Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / MS / Internal / IO / Packaging / ContainerActivationHelper.cs / 1 / ContainerActivationHelper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Helper functions to be used for activating/hosting a container // // History: // 07/19/04: younggk Refacted out from Application.cs and DocobjHost.cs // 07/19/04: younggk Added side-by-side support for Metro Package // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.IO; using System.IO.Packaging; using System.Reflection; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Navigation; using System.Windows.Markup; using MS.Internal.IO.Packaging.CompoundFile; using MS.Internal.Utility; using MS.Internal.PresentationFramework; using MS.Utility; // MimeType using MS.Internal.Documents; // DocumentApplication namespace MS.Internal.IO.Packaging { ////// Helper class to be used for activating or hosting a container /// static internal class ContainerActivationHelper { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- // NONE //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- // NONE //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ // NONE //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // NONE //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Load an app from a container /// ////// ////// Critical: This sets the container path, which is critical, and handles other critical data, such as the /// uri, rootStorage, container, and ServiceProvider /// [SecurityCritical] static internal Application LoadFromContainer(Uri containerUri, #if CF_Envelope_Activation_Enabled StorageRoot rootStorage, #endif Package container, IServiceProvider isp) { #if CF_Envelope_Activation_Enabled CheckAndAssertForContainer(rootStorage, container); #else CheckAndAssertForContainer(container); #endif #if CF_Envelope_Activation_Enabled if (rootStorage != null) { // // This is RM Initialization event. for Compound File case DataSpaceManager dataSpaceManager = rootStorage.GetDataSpaceManager(); dataSpaceManager.OnTransformInitialization += new DataSpaceManager.TransformInitializeEventHandler(Application.HandleCompoundFileRmTransformInitConsumption); } BindUriHelper.Container = rootStorage; #endif // CF_Envelope_Activation_Enabled #if CF_Envelope_Activation_Enabled if (rootStorage != null) { // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope // this includes the container and is in the ssres scheme // BindUriHelper.BaseUri = CompoundFileUri.CreateFromAbsoluteUri(containerUri); } else { #endif Debug.Assert(container != null); // Case sensitive is fine here because Uri.Scheme contract is to return in lower case only. if (!SecurityHelper.AreStringTypesEqual(containerUri.Scheme, PackUriHelper.UriSchemePack)) { // this includes the container and is in the pack scheme BindUriHelper.BaseUri = PackUriHelper.Create(containerUri); } #if CF_Envelope_Activation_Enabled } return GetContainerEntryPoint(rootStorage, container, isp); #else return GetContainerEntryPoint(container, isp); #endif } #endregion Internal Methods //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods #if CF_Envelope_Activation_Enabled // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope ////// Get the application from a container /// ////// /// ToDo (younggk): PS# 25616 Remove the parameter, rootStorage, once we make full transition to Metro ////// Critical because it sets the IServiceProvider (critical data) based on input parameters. /// [SecurityCritical] static private Application GetContainerEntryPoint(StorageRoot rootStorage, Package container, IServiceProvider isp) { if (container != null) { return GetContainerEntryPoint(container, isp); } if (rootStorage != null) { return GetContainerEntryPoint(rootStorage, isp); } return null; } ////// Get the application from a container (CF) /// ////// /// ToDo (younggk): PS# 25616 Remove this method once we make full transition to Metro ////// Critical because it sets the apps IServiceProvider (critical data) based