Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntityDesign / Design / System / Data / EntityModel / Emitters / StructuredTypeEmitter.cs / 1 / StructuredTypeEmitter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.CodeDom; using System.Data; using System.Data.EntityModel.SchemaObjectModel; using Som = System.Data.EntityModel.SchemaObjectModel; using System.Data.Entity.Design; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Reflection; using System.Data.Objects.DataClasses; using System.Data.Entity.Design.Common; using System.Data.Entity.Design.SsdlGenerator; namespace System.Data.EntityModel.Emitters { ////// Summary description for StructuredTypeEmitter. /// internal abstract class StructuredTypeEmitter : SchemaTypeEmitter { #region Public Methods private bool _usingStandardBaseClass = true; ////// /// ///public override CodeTypeDeclarationCollection EmitApiClass() { Validate(); // emitter-specific validation CodeTypeReference baseType = this.GetBaseType(); // raise the TypeGenerated event TypeGeneratedEventArgs eventArgs = new TypeGeneratedEventArgs(Item, baseType); this.Generator.RaiseTypeGeneratedEvent(eventArgs); // public [abstract] partial class ClassName CodeTypeDeclaration typeDecl = new CodeTypeDeclaration(Item.Name); typeDecl.IsPartial = true; typeDecl.TypeAttributes = System.Reflection.TypeAttributes.Class; if (Item.Abstract) { typeDecl.TypeAttributes |= System.Reflection.TypeAttributes.Abstract; } SetTypeVisibility(typeDecl); EmitTypeAttributes(Item.Name, typeDecl, eventArgs.AdditionalAttributes); // : baseclass AssignBaseType(typeDecl, baseType, eventArgs.BaseType); AddInterfaces(Item.Name, typeDecl, eventArgs.AdditionalInterfaces); CommentEmitter.EmitSummaryComments(Item, typeDecl.Comments); // Since abstract types cannot be instantiated, skip the factory method for abstract types if ( (typeDecl.TypeAttributes & System.Reflection.TypeAttributes.Abstract) == 0) EmitFactoryMethod(typeDecl); EmitProperties(typeDecl); // additional members, if provided by the event subscriber this.AddMembers(Item.Name, typeDecl, eventArgs.AdditionalMembers); CodeTypeDeclarationCollection typeDecls = new CodeTypeDeclarationCollection(); typeDecls.Add(typeDecl); return typeDecls; } #endregion #region Protected Methods /// /// /// ///protected virtual CodeTypeReference GetBaseType() { if (Item.BaseType == null) return null; return Generator.GetLeastPossibleQualifiedTypeReference(Item.BaseType); } /// /// /// /// /// protected StructuredTypeEmitter(ClientApiGenerator generator, StructuralType structuralType) : base(generator, structuralType) { } ////// /// /// protected override void EmitTypeAttributes(CodeTypeDeclaration typeDecl) { Generator.AttributeEmitter.EmitTypeAttributes(this, typeDecl); base.EmitTypeAttributes(typeDecl); } ////// /// /// protected virtual void EmitProperties(CodeTypeDeclaration typeDecl) { foreach (EdmProperty property in Item.GetDeclaredOnlyMembers()) { PropertyEmitter propertyEmitter = new PropertyEmitter(Generator, property, _usingStandardBaseClass); propertyEmitter.Emit(typeDecl); } } protected abstract ReadOnlyMetadataCollection GetProperties(); /// /// Emit static factory method which creates an instance of the class and initializes /// non-nullable properties (taken as arguments) /// /// protected virtual void EmitFactoryMethod(CodeTypeDeclaration typeDecl) { // build list of non-nullable properties ReadOnlyMetadataCollectionproperties = GetProperties(); List parameters = new List (properties.Count); foreach (EdmProperty property in properties) { bool include = IncludeFieldInFactoryMethod(property); if (include) { parameters.Add(property); } } // if there