Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Serializer / PropertySegmentSerializer.cs / 1305376 / PropertySegmentSerializer.cs
namespace System.Workflow.ComponentModel.Serialization { using System; using System.Reflection; using System.Xml; using System.ComponentModel.Design.Serialization; using System.Collections; using System.Workflow.ComponentModel.Design; using System.ComponentModel; #region Class PropertySegmentSerializer internal sealed class PropertySegmentSerializer : WorkflowMarkupSerializer { private WorkflowMarkupSerializer containedSerializer = null; public PropertySegmentSerializer(WorkflowMarkupSerializer containedSerializer) { this.containedSerializer = containedSerializer; } protected internal override PropertyInfo[] GetProperties(WorkflowMarkupSerializationManager serializationManager, object obj) { if (this.containedSerializer != null) return this.containedSerializer.GetProperties(serializationManager, obj); if (obj != null && obj.GetType() == typeof(PropertySegment)) return (obj as PropertySegment).GetProperties(serializationManager); else return base.GetProperties(serializationManager, obj); } protected override object CreateInstance(WorkflowMarkupSerializationManager serializationManager, Type type) { if (typeof(PropertySegment) == type) return Activator.CreateInstance(type, new object[] { serializationManager as IServiceProvider, serializationManager.Context.Current }); else return base.CreateInstance(serializationManager, type); } protected internal override bool ShouldSerializeValue(WorkflowMarkupSerializationManager serializationManager, object value) { return true; } protected internal override bool CanSerializeToString(WorkflowMarkupSerializationManager serializationManager, object value) { bool canSerializeToString = false; if (value != null) { ITypeDescriptorContext context = null; TypeConverter converter = GetTypeConversionInfoForPropertySegment(serializationManager, value.GetType(), out context); if (converter != null) canSerializeToString = converter.CanConvertTo(context, typeof(string)); if (!canSerializeToString) { if (this.containedSerializer != null) canSerializeToString = this.containedSerializer.CanSerializeToString(serializationManager, value); else canSerializeToString = base.CanSerializeToString(serializationManager, value); } } else { canSerializeToString = true; } return canSerializeToString; } protected internal override string SerializeToString(WorkflowMarkupSerializationManager serializationManager, object value) { String stringValue = String.Empty; if (value == null) { stringValue = "*null"; } else { ITypeDescriptorContext context = null; TypeConverter converter = GetTypeConversionInfoForPropertySegment(serializationManager, value.GetType(), out context); if (converter != null && converter.CanConvertTo(context, typeof(string))) stringValue = converter.ConvertToString(context, value); else if (this.containedSerializer != null) stringValue = this.containedSerializer.SerializeToString(serializationManager, value); else stringValue = base.SerializeToString(serializationManager, value); } return stringValue; } protected internal override object DeserializeFromString(WorkflowMarkupSerializationManager serializationManager, Type propertyType, string value) { if (serializationManager == null) throw new ArgumentNullException("serializationManager"); if (propertyType == null) throw new ArgumentNullException("propertyType"); if (value == null) throw new ArgumentNullException("value"); object convertedValue = null; if (string.Equals(value, "*null", StringComparison.Ordinal)) { convertedValue = null; } else { ITypeDescriptorContext context = null; TypeConverter converter = GetTypeConversionInfoForPropertySegment(serializationManager, propertyType, out context); if (converter != null && converter.CanConvertFrom(context, typeof(string))) convertedValue = converter.ConvertFromString(context, value); else if (this.containedSerializer != null) convertedValue = this.containedSerializer.DeserializeFromString(serializationManager, propertyType, value); else convertedValue = base.DeserializeFromString(serializationManager, propertyType, value); } return convertedValue; } protected internal override IList GetChildren(WorkflowMarkupSerializationManager serializationManager, object obj) { if (this.containedSerializer != null) return this.containedSerializer.GetChildren(serializationManager, obj); return null; } protected internal override void ClearChildren(WorkflowMarkupSerializationManager serializationManager, object obj) { if (this.containedSerializer != null) this.containedSerializer.ClearChildren(serializationManager, obj); } protected internal override void AddChild(WorkflowMarkupSerializationManager serializationManager, object obj, object childObj) { if (this.containedSerializer != null) this.containedSerializer.AddChild(serializationManager, obj, childObj); } private