on an input parameter /// [SecurityCritical] static private Application GetContainerEntryPoint(StorageRoot root, IServiceProvider isp) { StorageInfo rootStorage = (StorageInfo) root; StreamInfo startupPageStreamInfo = new StreamInfo(rootStorage, StartupPageStreamName); if (!startupPageStreamInfo.Exists) { throw new NotSupportedException(SR.Get(SRID.UnknownContainerFormat)); } string startupPage = GetStringFromStreamInfo(startupPageStreamInfo); if (startupPage == null || startupPage.Length == 0) { throw new NotSupportedException(SR.Get(SRID.UnknownContainerFormat)); } Application appObject = new Application(); appObject.StartupUri = new Uri(startupPage, UriKind.RelativeOrAbsolute); if (isp != null) { appObject.ServiceProvider = isp; } return appObject; } #endif ////// Get the application from a container /// ////// /// ToDo (younggk): PS# 25616 Make this one internal and remove other overloads /// once we make full transition to Metro ////// Critical because it sets the apps IServiceProvider (critical data) based on an input parameter /// [SecurityCritical] static private Application GetContainerEntryPoint(Package container, IServiceProvider isp) { // check to make sure that StartingPart is xaml PackagePart startingPart = GetReachPackageStartingPart(container); // the only supported mime types in a container if ((startingPart == null) || (!startingPart.ValidatedContentType.AreTypeAndSubTypeEqual( MimeTypeMapper.FixedDocumentSequenceMime ))) { throw new NotSupportedException(SR.Get(SRID.UnknownContainerFormat)); } // load DocumentApplication DocumentApplication appObject = new DocumentApplication(); // assign StartPart as Startup Uri appObject.StartupUri = startingPart.Uri; if (isp != null) { appObject.ServiceProvider = isp; } return appObject; } #if CF_Envelope_Activation_Enabled // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope ////// Get a string from the stream /// ////// /// ToDo (younggk): PS# 25616 Remove this method once we make full transition to Metro private static string GetStringFromStreamInfo(StreamInfo streamInfo) { string streamString; using(Stream stream = streamInfo.Open(FileMode.Open, FileAccess.Read)) { using(BinaryReader binaryReader = new BinaryReader(stream)) { streamString = binaryReader.ReadString(); } } return streamString; } ////// Get the Uri of the stream given base Uri /// ////// /// ToDo (younggk): PS# 25616 Remove this method once we make full transition to Metro ////// Critical as it access the base uri through GetResolvedUri /// TreatAsSafe since it demands unrestricted permission /// [SecurityCritical, SecurityTreatAsSafe] private static Uri GetUriForStream(Uri baseBpu, String streamFullName) { SecurityHelper.DemandUnmanagedCode(); Debug.Assert(streamFullName != null && streamFullName.Length > 0); if (streamFullName == null || streamFullName.Length < 1) return null; // // Create Uri for the given stream // Uri bpu = new Uri(streamFullName.Replace('\\', '/'), UriKind.RelativeOrAbsolute); if (bpu.IsAbsoluteUri == false) bpu = BindUriHelper.GetResolvedUri(CompoundFileUri.CreateFromAbsoluteUri(baseBpu), bpu); return bpu; } #endif static private void CheckAndAssertForContainer( #if CF_Envelope_Activation_Enabled StorageRoot root, #endif Package container) { #if CF_Envelope_Activation_Enabled // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope Debug.Assert((root != null && container == null) || (root == null && container != null), "Only one of CF-specific or Metro container should be opened"); #else Debug.Assert(container != null, "No container is opened"); #endif } ////// Gets StartingPart of the Reach Package. /// ///PackagePart static private PackagePart GetReachPackageStartingPart(Package package) { Debug.Assert(package != null, "package cannot be null"); PackageRelationship startingPartRelationship = null; foreach (PackageRelationship rel in package.GetRelationshipsByType(ReachPackageStartingPartRelationshipType)) { if (startingPartRelationship != null) { throw new