Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / ComponentModel / EventDescriptorCollection.cs / 1 / EventDescriptorCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Diagnostics.CodeAnalysis; /* This class has the HostProtectionAttribute. The purpose of this attribute is to enforce host-specific programming model guidelines, not security behavior. Suppress FxCop message - BUT REVISIT IF ADDING NEW SECURITY ATTRIBUTES. */ [assembly: SuppressMessage("Microsoft.Security", "CA2112:SecuredTypesShouldNotExposeFields", Scope="type", Target="System.ComponentModel.EventDescriptorCollection")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.get_IsFixedSize():System.Boolean")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.ICollection.get_SyncRoot():System.Object")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.ICollection.get_IsSynchronized():System.Boolean")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.get_IsReadOnly():System.Boolean")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.Clear():System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IEnumerable.GetEnumerator():System.Collections.IEnumerator")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.ICollection.CopyTo(System.Array,System.Int32):System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.ICollection.get_Count():System.Int32")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.Contains(System.Object):System.Boolean")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.Remove(System.Object):System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.get_Item(System.Int32):System.Object")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.set_Item(System.Int32,System.Object):System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.Add(System.Object):System.Int32")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.IndexOf(System.Object):System.Int32")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.RemoveAt(System.Int32):System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.Insert(System.Int32,System.Object):System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands", Scope="member", Target="System.ComponentModel.EventDescriptorCollection..ctor(System.ComponentModel.EventDescriptor[],System.Boolean)")] [assembly: SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.get_Count():System.Int32")] [assembly: SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.GetEnumerator():System.Collections.IEnumerator")] namespace System.ComponentModel { using System.Runtime.InteropServices; using System.Diagnostics; using Microsoft.Win32; using System.Collections; using System.Globalization; ////// [System.Runtime.InteropServices.ComVisible(true)] [System.Security.Permissions.HostProtection(Synchronization=true)] public class EventDescriptorCollection : ICollection, IList { private EventDescriptor[] events; private string[] namedSort; private IComparer comparer; private bool eventsOwned = true; private bool needSort = false; private int eventCount; private bool readOnly = false; ////// Represents a collection of events. /// ////// An empty AttributeCollection that can used instead of creating a new one with no items. /// public static readonly EventDescriptorCollection Empty = new EventDescriptorCollection(null, true); ////// public EventDescriptorCollection(EventDescriptor[] events) { this.events = events; if (events == null) { this.events = new EventDescriptor[0]; this.eventCount = 0; } else { this.eventCount = this.events.Length; } this.eventsOwned = true; } ////// Initializes a new instance of the ///class. /// /// Initializes a new instance of an event descriptor collection, and allows you to mark the /// collection as read-only so it cannot be modified. /// public EventDescriptorCollection(EventDescriptor[] events, bool readOnly) : this(events) { this.readOnly = readOnly; } private EventDescriptorCollection(EventDescriptor[] events, int eventCount, string[] namedSort, IComparer comparer) { this.eventsOwned = false; if (namedSort != null) { this.namedSort = (string[])namedSort.Clone(); } this.comparer = comparer; this.events = events; this.eventCount = eventCount; this.needSort = true; } ////// public int Count { get { return eventCount; } } ////// Gets the number /// of event descriptors in the