Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / TemplateGroupCollection.cs / 1 / TemplateGroupCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Collections; using System.Design; using System.Globalization; ////// /// Provides the necessary functionality for a template editing verb collection /// public sealed class TemplateGroupCollection : IList { private ArrayList _list; ///public TemplateGroupCollection() { } internal TemplateGroupCollection(TemplateGroup[] verbs) { for (int i = 0; i < verbs.Length; i++) { Add(verbs[i]); } } /// public int Count { get { return InternalList.Count; } } private ArrayList InternalList { get { if (_list == null) { _list = new ArrayList(); } return _list; } } /// public TemplateGroup this[int index] { get { return (TemplateGroup)InternalList[index]; } set { InternalList[index] = value; } } /// public int Add(TemplateGroup group) { return InternalList.Add(group); } public void AddRange(TemplateGroupCollection groups) { InternalList.AddRange(groups); } /// public void Clear() { InternalList.Clear(); } /// public bool Contains(TemplateGroup group) { return InternalList.Contains(group); } /// public void CopyTo(TemplateGroup[] array, int index) { InternalList.CopyTo(array, index); } /// public int IndexOf(TemplateGroup group) { return InternalList.IndexOf(group); } /// public void Insert(int index, TemplateGroup group) { InternalList.Insert(index, group); } /// public void Remove(TemplateGroup group) { InternalList.Remove(group); } /// public void RemoveAt(int index) { InternalList.RemoveAt(index); } #region IList implementation /// /// int ICollection.Count { get { return Count; } } /// /// bool IList.IsFixedSize { get { return InternalList.IsFixedSize; } } /// /// bool IList.IsReadOnly { get { return InternalList.IsReadOnly; } } /// /// bool ICollection.IsSynchronized { get { return InternalList.IsSynchronized; } } /// /// object ICollection.SyncRoot { get { return InternalList.SyncRoot; } } /// /// object IList.this[int index] { get { return this[index]; } set { if (!(value is TemplateGroup)) { throw new ArgumentException(String.Format(CultureInfo.InvariantCulture, SR.GetString(SR.WrongType), "TemplateGroup"), "value"); } this[index] = (TemplateGroup)value; } } /// /// int IList.Add(object o) { if (!(o is TemplateGroup)) { throw new ArgumentException(String.Format(CultureInfo.InvariantCulture, SR.GetString(SR.WrongType), "TemplateGroup"), "o"); } return Add((TemplateGroup)o); } /// /// void IList.Clear() { Clear(); } /// /// bool IList.Contains(object o) { if (!(o is TemplateGroup)) { throw new ArgumentException(String.Format(CultureInfo.InvariantCulture, SR.GetString(SR.WrongType), "TemplateGroup"), "o"); } return Contains((TemplateGroup)o); } /// /// void ICollection.CopyTo(Array array, int index) { InternalList.CopyTo(array, index); } /// /// IEnumerator IEnumerable.GetEnumerator() { return InternalList.GetEnumerator(); } /// /// int IList.IndexOf(object o) { if (!(o is TemplateGroup)) { throw new ArgumentException(String.Format(CultureInfo.InvariantCulture, SR.GetString(SR.WrongType), "TemplateGroup"), "o"); } return IndexOf((TemplateGroup)o); } /// /// void IList.Insert(int index, object o) { if (!