are no parameters, we don't emit anything (1 is for the null element) // nor do we emit everything if this is the Ref propertied ctor and the parameter list is the same as the many parametered ctor if (parameters.Count < 1) { return; } CodeMemberMethod method = new CodeMemberMethod(); CodeTypeReference typeRef = TypeReference.FromString(Item.Name); UniqueIdentifierService uniqueIdentifierService = new UniqueIdentifierService(Generator.IsLanguageCaseSensitive, name => Utils.FixParameterName(name)); string instanceName = uniqueIdentifierService.AdjustIdentifier(Item.Name); // public static Class CreateClass(...) method.Attributes = MemberAttributes.Static|MemberAttributes.Public; method.Name = "Create" + Item.Name; if (NavigationPropertyEmitter.IsNameAlreadyAMemberName(Item, method.Name, Generator.LanguageAppropriateStringComparer)) { Generator.AddError(Strings.GeneratedFactoryMethodNameConflict(method.Name, Item.Name), ModelBuilderErrorCode.GeneratedFactoryMethodNameConflict, EdmSchemaErrorSeverity.Error, Item.FullName); } method.ReturnType = typeRef; // output method summary comments CommentEmitter.EmitSummaryComments(Strings.FactoryMethodSummaryComment(Item.Name), method.Comments); // Class class = new Class(); CodeVariableDeclarationStatement createNewInstance = new CodeVariableDeclarationStatement( typeRef, instanceName, new CodeObjectCreateExpression(typeRef)); method.Statements.Add(createNewInstance); CodeVariableReferenceExpression instanceRef = new CodeVariableReferenceExpression(instanceName); // iterate over the properties figuring out which need included in the factory method foreach (EdmProperty property in parameters) { // CreateClass( ... , propType propName ...) PropertyEmitter propertyEmitter = new PropertyEmitter(Generator, property, UsingStandardBaseClass); CodeTypeReference propertyTypeReference = propertyEmitter.PropertyType; String parameterName = uniqueIdentifierService.AdjustIdentifier(propertyEmitter.PropertyName); CodeParameterDeclarationExpression paramDecl = new CodeParameterDeclarationExpression( propertyTypeReference, parameterName); CodeArgumentReferenceExpression paramRef = new CodeArgumentReferenceExpression(paramDecl.Name); method.Parameters.Add(paramDecl); // add comment describing the parameter CommentEmitter.EmitParamComments(paramDecl, Strings.FactoryParamCommentGeneral(propertyEmitter.PropertyName), method.Comments); CodeExpression newPropertyValue; if (TypeSemantics.IsComplexType(propertyEmitter.Item.TypeUsage)) { List complexVerifyParameters = new List (); complexVerifyParameters.Add(paramRef); complexVerifyParameters.Add(new CodePrimitiveExpression(propertyEmitter.PropertyName)); newPropertyValue = new CodeMethodInvokeExpression( PropertyEmitter.CreateEdmStructuralObjectRef(TypeReference), Utils.VerifyComplexObjectIsNotNullName, complexVerifyParameters.ToArray()); } else { newPropertyValue = paramRef; } // Scalar property: // Property = param; // Complex property: // Property = StructuralObject.VerifyComplexObjectIsNotNull(param, propertyName); method.Statements.Add(new CodeAssignStatement(new CodePropertyReferenceExpression(instanceRef, propertyEmitter.PropertyName), newPropertyValue)); } // return class; method.Statements.Add(new CodeMethodReturnStatement(instanceRef)); // actually add the method to the class typeDecl.Members.Add(method); } #endregion #region Protected Properties internal new StructuralType Item { get { return base.Item as StructuralType; } } protected bool UsingStandardBaseClass { get { return _usingStandardBaseClass; } } #endregion #region Private Methods /// /// /// /// ///private bool IncludeFieldInFactoryMethod(EdmProperty