Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / CngProvider.cs / 1305376 / CngProvider.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics.CodeAnalysis; using System.Diagnostics.Contracts; namespace System.Security.Cryptography { ////// Utility class to strongly type providers used with CNG. Since all CNG APIs which require a /// provider name take the name as a string, we use this string wrapper class to specifically mark /// which parameters are expected to be providers. We also provide a list of well known provider /// names, which helps Intellisense users find a set of good providernames to use. /// [Serializable] [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CngProvider : IEquatable{ private static CngProvider s_msSmartCardKsp; private static CngProvider s_msSoftwareKsp; private string m_provider; public CngProvider(string provider) { Contract.Ensures(!String.IsNullOrEmpty(m_provider)); if (provider == null) { throw new ArgumentNullException("provider"); } if (provider.Length == 0) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidProviderName, provider), "provider"); } m_provider = provider; } /// /// Name of the CNG provider /// public string Provider { get { Contract.Ensures(!String.IsNullOrEmpty(Contract.Result())); return m_provider; } } public static bool operator ==(CngProvider left, CngProvider right) { if (Object.ReferenceEquals(left, null)) { return Object.ReferenceEquals(right, null); } return left.Equals(right); } [Pure] public static bool operator !=(CngProvider left, CngProvider right) { if (Object.ReferenceEquals(left, null)) { return !Object.ReferenceEquals(right, null); } return !left.Equals(right); } public override bool Equals(object obj) { Contract.Assert(m_provider != null); return Equals(obj as CngProvider); } public bool Equals(CngProvider other) { if (Object.ReferenceEquals(other, null)) { return false; } return m_provider.Equals(other.Provider); } public override int GetHashCode() { Contract.Assert(m_provider != null); return m_provider.GetHashCode(); } public override string ToString() { Contract.Assert(m_provider != null); return m_provider.ToString(); } // // Well known NCrypt KSPs // [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", MessageId = "CardKey", Justification = "This is not 'Smart Cardkey', but 'Smart Card Key'")] [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", MessageId = "SmartCard", Justification = "Smart Card is two words in the ncrypt usage")] public static CngProvider MicrosoftSmartCardKeyStorageProvider { get { Contract.Ensures(Contract.Result () != null); if (s_msSmartCardKsp == null) { s_msSmartCardKsp = new CngProvider("Microsoft Smart Card Key Storage Provider"); // MS_SMART_CARD_KEY_STORAGE_PROVIDER } return s_msSmartCardKsp; } } public static CngProvider MicrosoftSoftwareKeyStorageProvider { get { Contract.Ensures(Contract.Result () != null); if (s_msSoftwareKsp == null) { s_msSoftwareKsp = new CngProvider("Microsoft Software Key Storage Provider"); // MS_KEY_STORAGE_PROVIDER } return s_msSoftwareKsp; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics.CodeAnalysis; using System.Diagnostics.Contracts; namespace System.Security.Cryptography { /// /// Utility class to strongly type providers used with CNG. Since all CNG APIs which require a /// provider name take the name as a string, we use this string wrapper class to specifically mark /// which parameters are expected to be providers. We also provide a list of well known provider /// names, which helps Intellisense users find a set of good providernames to use. /// [Serializable] [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CngProvider : IEquatable{ private static CngProvider s_msSmartCardKsp; private static CngProvider s_msSoftwareKsp; private string m_provider; public CngProvider(string provider) { Contract.Ensures(!String.IsNullOrEmpty(m_provider)); if (provider == null) { throw new ArgumentNullException("provider"); } if (provider.Length == 0) { throw new ArgumentException(SR.GetString(SR.Cryptography_InvalidProviderName, provider), "provider"); } m_provider = provider; } /// /// Name of the CNG provider /// public string Provider { get { Contract.Ensures(!String.IsNullOrEmpty(Contract.Result())); return m_provider; } } public static bool operator ==(CngProvider left, CngProvider right) { if (Object.ReferenceEquals(left, null)) { return Object.ReferenceEquals(right, null); } return left.Equals(right); } [Pure] public static bool operator !