TypeConverter GetTypeConversionInfoForPropertySegment(WorkflowMarkupSerializationManager serializationManager, Type propertyType, out ITypeDescriptorContext context) { TypeConverter converter = null; context = null; PropertySegmentPropertyInfo propertyInfo = serializationManager.Context[typeof(PropertySegmentPropertyInfo)] as PropertySegmentPropertyInfo; if (propertyInfo.PropertySegment != null) { if (propertyInfo.PropertySegment.PropertyDescriptor != null) { context = new TypeDescriptorContext(propertyInfo.PropertySegment.ServiceProvider, propertyInfo.PropertySegment.PropertyDescriptor, propertyInfo.PropertySegment.Object); converter = propertyInfo.PropertySegment.PropertyDescriptor.Converter; } else if (propertyInfo.PropertySegment.Object != null) { PropertyDescriptor propertyDescriptor = TypeDescriptor.GetProperties(propertyInfo.PropertySegment.Object)[propertyInfo.Name]; if (propertyDescriptor != null) { context = new TypeDescriptorContext(propertyInfo.PropertySegment.ServiceProvider, propertyDescriptor, propertyInfo.PropertySegment.Object); converter = propertyDescriptor.Converter; } } } if (propertyType != null && converter == null) { converter = TypeDescriptor.GetConverter(propertyType); } return converter; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.ComponentModel.Serialization { using System; using System.Reflection; using System.Xml; using System.ComponentModel.Design.Serialization; using System.Collections; using System.Workflow.ComponentModel.Design; using System.ComponentModel; #region Class PropertySegmentSerializer internal sealed class PropertySegmentSerializer : WorkflowMarkupSerializer { private WorkflowMarkupSerializer containedSerializer = null; public PropertySegmentSerializer(WorkflowMarkupSerializer containedSerializer) { this.containedSerializer = containedSerializer; } protected internal override PropertyInfo[] GetProperties(WorkflowMarkupSerializationManager serializationManager, object obj) { if (this.containedSerializer != null) return this.containedSerializer.GetProperties(serializationManager, obj); if (obj != null && obj.GetType() == typeof(PropertySegment)) return (obj as PropertySegment).GetProperties(serializationManager); else return base.GetProperties(serializationManager, obj); } protected override object CreateInstance(WorkflowMarkupSerializationManager serializationManager, Type type) { if (typeof(PropertySegment) == type) return Activator.CreateInstance(type, new object[] { serializationManager as IServiceProvider, serializationManager.Context.Current }); else return base.CreateInstance(serializationManager, type); } protected internal override bool ShouldSerializeValue(WorkflowMarkupSerializationManager serializationManager, object value) { return true; } protected internal override bool CanSerializeToString(WorkflowMarkupSerializationManager serializationManager, object value) { bool canSerializeToString = false; if (value != null) { ITypeDescriptorContext context = null; TypeConverter converter = GetTypeConversionInfoForPropertySegment(serializationManager, value.GetType(), out context); if (converter != null) canSerializeToString = converter.CanConvertTo(context, typeof(string)); if (!canSerializeToString) { if (this.containedSerializer != null) canSerializeToString = this.containedSerializer.CanSerializeToString(serializationManager, value); else canSerializeToString = base.CanSerializeToString(serializationManager, value); } } else { canSerializeToString = true; } return canSerializeToString; } protected internal override string SerializeToString(WorkflowMarkupSerializationManager serializationManager, object value) { String stringValue = String.Empty; if (value == null) { stringValue = "*null"; } else { ITypeDescriptorContext context = null; TypeConverter converter = GetTypeConversionInfoForPropertySegment(serializationManager, value.GetType(), out context); if (converter != null && converter.CanConvertTo(context, typeof(string))) stringValue = converter.ConvertToString(context, value); else if (this.containedSerializer != null) stringValue = this.containedSerializer.SerializeToString(serializationManager, value); else stringValue = base.SerializeToString(serializationManager, value); } return stringValue; } protected internal override object DeserializeFromString(WorkflowMarkupSerializationManager serializationManager, Type propertyType, string value) { if (serializationManager == null) throw new ArgumentNullException("serializationManager"); if (propertyType == null) throw new ArgumentNullException("propertyType"); if (value == null) throw new ArgumentNullException("value"); object convertedValue = null; if (string.Equals(value, "*null", StringComparison.Ordinal)) { convertedValue = null; } else { ITypeDescriptorContext context = null; TypeConverter converter = GetTypeConversionInfoForPropertySegment(serializationManager, propertyType, out