Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / DiffuseMaterial.cs / 1 / DiffuseMaterial.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class DiffuseMaterial : Material { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new DiffuseMaterial Clone() { return (DiffuseMaterial)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new DiffuseMaterial CloneCurrentValue() { return (DiffuseMaterial)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DiffuseMaterial target = ((DiffuseMaterial) d); target.PropertyChanged(ColorProperty); } private static void AmbientColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DiffuseMaterial target = ((DiffuseMaterial) d); target.PropertyChanged(AmbientColorProperty); } private static void BrushPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // The first change to the default value of a mutable collection property (e.g. GeometryGroup.Children) // will promote the property value from a default value to a local value. This is technically a sub-property // change because the collection was changed and not a new collection set (GeometryGroup.Children. // Add versus GeometryGroup.Children = myNewChildrenCollection). However, we never marshalled // the default value to the compositor. If the property changes from a default value, the new local value // needs to be marshalled to the compositor. We detect this scenario with the second condition // e.OldValueSource != e.NewValueSource. Specifically in this scenario the OldValueSource will be // Default and the NewValueSource will be Local. if (e.IsASubPropertyChange && (e.OldValueSource == e.NewValueSource)) { return; } DiffuseMaterial target = ((DiffuseMaterial) d); Brush oldV = (Brush) e.OldValue; Brush newV = (Brush) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(BrushProperty); } #region Public Properties ////// Color - Color. Default value is Colors.White. /// public Color Color { get { return (Color) GetValue(ColorProperty); } set { SetValueInternal(ColorProperty, value); } } ////// AmbientColor - Color. Default value is Colors.White. /// public Color AmbientColor { get { return (Color) GetValue(AmbientColorProperty); } set { SetValueInternal(AmbientColorProperty, value); } } ////// Brush - Brush. Default value is null. /// public Brush Brush { get { return (Brush) GetValue(BrushProperty); } set { SetValueInternal(BrushProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new DiffuseMaterial(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Brush vBrush = Brush; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hBrush = vBrush != null ? ((DUCE.IResource)vBrush).GetHandle(channel) : DUCE.ResourceHandle.Null; // Pack & send command packet DUCE.MILCMD_DIFFUSEMATERIAL data; unsafe { data.Type = MILCMD.MilCmdDiffuseMaterial; data.Handle = _duceResource.GetHandle(channel); data.color = CompositionResourceManager.ColorToMilColorF(Color); data.ambientColor = CompositionResourceManager.ColorToMilColorF(AmbientColor); data.hbrush = hBrush; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_DIFFUSEMATERIAL)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_DIFFUSEMATERIAL)) { Brush vBrush = Brush; if (vBrush != null) ((DUCE.IResource)vBrush).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Brush vBrush = Brush; if (vBrush != null) ((DUCE.IResource)vBrush).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Brush // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the DiffuseMaterial.Color property. /// public static readonly DependencyProperty ColorProperty; ////// The DependencyProperty for the DiffuseMaterial.AmbientColor property. /// public static readonly DependencyProperty AmbientColorProperty; ////// The DependencyProperty for the DiffuseMaterial.Brush property. /// public static readonly DependencyProperty BrushProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal static Color s_Color = Colors.White; internal static Color s_AmbientColor = Colors.White; internal static Brush s_Brush = null; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static DiffuseMaterial() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_Brush == null || s_Brush.IsFrozen, "Detected context bound default value