InvalidDataException(SR.Get(SRID.MoreThanOneStartingParts)); } startingPartRelationship = rel; } if (startingPartRelationship != null) { Uri startPartUri = PackUriHelper.ResolvePartUri(startingPartRelationship.SourceUri, startingPartRelationship.TargetUri); if(package.PartExists(startPartUri)) return (package.GetPart(startPartUri)); else return null; } return null; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Members //we use this dummy URI to resolve relative URIs treating the container as the authority. private static readonly Uri _defaultUri = new Uri("http://defaultcontainer/"); private static readonly string ReachPackageStartingPartRelationshipType = "http://schemas.microsoft.com/xps/2005/06/fixedrepresentation"; #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Helper functions to be used for activating/hosting a container // // History: // 07/19/04: younggk Refacted out from Application.cs and DocobjHost.cs // 07/19/04: younggk Added side-by-side support for Metro Package // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.IO; using System.IO.Packaging; using System.Reflection; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Navigation; using System.Windows.Markup; using MS.Internal.IO.Packaging.CompoundFile; using MS.Internal.Utility; using MS.Internal.PresentationFramework; using MS.Utility; // MimeType using MS.Internal.Documents; // DocumentApplication namespace MS.Internal.IO.Packaging { ////// Helper class to be used for activating or hosting a container /// static internal class ContainerActivationHelper { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- // NONE //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- // NONE //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ // NONE //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // NONE //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Load an app from a container /// ////// ////// Critical: This sets the container path, which is critical, and handles other critical data, such as the /// uri, rootStorage, container, and ServiceProvider /// [SecurityCritical] static internal Application LoadFromContainer(Uri containerUri, #if CF_Envelope_Activation_Enabled StorageRoot rootStorage, #endif Package container, IServiceProvider isp) { #if CF_Envelope_Activation_Enabled CheckAndAssertForContainer(rootStorage, container); #else CheckAndAssertForContainer(container); #endif #if CF_Envelope_Activation_Enabled if (rootStorage != null) { // // This is RM Initialization event. for Compound File case DataSpaceManager dataSpaceManager = rootStorage.GetDataSpaceManager(); dataSpaceManager.OnTransformInitialization += new DataSpaceManager.TransformInitializeEventHandler(Application.HandleCompoundFileRmTransformInitConsumption); } BindUriHelper.Container = rootStorage; #endif // CF_Envelope_Activation_Enabled #if CF_Envelope_Activation_Enabled if (rootStorage != null) { // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope // this includes the container and is in the ssres scheme // BindUriHelper.BaseUri = CompoundFileUri.CreateFromAbsoluteUri(containerUri); } else { #endif Debug.Assert(container != null); // Case sensitive is fine here because Uri.Scheme contract is to return in lower case only. if (!SecurityHelper.AreStringTypesEqual(containerUri.Scheme, PackUriHelper.UriSchemePack)) { // this includes the container and is in the pack scheme BindUriHelper.BaseUri = PackUriHelper.Create(containerUri); } #if CF_Envelope_Activation_Enabled } return GetContainerEntryPoint(rootStorage, container, isp); #else return GetContainerEntryPoint(container, isp); #endif } #endregion Internal Methods //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods #if CF_Envelope_Activation_Enabled // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope ////// Get the application from a container /// ////// /// ToDo (younggk): PS# 25616 Remove the parameter, rootStorage, once we make full transition to Metro ////// Critical because it sets the IServiceProvider (critical data) based on input parameters. /// [SecurityCritical] static private Application GetContainerEntryPoint(StorageRoot rootStorage, Package container, IServiceProvider isp) { if (container != null) { return GetContainerEntryPoint(container, isp); } if (rootStorage != null) { return GetContainerEntryPoint(rootStorage, isp); } return null; } ////// Get the application from a container (CF) /// ////// /// ToDo (younggk): PS# 25616 Remove this method once we make full transition to Metro ////// Critical because it sets the apps IServiceProvider (critical data) based on an input parameter /// [SecurityCritical] static private Application GetContainerEntryPoint(StorageRoot root, IServiceProvider isp) { StorageInfo rootStorage = (StorageInfo) root; StreamInfo startupPageStreamInfo = new StreamInfo(rootStorage, StartupPageStreamName); if (!startupPageStreamInfo.Exists) { throw new NotSupportedException(SR.Get(SRID.UnknownContainerFormat)); } string startupPage = GetStringFromStreamInfo(startupPageStreamInfo); if (startupPage == null || startupPage.Length == 0) { throw new NotSupportedException(SR.Get(SRID.UnknownContainerFormat)); } Application appObject = new Application(); appObject.StartupUri = new Uri(startupPage, UriKind.RelativeOrAbsolute); if (isp != null) { appObject.ServiceProvider = isp; } return appObject; } #endif ////// Get the application from a container /// ////// /// ToDo (younggk): PS# 25616 Make this one internal and remove other overloads /// once we make full transition to Metro ////// Critical because it sets the apps IServiceProvider (critical data) based on an input parameter /// [SecurityCritical] static private Application GetContainerEntryPoint(Package container, IServiceProvider isp) { // check to make sure that StartingPart is xaml PackagePart startingPart = GetReachPackageStartingPart(container); // the only supported mime types in a container if ((startingPart == null) || (!startingPart.ValidatedContentType.AreTypeAndSubTypeEqual( MimeTypeMapper.FixedDocumentSequenceMime ))) { throw new NotSupportedException(SR.Get(SRID.UnknownContainerFormat)); } // load DocumentApplication DocumentApplication appObject = new DocumentApplication(); // assign StartPart as Startup Uri appObject.StartupUri = startingPart.Uri; if (isp != null) { appObject.ServiceProvider = isp; } return appObject; } #if CF_Envelope_Activation_Enabled // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope ////// Get a string from the stream /// ////// /// ToDo (younggk): PS# 25616 Remove this method once we make full transition to Metro private static string GetStringFromStreamInfo(StreamInfo streamInfo) { string streamString; using(Stream stream = streamInfo.Open(FileMode.Open, FileAccess.Read)) { using(BinaryReader binaryReader = new BinaryReader(stream)) { streamString = binaryReader.ReadString(); } } return streamString; } ////// Get the Uri of the stream given base Uri /// ////// /// ToDo (younggk): PS# 25616 Remove this method once we make full transition to Metro ////// Critical as it access the base uri through GetResolvedUri /// TreatAsSafe since it demands unrestricted permission /// [SecurityCritical, SecurityTreatAsSafe] private static Uri GetUriForStream(Uri baseBpu, String streamFullName) { SecurityHelper.DemandUnmanagedCode(); Debug.Assert(streamFullName != null && streamFullName.Length > 0); if (streamFullName == null || streamFullName.Length < 1) return null; // // Create Uri for the given stream // Uri bpu = new Uri(streamFullName.Replace('\\', '/'), UriKind.RelativeOrAbsolute); if (bpu.IsAbsoluteUri == false) bpu = BindUriHelper.GetResolvedUri(CompoundFileUri.CreateFromAbsoluteUri(baseBpu), bpu); return bpu; } #endif static private void CheckAndAssertForContainer( #if CF_Envelope_Activation_Enabled StorageRoot root, #endif Package container) { #if CF_Envelope_Activation_Enabled // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope // ToDo (younggk: CF Envelope): We need this when we implement CF