Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Controls / Primitives / StatusBar.cs / 1 / StatusBar.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Peers; using System.Windows.Media; using System.Windows.Threading; using MS.Internal.KnownBoxes; namespace System.Windows.Controls.Primitives { ////// StatusBar is a visual indicator of the operational status of an application and/or /// its components running in a window. StatusBar control consists of a series of zones /// on a band that can display text, graphics, or other rich content. The control can /// group items within these zones to emphasize relational similarities or functional /// connections. The StatusBar can accommodate multiple sets of UI or functionality that /// can be chosen even within the same application. /// [StyleTypedProperty(Property = "ItemContainerStyle", StyleTargetType = typeof(StatusBarItem))] public class StatusBar : ItemsControl { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors static StatusBar() { DefaultStyleKeyProperty.OverrideMetadata(typeof(StatusBar), new FrameworkPropertyMetadata(typeof(StatusBar))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(StatusBar)); IsTabStopProperty.OverrideMetadata(typeof(StatusBar), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); ItemsPanelTemplate template = new ItemsPanelTemplate(new FrameworkElementFactory(typeof(DockPanel))); template.Seal(); ItemsPanelProperty.OverrideMetadata(typeof(StatusBar), new FrameworkPropertyMetadata(template)); } #endregion //-------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------- #region Protected Methods ////// Return true if the item is (or is eligible to be) its own ItemUI /// protected override bool IsItemItsOwnContainerOverride(object item) { return (item is StatusBarItem) || (item is Separator); } ////// Create or identify the element used to display the given item /// protected override DependencyObject GetContainerForItemOverride() { return new StatusBarItem(); } ////// Prepare the element to display the item. This may involve /// applying styles, setting bindings, etc. /// protected override void PrepareContainerForItemOverride(DependencyObject element, object item) { base.PrepareContainerForItemOverride(element, item); Separator separator = element as Separator; if (separator != null) { bool hasModifiers; BaseValueSourceInternal vs = separator.GetValueSource(StyleProperty, null, out hasModifiers); if (vs <= BaseValueSourceInternal.ImplicitReference) separator.SetResourceReference(StyleProperty, SeparatorStyleKey); separator.DefaultStyleKey = SeparatorStyleKey; } } ////// Determine whether the ItemContainerStyle/StyleSelector should apply to the container /// ///false if item is a Separator, otherwise return true protected override bool ShouldApplyItemContainerStyle(DependencyObject container, object item) { if (item is Separator) { return false; } else { return base.ShouldApplyItemContainerStyle(container, item); } } #endregion #region Accessibility ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new StatusBarAutomationPeer(this); } #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey #region ItemsStyleKey ///) /// /// Resource Key for the SeparatorStyle /// public static ResourceKey SeparatorStyleKey { get { if (_cacheSeparatorStyle == null) { _cacheSeparatorStyle = new SystemThemeKey(SystemResourceKeyID.StatusBarSeparatorStyle); } return _cacheSeparatorStyle; } } private static SystemThemeKey _cacheSeparatorStyle; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Peers; using System.Windows.Media; using System.Windows.Threading; using MS.Internal.KnownBoxes; namespace System.Windows.Controls.Primitives { ////// StatusBar is a visual indicator of the operational status of an application and/or /// its components running in a window. StatusBar control consists of a series of zones /// on a band that can display text, graphics, or other rich content. The control can /// group items within these zones to emphasize relational similarities or functional /// connections. The StatusBar can accommodate multiple sets of UI or functionality that /// can be chosen even within the same application. /// [StyleTypedProperty(Property = "ItemContainerStyle", StyleTargetType = typeof(StatusBarItem))] public class StatusBar : ItemsControl { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors static StatusBar() { DefaultStyleKeyProperty.OverrideMetadata(typeof(StatusBar), new FrameworkPropertyMetadata(typeof(StatusBar))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(StatusBar)); IsTabStopProperty.OverrideMetadata(typeof(StatusBar), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); ItemsPanelTemplate template = new