(o is TemplateGroup)) { throw new ArgumentException(String.Format(CultureInfo.InvariantCulture, SR.GetString(SR.WrongType), "TemplateGroup"), "o"); } Insert(index, (TemplateGroup)o); } /// /// void IList.Remove(object o) { if (!(o is TemplateGroup)) { throw new ArgumentException(String.Format(CultureInfo.InvariantCulture, SR.GetString(SR.WrongType), "TemplateGroup"), "o"); } Remove((TemplateGroup)o); } /// /// void IList.RemoveAt(int index) { RemoveAt(index); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
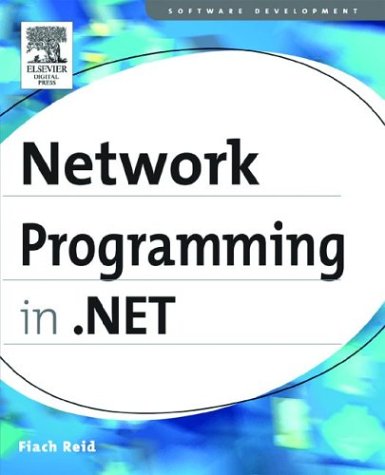
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableLayoutStyleCollection.cs
- WebPartCloseVerb.cs
- CompilerErrorCollection.cs
- XmlWrappingReader.cs
- TaskFileService.cs
- ObjectViewFactory.cs
- EmissiveMaterial.cs
- ArgumentNullException.cs
- XmlSchemaInfo.cs
- StyleTypedPropertyAttribute.cs
- UnauthorizedAccessException.cs
- DragCompletedEventArgs.cs
- ValidationPropertyAttribute.cs
- PlainXmlDeserializer.cs
- CatalogZoneDesigner.cs
- AttachedPropertyInfo.cs
- FixedDocumentPaginator.cs
- iisPickupDirectory.cs
- SamlAdvice.cs
- BaseTemplateCodeDomTreeGenerator.cs
- Stylus.cs
- InvalidDataContractException.cs
- ClassHandlersStore.cs
- StrongNamePublicKeyBlob.cs
- dbdatarecord.cs
- RegexInterpreter.cs
- WindowsListViewItemStartMenu.cs
- OutputCacheModule.cs
- HttpProfileGroupBase.cs
- WmlValidatorAdapter.cs
- Documentation.cs
- ReflectTypeDescriptionProvider.cs
- FrameworkContentElementAutomationPeer.cs
- AppDomainFactory.cs
- CultureInfoConverter.cs
- VectorAnimationBase.cs
- MissingMemberException.cs
- TriggerCollection.cs
- HitTestWithGeometryDrawingContextWalker.cs
- ListViewEditEventArgs.cs
- GridViewHeaderRowPresenter.cs
- AutoGeneratedField.cs
- Icon.cs
- versioninfo.cs
- CodeCompileUnit.cs
- SearchForVirtualItemEventArgs.cs
- UnsafeNativeMethodsTablet.cs
- ChooseAction.cs
- Subtree.cs
- HtmlShimManager.cs
- _TimerThread.cs
- RequestCachingSection.cs
- TreeWalker.cs
- TwoPhaseCommit.cs
- StringUtil.cs
- SafeHandle.cs
- Fx.cs
- OrderByQueryOptionExpression.cs
- IntellisenseTextBox.cs
- WindowsRichEditRange.cs
- HotCommands.cs
- HtmlInputPassword.cs
- DataServiceHost.cs
- TextWriter.cs
- ColumnBinding.cs
- TargetControlTypeCache.cs
- TabControlAutomationPeer.cs
- CustomTokenProvider.cs
- DriveNotFoundException.cs
- ReflectionServiceProvider.cs
- Cursors.cs
- Command.cs
- WebResponse.cs
- SystemIPInterfaceStatistics.cs
- ExceptionNotification.cs
- DataMemberFieldConverter.cs
- SystemNetworkInterface.cs
- MasterPageCodeDomTreeGenerator.cs
- returneventsaver.cs
- RayMeshGeometry3DHitTestResult.cs
- CreateParams.cs
- SystemColors.cs
- WizardSideBarListControlItem.cs
- ProfileEventArgs.cs
- TemplateComponentConnector.cs
- SHA1CryptoServiceProvider.cs
- ContextStaticAttribute.cs
- DummyDataSource.cs
- WindowsImpersonationContext.cs
- Types.cs
- XPathNodeHelper.cs
- BrowserInteropHelper.cs
- StateRuntime.cs
- DesignerProperties.cs
- PropertyPathConverter.cs
- ViewStateAttachedPropertyFeature.cs
- Blend.cs
- DataStorage.cs
- AnchorEditor.cs
- RegexStringValidatorAttribute.cs