Envelope Debug.Assert((root != null && container == null) || (root == null && container != null), "Only one of CF-specific or Metro container should be opened"); #else Debug.Assert(container != null, "No container is opened"); #endif } ////// Gets StartingPart of the Reach Package. /// ///PackagePart static private PackagePart GetReachPackageStartingPart(Package package) { Debug.Assert(package != null, "package cannot be null"); PackageRelationship startingPartRelationship = null; foreach (PackageRelationship rel in package.GetRelationshipsByType(ReachPackageStartingPartRelationshipType)) { if (startingPartRelationship != null) { throw new InvalidDataException(SR.Get(SRID.MoreThanOneStartingParts)); } startingPartRelationship = rel; } if (startingPartRelationship != null) { Uri startPartUri = PackUriHelper.ResolvePartUri(startingPartRelationship.SourceUri, startingPartRelationship.TargetUri); if(package.PartExists(startPartUri)) return (package.GetPart(startPartUri)); else return null; } return null; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Members //we use this dummy URI to resolve relative URIs treating the container as the authority. private static readonly Uri _defaultUri = new Uri("http://defaultcontainer/"); private static readonly string ReachPackageStartingPartRelationshipType = "http://schemas.microsoft.com/xps/2005/06/fixedrepresentation"; #endregion Private Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
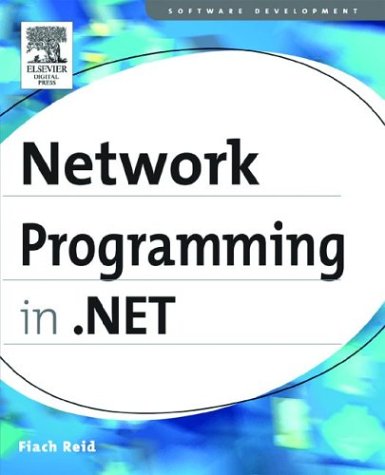
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DictionaryEntry.cs
- AmbientLight.cs
- SecureUICommand.cs
- AlphabeticalEnumConverter.cs
- SqlConnectionString.cs
- CodeDOMProvider.cs
- OpenFileDialog.cs
- InkSerializer.cs
- ToolStripRendererSwitcher.cs
- MustUnderstandSoapException.cs
- COM2PropertyPageUITypeConverter.cs
- ParserContext.cs
- ControlBuilderAttribute.cs
- RsaSecurityTokenAuthenticator.cs
- StructuredTypeEmitter.cs
- PropertyChangedEventArgs.cs
- SmtpException.cs
- ObjectConverter.cs
- HttpDebugHandler.cs
- EntityProviderFactory.cs
- ZipIOLocalFileHeader.cs
- SizeAnimation.cs
- TraceListeners.cs
- SchemaCollectionCompiler.cs
- ToolStripDropTargetManager.cs
- DataTableExtensions.cs
- _DynamicWinsockMethods.cs
- CommonGetThemePartSize.cs
- JpegBitmapDecoder.cs
- CodeDOMUtility.cs
- DataGridViewRowCollection.cs
- TextParentUndoUnit.cs
- ObjectRef.cs
- UInt64Converter.cs
- WhitespaceRuleLookup.cs
- PropertyFilterAttribute.cs
- ResourceContainer.cs
- PaintValueEventArgs.cs
- IISMapPath.cs
- CloseCryptoHandleRequest.cs
- UpDownBase.cs
- NonBatchDirectoryCompiler.cs
- GraphicsPath.cs
- CodeSubDirectoriesCollection.cs
- ObjectStateEntryDbDataRecord.cs
- HtmlInputControl.cs
- PackagingUtilities.cs
- LocatorPartList.cs
- httpserverutility.cs
- TextParentUndoUnit.cs
- Sql8ExpressionRewriter.cs
- StringBlob.cs
- UTF8Encoding.cs
- TableItemPatternIdentifiers.cs
- MailBnfHelper.cs
- AnonymousIdentificationModule.cs
- DoubleStorage.cs
- WebPartZoneBase.cs
- DataListItemCollection.cs
- DataGridViewColumnCollection.cs
- MenuAdapter.cs
- RightsManagementManager.cs
- ReadOnlyHierarchicalDataSourceView.cs
- WebPartZone.cs
- ProcessInputEventArgs.cs
- securitycriticaldata.cs
- RuntimeConfigurationRecord.cs
- TextViewBase.cs
- DefaultMemberAttribute.cs
- SystemIPGlobalProperties.cs
- WorkflowViewManager.cs
- XmlBoundElement.cs
- XmlTypeAttribute.cs
- InputScopeManager.cs
- UriSection.cs
- XmlCharCheckingReader.cs
- updatecommandorderer.cs
- HandleCollector.cs
- ModelItem.cs
- ErrorFormatterPage.cs
- MonthCalendar.cs
- Line.cs
- DataBindingValueUIHandler.cs
- PropertyTab.cs
- SoapRpcMethodAttribute.cs
- ImportCatalogPart.cs
- TreeNodeConverter.cs
- ChooseAction.cs
- NetworkInformationException.cs
- MultiByteCodec.cs
- COMException.cs
- PointConverter.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- FontFaceLayoutInfo.cs
- StylusPlugin.cs
- SrgsElementFactoryCompiler.cs
- httpserverutility.cs
- SmiGettersStream.cs
- EmissiveMaterial.cs
- CommandBinding.cs