Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Abstractions / HttpServerUtilityBase.cs / 1305376 / HttpServerUtilityBase.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Collections.Specialized; using System.Diagnostics.CodeAnalysis; using System.IO; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public abstract class HttpServerUtilityBase { public virtual string MachineName { get { throw new NotImplementedException(); } } public virtual int ScriptTimeout { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual void ClearError() { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1706:ShortAcronymsShouldBeUppercase", MessageId="ID")] [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID")] public virtual object CreateObject(string progID) { throw new NotImplementedException(); } public virtual object CreateObject(Type type) { throw new NotImplementedException(); } public virtual object CreateObjectFromClsid(string clsid) { throw new NotImplementedException(); } public virtual void Execute(string path) { throw new NotImplementedException(); } public virtual void Execute(string path, TextWriter writer) { throw new NotImplementedException(); } public virtual void Execute(string path, bool preserveForm) { throw new NotImplementedException(); } public virtual void Execute(string path, TextWriter writer, bool preserveForm) { throw new NotImplementedException(); } public virtual void Execute(IHttpHandler handler, TextWriter writer, bool preserveForm) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification = "Matches HttpServerUtility class")] public virtual Exception GetLastError() { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual string HtmlDecode(string s) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual void HtmlDecode(string s, TextWriter output) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual string HtmlEncode(string s) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual void HtmlEncode(string s, TextWriter output) { throw new NotImplementedException(); } public virtual string MapPath(string path) { throw new NotImplementedException(); } public virtual void Transfer(string path, bool preserveForm) { throw new NotImplementedException(); } public virtual void Transfer(string path) { throw new NotImplementedException(); } public virtual void Transfer(IHttpHandler handler, bool preserveForm) { throw new NotImplementedException(); } public virtual void TransferRequest(string path) { throw new NotImplementedException(); } public virtual void TransferRequest(string path, bool preserveForm) { throw new NotImplementedException(); } public virtual void TransferRequest(string path, bool preserveForm, string method, NameValueCollection headers) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] [SuppressMessage("Microsoft.Design", "CA1055:UriReturnValuesShouldNotBeStrings", Justification = "Matches HttpServerUtility class")] public virtual string UrlDecode(string s) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual void UrlDecode(string s, TextWriter output) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] [SuppressMessage("Microsoft.Design", "CA1055:UriReturnValuesShouldNotBeStrings", Justification = "Matches HttpServerUtility class")] public virtual string UrlEncode(string s) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual void UrlEncode(string s, TextWriter output) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1055:UriReturnValuesShouldNotBeStrings", Justification = "Matches HttpServerUtility class")] [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual string UrlPathEncode(string s) { throw new NotImplementedException(); } public virtual byte[] UrlTokenDecode(string input) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1055:UriReturnValuesShouldNotBeStrings", Justification = "Matches HttpServerUtility class")] public virtual string UrlTokenEncode(byte[] input) { throw new NotImplementedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web { using System.Collections.Specialized; using System.Diagnostics.CodeAnalysis; using System.IO; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.Web.Abstractions, Version=3.5.0.0, Culture=Neutral, PublicKeyToken=31bf3856ad364e35")] public abstract class HttpServerUtilityBase { public virtual string MachineName { get { throw new NotImplementedException(); } } public virtual int ScriptTimeout { get { throw new NotImplementedException(); } set { throw new NotImplementedException(); } } public virtual void ClearError() { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1706:ShortAcronymsShouldBeUppercase", MessageId="ID")] [SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "ID")] public virtual object CreateObject(string progID) { throw new NotImplementedException(); } public virtual object CreateObject(Type type) { throw new NotImplementedException(); } public virtual object CreateObjectFromClsid(string clsid) { throw new NotImplementedException(); } public virtual void Execute(string path) { throw new NotImplementedException(); } public virtual void Execute(string path, TextWriter writer) { throw new NotImplementedException(); } public virtual void Execute(string path, bool preserveForm) { throw new NotImplementedException(); } public virtual void Execute(string path, TextWriter writer, bool preserveForm) { throw new NotImplementedException(); } public