collection. /// ////// public virtual EventDescriptor this[int index] { get { if (index >= eventCount) { throw new IndexOutOfRangeException(); } EnsureEventsOwned(); return events[index]; } } ///Gets the event with the specified index /// number. ////// public virtual EventDescriptor this[string name] { get { return Find(name, false); } } ////// Gets the event with the specified name. /// ////// public int Add(EventDescriptor value) { if (readOnly) { throw new NotSupportedException(); } EnsureSize(eventCount + 1); events[eventCount++] = value; return eventCount - 1; } ///[To be supplied.] ////// public void Clear() { if (readOnly) { throw new NotSupportedException(); } eventCount = 0; } ///[To be supplied.] ////// public bool Contains(EventDescriptor value) { return IndexOf(value) >= 0; } ///[To be supplied.] ///void ICollection.CopyTo(Array array, int index) { EnsureEventsOwned(); Array.Copy(events, 0, array, index, Count); } private void EnsureEventsOwned() { if (!eventsOwned) { eventsOwned = true; if (events != null) { EventDescriptor[] newEvents = new EventDescriptor[Count]; Array.Copy(events, 0, newEvents, 0, Count); this.events = newEvents; } } if (needSort) { needSort = false; InternalSort(this.namedSort); } } private void EnsureSize(int sizeNeeded) { if (sizeNeeded <= events.Length) { return; } if (events == null || events.Length == 0) { eventCount = 0; events = new EventDescriptor[sizeNeeded]; return; } EnsureEventsOwned(); int newSize = Math.Max(sizeNeeded, events.Length * 2); EventDescriptor[] newEvents = new EventDescriptor[newSize]; Array.Copy(events, 0, newEvents, 0, eventCount); events = newEvents; } /// /// public virtual EventDescriptor Find(string name, bool ignoreCase) { EventDescriptor p = null; if (ignoreCase) { for(int i = 0; i < Count; i++) { if (String.Equals(events[i].Name, name, StringComparison.OrdinalIgnoreCase)) { p = events[i]; break; } } } else { for(int i = 0; i < Count; i++) { if (String.Equals(events[i].Name, name, StringComparison.Ordinal)) { p = events[i]; break; } } } return p; } ////// Gets the description of the event with the specified /// name /// in the collection. /// ////// public int IndexOf(EventDescriptor value) { return Array.IndexOf(events, value, 0, eventCount); } ///[To be supplied.] ////// public void Insert(int index, EventDescriptor value) { if (readOnly) { throw new NotSupportedException(); } EnsureSize(eventCount + 1); if (index < eventCount) { Array.Copy(events, index, events, index + 1, eventCount - index); } events[index] = value; eventCount++; } ///[To be supplied.] ////// public void Remove(EventDescriptor value) { if (readOnly) { throw new NotSupportedException(); } int index = IndexOf(value); if (index != -1) { RemoveAt(index); } } ///[To be supplied.] ////// public void RemoveAt(int index) { if (readOnly) { throw new NotSupportedException(); } if (index < eventCount - 1) { Array.Copy(events, index + 1, events, index, eventCount - index - 1); } events[eventCount - 1] = null; eventCount--; } ///[To be supplied.] ////// public IEnumerator GetEnumerator() { // we can only return an enumerator on the events we actually have... if (events.Length == eventCount) { return events.GetEnumerator(); } else { return new ArraySubsetEnumerator(events, eventCount); } } ////// Gets an enumerator for this ///. /// /// public virtual EventDescriptorCollection Sort() { return new EventDescriptorCollection(this.events, this.eventCount, this.namedSort, this.comparer); } ////// Sorts the members of this EventDescriptorCollection, using the default sort for this collection, /// which is usually alphabetical. /// ////// public virtual EventDescriptorCollection Sort(string[] names) { return new EventDescriptorCollection(this.events, this.eventCount, names, this.comparer); } ////// Sorts the members of this EventDescriptorCollection. Any specified NamedSort arguments will /// be applied first, followed by sort using the specified IComparer. /// ////// public virtual EventDescriptorCollection Sort(string[] names, IComparer comparer) { return new EventDescriptorCollection(this.events, this.eventCount, names, comparer); } ////// Sorts the members of this EventDescriptorCollection. Any specified NamedSort arguments will /// be applied first, followed by sort using the specified IComparer. /// ////// public virtual