Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Command / RoutedCommand.cs / 1305600 / RoutedCommand.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.ComponentModel; using System.Collections; using System.Windows; using System.Windows.Input; using System.Windows.Markup; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// A command that causes handlers associated with it to be called. /// [TypeConverter("System.Windows.Input.CommandConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [ValueSerializer("System.Windows.Input.CommandValueSerializer, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] public class RoutedCommand : ICommand { #region Constructors ////// Default Constructor - needed to allow markup creation /// public RoutedCommand() { _name = String.Empty; _ownerType = null; _inputGestureCollection = null; } ////// RoutedCommand Constructor with Name and OwnerType /// /// Declared Name of the RoutedCommand for Serialization /// Type that is registering the property public RoutedCommand(string name, Type ownerType) : this(name, ownerType, null) { } ////// RoutedCommand Constructor with Name and OwnerType /// /// Declared Name of the RoutedCommand for Serialization /// Type that is registering the property /// Default Input Gestures associated public RoutedCommand(string name, Type ownerType, InputGestureCollection inputGestures) { if (name == null) { throw new ArgumentNullException("name"); } if (name.Length == 0) { throw new ArgumentException(SR.Get(SRID.StringEmpty), "name"); } if (ownerType == null) { throw new ArgumentNullException("ownerType"); } _name = name; _ownerType = ownerType; _inputGestureCollection = inputGestures; } ////// RoutedCommand Constructor with Name and OwnerType and command identifier. /// /// Declared Name of the RoutedCommand for Serialization /// Type that is registering the property /// Byte identifier for the command assigned by the owning type internal RoutedCommand(string name, Type ownerType, byte commandId) : this(name, ownerType, null) { _commandId = commandId; } #endregion #region ICommand ////// Executes the command with the given parameter on the currently focused element. /// /// Parameter to pass to any command handlers. void ICommand.Execute(object parameter) { Execute(parameter, FilterInputElement(Keyboard.FocusedElement)); } ////// Whether the command can be executed with the given parameter on the currently focused element. /// /// Parameter to pass to any command handlers. ///true if the command can be executed, false otherwise. ////// Critical: This code takes in a trusted bit which can be used to cause elevations for paste /// PublicOK: This code passes the flag in as false /// [SecurityCritical] bool ICommand.CanExecute(object parameter) { bool unused; return CanExecuteImpl(parameter, FilterInputElement(Keyboard.FocusedElement), false, out unused); } ////// Raised when CanExecute should be requeried on commands. /// Since commands are often global, it will only hold onto the handler as a weak reference. /// Users of this event should keep a strong reference to their event handler to avoid /// it being garbage collected. This can be accomplished by having a private field /// and assigning the handler as the value before or after attaching to this event. /// public event EventHandler CanExecuteChanged { add { CommandManager.RequerySuggested += value; } remove { CommandManager.RequerySuggested -= value; } } #endregion #region Public Methods ////// Executes the command with the given parameter on the given target. /// /// Parameter to be passed to any command handlers. /// Element at which to begin looking for command handlers. ////// Critical - calls critical function (ExecuteImpl) /// PublicOk - always passes in false for userInitiated, which is safe /// [SecurityCritical] public void Execute(object parameter, IInputElement target) { // We only support UIElement, ContentElement and UIElement3D if ((target != null) && !InputElement.IsValid(target)) { throw new InvalidOperationException(SR.Get(SRID.Invalid_IInputElement, target.GetType())); } if (target == null) { target = FilterInputElement(Keyboard.FocusedElement); } ExecuteImpl(parameter, target, false); } ////// Whether the command can be executed with the given parameter on the given target. /// /// Parameter to be passed to any command handlers. /// The target element on which to begin looking for command handlers. ///true if the command can be executed, false otherwise. ////// Critical: This can be used to spoof input and cause userinitiated permission to be asserted /// PublicOK: The call sets trusted bit to false which prevents user initiated permission from being asserted /// [SecurityCritical] public bool CanExecute(object parameter, IInputElement target) { bool unused; return CriticalCanExecute(parameter, target, false, out unused); } ////// Whether the command can be executed with the given parameter on the given target. /// /// Parameter to be passed to any command handlers. /// The target element on which to begin looking for command handlers. /// Determines whether this call will elevate for userinitiated input or not. /// Determines whether the