ItemsPanelTemplate(new FrameworkElementFactory(typeof(DockPanel))); template.Seal(); ItemsPanelProperty.OverrideMetadata(typeof(StatusBar), new FrameworkPropertyMetadata(template)); } #endregion //-------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------- #region Protected Methods ////// Return true if the item is (or is eligible to be) its own ItemUI /// protected override bool IsItemItsOwnContainerOverride(object item) { return (item is StatusBarItem) || (item is Separator); } ////// Create or identify the element used to display the given item /// protected override DependencyObject GetContainerForItemOverride() { return new StatusBarItem(); } ////// Prepare the element to display the item. This may involve /// applying styles, setting bindings, etc. /// protected override void PrepareContainerForItemOverride(DependencyObject element, object item) { base.PrepareContainerForItemOverride(element, item); Separator separator = element as Separator; if (separator != null) { bool hasModifiers; BaseValueSourceInternal vs = separator.GetValueSource(StyleProperty, null, out hasModifiers); if (vs <= BaseValueSourceInternal.ImplicitReference) separator.SetResourceReference(StyleProperty, SeparatorStyleKey); separator.DefaultStyleKey = SeparatorStyleKey; } } ////// Determine whether the ItemContainerStyle/StyleSelector should apply to the container /// ///false if item is a Separator, otherwise return true protected override bool ShouldApplyItemContainerStyle(DependencyObject container, object item) { if (item is Separator) { return false; } else { return base.ShouldApplyItemContainerStyle(container, item); } } #endregion #region Accessibility ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new StatusBarAutomationPeer(this); } #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey #region ItemsStyleKey ///) /// /// Resource Key for the SeparatorStyle /// public static ResourceKey SeparatorStyleKey { get { if (_cacheSeparatorStyle == null) { _cacheSeparatorStyle = new SystemThemeKey(SystemResourceKeyID.StatusBarSeparatorStyle); } return _cacheSeparatorStyle; } } private static SystemThemeKey _cacheSeparatorStyle; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
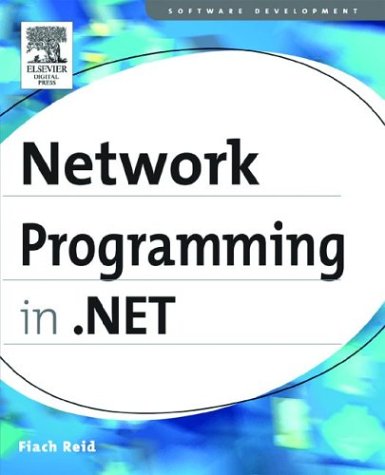
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ChangeBlockUndoRecord.cs
- ReliabilityContractAttribute.cs
- EntityWrapper.cs
- ListBox.cs
- DetailsViewDeletedEventArgs.cs
- StringResourceManager.cs
- DesignerDataView.cs
- LinkButton.cs
- XPathSingletonIterator.cs
- IntPtr.cs
- DataGridRowClipboardEventArgs.cs
- Property.cs
- BindingMemberInfo.cs
- ConnectionPoint.cs
- ListControl.cs
- IdentityReference.cs
- ObjectStateManager.cs
- DataControlField.cs
- TextElementEditingBehaviorAttribute.cs
- DebugHandleTracker.cs
- MemoryRecordBuffer.cs
- LambdaCompiler.Logical.cs
- SqlTopReducer.cs
- RightsManagementInformation.cs
- BridgeDataReader.cs
- WebProxyScriptElement.cs
- RegexCompilationInfo.cs
- Soap11ServerProtocol.cs
- ADRoleFactoryConfiguration.cs
- PassportAuthenticationEventArgs.cs
- RegexReplacement.cs
- Thickness.cs
- NotifyIcon.cs
- HttpServerUtilityBase.cs
- BitmapInitialize.cs
- CheckBoxRenderer.cs
- DeclarationUpdate.cs
- SecurityRuntime.cs
- AsymmetricSignatureDeformatter.cs
- DataGridViewCellLinkedList.cs
- HtmlLinkAdapter.cs
- DurationConverter.cs
- SemaphoreFullException.cs
- SnapLine.cs
- AnnotationStore.cs
- MsmqReceiveHelper.cs
- KnownTypeDataContractResolver.cs
- Symbol.cs
- SmiEventStream.cs
- CombinedGeometry.cs
- ZipIOFileItemStream.cs
- ToolStripMenuItem.cs
- Duration.cs
- BinaryMethodMessage.cs
- SoapAttributes.cs
- SocketInformation.cs
- SiteMembershipCondition.cs
- CodeStatementCollection.cs
- ListChangedEventArgs.cs
- PersonalizablePropertyEntry.cs
- AddingNewEventArgs.cs
- EncodedStreamFactory.cs
- SizeF.cs
- ToolStripSeparator.cs
- BindingCompleteEventArgs.cs
- HttpCacheVaryByContentEncodings.cs
- figurelengthconverter.cs
- VerificationAttribute.cs
- InstancePersistenceEvent.cs
- Part.cs
- DataViewManagerListItemTypeDescriptor.cs
- MasterPageParser.cs
- CustomAttributeFormatException.cs
- TransactionsSectionGroup.cs
- XmlReturnReader.cs
- GenerateHelper.cs
- QueryOperationResponseOfT.cs
- ExplicitDiscriminatorMap.cs
- sitestring.cs
- UInt16Storage.cs
- DefaultAsyncDataDispatcher.cs
- Scene3D.cs
- EventLogPermissionEntry.cs
- ContextDataSourceView.cs
- CacheEntry.cs
- WebUtil.cs
- SecurityAccessDeniedException.cs
- MenuItem.cs
- TypeDescriptor.cs
- SafeSecurityHandles.cs
- ProvideValueServiceProvider.cs
- SrgsText.cs
- Light.cs
- Int64AnimationBase.cs
- StickyNoteContentControl.cs
- VisualBrush.cs
- StringStorage.cs
- SupportsEventValidationAttribute.cs
- MonthChangedEventArgs.cs
- XmlSchemaException.cs