DiffuseMaterial.s_Brush (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(DiffuseMaterial); ColorProperty = RegisterProperty("Color", typeof(Color), typeofThis, Colors.White, new PropertyChangedCallback(ColorPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); AmbientColorProperty = RegisterProperty("AmbientColor", typeof(Color), typeofThis, Colors.White, new PropertyChangedCallback(AmbientColorPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); BrushProperty = RegisterProperty("Brush", typeof(Brush), typeofThis, null, new PropertyChangedCallback(BrushPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Media3D { sealed partial class DiffuseMaterial : Material { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new DiffuseMaterial Clone() { return (DiffuseMaterial)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new DiffuseMaterial CloneCurrentValue() { return (DiffuseMaterial)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DiffuseMaterial target = ((DiffuseMaterial) d); target.PropertyChanged(ColorProperty); } private static void AmbientColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { DiffuseMaterial target = ((DiffuseMaterial) d); target.PropertyChanged(AmbientColorProperty); } private static void BrushPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { // The first change to the default value of a mutable collection property (e.g. GeometryGroup.Children) // will promote the property value from a default value to a local value. This is technically a sub-property // change because the collection was changed and not a new collection set (GeometryGroup.Children. // Add versus GeometryGroup.Children = myNewChildrenCollection). However, we never marshalled // the default value to the compositor. If the property changes from a default value, the new local value // needs to be marshalled to the compositor. We detect this scenario with the second condition // e.OldValueSource != e.NewValueSource. Specifically in this scenario the OldValueSource will be // Default and the NewValueSource will be Local. if (e.IsASubPropertyChange && (e.OldValueSource == e.NewValueSource)) { return; } DiffuseMaterial target = ((DiffuseMaterial) d); Brush oldV = (Brush) e.OldValue; Brush newV = (Brush) e.NewValue; System.Windows.Threading.Dispatcher dispatcher = target.Dispatcher; if (dispatcher != null) { DUCE.IResource targetResource = (DUCE.IResource)target; using (CompositionEngineLock.Acquire()) { int channelCount = targetResource.GetChannelCount(); for (int channelIndex = 0; channelIndex < channelCount; channelIndex++) { DUCE.Channel channel = targetResource.GetChannel(channelIndex); Debug.Assert(!channel.IsOutOfBandChannel); Debug.Assert(!targetResource.GetHandle(channel).IsNull); target.ReleaseResource(oldV,channel); target.AddRefResource(newV,channel); } } } target.PropertyChanged(BrushProperty); } #region Public Properties ////// Color - Color. Default value is Colors.White. /// public Color Color { get { return (Color) GetValue(ColorProperty); } set { SetValueInternal(ColorProperty, value); } } ////// AmbientColor - Color. Default value is Colors.White. /// public Color AmbientColor { get { return (Color) GetValue(AmbientColorProperty); } set { SetValueInternal(AmbientColorProperty, value); } } ////// Brush - Brush. Default value is null. /// public Brush Brush { get { return (Brush) GetValue(BrushProperty); } set { SetValueInternal(BrushProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new DiffuseMaterial(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Read values of properties into local variables Brush vBrush = Brush; // Obtain handles for properties that implement DUCE.IResource DUCE.ResourceHandle hBrush = vBrush != null ? ((DUCE.IResource)vBrush).GetHandle(channel) : DUCE.ResourceHandle.Null; // Pack & send command packet DUCE.MILCMD_DIFFUSEMATERIAL data; unsafe { data.Type = MILCMD.MilCmdDiffuseMaterial; data.Handle = _duceResource.GetHandle(channel); data.color = CompositionResourceManager.ColorToMilColorF(Color); data.ambientColor = CompositionResourceManager.ColorToMilColorF(AmbientColor); data.hbrush = hBrush; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_DIFFUSEMATERIAL)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_DIFFUSEMATERIAL)) { Brush