Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / TimelineGroup.cs / 1305600 / TimelineGroup.cs
//---------------------------------------------------------------------------- //// Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using System.Windows.Markup; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { ////// This class represents base class for Timelines that have functionality /// related to having Children. /// [ContentProperty("Children")] public abstract partial class TimelineGroup : Timeline, IAddChild { #region Constructors ////// Creates a TimelineGroup with default properties. /// protected TimelineGroup() : base() { } ////// Creates a TimelineGroup with the specified BeginTime. /// /// /// The scheduled BeginTime for this TimelineGroup. /// protected TimelineGroup(NullablebeginTime) : base(beginTime) { } /// /// Creates a TimelineGroup with the specified begin time and duration. /// /// /// The scheduled BeginTime for this TimelineGroup. /// /// /// The simple Duration of this TimelineGroup. /// protected TimelineGroup(NullablebeginTime, Duration duration) : base(beginTime, duration) { } /// /// Creates a TimelineGroup with the specified BeginTime, Duration and RepeatBehavior. /// /// /// The scheduled BeginTime for this TimelineGroup. /// /// /// The simple Duration of this TimelineGroup. /// /// /// The RepeatBehavior for this TimelineGroup. /// protected TimelineGroup(NullablebeginTime, Duration duration, RepeatBehavior repeatBehavior) : base(beginTime, duration, repeatBehavior) { } #endregion #region Timeline /// /// /// ///protected internal override Clock AllocateClock() { return new ClockGroup(this); } /// /// Creates a new ClockGroup using this TimelineGroup. /// ///A new ClockGroup. new public ClockGroup CreateClock() { return (ClockGroup)base.CreateClock(); } #endregion #region IAddChild interface ////// Adds a child object to this TimelineGroup. /// /// /// The child object to add. /// ////// A Timeline only accepts another Timeline (or derived class) as /// a child. /// void IAddChild.AddChild(object child) { WritePreamble(); if (child == null) { throw new ArgumentNullException("child"); } AddChild(child); WritePostscript(); } ////// This method performs the core functionality of the AddChild() /// method on the IAddChild interface. For a Timeline this means /// determining adding the child parameter to the Children collection /// if it's a Timeline. /// ////// This method is the only core implementation. It does not call /// WritePreamble() or WritePostscript(). It also doesn't throw an /// ArgumentNullException if the child parameter is null. These tasks /// are performed by the interface implementation. Therefore, it's OK /// for a derived class to override this method and call the base /// class implementation only if they determine that it's the right /// course of action. The derived class can rely on Timeline's /// implementation of IAddChild.AddChild or implement their own /// following the Freezable pattern since that would be a public /// method. /// /// An object representing the child that /// should be added. If this is a Timeline it will be added to the /// Children collection; otherwise an exception will be thrown. ///The child parameter is not a /// Timeline. [EditorBrowsable(EditorBrowsableState.Advanced)] protected virtual void AddChild(object child) { Timeline timelineChild = child as Timeline; if (timelineChild == null) { throw new ArgumentException(SR.Get(SRID.Timing_ChildMustBeTimeline), "child"); } else { Children.Add(timelineChild); } } ////// Adds a text string as a child of this Timeline. /// /// /// The text to add. /// ////// A Timeline does not accept text as a child, so this method will /// raise an InvalididOperationException unless a derived class has /// overridden the behavior to add text. /// ///The childText parameter is /// null. void IAddChild.AddText(string childText) { WritePreamble(); if (childText == null) { throw new ArgumentNullException("childText"); } AddText(childText); WritePostscript(); } ////// This method performs the core functionality of the AddText() /// method on the IAddChild interface. For a Timeline this means /// throwing and InvalidOperationException because it doesn't /// support adding text. /// ////// This method is the only core implementation. It does not call /// WritePreamble() or WritePostscript(). It also doesn't throw an /// ArgumentNullException if the childText parameter is null. These tasks /// are performed by the interface implementation. Therefore, it's OK /// for a derived class to override this method and call the base /// class implementation only if they determine that it's the right /// course of action. The derived class can rely on Timeline's /// implementation of IAddChild.AddChild or implement their own /// following the Freezable pattern since that would be a public /// method. /// /// A string representing the child text that /// should be added. If this is a Timeline an exception will be /// thrown. ///Timelines have no way /// of adding text. [EditorBrowsable(EditorBrowsableState.Advanced)] protected virtual void AddText(string childText) { throw new InvalidOperationException(SR.Get(SRID.Timing_NoTextChildren)); } #endregion // IAddChild interface } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
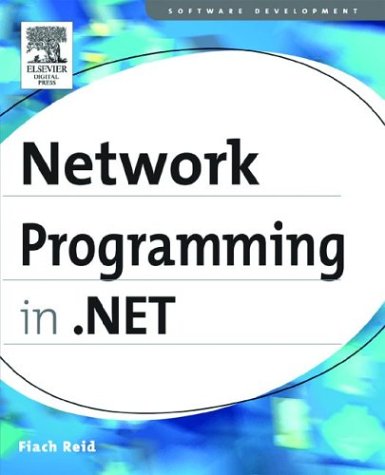
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleDbDataAdapter.cs
- BooleanToVisibilityConverter.cs
- Certificate.cs
- ComponentChangingEvent.cs
- WebPartEditVerb.cs
- RowUpdatingEventArgs.cs
- Cursors.cs
- GeneralEndpointIdentity.cs
- FileUpload.cs
- GZipObjectSerializer.cs
- MD5CryptoServiceProvider.cs
- PropertyDescriptor.cs
- InputScope.cs
- GlobalEventManager.cs
- InProcStateClientManager.cs
- ValidationSummary.cs
- EntityDesignerUtils.cs
- ScaleTransform3D.cs
- RightsManagementSuppressedStream.cs
- BamlVersionHeader.cs
- SafeNativeHandle.cs
- ByteFacetDescriptionElement.cs
- Int64AnimationUsingKeyFrames.cs
- ResourceExpressionEditorSheet.cs
- StrokeCollection2.cs
- CodeTypeDeclaration.cs
- DurableInstance.cs
- DefaultEventAttribute.cs
- FormViewDeletedEventArgs.cs
- DesignTimeVisibleAttribute.cs
- base64Transforms.cs
- Pointer.cs
- QualifiedCellIdBoolean.cs
- MimeTypeAttribute.cs
- AdCreatedEventArgs.cs
- XamlToRtfWriter.cs
- EntityDataSourceConfigureObjectContext.cs
- FigureHelper.cs
- CaseInsensitiveHashCodeProvider.cs
- AttributeQuery.cs
- DataGrid.cs
- AttachedProperty.cs
- OutKeywords.cs
- CryptoConfig.cs
- PopupRootAutomationPeer.cs
- PolicyStatement.cs
- DataGridViewComboBoxEditingControl.cs
- XslCompiledTransform.cs
- CommandEventArgs.cs
- XmlRawWriterWrapper.cs
- _PooledStream.cs
- Material.cs
- PartialList.cs
- ProviderSettings.cs
- BaseDataList.cs
- SendActivityDesigner.cs
- QueryStringParameter.cs
- DataGridSortCommandEventArgs.cs
- DataSourceXmlAttributeAttribute.cs
- MetadataArtifactLoaderCompositeFile.cs
- TypeLoadException.cs
- CodeTypeConstructor.cs
- StringWriter.cs
- XmlDocumentFieldSchema.cs
- ApplicationContext.cs
- ISAPIWorkerRequest.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- Transform.cs
- DocumentPage.cs
- MasterPage.cs
- DataKeyArray.cs
- RadioButtonPopupAdapter.cs
- StreamHelper.cs
- PackageRelationshipSelector.cs
- UpdateEventArgs.cs
- HttpRequestCacheValidator.cs
- UIElementParagraph.cs
- _ConnectionGroup.cs
- DataSourceControl.cs
- TailCallAnalyzer.cs
- HttpException.cs
- EntityDataSourceDataSelectionPanel.cs
- NullableLongSumAggregationOperator.cs
- TreeNodeMouseHoverEvent.cs
- CompilationUtil.cs
- URL.cs
- ITreeGenerator.cs
- TimelineCollection.cs
- AsyncOperationManager.cs
- IISUnsafeMethods.cs
- DbConnectionPoolOptions.cs
- ClientOptions.cs
- HMACRIPEMD160.cs
- AuthenticationException.cs
- SqlDependency.cs
- Interlocked.cs
- RoutedUICommand.cs
- RawStylusSystemGestureInputReport.cs
- MDIClient.cs
- WebPartZone.cs