virtual void Execute(IHttpHandler handler, TextWriter writer, bool preserveForm) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1024:UsePropertiesWhereAppropriate", Justification = "Matches HttpServerUtility class")] public virtual Exception GetLastError() { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual string HtmlDecode(string s) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual void HtmlDecode(string s, TextWriter output) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual string HtmlEncode(string s) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual void HtmlEncode(string s, TextWriter output) { throw new NotImplementedException(); } public virtual string MapPath(string path) { throw new NotImplementedException(); } public virtual void Transfer(string path, bool preserveForm) { throw new NotImplementedException(); } public virtual void Transfer(string path) { throw new NotImplementedException(); } public virtual void Transfer(IHttpHandler handler, bool preserveForm) { throw new NotImplementedException(); } public virtual void TransferRequest(string path) { throw new NotImplementedException(); } public virtual void TransferRequest(string path, bool preserveForm) { throw new NotImplementedException(); } public virtual void TransferRequest(string path, bool preserveForm, string method, NameValueCollection headers) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] [SuppressMessage("Microsoft.Design", "CA1055:UriReturnValuesShouldNotBeStrings", Justification = "Matches HttpServerUtility class")] public virtual string UrlDecode(string s) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual void UrlDecode(string s, TextWriter output) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] [SuppressMessage("Microsoft.Design", "CA1055:UriReturnValuesShouldNotBeStrings", Justification = "Matches HttpServerUtility class")] public virtual string UrlEncode(string s) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual void UrlEncode(string s, TextWriter output) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1055:UriReturnValuesShouldNotBeStrings", Justification = "Matches HttpServerUtility class")] [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", Justification = "Matches HttpServerUtility class")] public virtual string UrlPathEncode(string s) { throw new NotImplementedException(); } public virtual byte[] UrlTokenDecode(string input) { throw new NotImplementedException(); } [SuppressMessage("Microsoft.Design", "CA1055:UriReturnValuesShouldNotBeStrings", Justification = "Matches HttpServerUtility class")] public virtual string UrlTokenEncode(byte[] input) { throw new NotImplementedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
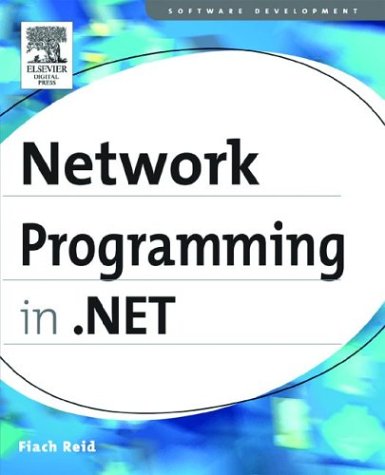
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Byte.cs
- StylusPointProperties.cs
- XmlQuerySequence.cs
- ServerIdentity.cs
- _CookieModule.cs
- FontWeightConverter.cs
- Int64Converter.cs
- RepeaterItemEventArgs.cs
- DataGridHeaderBorder.cs
- SafeNativeMethods.cs
- DataGridViewRowPostPaintEventArgs.cs
- ClickablePoint.cs
- Journaling.cs
- XmlBoundElement.cs
- InteropAutomationProvider.cs
- EventHandlingScope.cs
- ListViewContainer.cs
- Viewport3DAutomationPeer.cs
- IssuanceLicense.cs
- WebExceptionStatus.cs
- ComponentDispatcher.cs
- DispatcherExceptionFilterEventArgs.cs
- CodeFieldReferenceExpression.cs
- OdbcConnectionString.cs
- hebrewshape.cs
- SqlLiftWhereClauses.cs
- ProtocolsConfigurationHandler.cs
- PackageRelationshipCollection.cs
- ExitEventArgs.cs
- XhtmlBasicTextViewAdapter.cs
- RuleInfoComparer.cs
- ActivationArguments.cs
- SchemaElementDecl.cs
- COM2Properties.cs
- EngineSite.cs
- DesignerMetadata.cs
- MatrixAnimationUsingKeyFrames.cs
- CryptoConfig.cs
- BuildProviderUtils.cs
- TabPage.cs
- ExpressionBuilderContext.cs
- FilteredXmlReader.cs
- ToolStripDropDownClosingEventArgs.cs
- DuplicateWaitObjectException.cs
- ExitEventArgs.cs
- ItemCollection.cs
- Vector3D.cs
- TextElementEnumerator.cs
- ConcurrentBag.cs
- SerializationHelper.cs
- OperationValidationEventArgs.cs
- DrawingVisualDrawingContext.cs
- BaseResourcesBuildProvider.cs
- FieldTemplateUserControl.cs
- Subtract.cs
- NamespaceMapping.cs
- GenerateTemporaryTargetAssembly.cs
- PeerTransportSecuritySettings.cs
- XmlBindingWorker.cs
- DataRelationCollection.cs
- Pens.cs
- XmlIgnoreAttribute.cs
- HttpWebRequestElement.cs
- CellTreeNode.cs
- COM2AboutBoxPropertyDescriptor.cs
- DirtyTextRange.cs
- EventMappingSettings.cs
- NativeMethods.cs
- DeleteWorkflowOwnerCommand.cs
- WebBrowserNavigatedEventHandler.cs
- CompilerError.cs
- BaseTemplateCodeDomTreeGenerator.cs
- NamespaceList.cs
- TargetControlTypeAttribute.cs
- DataServiceResponse.cs
- RadioButtonStandardAdapter.cs
- SimpleHandlerFactory.cs
- HtmlInputText.cs
- PhysicalOps.cs
- MetadataElement.cs
- OleDbRowUpdatingEvent.cs
- WCFServiceClientProxyGenerator.cs
- DataViewListener.cs
- CapabilitiesState.cs
- DataColumnMappingCollection.cs
- EnumerableRowCollectionExtensions.cs
- RectangleF.cs
- _Connection.cs
- SingleResultAttribute.cs
- RequestQueue.cs
- EntityTemplateUserControl.cs
- VirtualDirectoryMappingCollection.cs
- NetNamedPipeBinding.cs
- BitmapSource.cs
- COM2EnumConverter.cs
- ContractMapping.cs
- CreateUserWizardDesigner.cs
- StorageEntityTypeMapping.cs
- GroupBoxDesigner.cs
- XmlSerializerVersionAttribute.cs