vBrush = Brush; if (vBrush != null) ((DUCE.IResource)vBrush).AddRefOnChannel(channel); AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { Brush vBrush = Brush; if (vBrush != null) ((DUCE.IResource)vBrush).ReleaseOnChannel(channel); ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // Brush // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the DiffuseMaterial.Color property. /// public static readonly DependencyProperty ColorProperty; ////// The DependencyProperty for the DiffuseMaterial.AmbientColor property. /// public static readonly DependencyProperty AmbientColorProperty; ////// The DependencyProperty for the DiffuseMaterial.Brush property. /// public static readonly DependencyProperty BrushProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal static Color s_Color = Colors.White; internal static Color s_AmbientColor = Colors.White; internal static Brush s_Brush = null; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static DiffuseMaterial() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_Brush == null || s_Brush.IsFrozen, "Detected context bound default value DiffuseMaterial.s_Brush (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(DiffuseMaterial); ColorProperty = RegisterProperty("Color", typeof(Color), typeofThis, Colors.White, new PropertyChangedCallback(ColorPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); AmbientColorProperty = RegisterProperty("AmbientColor", typeof(Color), typeofThis, Colors.White, new PropertyChangedCallback(AmbientColorPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); BrushProperty = RegisterProperty("Brush", typeof(Brush), typeofThis, null, new PropertyChangedCallback(BrushPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
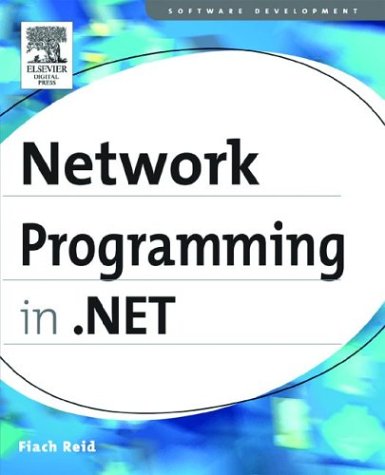
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Reference.cs
- EventLogQuery.cs
- EncodedStreamFactory.cs
- FileLoadException.cs
- ListViewItem.cs
- EnumMember.cs
- Table.cs
- DataServiceKeyAttribute.cs
- CorrelationValidator.cs
- ColumnResizeAdorner.cs
- SignatureToken.cs
- Expression.cs
- DetailsViewDeleteEventArgs.cs
- SafeMILHandle.cs
- VirtualizingStackPanel.cs
- ToolZone.cs
- PropertyRef.cs
- NamedPipeTransportBindingElement.cs
- KeyProperty.cs
- OleDbRowUpdatingEvent.cs
- CombinedGeometry.cs
- HwndKeyboardInputProvider.cs
- PersonalizationStateInfo.cs
- DataGridViewLayoutData.cs
- EventSinkActivity.cs
- SurrogateSelector.cs
- HeaderCollection.cs
- __ConsoleStream.cs
- ArraySortHelper.cs
- DocumentAutomationPeer.cs
- FormClosedEvent.cs
- SubqueryRules.cs
- CodeTypeParameter.cs
- SectionUpdates.cs
- Missing.cs
- Cursor.cs
- CodeDomSerializationProvider.cs
- StaticDataManager.cs
- Comparer.cs
- Int16AnimationBase.cs
- SystemTcpConnection.cs
- BindingValueChangedEventArgs.cs
- ProxyOperationRuntime.cs
- SerializerProvider.cs
- MsdtcClusterUtils.cs
- BoolExpressionVisitors.cs
- ReadOnlyObservableCollection.cs
- Speller.cs
- SegmentInfo.cs
- CustomAssemblyResolver.cs
- SmtpReplyReader.cs
- WorkflowMarkupSerializationProvider.cs
- Win32Native.cs
- ToolstripProfessionalRenderer.cs
- DesignUtil.cs
- CodeLabeledStatement.cs
- AccessedThroughPropertyAttribute.cs
- RoutedCommand.cs
- SqlBooleanMismatchVisitor.cs
- ProcessThread.cs
- DataRowView.cs
- RealProxy.cs
- HttpCachePolicy.cs
- Queue.cs
- RequestTimeoutManager.cs
- ListBox.cs
- CalendarDay.cs
- DataSetViewSchema.cs
- ComboBoxRenderer.cs
- VisualStateManager.cs
- AspNetRouteServiceHttpHandler.cs
- TextEditorMouse.cs
- ZipQueryOperator.cs
- TableLayoutRowStyleCollection.cs
- GridViewCancelEditEventArgs.cs
- StylusDownEventArgs.cs
- RuleInfoComparer.cs
- ExpressionConverter.cs
- ExtractedStateEntry.cs
- AssertSection.cs
- TagElement.cs
- SpStreamWrapper.cs
- OperatingSystemVersionCheck.cs
- SplitterDesigner.cs
- DispatcherHooks.cs
- IntSecurity.cs
- ParallelDesigner.cs
- StylusPlugin.cs
- DataGridViewComboBoxColumn.cs
- StaticFileHandler.cs
- IdnElement.cs
- NonBatchDirectoryCompiler.cs
- ErrorStyle.cs
- XslAst.cs
- Font.cs
- ItemsPresenter.cs
- BlurBitmapEffect.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- SafeSerializationManager.cs
- ValidationVisibilityAttribute.cs