Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / Design / EventsTab.cs / 1 / EventsTab.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.ComponentModel.Design; using System.Windows.Forms; using System.Windows.Forms.Design; using System.Collections; using Microsoft.Win32; ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class EventsTab : PropertyTab { private IServiceProvider sp; private IDesignerHost currentHost; private bool sunkEvent; ///Provides a tab on the property browser to display events for selection and linking. ////// /// public EventsTab(IServiceProvider sp){ this.sp = sp; } ///Initializes a new instance of the ///class. /// /// public override string TabName { get { return SR.GetString(SR.PBRSToolTipEvents); } } ///Gets or sets the name of the tab. ////// /// public override string HelpKeyword { get { return "Events"; // do not localize. } } // override this to reject components you don't want to support. ///Gets or sets the help keyword for the tab. ////// /// public override bool CanExtend(Object extendee) { return !Marshal.IsComObject(extendee); } private void OnActiveDesignerChanged(object sender, ActiveDesignerEventArgs adevent){ currentHost = adevent.NewDesigner; } ///Gets a value indicating whether the specified object can be extended. ////// /// public override PropertyDescriptor GetDefaultProperty(object obj) { IEventBindingService eventPropertySvc = GetEventPropertyService(obj, null); if (eventPropertySvc == null) { return null; } // Find the default event. Note that we rely on GetEventProperties caching // the property to event match, so we can == on the default event. // We assert that this always works. // EventDescriptor defEvent = TypeDescriptor.GetDefaultEvent(obj); if (defEvent != null) { return eventPropertySvc.GetEventProperty(defEvent); } return null; } private IEventBindingService GetEventPropertyService(object obj, ITypeDescriptorContext context) { IEventBindingService eventPropertySvc = null; if (!sunkEvent){ IDesignerEventService des = (IDesignerEventService)sp.GetService(typeof(IDesignerEventService)); if (des != null){ des.ActiveDesignerChanged += new ActiveDesignerEventHandler(this.OnActiveDesignerChanged); } sunkEvent = true; } if (eventPropertySvc == null && currentHost != null) { eventPropertySvc = (IEventBindingService)currentHost.GetService(typeof(IEventBindingService)); } if (eventPropertySvc == null && obj is IComponent){ ISite site = ((IComponent)obj).Site; if (site != null) { eventPropertySvc = (IEventBindingService)site.GetService(typeof(IEventBindingService)); } } if (eventPropertySvc == null && context != null) { eventPropertySvc = (IEventBindingService)context.GetService(typeof(IEventBindingService)); } return eventPropertySvc; } ///Gets the default property from the specified object. ////// /// public override PropertyDescriptorCollection GetProperties(object component, Attribute[] attributes) { return GetProperties(null, component, attributes); } ///Gets all the properties of the tab. ////// /// public override PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object component, Attribute[] attributes) { //Debug.Assert(component != null, "Can't get properties for a null item!"); IEventBindingService eventPropertySvc = GetEventPropertyService(component, context); if (eventPropertySvc == null) { return new PropertyDescriptorCollection(null); } EventDescriptorCollection events = TypeDescriptor.GetEvents(component, attributes); PropertyDescriptorCollection realEvents = eventPropertySvc.GetEventProperties(events); // Add DesignerSerializationVisibilityAttribute.Content to attributes to see if we have any. Attribute[] attributesPlusNamespace = new Attribute[attributes.Length + 1]; Array.Copy(attributes, 0, attributesPlusNamespace, 0, attributes.Length); attributesPlusNamespace[attributes.Length] = DesignerSerializationVisibilityAttribute.Content; // If we do, then we traverse them to see if they have any events under the current attributes, // and if so, we'll show them as top-level properties so they can be drilled down into to get events. PropertyDescriptorCollection namespaceProperties = TypeDescriptor.GetProperties(component, attributesPlusNamespace); if (namespaceProperties.Count > 0) { ArrayList list = null; for (int i = 0; i < namespaceProperties.Count; i++) { PropertyDescriptor nsProp = namespaceProperties[i]; TypeConverter tc = nsProp.Converter; if (!tc.GetPropertiesSupported()) { continue; } Object namespaceValue = nsProp.GetValue(component); EventDescriptorCollection namespaceEvents = TypeDescriptor.GetEvents(namespaceValue, attributes); if (namespaceEvents.Count > 0) { if (list == null) { list = new ArrayList(); } // make this non-mergable // nsProp = TypeDescriptor.CreateProperty(nsProp.ComponentType, nsProp, MergablePropertyAttribute.No); list.Add(nsProp); } } // we've found some, so add them to the event list. if (list != null) { PropertyDescriptor[] realNamespaceProperties = new PropertyDescriptor[list.Count]; list.CopyTo(realNamespaceProperties, 0); PropertyDescriptor[] finalEvents = new PropertyDescriptor[realEvents.Count + realNamespaceProperties.Length]; realEvents.CopyTo(finalEvents, 0); Array.Copy(realNamespaceProperties, 0, finalEvents, realEvents.Count, realNamespaceProperties.Length); realEvents = new PropertyDescriptorCollection(finalEvents); } } return realEvents; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Gets the properties of the specified component... ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.ComponentModel.Design; using System.Windows.Forms; using System.Windows.Forms.Design; using System.Collections; using Microsoft.Win32; ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class EventsTab : PropertyTab { private IServiceProvider sp; private IDesignerHost currentHost; private bool sunkEvent; ///Provides a tab on the property browser to display events for selection and linking. ////// /// public EventsTab(IServiceProvider sp){ this.sp = sp; } ///Initializes a new instance of the ///class. /// /// public override string TabName { get { return SR.GetString(SR.PBRSToolTipEvents); } } ///Gets or sets the name of the tab. ////// /// public override string HelpKeyword { get { return "Events"; // do not localize. } } // override this to reject components you don't want to support. ///Gets or sets the help keyword for the tab. ////// /// public override bool CanExtend(Object extendee) { return !Marshal.IsComObject(extendee); } private void OnActiveDesignerChanged(object sender, ActiveDesignerEventArgs adevent){ currentHost = adevent.NewDesigner; } ///Gets a value indicating whether the specified object can be extended. ////// /// public override PropertyDescriptor GetDefaultProperty(object obj) { IEventBindingService eventPropertySvc = GetEventPropertyService(obj, null); if (eventPropertySvc == null) { return null; } // Find the default event. Note that we rely on GetEventProperties caching // the property to event match, so we can == on the default event. // We assert that this always works. // EventDescriptor defEvent = TypeDescriptor.GetDefaultEvent(obj); if (defEvent != null) { return eventPropertySvc.GetEventProperty(defEvent); } return null; } private IEventBindingService GetEventPropertyService(object obj, ITypeDescriptorContext context) { IEventBindingService eventPropertySvc = null; if (!sunkEvent){ IDesignerEventService des = (IDesignerEventService)sp.GetService(typeof(IDesignerEventService)); if (des != null){ des.ActiveDesignerChanged += new ActiveDesignerEventHandler(this.OnActiveDesignerChanged); } sunkEvent = true; } if (eventPropertySvc == null && currentHost != null) { eventPropertySvc = (IEventBindingService)currentHost.GetService(typeof(IEventBindingService)); } if (eventPropertySvc == null && obj is IComponent){ ISite site = ((IComponent)obj).Site; if (site != null) { eventPropertySvc = (IEventBindingService)site.GetService(typeof(IEventBindingService)); } } if (eventPropertySvc == null && context != null) { eventPropertySvc = (IEventBindingService)context.GetService(typeof(IEventBindingService)); } return eventPropertySvc; } ///Gets the default property from the specified object. ////// /// public override PropertyDescriptorCollection GetProperties(object component, Attribute[] attributes) { return GetProperties(null, component, attributes); } ///Gets all the properties of the tab. ////// /// public override PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object component, Attribute[] attributes) { //Debug.Assert(component != null, "Can't get properties for a null item!"); IEventBindingService eventPropertySvc = GetEventPropertyService(component, context); if (eventPropertySvc == null) { return new PropertyDescriptorCollection(null); } EventDescriptorCollection events = TypeDescriptor.GetEvents(component, attributes); PropertyDescriptorCollection