Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / EntityDesignerUtils.cs / 1 / EntityDesignerUtils.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.IO; using System.Text; using System.Xml; using System.Data.Metadata.Edm; using System.Data.Mapping; namespace System.Data.Entity.Design { internal static class EntityDesignerUtils { internal static readonly string _edmxNamespace = "http://schemas.microsoft.com/ado/2007/06/edmx"; internal static readonly string _edmxFileExtension = ".edmx"; ////// Extract the Conceptual, Mapping and Storage nodes from an EDMX input streams, and extract the value of the metadataArtifactProcessing property. /// /// /// /// /// /// internal static void ExtractConceptualMappingAndStorageNodes(StreamReader edmxInputStream, out XmlElement conceptualSchemaNode, out XmlElement mappingNode, out XmlElement storageSchemaNode, out string metadataArtifactProcessingValue) { // load up an XML document representing the edmx file XmlDocument xmlDocument = new XmlDocument(); xmlDocument.Load(edmxInputStream); XmlNamespaceManager nsMgr = new XmlNamespaceManager(xmlDocument.NameTable); nsMgr.AddNamespace("edmx", _edmxNamespace); nsMgr.AddNamespace("edm", XmlConstants.ModelNamespace); nsMgr.AddNamespace("ssdl", XmlConstants.TargetNamespace); nsMgr.AddNamespace("map", StorageMslConstructs.NamespaceURI); // find the ConceptualModel Schema node conceptualSchemaNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:ConceptualModels/edm:Schema", nsMgr); // find the StorageModel Schema node storageSchemaNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:StorageModels/ssdl:Schema", nsMgr); // find the Mapping node mappingNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:Mappings/map:Mapping", nsMgr); // find the Connection node metadataArtifactProcessingValue = String.Empty; XmlNodeList connectionProperties = xmlDocument.SelectNodes( "/edmx:Edmx/edmx:Designer/edmx:Connection/edmx:DesignerInfoPropertySet/edmx:DesignerProperty", nsMgr); if (connectionProperties != null) { foreach (XmlNode propertyNode in connectionProperties) { foreach (XmlAttribute a in propertyNode.Attributes) { // treat attribute names case-sensitive (since it is xml), but attribute value case-insensitive to be accommodating . if (a.Name.Equals("Name", StringComparison.Ordinal) && a.Value.Equals("MetadataArtifactProcessing", StringComparison.OrdinalIgnoreCase)) { foreach (XmlAttribute a2 in propertyNode.Attributes) { if (a2.Name.Equals("Value", StringComparison.Ordinal)) { metadataArtifactProcessingValue = a2.Value; break; } } } } } } } // utility method to ensure an XmlElement (containing the C, M or S element // from the Edmx file) is sent out to a stream in the same format internal static void OutputXmlElementToStream(XmlElement xmlElement, Stream stream) { XmlWriterSettings settings = new XmlWriterSettings(); settings.Encoding = Encoding.UTF8; settings.Indent = true; // set up output document XmlDocument outputXmlDoc = new XmlDocument(); XmlNode importedElement = outputXmlDoc.ImportNode(xmlElement, true); outputXmlDoc.AppendChild(importedElement); // write out XmlDocument XmlWriter writer = null; try { writer = XmlWriter.Create(stream, settings); outputXmlDoc.WriteTo(writer); } finally { if (writer != null) { writer.Close(); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.IO; using System.Text; using System.Xml; using System.Data.Metadata.Edm; using System.Data.Mapping; namespace System.Data.Entity.Design { internal static class EntityDesignerUtils { internal static readonly string _edmxNamespace = "http://schemas.microsoft.com/ado/2007/06/edmx"; internal static readonly string _edmxFileExtension = ".edmx"; ////// Extract the Conceptual, Mapping and Storage nodes from an EDMX input streams, and extract the value of the metadataArtifactProcessing property. /// /// /// /// /// /// internal static void ExtractConceptualMappingAndStorageNodes(StreamReader edmxInputStream, out XmlElement conceptualSchemaNode, out XmlElement mappingNode, out XmlElement storageSchemaNode, out string metadataArtifactProcessingValue) { // load up an XML document representing the edmx file XmlDocument xmlDocument = new XmlDocument(); xmlDocument.Load(edmxInputStream); XmlNamespaceManager nsMgr = new XmlNamespaceManager(xmlDocument.NameTable); nsMgr.AddNamespace("edmx", _edmxNamespace); nsMgr.AddNamespace("edm", XmlConstants.ModelNamespace); nsMgr.AddNamespace("ssdl", XmlConstants.TargetNamespace); nsMgr.AddNamespace("map", StorageMslConstructs.NamespaceURI); // find