Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / DataTrigger.cs / 1 / DataTrigger.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines DataTrigger object, akin to Trigger except it // gets values from data. // //--------------------------------------------------------------------------- using System; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Windows.Data; using System.Windows.Markup; namespace System.Windows { ////// A single Style data conditional dependency driver /// [ContentProperty("Setters")] public class DataTrigger : TriggerBase, IAddChild { ////// Binding declaration of the conditional /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // Not localizable by-default public BindingBase Binding { get { // Verify Context Access VerifyAccess(); return _binding; } set { // Verify Context Access VerifyAccess(); if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "DataTrigger")); } _binding = value; } } ////// Value of the condition (equality check) /// [DependsOn("Binding")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // Not localizable by-default public object Value { get { // Verify Context Access VerifyAccess(); return _value; } set { // Verify Context Access VerifyAccess(); if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "DataTrigger")); } if (value is MarkupExtension) { throw new ArgumentException(SR.Get(SRID.ConditionValueOfMarkupExtensionNotSupported, value.GetType().Name)); } if( value is Expression ) { throw new ArgumentException(SR.Get(SRID.ConditionValueOfExpressionNotSupported)); } _value = value; } } ////// Collection of Setter objects, which describes what to apply /// when this trigger is active. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public SetterBaseCollection Setters { get { // Verify Context Access VerifyAccess(); if( _setters == null ) { _setters = new SetterBaseCollection(); } return _setters; } } ////// This method is called to Add a Setter object as a child of the Style. /// /// /// The object to add as a child; it must be a Setter or subclass. /// void IAddChild.AddChild (Object value) { // Verify Context Access VerifyAccess(); Setters.Add(Trigger.CheckChildIsSetter(value)); } ////// This method is called by the parser when text appears under the tag in markup. /// As default Styles do not support text, calling this method has no effect. /// /// /// Text to add as a child. /// void IAddChild.AddText (string text) { // Verify Context Access VerifyAccess(); XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } internal sealed override void Seal() { if (IsSealed) { return; } // Process the _setters collection: Copy values into PropertyValueList and seal the Setter objects. ProcessSettersCollection(_setters); // Freeze the value for the trigger StyleHelper.SealIfSealable(_value); // Build conditions array from collection TriggerConditions = new TriggerCondition[] { new TriggerCondition( _binding, LogicalOp.Equals, _value) }; // Set Condition for all data triggers for (int i = 0; i < PropertyValues.Count; i++) { PropertyValue propertyValue = PropertyValues[i]; propertyValue.Conditions = TriggerConditions; switch (propertyValue.ValueType) { case PropertyValueType.Trigger: propertyValue.ValueType = PropertyValueType.DataTrigger; break; case PropertyValueType.PropertyTriggerResource: propertyValue.ValueType = PropertyValueType.DataTriggerResource; break; default: throw new InvalidOperationException(SR.Get(SRID.UnexpectedValueTypeForDataTrigger, propertyValue.ValueType)); } // Put back modified struct PropertyValues[i] = propertyValue; } base.Seal(); } // evaluate the current state of the trigger internal override bool GetCurrentState(DependencyObject container, UncommonFielddataField) { Debug.Assert( TriggerConditions != null && TriggerConditions.Length == 1, "This method assumes there is exactly one TriggerCondition." ); return TriggerConditions[0].ConvertAndMatch(StyleHelper.GetDataTriggerValue(dataField, container, TriggerConditions[0].Binding)); } private BindingBase _binding; private object _value = DependencyProperty.UnsetValue; private SetterBaseCollection _setters = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines DataTrigger object, akin to Trigger except it // gets values from data. // //--------------------------------------------------------------------------- using System; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Windows.Data; using System.Windows.Markup; namespace System.Windows { ////// A single Style data conditional dependency driver /// [ContentProperty("Setters")] public class DataTrigger : TriggerBase, IAddChild { ////// Binding declaration of the conditional /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // Not localizable by-default public BindingBase Binding { get { // Verify Context Access VerifyAccess(); return _binding; } set { // Verify Context Access VerifyAccess(); if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "DataTrigger")); } _binding = value; } } ////// Value of the condition (equality check) /// [DependsOn("Binding")] [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // Not localizable by-default public object Value { get { // Verify Context Access VerifyAccess(); return _value; } set { // Verify Context Access VerifyAccess(); if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "DataTrigger")); } if (value is MarkupExtension) { throw new ArgumentException(SR.Get(SRID.ConditionValueOfMarkupExtensionNotSupported, value.GetType().Name)); } if( value is Expression ) { throw new ArgumentException(SR.Get(SRID.ConditionValueOfExpressionNotSupported)); } _value = value; } } ////// Collection of Setter objects, which describes what to apply /// when this trigger is active. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public SetterBaseCollection Setters { get { // Verify Context Access VerifyAccess(); if( _setters == null ) { _setters = new SetterBaseCollection(); } return _setters; } } ////// This method is called to Add a Setter object as a child of the Style. /// /// /// The object to add as a child; it must be a Setter or subclass. /// void IAddChild.AddChild (Object value) { // Verify Context Access VerifyAccess(); Setters.Add(Trigger.CheckChildIsSetter(value)); } ////// This method is called by the parser when text appears under the tag in markup. /// As default Styles do not support text, calling this method has no effect. /// /// /// Text to add as a child. /// void IAddChild.AddText (string text) { // Verify Context Access VerifyAccess(); XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } internal sealed override void Seal() { if (IsSealed) { return; } // Process the _setters collection: Copy values into PropertyValueList and seal the Setter objects. ProcessSettersCollection(_setters); // Freeze the value for the trigger StyleHelper.SealIfSealable(_value); // Build conditions array from collection TriggerConditions = new TriggerCondition[] { new TriggerCondition( _binding, LogicalOp.Equals, _value) }; // Set Condition for all data triggers for (int i = 0; i < PropertyValues.Count; i++) { PropertyValue propertyValue = PropertyValues[i]; propertyValue.Conditions = TriggerConditions; switch (propertyValue.ValueType) { case PropertyValueType.Trigger: propertyValue.ValueType = PropertyValueType.DataTrigger; break; case PropertyValueType.PropertyTriggerResource: propertyValue.ValueType = PropertyValueType.DataTriggerResource; break; default: throw new InvalidOperationException(SR.Get(SRID.UnexpectedValueTypeForDataTrigger, propertyValue.ValueType)); } // Put back modified struct PropertyValues[i] = propertyValue; } base.Seal(); } // evaluate the current state of the trigger internal override bool GetCurrentState(DependencyObject container, UncommonFielddataField) { Debug.Assert( TriggerConditions != null && TriggerConditions.Length == 1, "This method assumes there is exactly one TriggerCondition." ); return TriggerConditions[0].ConvertAndMatch(StyleHelper.GetDataTriggerValue(dataField, container, TriggerConditions[0].Binding)); } private BindingBase _binding; private object _value = DependencyProperty.UnsetValue; private SetterBaseCollection _setters = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
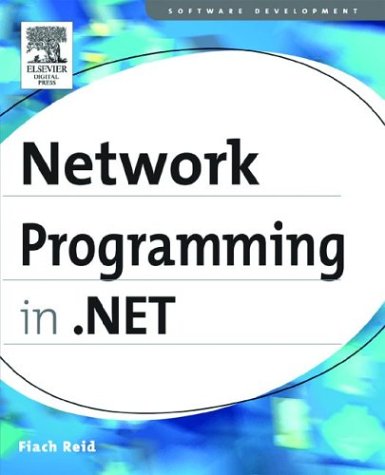
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EncoderBestFitFallback.cs
- Converter.cs
- SHA512Managed.cs
- COM2ExtendedBrowsingHandler.cs
- Geometry3D.cs
- EntityContainerEntitySetDefiningQuery.cs
- MarkupProperty.cs
- FilteredDataSetHelper.cs
- HttpModuleAction.cs
- WpfWebRequestHelper.cs
- UntypedNullExpression.cs
- DefaultAuthorizationContext.cs
- GridViewColumnHeaderAutomationPeer.cs
- SoapExtensionTypeElement.cs
- UserInitiatedRoutedEventPermission.cs
- AppDomainUnloadedException.cs
- DataFieldConverter.cs
- Button.cs
- SymmetricAlgorithm.cs
- BufferedGraphicsManager.cs
- NativeCppClassAttribute.cs
- HttpRuntimeSection.cs
- WorkflowInstanceSuspendedRecord.cs
- WebPartEditorApplyVerb.cs
- EventsTab.cs
- DbgCompiler.cs
- SharedConnectionInfo.cs
- VirtualPath.cs
- WmpBitmapDecoder.cs
- ReadOnlyHierarchicalDataSource.cs
- PartitionedDataSource.cs
- Table.cs
- ReaderWriterLockWrapper.cs
- SqlInternalConnectionSmi.cs
- WinFormsSpinner.cs
- XmlILCommand.cs
- EntitySet.cs
- AnonymousIdentificationModule.cs
- DesignerProperties.cs
- XmlCharType.cs
- DirectoryObjectSecurity.cs
- Msec.cs
- CellConstant.cs
- SubclassTypeValidator.cs
- Misc.cs
- DependencyProperty.cs
- HtmlEmptyTagControlBuilder.cs
- CodeSubDirectory.cs
- SizeFConverter.cs
- WorkflowApplicationAbortedEventArgs.cs
- DesignerCommandAdapter.cs
- ReachPageContentCollectionSerializerAsync.cs
- Typography.cs
- PageCatalogPart.cs
- CreateParams.cs
- HttpModuleCollection.cs
- SetterBaseCollection.cs
- ObjectStateEntry.cs
- ResourcesBuildProvider.cs
- HtmlInputCheckBox.cs
- WebPartConnectionsDisconnectVerb.cs
- ReadOnlyHierarchicalDataSourceView.cs
- VariableValue.cs
- PathSegment.cs
- HttpCachePolicy.cs
- TreeViewEvent.cs
- httpserverutility.cs
- NativeWrapper.cs
- DocumentViewerConstants.cs
- SymLanguageVendor.cs
- HttpListenerRequestUriBuilder.cs
- ListView.cs
- EmptyStringExpandableObjectConverter.cs
- StandardCommandToolStripMenuItem.cs
- ListBoxItemAutomationPeer.cs
- EventItfInfo.cs
- LicenseProviderAttribute.cs
- Debug.cs
- TokenizerHelper.cs
- XmlSchemaIdentityConstraint.cs
- PageStatePersister.cs
- XmlAnyElementAttributes.cs
- Parameter.cs
- DataListItemEventArgs.cs
- TypefaceMetricsCache.cs
- DataTablePropertyDescriptor.cs
- WebPartVerbsEventArgs.cs
- ApplicationInterop.cs
- ChtmlCalendarAdapter.cs
- CFStream.cs
- Error.cs
- ValueType.cs
- BamlTreeNode.cs
- XmlHierarchicalEnumerable.cs
- GenericNameHandler.cs
- InitiatorSessionSymmetricTransportSecurityProtocol.cs
- SystemGatewayIPAddressInformation.cs
- ListViewPagedDataSource.cs
- ExtentKey.cs
- Int16AnimationBase.cs