EventDescriptorCollection Sort(IComparer comparer) { return new EventDescriptorCollection(this.events, this.eventCount, this.namedSort, comparer); } ////// Sorts the members of this EventDescriptorCollection, using the specified IComparer to compare, /// the EventDescriptors contained in the collection. /// ////// protected void InternalSort(string[] names) { if (events == null || events.Length == 0) { return; } this.InternalSort(this.comparer); if (names != null && names.Length > 0) { ArrayList eventArrayList = new ArrayList(events); int foundCount = 0; int eventCount = events.Length; for (int i = 0; i < names.Length; i++) { for (int j = 0; j < eventCount; j++) { EventDescriptor currentEvent = (EventDescriptor)eventArrayList[j]; // Found a matching event. Here, we add it to our array. We also // mark it as null in our array list so we don't add it twice later. // if (currentEvent != null && currentEvent.Name.Equals(names[i])) { events[foundCount++] = currentEvent; eventArrayList[j] = null; break; } } } // At this point we have filled in the first "foundCount" number of propeties, one for each // name in our name array. If a name didn't match, then it is ignored. Next, we must fill // in the rest of the properties. We now have a sparse array containing the remainder, so // it's easy. // for (int i = 0; i < eventCount; i++) { if (eventArrayList[i] != null) { events[foundCount++] = (EventDescriptor)eventArrayList[i]; } } Debug.Assert(foundCount == eventCount, "We did not completely fill our event array"); } } ////// Sorts the members of this EventDescriptorCollection. Any specified NamedSort arguments will /// be applied first, followed by sort using the specified IComparer. /// ////// protected void InternalSort(IComparer sorter) { if (sorter == null) { TypeDescriptor.SortDescriptorArray(this); } else { Array.Sort(events, sorter); } } ////// Sorts the members of this EventDescriptorCollection using the specified IComparer. /// ///int ICollection.Count { get { return Count; } } /// bool ICollection.IsSynchronized { get { return false; } } /// object ICollection.SyncRoot { get { return null; } } /// IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// object IList.this[int index] { get { return this[index]; } set { if (readOnly) { throw new NotSupportedException(); } if (index >= eventCount) { throw new IndexOutOfRangeException(); } EnsureEventsOwned(); events[index] = (EventDescriptor)value; } } /// int IList.Add(object value) { return Add((EventDescriptor)value); } /// void IList.Clear() { Clear(); } /// bool IList.Contains(object value) { return Contains((EventDescriptor)value); } /// int IList.IndexOf(object value) { return IndexOf((EventDescriptor)value); } /// void IList.Insert(int index, object value) { Insert(index, (EventDescriptor)value); } /// void IList.Remove(object value) { Remove((EventDescriptor)value); } /// void IList.RemoveAt(int index) { RemoveAt(index); } /// bool IList.IsReadOnly { get { return readOnly; } } /// bool IList.IsFixedSize { get { return readOnly; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Diagnostics.CodeAnalysis; /* This class has the HostProtectionAttribute. The purpose of this attribute is to enforce host-specific programming model guidelines, not security behavior. Suppress FxCop message - BUT REVISIT IF ADDING NEW SECURITY ATTRIBUTES. */ [assembly: SuppressMessage("Microsoft.Security", "CA2112:SecuredTypesShouldNotExposeFields", Scope="type", Target="System.ComponentModel.EventDescriptorCollection")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.get_IsFixedSize():System.Boolean")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.ICollection.get_SyncRoot():System.Object")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.ICollection.get_IsSynchronized():System.Boolean")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.get_IsReadOnly():System.Boolean")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.Clear():System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IEnumerable.GetEnumerator():System.Collections.IEnumerator")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.ICollection.CopyTo(System.Array,System.Int32):System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.ICollection.get_Count():System.Int32")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