property) { if (property.Nullable) { return false; } if (PropertyEmitter.HasDefault(property)) { return false; } if (PropertyEmitter.GetGetterAccessibility(property) != MemberAttributes.Public && PropertyEmitter.GetSetterAccessibility(property) != MemberAttributes.Public) { return false; } return true; } private void AssignBaseType(CodeTypeDeclaration typeDecl, CodeTypeReference baseType, CodeTypeReference eventReturnedBaseType) { if (eventReturnedBaseType != null && !eventReturnedBaseType.Equals(baseType)) { _usingStandardBaseClass = false; typeDecl.BaseTypes.Add(eventReturnedBaseType); } else { if (baseType != null) { typeDecl.BaseTypes.Add(baseType); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.CodeDom; using System.Data; using System.Data.EntityModel.SchemaObjectModel; using Som = System.Data.EntityModel.SchemaObjectModel; using System.Data.Entity.Design; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Reflection; using System.Data.Objects.DataClasses; using System.Data.Entity.Design.Common; using System.Data.Entity.Design.SsdlGenerator; namespace System.Data.EntityModel.Emitters { ////// Summary description for StructuredTypeEmitter. /// internal abstract class StructuredTypeEmitter : SchemaTypeEmitter { #region Public Methods private bool _usingStandardBaseClass = true; ////// /// ///public override CodeTypeDeclarationCollection EmitApiClass() { Validate(); // emitter-specific validation CodeTypeReference baseType = this.GetBaseType(); // raise the TypeGenerated event TypeGeneratedEventArgs eventArgs = new TypeGeneratedEventArgs(Item, baseType); this.Generator.RaiseTypeGeneratedEvent(eventArgs); // public [abstract] partial class ClassName CodeTypeDeclaration typeDecl = new CodeTypeDeclaration(Item.Name); typeDecl.IsPartial = true; typeDecl.TypeAttributes = System.Reflection.TypeAttributes.Class; if (Item.Abstract) { typeDecl.TypeAttributes |= System.Reflection.TypeAttributes.Abstract; } SetTypeVisibility(typeDecl); EmitTypeAttributes(Item.Name, typeDecl, eventArgs.AdditionalAttributes); // : baseclass AssignBaseType(typeDecl, baseType, eventArgs.BaseType); AddInterfaces(Item.Name, typeDecl, eventArgs.AdditionalInterfaces); CommentEmitter.EmitSummaryComments(Item, typeDecl.Comments); // Since abstract types cannot be instantiated, skip the factory method for abstract types if ( (typeDecl.TypeAttributes & System.Reflection.TypeAttributes.Abstract) == 0) EmitFactoryMethod(typeDecl); EmitProperties(typeDecl); // additional members, if provided by the event subscriber this.AddMembers(Item.Name, typeDecl, eventArgs.AdditionalMembers); CodeTypeDeclarationCollection typeDecls = new CodeTypeDeclarationCollection(); typeDecls.Add(typeDecl); return typeDecls; } #endregion #region Protected Methods /// /// /// ///protected virtual CodeTypeReference GetBaseType() { if (Item.BaseType == null) return null; return Generator.GetLeastPossibleQualifiedTypeReference(Item.BaseType); } /// /// /// /// /// protected StructuredTypeEmitter(ClientApiGenerator generator, StructuralType structuralType) : base(generator, structuralType) { } ////// /// /// protected override void EmitTypeAttributes(CodeTypeDeclaration typeDecl) { Generator.AttributeEmitter.EmitTypeAttributes(this, typeDecl); base.EmitTypeAttributes(typeDecl); } ////// /// /// protected virtual void EmitProperties(CodeTypeDeclaration typeDecl) { foreach (EdmProperty property in Item.GetDeclaredOnlyMembers()) { PropertyEmitter propertyEmitter = new PropertyEmitter(Generator, property, _usingStandardBaseClass); propertyEmitter.Emit(typeDecl); } } protected abstract ReadOnlyMetadataCollection GetProperties(); /// /// Emit static factory