Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / SafeMILHandle.cs / 1 / SafeMILHandle.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // A safe way to deal with unmanaged MIL interface pointers. //--------------------------------------------------------------------------- using System; using System.IO; using System.Security; using System.Security.Permissions; using System.Collections; using System.Reflection; using MS.Internal; using MS.Win32; using System.Diagnostics; using System.Windows.Media; using System.Runtime; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using Microsoft.Win32.SafeHandles; using Microsoft.Internal; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { internal class SafeMILHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Use this constructor if the handle isn't ready yet and later /// set the handle with SetHandle. SafeMILHandle owns the release /// of the handle. /// ////// Critical: This derives from a class that has a link demand and inheritance demand /// TreatAsSafe: Ok to call constructor /// [SecurityCritical,SecurityTreatAsSafe] internal SafeMILHandle() : base(true) { } ////// Use this constructor if the handle exists at construction time. /// SafeMILHandle owns the release of the parameter. /// ////// Critical: This code calls UpdateEstimatedSize. /// It is used to keep memory around /// [SecurityCritical] internal SafeMILHandle(IntPtr handle, long estimatedSize) : base(true) { SetHandle(handle); // // Hint the GC at the size of the unmanaged memory associated with // this object. We release pressure in the finalizer. // UpdateEstimatedSize(estimatedSize); } ////// Change our size to the new size specified /// ////// Critical: This code calls into AddMemoryPressure and RemoveMemoryPressure /// both of which have link demands. It is used to keep memory around /// [SecurityCritical] internal void UpdateEstimatedSize(long estimatedSize) { if (_gcPressure > 0) { MemoryPressure.Remove(_gcPressure); } _gcPressure = estimatedSize; if (_gcPressure > 0) { MemoryPressure.Add(_gcPressure); } } ////// Critical - calls unmanaged code, not treat as safe because you must /// validate that handle is a valid COM object. /// [SecurityCritical] protected override bool ReleaseHandle() { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref handle); // // We've released the unmangaed memory, so remove associated // GC pressure. // UpdateEstimatedSize(0); return true; } // // Estimated size in bytes of the unmanaged memory we are holding onto // private long _gcPressure; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // A safe way to deal with unmanaged MIL interface pointers. //--------------------------------------------------------------------------- using System; using System.IO; using System.Security; using System.Security.Permissions; using System.Collections; using System.Reflection; using MS.Internal; using MS.Win32; using System.Diagnostics; using System.Windows.Media; using System.Runtime; using System.Runtime.InteropServices; using System.Runtime.CompilerServices; using Microsoft.Win32.SafeHandles; using Microsoft.Internal; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { internal class SafeMILHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Use this constructor if the handle isn't ready yet and later /// set the handle with SetHandle. SafeMILHandle owns the release /// of the handle. /// ////// Critical: This derives from a class that has a link demand and inheritance demand /// TreatAsSafe: Ok to call constructor /// [SecurityCritical,SecurityTreatAsSafe] internal SafeMILHandle() : base(true) { } ////// Use this constructor if the handle exists at construction time. /// SafeMILHandle owns the release of the parameter. /// ////// Critical: This code calls UpdateEstimatedSize. /// It is used to keep memory around /// [SecurityCritical] internal SafeMILHandle(IntPtr handle, long estimatedSize) : base(true) { SetHandle(handle); // // Hint the GC at the size of the unmanaged memory associated with // this object. We release pressure in the finalizer. // UpdateEstimatedSize(estimatedSize); } ////// Change our size to the new size specified /// ////// Critical: This code calls into AddMemoryPressure and RemoveMemoryPressure /// both of which have link demands. It is used to keep memory around /// [SecurityCritical] internal void UpdateEstimatedSize(long estimatedSize) { if (_gcPressure > 0) { MemoryPressure.Remove(_gcPressure); } _gcPressure = estimatedSize; if (_gcPressure > 0) { MemoryPressure.Add(_gcPressure); } } ////// Critical - calls unmanaged code, not treat as safe because you must /// validate that handle is a valid COM object. /// [SecurityCritical] protected override bool ReleaseHandle() { UnsafeNativeMethods.MILUnknown.ReleaseInterface(ref handle); // // We've released the unmangaed memory, so remove associated // GC pressure. // UpdateEstimatedSize(0); return true; } // // Estimated size in bytes of the unmanaged memory we are holding onto // private long _gcPressure; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
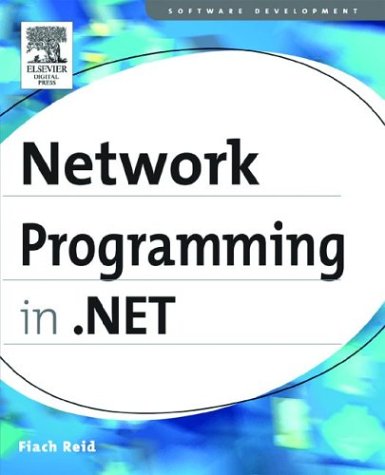
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssociationTypeEmitter.cs
- BoolExpr.cs
- HashHelper.cs
- IApplicationTrustManager.cs
- TraceSource.cs
- ConsumerConnectionPoint.cs
- MultipleViewPatternIdentifiers.cs
- SortFieldComparer.cs
- ElapsedEventArgs.cs
- NameValuePermission.cs
- EntityRecordInfo.cs
- TraceEventCache.cs
- InkCanvas.cs
- TextInfo.cs
- InvokeHandlers.cs
- CapiHashAlgorithm.cs
- DivideByZeroException.cs
- ThicknessKeyFrameCollection.cs
- TextContainer.cs
- CapabilitiesUse.cs
- WorkflowDefinitionDispenser.cs
- InternalsVisibleToAttribute.cs
- ConditionCollection.cs
- GlobalEventManager.cs
- BitmapMetadataEnumerator.cs
- RuleSettingsCollection.cs
- PerformanceCounterScope.cs
- Command.cs
- TypeContext.cs
- MouseBinding.cs
- WebEventTraceProvider.cs
- JsonWriter.cs
- TimestampInformation.cs
- XLinq.cs
- ImageIndexConverter.cs
- BasicDesignerLoader.cs
- ContractDescription.cs
- Logging.cs
- CurrencyWrapper.cs
- LineBreakRecord.cs
- KnownBoxes.cs
- BrushMappingModeValidation.cs
- MatrixAnimationUsingPath.cs
- AssociationTypeEmitter.cs
- PingOptions.cs
- FlowPosition.cs
- ErrorWrapper.cs
- XmlSchemaInferenceException.cs
- SRef.cs
- WebPartCatalogAddVerb.cs
- SelectionUIService.cs
- WindowsImpersonationContext.cs
- CachedFontFamily.cs
- CommandSet.cs
- ClientRoleProvider.cs
- ToolStripControlHost.cs
- InstanceLockException.cs
- Button.cs
- ContractListAdapter.cs
- TargetConverter.cs
- ToolStripSystemRenderer.cs
- DataGridItemAttachedStorage.cs
- DispatcherFrame.cs
- DataGridColumnCollection.cs
- EntityDesignerBuildProvider.cs
- SessionStateModule.cs
- DetailsViewCommandEventArgs.cs
- AppSecurityManager.cs
- WebBrowserBase.cs
- ToolStripSettings.cs
- base64Transforms.cs
- InsufficientMemoryException.cs
- HttpFileCollection.cs
- RegularExpressionValidator.cs
- SizeF.cs
- StickyNote.cs
- ViewBase.cs
- IsolatedStorageFileStream.cs
- ChangeConflicts.cs
- RecognizeCompletedEventArgs.cs
- SmiMetaDataProperty.cs
- SequenceDesigner.xaml.cs
- GraphicsContext.cs
- ParseElementCollection.cs
- Token.cs
- PropertySegmentSerializer.cs
- EventlogProvider.cs
- CompiledRegexRunnerFactory.cs
- SpinLock.cs
- WebControl.cs
- DesigntimeLicenseContext.cs
- UserControl.cs
- MD5HashHelper.cs
- PolicyStatement.cs
- RegistryPermission.cs
- ByteStreamGeometryContext.cs
- ClientRoleProvider.cs
- ToolStripComboBox.cs
- AvTrace.cs
- ShaderRenderModeValidation.cs