaringType; private CodeMemberEvent codeDomEvent; internal DesignTimeEventInfo(DesignTimeType declaringType, CodeMemberEvent codeDomEvent) { if (declaringType == null) { throw new ArgumentNullException("Declaring Type"); } if (codeDomEvent == null) { throw new ArgumentNullException("codeDomEvent"); } this.declaringType = declaringType; this.codeDomEvent = codeDomEvent; this.name = Helper.EnsureTypeName(codeDomEvent.Name); this.memberAttributes = codeDomEvent.Attributes; this.addMethod = null; this.removeMethod = null; } #endregion #region Event Info overrides public override MethodInfo GetAddMethod(bool nonPublic) { if (this.addMethod == null) { Type handlerType = declaringType.ResolveType(DesignTimeType.GetTypeNameFromCodeTypeReference(this.codeDomEvent.Type, declaringType)); if (handlerType != null) { CodeMemberMethod codeAddMethod = new CodeMemberMethod(); codeAddMethod.Name = "add_" + this.name; codeAddMethod.ReturnType = new CodeTypeReference(typeof(void)); codeAddMethod.Parameters.Add(new CodeParameterDeclarationExpression(this.codeDomEvent.Type, "Handler")); codeAddMethod.Attributes = this.memberAttributes; this.addMethod = new DesignTimeMethodInfo(this.declaringType, codeAddMethod, true); } } return this.addMethod; } public override MethodInfo GetRemoveMethod(bool nonPublic) { if (this.removeMethod == null) { Type handlerType = declaringType.ResolveType(DesignTimeType.GetTypeNameFromCodeTypeReference(this.codeDomEvent.Type, declaringType)); if (handlerType != null) { CodeMemberMethod codeRemoveMethod = new CodeMemberMethod(); codeRemoveMethod.Name = "remove_" + this.name; codeRemoveMethod.ReturnType = new CodeTypeReference(typeof(void)); codeRemoveMethod.Parameters.Add(new CodeParameterDeclarationExpression(handlerType, "Handler")); codeRemoveMethod.Attributes = this.memberAttributes; this.removeMethod = new DesignTimeMethodInfo(declaringType, codeRemoveMethod, true); } } return this.removeMethod; } public override MethodInfo GetRaiseMethod(bool nonPublic) { return null; } public override EventAttributes Attributes { //We're not interested in this flag get { return default(EventAttributes); } } #endregion #region MemberInfo Overrides public override string Name { get { return this.name; } } public override Type DeclaringType { get { return this.declaringType; } } public override Type ReflectedType { get { return this.declaringType; } } public override object[] GetCustomAttributes(bool inherit) { return GetCustomAttributes(typeof(object), inherit); } public override object[] GetCustomAttributes(Type attributeType, bool inherit) { if (attributeType == null) throw new ArgumentNullException("attributeType"); if (this.attributes == null) this.attributes = Helper.LoadCustomAttributes(this.codeDomEvent.CustomAttributes, this.DeclaringType as DesignTimeType); return Helper.GetCustomAttributes(attributeType, inherit, this.attributes, this); } public override bool IsDefined(Type attributeType, bool inherit) { if (attributeType == null) throw new ArgumentNullException("attributeType"); if (this.attributes == null) this.attributes = Helper.LoadCustomAttributes(this.codeDomEvent.CustomAttributes, this.DeclaringType as DesignTimeType); if (Helper.IsDefined(attributeType, inherit, attributes, this)) return true; return false; } #endregion #region Helpers internal bool IsPublic { get { return ((memberAttributes & MemberAttributes.Public) != 0); } } internal bool IsStatic { get { return ((memberAttributes & MemberAttributes.Static) != 0); } } #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.ComponentModel.Compiler { using System; using System.CodeDom; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Globalization; using System.Reflection; #region DesignTimeEventInfo internal sealed class DesignTimeEventInfo: EventInfo { #region Members and Constructors private string name; private DesignTimeMethodInfo addMethod = null; private DesignTimeMethodInfo removeMethod = null; private Attribute[] attributes = null; private MemberAttributes memberAttributes; private DesignTimeType declaringType; private CodeMemberEvent codeDomEvent; internal DesignTimeEventInfo(DesignTimeType declaringType, CodeMemberEvent codeDomEvent) { if (declaringType == null) { throw new ArgumentNullException("Declaring Type"); } if (codeDomEvent == null) { throw new ArgumentNullException("codeDomEvent"); } this.declaringType = declaringType; this.codeDomEvent = codeDomEvent; this.name = Helper.EnsureTypeName(codeDomEvent.Name); this.memberAttributes = codeDomEvent.Attributes; this.addMethod = null; this.removeMethod = null; } #endregion #region Event Info overrides public override MethodInfo GetAddMethod(bool nonPublic) { if (this.addMethod == null) { Type handlerType = declaringType.ResolveType(DesignTimeType.GetTypeNameFromCodeTypeReference(this.codeDomEvent.Type, declaringType)); if (handlerType != null) { CodeMemberMethod codeAddMethod = new CodeMemberMethod(); codeAddMethod.Name = "add_" + this.name; codeAddMethod.ReturnType = new CodeTypeReference(typeof(void)); codeAddMethod.Parameters.Add(new CodeParameterDeclarationExpression(this.codeDomEvent.Type, "Handler")); codeAddMethod.Attributes = this.memberAttributes; this.addMethod = new DesignTimeMethodInfo(this.declaringType, codeAddMethod, true); } } return this.addMethod; } public override MethodInfo GetRemoveMethod(bool nonPublic) { if (this.removeMethod == null) { Type handlerType = declaringType.ResolveType(DesignTimeType.GetTypeNameFromCodeTypeReference(this.codeDomEvent.Type, declaringType)); if (handlerType != null) { CodeMemberMethod codeRemoveMethod = new CodeMemberMethod(); codeRemoveMethod.Name = "remove_" + this.name; codeRemoveMethod.ReturnType = new CodeTypeReference(typeof(void)); codeRemoveMethod.Parameters.Add(new CodeParameterDeclarationExpression(handlerType, "Handler")); codeRemoveMethod.Attributes = this.memberAttributes; this.removeMethod = new DesignTimeMethodInfo(declaringType, codeRemoveMethod, true); } } return this.removeMethod; } public override MethodInfo GetRaiseMethod(bool nonPublic) { return null; } public override EventAttributes Attributes { //We're not interested in this flag get { return default(EventAttributes); } } #endregion #region MemberInfo Overrides public override string Name { get { return this.name; } } public override Type DeclaringType { get { return this.declaringType; } } public override Type ReflectedType { get { return this.declaringType; } } public override object[] GetCustomAttributes(bool inherit) { return GetCustomAttributes(typeof(object), inherit); } public override object[] GetCustomAttributes(Type attributeType, bool inherit) { if (attributeType == null) throw new ArgumentNullException("attributeType"); if (this.attributes == null) this.attributes = Helper.LoadCustomAttributes(this.codeDomEvent.CustomAttributes, this.DeclaringType as DesignTimeType); return Helper.GetCustomAttributes(attributeType, inherit, this.attributes, this); } public override bool IsDefined(Type attributeType, bool inherit) { if (attributeType == null) throw new ArgumentNullException("attributeType"); if (this.attributes == null) this.attributes = Helper.LoadCustomAttributes(this.codeDomEvent.CustomAttributes, this.DeclaringType as DesignTimeType); if (Helper.IsDefined(attributeType, inherit, attributes, this)) return true; return false; } #endregion #region Helpers internal bool IsPublic { get { return ((memberAttributes & MemberAttributes.Public) != 0); } } internal bool IsStatic { get { return ((memberAttributes & MemberAttributes.Static) != 0); } } #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
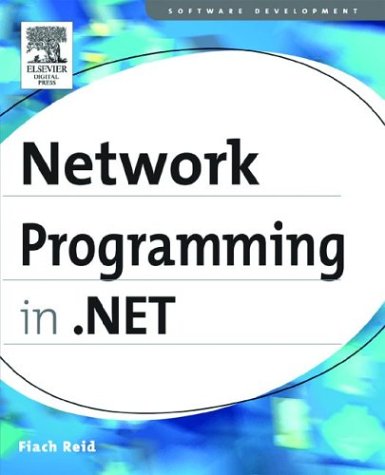
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThreadStaticAttribute.cs
- HelpEvent.cs
- CodeDelegateInvokeExpression.cs
- HostedHttpRequestAsyncResult.cs
- SecUtil.cs
- ADConnectionHelper.cs
- ListDictionary.cs
- RotateTransform3D.cs
- MemberInfoSerializationHolder.cs
- NativeMethodsOther.cs
- HatchBrush.cs
- SqlParameterizer.cs
- MDIClient.cs
- HttpRequestMessageProperty.cs
- BulletedListDesigner.cs
- ColorInterpolationModeValidation.cs
- SynchronizationLockException.cs
- PeerCustomResolverBindingElement.cs
- StateWorkerRequest.cs
- SqlDataSourceStatusEventArgs.cs
- ZoomPercentageConverter.cs
- ExpressionCopier.cs
- PageThemeBuildProvider.cs
- QueryableDataSourceHelper.cs
- ChangeTracker.cs
- TreeNodeStyleCollection.cs
- OleDbSchemaGuid.cs
- PersonalizationState.cs
- IApplicationTrustManager.cs
- StaticExtensionConverter.cs
- SequentialUshortCollection.cs
- ShapeTypeface.cs
- ProfileManager.cs
- ListBoxItem.cs
- AccessorTable.cs
- BackStopAuthenticationModule.cs
- DataGridClipboardCellContent.cs
- ActiveXHost.cs
- PeerName.cs
- MetadataCollection.cs
- SamlAssertionKeyIdentifierClause.cs
- WindowsRebar.cs
- LongAverageAggregationOperator.cs
- SmtpTransport.cs
- SplashScreen.cs
- ACL.cs
- FileChangesMonitor.cs
- FontWeight.cs
- altserialization.cs
- WebPartEditVerb.cs
- HtmlControlPersistable.cs
- MetaChildrenColumn.cs
- MessageSecurityOverTcp.cs
- NumericUpDown.cs
- BamlRecordReader.cs
- CodeMemberField.cs
- ASCIIEncoding.cs
- TableRow.cs
- WizardStepBase.cs
- Privilege.cs
- DictionaryTraceRecord.cs
- XmlNamespaceManager.cs
- InputLangChangeEvent.cs
- TriggerBase.cs
- ObjectViewEntityCollectionData.cs
- HtmlToClrEventProxy.cs
- WindowsGrip.cs
- AssociationTypeEmitter.cs
- MarkupCompilePass1.cs
- ThrowOnMultipleAssignment.cs
- Application.cs
- HttpTransportElement.cs
- ReachFixedPageSerializerAsync.cs
- RectValueSerializer.cs
- LookupNode.cs
- QilXmlReader.cs
- Slider.cs
- TraceContextRecord.cs
- SchemaObjectWriter.cs
- InstanceHandleReference.cs
- ProofTokenCryptoHandle.cs
- ToolBarTray.cs
- JsonServiceDocumentSerializer.cs
- TreeNodeClickEventArgs.cs
- AmbiguousMatchException.cs
- TextHidden.cs
- AudioException.cs
- DispatcherEventArgs.cs
- PinnedBufferMemoryStream.cs
- LoadedOrUnloadedOperation.cs
- WebServiceFaultDesigner.cs
- CrossAppDomainChannel.cs
- SafeCryptoHandles.cs
- ResourceContainer.cs
- SystemException.cs
- NumericUpDown.cs
- WebConfigurationFileMap.cs
- BackgroundWorker.cs
- TextTrailingCharacterEllipsis.cs
- listitem.cs