method which creates an instance of the class and initializes /// non-nullable properties (taken as arguments) /// /// protected virtual void EmitFactoryMethod(CodeTypeDeclaration typeDecl) { // build list of non-nullable properties ReadOnlyMetadataCollectionproperties = GetProperties(); List parameters = new List (properties.Count); foreach (EdmProperty property in properties) { bool include = IncludeFieldInFactoryMethod(property); if (include) { parameters.Add(property); } } // if there are no parameters, we don't emit anything (1 is for the null element) // nor do we emit everything if this is the Ref propertied ctor and the parameter list is the same as the many parametered ctor if (parameters.Count < 1) { return; } CodeMemberMethod method = new CodeMemberMethod(); CodeTypeReference typeRef = TypeReference.FromString(Item.Name); UniqueIdentifierService uniqueIdentifierService = new UniqueIdentifierService(Generator.IsLanguageCaseSensitive, name => Utils.FixParameterName(name)); string instanceName = uniqueIdentifierService.AdjustIdentifier(Item.Name); // public static Class CreateClass(...) method.Attributes = MemberAttributes.Static|MemberAttributes.Public; method.Name = "Create" + Item.Name; if (NavigationPropertyEmitter.IsNameAlreadyAMemberName(Item, method.Name, Generator.LanguageAppropriateStringComparer)) { Generator.AddError(Strings.GeneratedFactoryMethodNameConflict(method.Name, Item.Name), ModelBuilderErrorCode.GeneratedFactoryMethodNameConflict, EdmSchemaErrorSeverity.Error, Item.FullName); } method.ReturnType = typeRef; // output method summary comments CommentEmitter.EmitSummaryComments(Strings.FactoryMethodSummaryComment(Item.Name), method.Comments); // Class class = new Class(); CodeVariableDeclarationStatement createNewInstance = new CodeVariableDeclarationStatement( typeRef, instanceName, new CodeObjectCreateExpression(typeRef)); method.Statements.Add(createNewInstance); CodeVariableReferenceExpression instanceRef = new CodeVariableReferenceExpression(instanceName); // iterate over the properties figuring out which need included in the factory method foreach (EdmProperty property in parameters) { // CreateClass( ... , propType propName ...) PropertyEmitter propertyEmitter = new PropertyEmitter(Generator, property, UsingStandardBaseClass); CodeTypeReference propertyTypeReference = propertyEmitter.PropertyType; String parameterName = uniqueIdentifierService.AdjustIdentifier(propertyEmitter.PropertyName); CodeParameterDeclarationExpression paramDecl = new CodeParameterDeclarationExpression( propertyTypeReference, parameterName); CodeArgumentReferenceExpression paramRef = new CodeArgumentReferenceExpression(paramDecl.Name); method.Parameters.Add(paramDecl); // add comment describing the parameter CommentEmitter.EmitParamComments(paramDecl, Strings.FactoryParamCommentGeneral(propertyEmitter.PropertyName), method.Comments); CodeExpression newPropertyValue; if (TypeSemantics.IsComplexType(propertyEmitter.Item.TypeUsage)) { List complexVerifyParameters = new List (); complexVerifyParameters.Add(paramRef); complexVerifyParameters.Add(new CodePrimitiveExpression(propertyEmitter.PropertyName)); newPropertyValue = new CodeMethodInvokeExpression( PropertyEmitter.CreateEdmStructuralObjectRef(TypeReference), Utils.VerifyComplexObjectIsNotNullName, complexVerifyParameters.ToArray()); } else { newPropertyValue = paramRef; } // Scalar property: // Property = param; // Complex property: // Property = StructuralObject.VerifyComplexObjectIsNotNull(param, propertyName); method.Statements.Add(new CodeAssignStatement(new CodePropertyReferenceExpression(instanceRef, propertyEmitter.PropertyName), newPropertyValue)); } // return