.Contains(System.Object):System.Boolean")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.Remove(System.Object):System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.get_Item(System.Int32):System.Object")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.set_Item(System.Int32,System.Object):System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.Add(System.Object):System.Int32")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.IndexOf(System.Object):System.Int32")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.RemoveAt(System.Int32):System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.System.Collections.IList.Insert(System.Int32,System.Object):System.Void")] [assembly: SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands", Scope="member", Target="System.ComponentModel.EventDescriptorCollection..ctor(System.ComponentModel.EventDescriptor[],System.Boolean)")] [assembly: SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.get_Count():System.Int32")] [assembly: SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands", Scope="member", Target="System.ComponentModel.EventDescriptorCollection.GetEnumerator():System.Collections.IEnumerator")] namespace System.ComponentModel { using System.Runtime.InteropServices; using System.Diagnostics; using Microsoft.Win32; using System.Collections; using System.Globalization; ////// [System.Runtime.InteropServices.ComVisible(true)] [System.Security.Permissions.HostProtection(Synchronization=true)] public class EventDescriptorCollection : ICollection, IList { private EventDescriptor[] events; private string[] namedSort; private IComparer comparer; private bool eventsOwned = true; private bool needSort = false; private int eventCount; private bool readOnly = false; ////// Represents a collection of events. /// ////// An empty AttributeCollection that can used instead of creating a new one with no items. /// public static readonly EventDescriptorCollection Empty = new EventDescriptorCollection(null, true); ////// public EventDescriptorCollection(EventDescriptor[] events) { this.events = events; if (events == null) { this.events = new EventDescriptor[0]; this.eventCount = 0; } else { this.eventCount = this.events.Length; } this.eventsOwned = true; } ////// Initializes a new instance of the ///class. /// /// Initializes a new instance of an event descriptor collection, and allows you to mark the /// collection as read-only so it cannot be modified. /// public EventDescriptorCollection(EventDescriptor[] events, bool readOnly) : this(events) { this.readOnly = readOnly; } private EventDescriptorCollection(EventDescriptor[] events, int eventCount, string[] namedSort, IComparer comparer) { this.eventsOwned = false; if (namedSort != null) { this.namedSort = (string[])namedSort.Clone(); } this.comparer = comparer; this.events = events; this.eventCount = eventCount; this.needSort = true; } ////// public int Count { get { return eventCount; } } ////// Gets the number /// of event descriptors in the collection. /// ////// public virtual EventDescriptor this[int index] { get { if (index >= eventCount) { throw new IndexOutOfRangeException(); } EnsureEventsOwned(); return events[index]; } } ///Gets the event with the specified index /// number. ////// public virtual EventDescriptor this[string name] { get { return Find(name, false); } } ////// Gets the event with the specified name. /// ////// public int Add(EventDescriptor value) { if (readOnly) { throw new NotSupportedException(); } EnsureSize(eventCount + 1); events[eventCount++] = value; return eventCount - 1; } ///[To be supplied.] ////// public void Clear() { if (readOnly) { throw new NotSupportedException(); } eventCount = 0; } ///[To be supplied.] ////// public bool Contains(EventDescriptor value) { return IndexOf(value) >= 0; } ///[To be supplied.] ///void ICollection.CopyTo(Array array, int index) { EnsureEventsOwned(); Array.Copy(events, 0, array, index, Count); } private void EnsureEventsOwned() { if (!eventsOwned) { eventsOwned = true; if (events != null) { EventDescriptor[] newEvents = new EventDescriptor[Count]; Array.Copy(events, 0, newEvents, 0, Count); this.events = newEvents; } } if (needSort) { needSort = false; InternalSort(this.namedSort); } } private void EnsureSize(int sizeNeeded) { if (sizeNeeded <= events.Length) { return; } if (events == null || events.Length == 0) { eventCount = 0; events = new EventDescriptor[sizeNeeded]; return; } EnsureEventsOwned(); int newSize = Math.Max(sizeNeeded, events.Length * 2); EventDescriptor[] newEvents = new EventDescriptor[newSize]; Array.Copy(events, 0, newEvents, 0, eventCount); events = newEvents; } /// /// public virtual EventDescriptor Find(string name, bool ignoreCase) { EventDescriptor p = null; if (ignoreCase) { for(int i = 0; i < Count; i++) { if (String.Equals(events[i].Name, name, StringComparison.OrdinalIgnoreCase)) { p = events[i]; break; } } } else { for(int i = 0; i < Count; i++) { if (String.Equals(events[i].Name, name, StringComparison.Ordinal)) { p = events[i]; break; } } } return p; } ////// Gets the description of the event with the specified /// name /// in the collection. /// ////// public int IndexOf(EventDescriptor value) { return Array.IndexOf(events, value, 0, eventCount); } ///[To be supplied.] ////// public void Insert(int index, EventDescriptor value) { if (readOnly) { throw new NotSupportedException(); } EnsureSize(eventCount + 1); if (index < eventCount) { Array.Copy(events, index, events, index + 1, eventCount - index); } events[index] = value; eventCount++; } ///[To be supplied.] ////// public void Remove(EventDescriptor value) { if (readOnly) { throw new NotSupportedException(); } int index = IndexOf(value); if (index != -1) { RemoveAt(index); } } ///[To be supplied.] ////// public void RemoveAt(int index) { if (readOnly) { throw new NotSupportedException(); } if (index < eventCount - 1) { Array.Copy(events, index + 1, events, index, eventCount - index - 1); } events[eventCount - 1] = null; eventCount--; } ///[To be supplied.] ////// public IEnumerator GetEnumerator() { // we can only return an enumerator on the events we actually have... if (events.Length == eventCount) { return events.GetEnumerator(); } else { return new ArraySubsetEnumerator(events, eventCount); } } ////// Gets an enumerator for this ///. /// /// public virtual EventDescriptorCollection Sort() { return new EventDescriptorCollection(this.events, this.eventCount, this.namedSort, this.comparer); } ////// Sorts the members of this EventDescriptorCollection, using the default sort for this collection, /// which is usually alphabetical. /// ////// public virtual EventDescriptorCollection Sort(string[] names) { return new EventDescriptorCollection(this.events, this.eventCount, names, this.comparer); } ////// Sorts the members of this EventDescriptorCollection. Any specified NamedSort arguments will /// be applied first, followed by sort using the specified IComparer. /// ////// public virtual EventDescriptorCollection Sort(string[] names, IComparer comparer) { return new EventDescriptorCollection(this.events, this.eventCount, names, comparer); } ////// Sorts the members of this EventDescriptorCollection. Any specified NamedSort arguments will /// be applied first, followed by sort using the specified IComparer. /// ////// public virtual EventDescriptorCollection Sort(IComparer comparer) { return new EventDescriptorCollection(this.events, this.eventCount, this.namedSort, comparer); } ////// Sorts the members of this EventDescriptorCollection, using the specified IComparer to compare, /// the EventDescriptors contained in the collection. /// ////// protected void InternalSort(string[] names) { if (events == null || events.Length == 0) { return; } this.InternalSort(this.comparer); if (names != null && names.Length > 0) { ArrayList eventArrayList = new ArrayList(events); int foundCount = 0; int eventCount = events.Length; for (int i = 0; i < names.Length; i++) { for (int j = 0; j < eventCount; j++) { EventDescriptor currentEvent = (EventDescriptor)eventArrayList[j]; // Found a matching event. Here, we add it to our array. We also // mark it as null in our array list so we don't add it twice later. // if (currentEvent != null && currentEvent.Name.Equals(names[i])) { events[foundCount++] = currentEvent; eventArrayList[j] = null; break; } } } // At this point we have filled in the first "foundCount" number of propeties, one for each // name in our name array. If a name didn't match, then it is ignored. Next, we must fill // in the rest of the properties. We now have a sparse array containing