context); if (converter != null && converter.CanConvertFrom(context, typeof(string))) convertedValue = converter.ConvertFromString(context, value); else if (this.containedSerializer != null) convertedValue = this.containedSerializer.DeserializeFromString(serializationManager, propertyType, value); else convertedValue = base.DeserializeFromString(serializationManager, propertyType, value); } return convertedValue; } protected internal override IList GetChildren(WorkflowMarkupSerializationManager serializationManager, object obj) { if (this.containedSerializer != null) return this.containedSerializer.GetChildren(serializationManager, obj); return null; } protected internal override void ClearChildren(WorkflowMarkupSerializationManager serializationManager, object obj) { if (this.containedSerializer != null) this.containedSerializer.ClearChildren(serializationManager, obj); } protected internal override void AddChild(WorkflowMarkupSerializationManager serializationManager, object obj, object childObj) { if (this.containedSerializer != null) this.containedSerializer.AddChild(serializationManager, obj, childObj); } private TypeConverter GetTypeConversionInfoForPropertySegment(WorkflowMarkupSerializationManager serializationManager, Type propertyType, out ITypeDescriptorContext context) { TypeConverter converter = null; context = null; PropertySegmentPropertyInfo propertyInfo = serializationManager.Context[typeof(PropertySegmentPropertyInfo)] as PropertySegmentPropertyInfo; if (propertyInfo.PropertySegment != null) { if (propertyInfo.PropertySegment.PropertyDescriptor != null) { context = new TypeDescriptorContext(propertyInfo.PropertySegment.ServiceProvider, propertyInfo.PropertySegment.PropertyDescriptor, propertyInfo.PropertySegment.Object); converter = propertyInfo.PropertySegment.PropertyDescriptor.Converter; } else if (propertyInfo.PropertySegment.Object != null) { PropertyDescriptor propertyDescriptor = TypeDescriptor.GetProperties(propertyInfo.PropertySegment.Object)[propertyInfo.Name]; if (propertyDescriptor != null) { context = new TypeDescriptorContext(propertyInfo.PropertySegment.ServiceProvider, propertyDescriptor, propertyInfo.PropertySegment.Object); converter = propertyDescriptor.Converter; } } } if (propertyType != null && converter == null) { converter = TypeDescriptor.GetConverter(propertyType); } return converter; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
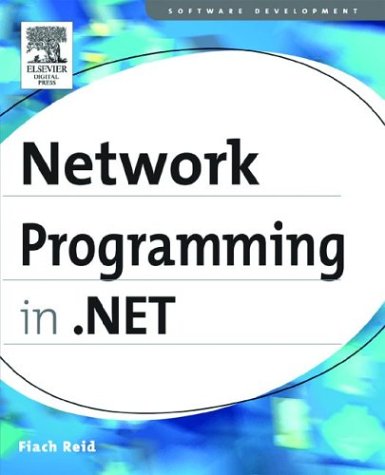
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ActiveDocumentEvent.cs
- Expression.cs
- Clipboard.cs
- ContractInstanceProvider.cs
- ListViewItem.cs
- ConfigurationValidatorBase.cs
- ExpressionTextBoxAutomationPeer.cs
- BamlWriter.cs
- NetNamedPipeBindingCollectionElement.cs
- RegexStringValidatorAttribute.cs
- ImmComposition.cs
- InheritablePropertyChangeInfo.cs
- Bookmark.cs
- TextEffect.cs
- ProgressBarBrushConverter.cs
- DataGridTable.cs
- DataServiceQueryException.cs
- QueuedDeliveryRequirementsMode.cs
- PageSettings.cs
- WebPartEventArgs.cs
- TypeExtensions.cs
- RegexMatch.cs
- TimelineGroup.cs
- SoapFormatExtensions.cs
- SourceSwitch.cs
- IpcManager.cs
- ValidationErrorEventArgs.cs
- RecordBuilder.cs
- PathGeometry.cs
- Pens.cs
- httpapplicationstate.cs
- DictionaryKeyPropertyAttribute.cs
- Vector3DKeyFrameCollection.cs
- Message.cs
- FocusTracker.cs
- ObjRef.cs
- Win32.cs
- TextRunCacheImp.cs
- SecurityTokenAuthenticator.cs
- WSTrust.cs
- CodeVariableDeclarationStatement.cs
- XmlDocumentSerializer.cs
- TextTrailingWordEllipsis.cs
- SqlError.cs
- RequestNavigateEventArgs.cs
- wgx_render.cs
- ValueType.cs
- IImplicitResourceProvider.cs
- ForceCopyBuildProvider.cs
- EntityWithKeyStrategy.cs
- TableProviderWrapper.cs
- UndoManager.cs
- MaskInputRejectedEventArgs.cs
- AutomationTextAttribute.cs
- EntityDataSourceDesignerHelper.cs
- AppliedDeviceFiltersDialog.cs
- UrlAuthorizationModule.cs
- AllMembershipCondition.cs
- CustomAttributeFormatException.cs
- Parsers.cs
- StringConverter.cs
- PreloadedPackages.cs
- WindowsAltTab.cs
- DataSourceUtil.cs
- CatalogPartCollection.cs
- MessagePartSpecification.cs
- AlphabeticalEnumConverter.cs
- JsonWriter.cs
- BaseAsyncResult.cs
- BindingExpressionUncommonField.cs
- DateTimeConverter.cs
- _NTAuthentication.cs
- MessageFilter.cs
- DEREncoding.cs
- KeyGestureConverter.cs
- PageContentAsyncResult.cs
- XmlAttributeOverrides.cs
- OdbcError.cs
- UniformGrid.cs
- Tokenizer.cs
- rsa.cs
- CoreSwitches.cs
- ConstraintManager.cs
- StackSpiller.cs
- FontEmbeddingManager.cs
- SafeRightsManagementHandle.cs
- ControlPaint.cs
- OrderByQueryOptionExpression.cs
- WorkflowMarkupSerializer.cs
- GeneratedView.cs
- BindingList.cs
- SystemMulticastIPAddressInformation.cs
- CompositeDesignerAccessibleObject.cs
- PreloadedPackages.cs
- FileUtil.cs
- Validator.cs
- WindowsStatic.cs
- SecurityTokenRequirement.cs
- LinqToSqlWrapper.cs
- StylusPointProperties.cs