=(CngProvider left, CngProvider right) { if (Object.ReferenceEquals(left, null)) { return !Object.ReferenceEquals(right, null); } return !left.Equals(right); } public override bool Equals(object obj) { Contract.Assert(m_provider != null); return Equals(obj as CngProvider); } public bool Equals(CngProvider other) { if (Object.ReferenceEquals(other, null)) { return false; } return m_provider.Equals(other.Provider); } public override int GetHashCode() { Contract.Assert(m_provider != null); return m_provider.GetHashCode(); } public override string ToString() { Contract.Assert(m_provider != null); return m_provider.ToString(); } // // Well known NCrypt KSPs // [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", MessageId = "CardKey", Justification = "This is not 'Smart Cardkey', but 'Smart Card Key'")] [SuppressMessage("Microsoft.Naming", "CA1702:CompoundWordsShouldBeCasedCorrectly", MessageId = "SmartCard", Justification = "Smart Card is two words in the ncrypt usage")] public static CngProvider MicrosoftSmartCardKeyStorageProvider { get { Contract.Ensures(Contract.Result () != null); if (s_msSmartCardKsp == null) { s_msSmartCardKsp = new CngProvider("Microsoft Smart Card Key Storage Provider"); // MS_SMART_CARD_KEY_STORAGE_PROVIDER } return s_msSmartCardKsp; } } public static CngProvider MicrosoftSoftwareKeyStorageProvider { get { Contract.Ensures(Contract.Result () != null); if (s_msSoftwareKsp == null) { s_msSoftwareKsp = new CngProvider("Microsoft Software Key Storage Provider"); // MS_KEY_STORAGE_PROVIDER } return s_msSoftwareKsp; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
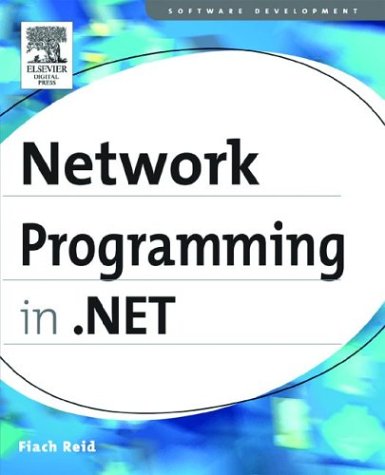
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ViewBase.cs
- RunClient.cs
- FullTextState.cs
- pingexception.cs
- AnnotationResource.cs
- CodeBlockBuilder.cs
- LinkDesigner.cs
- CachedCompositeFamily.cs
- RoleGroupCollection.cs
- WriteStateInfoBase.cs
- AnimationClockResource.cs
- InterleavedZipPartStream.cs
- GlyphingCache.cs
- NestedContainer.cs
- XamlFilter.cs
- ReferenceSchema.cs
- ClientSponsor.cs
- safex509handles.cs
- UserPersonalizationStateInfo.cs
- ServiceModelExtensionElement.cs
- PieceDirectory.cs
- AssemblyBuilderData.cs
- FontConverter.cs
- Paragraph.cs
- ByteViewer.cs
- AuthorizationSection.cs
- ClientData.cs
- SelectionPattern.cs
- AnimationLayer.cs
- FormattedText.cs
- GroupByExpressionRewriter.cs
- TraceUtility.cs
- WrapPanel.cs
- DecimalKeyFrameCollection.cs
- HttpHandlersSection.cs
- HeaderCollection.cs
- ClientSettingsStore.cs
- FilterUserControlBase.cs
- DataSourceCacheDurationConverter.cs
- TextServicesHost.cs
- StyleXamlParser.cs
- Quaternion.cs
- GAC.cs
- DecoderExceptionFallback.cs
- ValidatorCollection.cs
- StrongNameMembershipCondition.cs
- InstancePersistenceCommand.cs
- CompositeFontParser.cs
- PrivilegeNotHeldException.cs
- TakeQueryOptionExpression.cs
- TreeViewEvent.cs
- RotateTransform3D.cs
- ISFTagAndGuidCache.cs
- ScriptResourceAttribute.cs
- SqlDataSourceFilteringEventArgs.cs
- Verify.cs
- ImageKeyConverter.cs
- ProviderSettings.cs
- ListView.cs
- SapiGrammar.cs
- ImageAnimator.cs
- PathFigureCollectionConverter.cs
- Partitioner.cs
- TextBox.cs
- XomlCompilerError.cs
- ToolBarButtonClickEvent.cs
- ListView.cs
- SerializationEventsCache.cs
- sortedlist.cs
- LinqDataSourceValidationException.cs
- MultiDataTrigger.cs
- NameValueCollection.cs
- HttpCapabilitiesBase.cs
- SafeMILHandle.cs
- UnsafePeerToPeerMethods.cs
- PrimitiveList.cs
- GuidelineCollection.cs
- Visual3D.cs
- Highlights.cs
- NetworkCredential.cs
- CodeEntryPointMethod.cs
- TypePropertyEditor.cs
- BaseTemplateCodeDomTreeGenerator.cs
- ResizeGrip.cs
- DataGridViewCell.cs
- ReferenceEqualityComparer.cs
- WsdlBuildProvider.cs
- XmlObjectSerializer.cs
- GeometryGroup.cs
- AnnotationHighlightLayer.cs
- HashMembershipCondition.cs
- CompiledQuery.cs
- XamlStream.cs
- MimeImporter.cs
- TextEndOfLine.cs
- StylusPointCollection.cs
- SimpleHandlerBuildProvider.cs
- CheckBoxPopupAdapter.cs
- LineGeometry.cs
- ErrorWrapper.cs