the ConceptualModel Schema node conceptualSchemaNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:ConceptualModels/edm:Schema", nsMgr); // find the StorageModel Schema node storageSchemaNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:StorageModels/ssdl:Schema", nsMgr); // find the Mapping node mappingNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:Mappings/map:Mapping", nsMgr); // find the Connection node metadataArtifactProcessingValue = String.Empty; XmlNodeList connectionProperties = xmlDocument.SelectNodes( "/edmx:Edmx/edmx:Designer/edmx:Connection/edmx:DesignerInfoPropertySet/edmx:DesignerProperty", nsMgr); if (connectionProperties != null) { foreach (XmlNode propertyNode in connectionProperties) { foreach (XmlAttribute a in propertyNode.Attributes) { // treat attribute names case-sensitive (since it is xml), but attribute value case-insensitive to be accommodating . if (a.Name.Equals("Name", StringComparison.Ordinal) && a.Value.Equals("MetadataArtifactProcessing", StringComparison.OrdinalIgnoreCase)) { foreach (XmlAttribute a2 in propertyNode.Attributes) { if (a2.Name.Equals("Value", StringComparison.Ordinal)) { metadataArtifactProcessingValue = a2.Value; break; } } } } } } } // utility method to ensure an XmlElement (containing the C, M or S element // from the Edmx file) is sent out to a stream in the same format internal static void OutputXmlElementToStream(XmlElement xmlElement, Stream stream) { XmlWriterSettings settings = new XmlWriterSettings(); settings.Encoding = Encoding.UTF8; settings.Indent = true; // set up output document XmlDocument outputXmlDoc = new XmlDocument(); XmlNode importedElement = outputXmlDoc.ImportNode(xmlElement, true); outputXmlDoc.AppendChild(importedElement); // write out XmlDocument XmlWriter writer = null; try { writer = XmlWriter.Create(stream, settings); outputXmlDoc.WriteTo(writer); } finally { if (writer != null) { writer.Close(); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
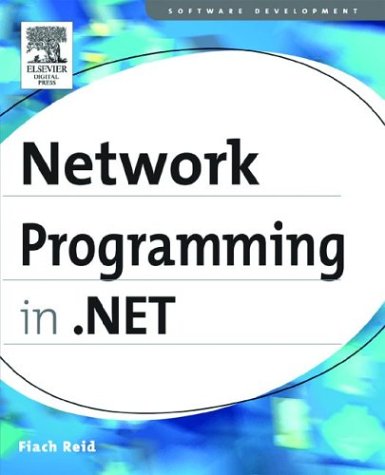
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeUserTokenHandle.cs
- HyperLinkDesigner.cs
- PriorityChain.cs
- DataGridViewRowsRemovedEventArgs.cs
- relpropertyhelper.cs
- MarginCollapsingState.cs
- HandleCollector.cs
- StylusPointProperties.cs
- DataGridViewBand.cs
- _SSPIWrapper.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- InputManager.cs
- MergePropertyDescriptor.cs
- RtfToXamlReader.cs
- RenameRuleObjectDialog.Designer.cs
- SoapObjectReader.cs
- Types.cs
- TableRow.cs
- EntityKey.cs
- WindowsScrollBar.cs
- XpsLiterals.cs
- InternalControlCollection.cs
- MetroSerializationManager.cs
- Thickness.cs
- FragmentNavigationEventArgs.cs
- SettingsProviderCollection.cs
- PointLight.cs
- ServiceErrorHandler.cs
- DictionaryEntry.cs
- ImageButton.cs
- ClickablePoint.cs
- ModifiableIteratorCollection.cs
- HttpApplication.cs
- CompilerTypeWithParams.cs
- EdmItemCollection.cs
- PromptBuilder.cs
- MenuItem.cs
- CodeComment.cs
- XmlMemberMapping.cs
- SQLString.cs
- BorderGapMaskConverter.cs
- FormatterConverter.cs
- DataGridTextBoxColumn.cs
- SByteStorage.cs
- SqlCharStream.cs
- PipeStream.cs
- EditorBrowsableAttribute.cs
- OleAutBinder.cs
- SByteConverter.cs
- LoadRetryConstantStrategy.cs
- VariableElement.cs
- DataGrid.cs
- DataTableNewRowEvent.cs
- RemotingConfiguration.cs
- CaseInsensitiveComparer.cs
- Roles.cs
- MobileFormsAuthentication.cs
- ReadOnlyNameValueCollection.cs
- SharedDp.cs
- WebPartZone.cs
- MasterPageBuildProvider.cs
- HebrewCalendar.cs
- SrgsNameValueTag.cs
- XmlException.cs
- SwitchCase.cs
- WebPartExportVerb.cs
- X509ScopedServiceCertificateElementCollection.cs
- WindowsEditBoxRange.cs
- CustomError.cs
- ToolStripItem.cs
- AddValidationError.cs
- SystemDropShadowChrome.cs
- WebServiceHandlerFactory.cs
- XmlMtomReader.cs
- EntityDataSourceSelectingEventArgs.cs
- WindowsScrollBarBits.cs
- Context.cs
- EntityKey.cs
- LayoutEditorPart.cs
- __FastResourceComparer.cs
- SessionStateUtil.cs
- DataSourceXmlSerializationAttribute.cs
- WindowsFormsSectionHandler.cs
- IfJoinedCondition.cs
- SqlBulkCopyColumnMapping.cs
- RecordBuilder.cs
- ImportFileRequest.cs
- OleCmdHelper.cs
- BaseInfoTable.cs
- Model3DGroup.cs
- FastPropertyAccessor.cs
- PackageRelationshipSelector.cs
- Collection.cs
- EncoderParameters.cs
- Pointer.cs
- NamedObject.cs
- ActivationServices.cs
- RoutedPropertyChangedEventArgs.cs
- MachineKeySection.cs
- MailMessageEventArgs.cs