the remainder, so // it's easy. // for (int i = 0; i < eventCount; i++) { if (eventArrayList[i] != null) { events[foundCount++] = (EventDescriptor)eventArrayList[i]; } } Debug.Assert(foundCount == eventCount, "We did not completely fill our event array"); } } ////// Sorts the members of this EventDescriptorCollection. Any specified NamedSort arguments will /// be applied first, followed by sort using the specified IComparer. /// ////// protected void InternalSort(IComparer sorter) { if (sorter == null) { TypeDescriptor.SortDescriptorArray(this); } else { Array.Sort(events, sorter); } } ////// Sorts the members of this EventDescriptorCollection using the specified IComparer. /// ///int ICollection.Count { get { return Count; } } /// bool ICollection.IsSynchronized { get { return false; } } /// object ICollection.SyncRoot { get { return null; } } /// IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } /// object IList.this[int index] { get { return this[index]; } set { if (readOnly) { throw new NotSupportedException(); } if (index >= eventCount) { throw new IndexOutOfRangeException(); } EnsureEventsOwned(); events[index] = (EventDescriptor)value; } } /// int IList.Add(object value) { return Add((EventDescriptor)value); } /// void IList.Clear() { Clear(); } /// bool IList.Contains(object value) { return Contains((EventDescriptor)value); } /// int IList.IndexOf(object value) { return IndexOf((EventDescriptor)value); } /// void IList.Insert(int index, object value) { Insert(index, (EventDescriptor)value); } /// void IList.Remove(object value) { Remove((EventDescriptor)value); } /// void IList.RemoveAt(int index) { RemoveAt(index); } /// bool IList.IsReadOnly { get { return readOnly; } } /// bool IList.IsFixedSize { get { return readOnly; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
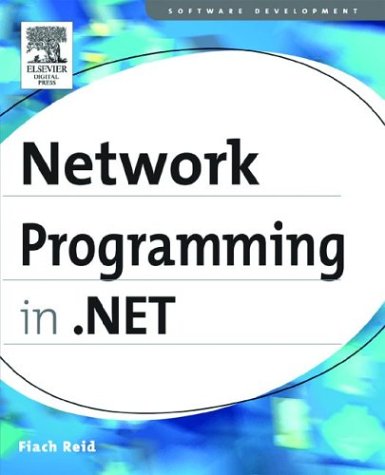
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HwndSource.cs
- ArrayElementGridEntry.cs
- BindingManagerDataErrorEventArgs.cs
- PeerResolverBindingElement.cs
- DynamicDataRoute.cs
- TdsParserStateObject.cs
- HttpWebRequestElement.cs
- ResourceContainer.cs
- WorkItem.cs
- HtmlButton.cs
- SiteOfOriginPart.cs
- ObjectItemNoOpAssemblyLoader.cs
- WebPartMovingEventArgs.cs
- TraversalRequest.cs
- EnterpriseServicesHelper.cs
- CompModSwitches.cs
- XMLSchema.cs
- PrintDialog.cs
- DataQuery.cs
- BatchServiceHost.cs
- NativeMethodsOther.cs
- SecurityElement.cs
- InvalidPrinterException.cs
- MapPathBasedVirtualPathProvider.cs
- ComPlusDiagnosticTraceRecords.cs
- SecurityTokenValidationException.cs
- MulticastOption.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- Schema.cs
- BindingSource.cs
- TextComposition.cs
- MimeMapping.cs
- ProcessHostMapPath.cs
- ArgumentException.cs
- SqlInfoMessageEvent.cs
- ToolStripSettings.cs
- InvokePattern.cs
- OrderingQueryOperator.cs
- LOSFormatter.cs
- ButtonFlatAdapter.cs
- UnsafeNativeMethods.cs
- base64Transforms.cs
- WinInetCache.cs
- SwitchLevelAttribute.cs
- CatalogZoneAutoFormat.cs
- WindowAutomationPeer.cs
- AppDomainGrammarProxy.cs
- AuthenticateEventArgs.cs
- HierarchicalDataSourceDesigner.cs
- BaseServiceProvider.cs
- WebPartConnection.cs
- ColorConverter.cs
- FixedDocumentPaginator.cs
- TagMapInfo.cs
- SerializationEventsCache.cs
- SQLDoubleStorage.cs
- RectAnimationBase.cs
- UserControl.cs
- ProviderConnectionPoint.cs
- NumberFunctions.cs
- DataGridViewTopLeftHeaderCell.cs
- SizeConverter.cs
- DirectionalLight.cs
- Simplifier.cs
- WindowClosedEventArgs.cs
- _ShellExpression.cs
- ServiceProviders.cs
- ToolboxItemFilterAttribute.cs
- RtType.cs
- PeerNameRegistration.cs
- TextBox.cs
- ExtractorMetadata.cs
- ColumnMapProcessor.cs
- SoapFault.cs
- Rfc2898DeriveBytes.cs
- TransformerInfoCollection.cs
- TabControl.cs
- ProcessHostServerConfig.cs
- EventWaitHandle.cs
- RectConverter.cs
- SetterTriggerConditionValueConverter.cs
- Expression.cs
- SqlProfileProvider.cs
- ConstraintConverter.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- OdbcParameter.cs
- MetadataUtilsSmi.cs
- TextDecorations.cs
- DiffuseMaterial.cs
- ClientBuildManager.cs
- EditorZone.cs
- WarningException.cs
- ByteStorage.cs
- DateTimeSerializationSection.cs
- CatalogPartCollection.cs
- Tablet.cs
- Int32AnimationBase.cs
- Section.cs
- FileResponseElement.cs
- CodeDefaultValueExpression.cs