realEvents = eventPropertySvc.GetEventProperties(events); // Add DesignerSerializationVisibilityAttribute.Content to attributes to see if we have any. Attribute[] attributesPlusNamespace = new Attribute[attributes.Length + 1]; Array.Copy(attributes, 0, attributesPlusNamespace, 0, attributes.Length); attributesPlusNamespace[attributes.Length] = DesignerSerializationVisibilityAttribute.Content; // If we do, then we traverse them to see if they have any events under the current attributes, // and if so, we'll show them as top-level properties so they can be drilled down into to get events. PropertyDescriptorCollection namespaceProperties = TypeDescriptor.GetProperties(component, attributesPlusNamespace); if (namespaceProperties.Count > 0) { ArrayList list = null; for (int i = 0; i < namespaceProperties.Count; i++) { PropertyDescriptor nsProp = namespaceProperties[i]; TypeConverter tc = nsProp.Converter; if (!tc.GetPropertiesSupported()) { continue; } Object namespaceValue = nsProp.GetValue(component); EventDescriptorCollection namespaceEvents = TypeDescriptor.GetEvents(namespaceValue, attributes); if (namespaceEvents.Count > 0) { if (list == null) { list = new ArrayList(); } // make this non-mergable // nsProp = TypeDescriptor.CreateProperty(nsProp.ComponentType, nsProp, MergablePropertyAttribute.No); list.Add(nsProp); } } // we've found some, so add them to the event list. if (list != null) { PropertyDescriptor[] realNamespaceProperties = new PropertyDescriptor[list.Count]; list.CopyTo(realNamespaceProperties, 0); PropertyDescriptor[] finalEvents = new PropertyDescriptor[realEvents.Count + realNamespaceProperties.Length]; realEvents.CopyTo(finalEvents, 0); Array.Copy(realNamespaceProperties, 0, finalEvents, realEvents.Count, realNamespaceProperties.Length); realEvents = new PropertyDescriptorCollection(finalEvents); } } return realEvents; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Gets the properties of the specified component... ///
Link Menu
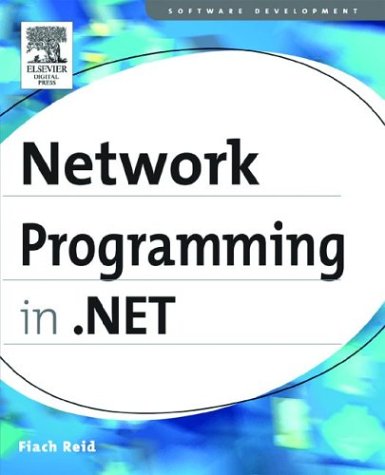
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WeakReferenceEnumerator.cs
- ScanQueryOperator.cs
- SqlBuilder.cs
- StopRoutingHandler.cs
- OleDbCommandBuilder.cs
- InputProviderSite.cs
- PreviewControlDesigner.cs
- CurrencyWrapper.cs
- DetailsViewRow.cs
- COMException.cs
- BinaryObjectWriter.cs
- XamlContextStack.cs
- SelectionChangedEventArgs.cs
- FormatPage.cs
- SyntaxCheck.cs
- UnsafeNativeMethodsCLR.cs
- XamlSerializationHelper.cs
- InlineUIContainer.cs
- Sql8ConformanceChecker.cs
- GPRECT.cs
- XmlReader.cs
- GenericAuthenticationEventArgs.cs
- DataGridAutoFormat.cs
- MailHeaderInfo.cs
- QualifiedId.cs
- UnsafeNativeMethods.cs
- Application.cs
- InvokePatternIdentifiers.cs
- EventLogSession.cs
- tibetanshape.cs
- MarkupProperty.cs
- CallId.cs
- Condition.cs
- Bits.cs
- WebPartHeaderCloseVerb.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- StrongName.cs
- HtmlElementCollection.cs
- SqlTriggerContext.cs
- Token.cs
- CallId.cs
- DefaultIfEmptyQueryOperator.cs
- SecurityTokenValidationException.cs
- RijndaelManagedTransform.cs
- TimeoutException.cs
- PostBackTrigger.cs
- LineInfo.cs
- MdiWindowListStrip.cs
- AccessControlList.cs
- Compensate.cs
- CodeTypeReferenceCollection.cs
- DataRowView.cs
- CompositeControl.cs
- GeometryHitTestParameters.cs
- BigInt.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- EventLogInternal.cs
- CompositeControl.cs
- DataGridViewCellStyleConverter.cs
- SqlUtils.cs
- ActiveXHost.cs
- Group.cs
- TemplateControlCodeDomTreeGenerator.cs
- ContextQuery.cs
- DateTimeValueSerializerContext.cs
- DecoderFallback.cs
- Size3DConverter.cs
- AudioFileOut.cs
- DataPointer.cs
- MetaModel.cs
- CompilationSection.cs
- PersonalizationDictionary.cs
- GroupBoxDesigner.cs
- BulletedList.cs
- QueryStringParameter.cs
- initElementDictionary.cs
- EndPoint.cs
- MetadataExchangeClient.cs
- LocalizationParserHooks.cs
- XPathItem.cs
- WebUtil.cs
- ColumnPropertiesGroup.cs
- GAC.cs
- TextBox.cs
- ClientConfigurationHost.cs
- DataTableMapping.cs
- HttpGetProtocolReflector.cs
- SmtpFailedRecipientsException.cs
- GridViewRowPresenter.cs
- IndexedGlyphRun.cs
- BulletDecorator.cs
- Queue.cs
- BitmapEffectCollection.cs
- ErrorWebPart.cs
- TemplateBindingExtension.cs
- HttpContextWrapper.cs
- GeneralTransform2DTo3DTo2D.cs
- SelectionGlyph.cs
- ViewStateModeByIdAttribute.cs
- TreeNodeStyle.cs