class; method.Statements.Add(new CodeMethodReturnStatement(instanceRef)); // actually add the method to the class typeDecl.Members.Add(method); } #endregion #region Protected Properties internal new StructuralType Item { get { return base.Item as StructuralType; } } protected bool UsingStandardBaseClass { get { return _usingStandardBaseClass; } } #endregion #region Private Methods /// /// /// /// ///private bool IncludeFieldInFactoryMethod(EdmProperty property) { if (property.Nullable) { return false; } if (PropertyEmitter.HasDefault(property)) { return false; } if (PropertyEmitter.GetGetterAccessibility(property) != MemberAttributes.Public && PropertyEmitter.GetSetterAccessibility(property) != MemberAttributes.Public) { return false; } return true; } private void AssignBaseType(CodeTypeDeclaration typeDecl, CodeTypeReference baseType, CodeTypeReference eventReturnedBaseType) { if (eventReturnedBaseType != null && !eventReturnedBaseType.Equals(baseType)) { _usingStandardBaseClass = false; typeDecl.BaseTypes.Add(eventReturnedBaseType); } else { if (baseType != null) { typeDecl.BaseTypes.Add(baseType); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
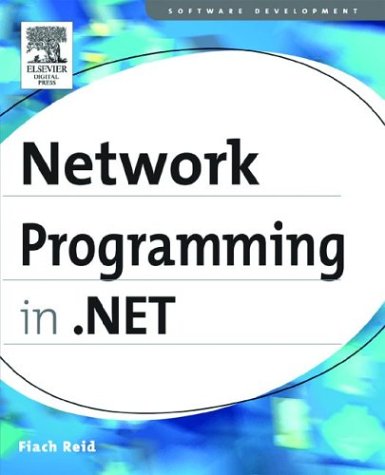
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UserPersonalizationStateInfo.cs
- WebPartConnectionsDisconnectVerb.cs
- ExpressionBinding.cs
- UrlSyndicationContent.cs
- NativeMethods.cs
- KeyInterop.cs
- ValidationHelper.cs
- EventLogEntryCollection.cs
- WindowAutomationPeer.cs
- HtmlTitle.cs
- TimeSpanValidator.cs
- TreeNodeCollection.cs
- WsdlWriter.cs
- MembershipUser.cs
- ViewStateModeByIdAttribute.cs
- AllMembershipCondition.cs
- BitmapCache.cs
- SpellCheck.cs
- TypeUsageBuilder.cs
- Update.cs
- DaylightTime.cs
- ActivityPreviewDesigner.cs
- validationstate.cs
- DataKey.cs
- SystemGatewayIPAddressInformation.cs
- MessagePropertyFilter.cs
- AbstractExpressions.cs
- ContainerTracking.cs
- FlatButtonAppearance.cs
- ShaderEffect.cs
- XPathDocumentNavigator.cs
- OrderedParallelQuery.cs
- MethodRental.cs
- ServiceTimeoutsElement.cs
- GeometryDrawing.cs
- PersistenceException.cs
- HttpGetClientProtocol.cs
- EventDescriptorCollection.cs
- ShapingWorkspace.cs
- PartialCachingControl.cs
- SqlBulkCopyColumnMapping.cs
- GraphicsContext.cs
- SqlDataSourceSelectingEventArgs.cs
- TemplateContainer.cs
- DataControlField.cs
- DataBoundControl.cs
- SelectionRange.cs
- TargetPerspective.cs
- ReplacementText.cs
- DPTypeDescriptorContext.cs
- ObjectViewQueryResultData.cs
- DataGridRowAutomationPeer.cs
- CorrelationQueryBehavior.cs
- GuidTagList.cs
- Item.cs
- TreeWalkHelper.cs
- QueryContext.cs
- TableStyle.cs
- EtwTrackingParticipant.cs
- DrawingContextWalker.cs
- XmlUnspecifiedAttribute.cs
- ContextMenu.cs
- ObjectSecurity.cs
- TextTreeNode.cs
- Set.cs
- XsltQilFactory.cs
- EntityDataSourceSelectingEventArgs.cs
- Listen.cs
- SQLBytesStorage.cs
- SqlWebEventProvider.cs
- x509utils.cs
- PolicyUnit.cs
- Geometry3D.cs
- QueryContinueDragEventArgs.cs
- SubtreeProcessor.cs
- StaticExtension.cs
- MultilineStringConverter.cs
- AccessedThroughPropertyAttribute.cs
- ConfigurationManager.cs
- MediaEntryAttribute.cs
- TreeBuilderXamlTranslator.cs
- CompletionBookmark.cs
- ArgumentNullException.cs
- BitmapEffectCollection.cs
- BufferedReadStream.cs
- BamlResourceDeserializer.cs
- ActivityExecutionFilter.cs
- Localizer.cs
- GridViewItemAutomationPeer.cs
- IDQuery.cs
- Knowncolors.cs
- ContextDataSourceView.cs
- ContextBase.cs
- ImageButton.cs
- ClientScriptManagerWrapper.cs
- SmtpTransport.cs
- HttpRequest.cs
- SevenBitStream.cs
- SecurityNegotiationException.cs
- XmlSchemaDocumentation.cs