input event (if any) that caused this command should continue its route. ///true if the command can be executed, false otherwise. ////// Critical: This code takes in a trusted bit which can be used to cause elevations for paste /// [SecurityCritical] internal bool CriticalCanExecute(object parameter, IInputElement target, bool trusted, out bool continueRouting) { // We only support UIElement, ContentElement, and UIElement3D if ((target != null) && !InputElement.IsValid(target)) { throw new InvalidOperationException(SR.Get(SRID.Invalid_IInputElement, target.GetType())); } if (target == null) { target = FilterInputElement(Keyboard.FocusedElement); } return CanExecuteImpl(parameter, target, trusted, out continueRouting); } #endregion #region Public Properties ////// Name - Declared time Name of the property/field where it is /// defined, for serialization/debug purposes only. /// Ex: public static RoutedCommand New { get { new RoutedCommand("New", .... ) } } /// public static RoutedCommand New = new RoutedCommand("New", ... ) ; /// public string Name { get { return _name; } } ////// Owning type of the property /// public Type OwnerType { get { return _ownerType; } } ////// Identifier assigned by the owning Type. Note that this is not a global command identifier. /// internal byte CommandId { get { return _commandId; } } ////// Input Gestures associated with RoutedCommand /// public InputGestureCollection InputGestures { get { if(InputGesturesInternal == null) { _inputGestureCollection = new InputGestureCollection(); } return _inputGestureCollection; } } internal InputGestureCollection InputGesturesInternal { get { if(_inputGestureCollection == null && AreInputGesturesDelayLoaded) { _inputGestureCollection = GetInputGestures(); AreInputGesturesDelayLoaded = false; } return _inputGestureCollection; } } ////// Fetches the default input gestures for the command by invoking the LoadDefaultGestureFromResource function on the owning type. /// ///collection of input gestures for the command private InputGestureCollection GetInputGestures() { if(OwnerType == typeof(ApplicationCommands)) { return ApplicationCommands.LoadDefaultGestureFromResource(_commandId); } else if(OwnerType == typeof(NavigationCommands)) { return NavigationCommands.LoadDefaultGestureFromResource(_commandId); } else if(OwnerType == typeof(MediaCommands)) { return MediaCommands.LoadDefaultGestureFromResource(_commandId); } else if(OwnerType == typeof(ComponentCommands)) { return ComponentCommands.LoadDefaultGestureFromResource(_commandId); } return new InputGestureCollection(); } ////// Rights Management Enabledness /// Will be set by Rights Management code. /// internal bool IsBlockedByRM { [SecurityCritical] get { return ReadPrivateFlag(PrivateFlags.IsBlockedByRM); } [UIPermissionAttribute(SecurityAction.LinkDemand, Unrestricted = true)] [SecurityCritical] set { WritePrivateFlag(PrivateFlags.IsBlockedByRM, value); } } /// /// Critical: Calls WritePrivateFlag /// TreatAsSafe: Setting IsBlockedByRM is a critical operation. Setting AreInputGesturesDelayLoaded isn't. /// internal bool AreInputGesturesDelayLoaded { get { return ReadPrivateFlag(PrivateFlags.AreInputGesturesDelayLoaded); } [SecurityCritical, SecurityTreatAsSafe] set { WritePrivateFlag(PrivateFlags.AreInputGesturesDelayLoaded, value); } } #endregion #region Implementation private static IInputElement FilterInputElement(IInputElement elem) { // We only support UIElement, ContentElement, and UIElement3D if ((elem != null) && InputElement.IsValid(elem)) { return elem; } return null; } /// /// /// /// ////// /// Critical: This code takes in a trusted bit which can be used to cause elevations for paste /// [SecurityCritical] private bool CanExecuteImpl(object parameter, IInputElement target, bool trusted, out bool continueRouting) { // If blocked by rights-management fall through and return false if ((target != null) && !IsBlockedByRM) { // Raise the Preview Event, check the Handled value, and raise the regular event. CanExecuteRoutedEventArgs args = new CanExecuteRoutedEventArgs(this, parameter); args.RoutedEvent = CommandManager.PreviewCanExecuteEvent; CriticalCanExecuteWrapper(parameter, target, trusted, args); if (!args.Handled) { args.RoutedEvent = CommandManager.CanExecuteEvent; CriticalCanExecuteWrapper(parameter, target, trusted, args); } continueRouting = args.ContinueRouting; return args.CanExecute; } else { continueRouting = false; return false; } } ////// Critical: This code takes in a trusted bit which can be used to cause elevations for paste /// [SecurityCritical] private void CriticalCanExecuteWrapper(object parameter, IInputElement target, bool trusted, CanExecuteRoutedEventArgs args) { // This cast is ok since we are already testing for UIElement, ContentElement, or UIElement3D // both of which derive from DO DependencyObject targetAsDO = (DependencyObject)target; if (InputElement.IsUIElement(targetAsDO)) { ((UIElement)targetAsDO).RaiseEvent(args, trusted); } else if (InputElement.IsContentElement(targetAsDO)) { ((ContentElement)targetAsDO).RaiseEvent(args, trusted); } else if (InputElement.IsUIElement3D(targetAsDO)) { ((UIElement3D)targetAsDO).RaiseEvent(args, trusted); } } ////// Critical - Calls ExecuteImpl, which sets the user initiated bit on a command, which is used /// for security purposes later. It is important to validate /// the callers of this, and the implementation to make sure /// that we only call MarkAsUserInitiated in the correct cases. /// [SecurityCritical] internal bool ExecuteCore(object parameter, IInputElement target, bool userInitiated) { if (target == null) { target = FilterInputElement(Keyboard.FocusedElement); } return ExecuteImpl(parameter, target, userInitiated); } ////// Critical - sets the user initiated bit on a command, which is used /// for security purposes later. It is important to validate /// the callers of this, and the implementation to make sure /// that we only call MarkAsUserInitiated in the correct cases. /// [SecurityCritical] private bool ExecuteImpl(object parameter, IInputElement target, bool userInitiated) { // If blocked by rights-management fall through and return false if ((target != null) && !IsBlockedByRM) { UIElement targetUIElement = target as UIElement; ContentElement targetAsContentElement = null; UIElement3D targetAsUIElement3D = null; // Raise the Preview Event and check for Handled value, and // Raise the regular ExecuteEvent. ExecutedRoutedEventArgs args = new ExecutedRoutedEventArgs(this, parameter); args.RoutedEvent = CommandManager.PreviewExecutedEvent; if (targetUIElement != null) { targetUIElement.RaiseEvent(args, userInitiated); } else { targetAsContentElement = target as ContentElement; if (targetAsContentElement != null) { targetAsContentElement.RaiseEvent(args, userInitiated); } else { targetAsUIElement3D = target as UIElement3D; if (targetAsUIElement3D != null) { targetAsUIElement3D.RaiseEvent(args, userInitiated); } } } if (!args.Handled) { args.RoutedEvent = CommandManager.ExecutedEvent; if (targetUIElement != null) { targetUIElement.RaiseEvent(args, userInitiated); } else if (targetAsContentElement != null) { targetAsContentElement.RaiseEvent(args, userInitiated); } else if (targetAsUIElement3D != null) { targetAsUIElement3D.RaiseEvent(args, userInitiated); } } return args.Handled; } return false; } #endregion #region PrivateMethods ////// Critical - Accesses _flags. Setting IsBlockedByRM is a critical operation. Setting other flags should be fine. /// [SecurityCritical] private void WritePrivateFlag(PrivateFlags bit, bool value) { if (value) { _flags.Value |= bit; } else { _flags.Value &= ~bit; } } private bool ReadPrivateFlag(PrivateFlags bit) { return (_flags.Value & bit) != 0; } #endregion PrivateMethods #region Data private string _name; ////// Critical : Holds the value to IsBlockedByRM which should not be settable from PartialTrust. /// private MS.Internal.SecurityCriticalDataForSet_flags; private enum PrivateFlags : byte { IsBlockedByRM = 0x01, AreInputGesturesDelayLoaded = 0x02 } private Type _ownerType; private InputGestureCollection _inputGestureCollection; private byte _commandId; //Note that this is NOT a global command identifier. It is specific to the owning type. //it represents one of the CommandID enums defined by each of the command types and will be cast to one of them when used. #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using System.ComponentModel; using System.Collections; using System.Windows; using System.Windows.Input; using System.Windows.Markup; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { /// /// A command that causes handlers associated with it to be called. /// [TypeConverter("System.Windows.Input.CommandConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [ValueSerializer("System.Windows.Input.CommandValueSerializer, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] public class RoutedCommand : ICommand { #region Constructors ////// Default Constructor - needed to allow markup creation /// public RoutedCommand() { _name = String.Empty; _ownerType = null; _inputGestureCollection = null; } ////// RoutedCommand Constructor with Name and OwnerType /// /// Declared Name of the RoutedCommand for Serialization /// Type that is registering the property public RoutedCommand(string name, Type ownerType) : this(name, ownerType, null) { } ////// RoutedCommand Constructor with Name and OwnerType /// /// Declared Name of the RoutedCommand for Serialization /// Type that is registering the property /// Default Input Gestures associated public RoutedCommand(string name, Type ownerType, InputGestureCollection inputGestures) { if (name == null) { throw new ArgumentNullException("name"); } if (name.Length == 0) { throw new ArgumentException(SR.Get(SRID.StringEmpty), "name"); } if (ownerType == null) { throw new ArgumentNullException("ownerType"); } _name = name; _ownerType = ownerType; _inputGestureCollection = inputGestures; } ////// RoutedCommand Constructor with Name and OwnerType and command identifier. /// /// Declared Name of the RoutedCommand for Serialization /// Type that is registering the property /// Byte identifier for the command assigned by the owning type internal RoutedCommand(string name, Type ownerType, byte commandId) : this(name, ownerType, null) { _commandId = commandId; } #endregion #region ICommand ////// Executes the command with the given parameter on the currently focused element. /// /// Parameter to pass to any command handlers. void ICommand.Execute(object parameter) { Execute(parameter, FilterInputElement(Keyboard.FocusedElement)); } ////// Whether the command can be executed with the given parameter on the currently focused element. /// /// Parameter to pass to any command handlers. ///true if the command can be executed, false otherwise. ////// Critical: This code takes in a trusted bit which can be used to cause elevations for paste /// PublicOK: This code passes the flag in as false /// [SecurityCritical] bool ICommand.CanExecute(object parameter) { bool unused; return CanExecuteImpl(parameter, FilterInputElement(Keyboard.FocusedElement), false, out unused); } ////// Raised when CanExecute should be requeried on commands. /// Since commands are often global, it will only hold onto the handler as a weak reference. /// Users of this event should keep a strong reference to their event handler to avoid /// it being garbage collected. This can be accomplished by having a private field /// and assigning the handler as the value before or after attaching to this event. /// public event EventHandler CanExecuteChanged { add { CommandManager.RequerySuggested += value; } remove { CommandManager.RequerySuggested -= value; } } #endregion #region Public Methods ////// Executes the command with the given parameter on the given target. /// /// Parameter to be passed to any command handlers. /// Element at which to begin looking for command handlers. ////// Critical - calls critical function (ExecuteImpl) /// PublicOk - always passes in false for userInitiated, which is safe /// [SecurityCritical] public void Execute(object parameter, IInputElement target) { // We only support UIElement, ContentElement and UIElement3D if ((target != null) && !InputElement.IsValid(target)) { throw new InvalidOperationException(SR.Get(SRID.Invalid_IInputElement, target.GetType())); } if (target == null) { target = FilterInputElement(Keyboard.FocusedElement); } ExecuteImpl(parameter, target, false); } ////// Whether the command can be executed with the given parameter on the given target. /// /// Parameter to be passed to any command handlers. /// The target element on which to begin looking for command handlers. ///true if the command can be executed, false otherwise. ////// Critical: This can be used to spoof input and cause userinitiated permission to be asserted /// PublicOK: The call sets trusted bit to false which prevents user initiated permission from being asserted /// [SecurityCritical] public bool CanExecute(object parameter, IInputElement target) { bool unused; return CriticalCanExecute(parameter, target, false, out unused); } ////// Whether the command can be executed with the given parameter on the given target. /// /// Parameter to be passed to any command handlers. /// The target element on which to begin looking for command handlers. /// Determines whether this call will elevate for userinitiated input or not. /// Determines whether the input event (if any) that caused this command should continue its route. ///true if the command can be executed, false otherwise. ////// Critical: This code takes in a trusted bit which can be used to cause elevations for paste /// [SecurityCritical] internal bool CriticalCanExecute(object parameter, IInputElement target, bool trusted, out bool continueRouting) { // We only support UIElement, ContentElement, and UIElement3D if ((target != null) && !InputElement.IsValid(target)) { throw new InvalidOperationException(SR.Get(SRID.Invalid_IInputElement, target.GetType())); } if (target == null) { target = FilterInputElement(Keyboard.FocusedElement); } return CanExecuteImpl(parameter, target, trusted, out continueRouting); } #endregion #region Public Properties ////// Name - Declared time Name of the property/field where it is /// defined, for serialization/debug purposes only. /// Ex: public static RoutedCommand New { get { new RoutedCommand("New", .... ) } } /// public static RoutedCommand New = new RoutedCommand("New", ... ) ; /// public string Name { get { return _name; } } ////// Owning type of the property /// public Type OwnerType { get { return _ownerType; } } ////// Identifier assigned by the owning Type. Note that this is not a global command identifier. /// internal byte CommandId { get { return _commandId; } } ////// Input Gestures associated with RoutedCommand /// public InputGestureCollection InputGestures { get { if(InputGesturesInternal == null) { _inputGestureCollection = new InputGestureCollection(); } return _inputGestureCollection; } } internal InputGestureCollection InputGesturesInternal { get { if(_inputGestureCollection == null && AreInputGesturesDelayLoaded) { _inputGestureCollection = GetInputGestures(); AreInputGesturesDelayLoaded = false; } return _inputGestureCollection; } } ////// Fetches the default input gestures for the command by invoking the LoadDefaultGestureFromResource function on the owning type. /// ///collection of input gestures for the command private InputGestureCollection GetInputGestures() { if(OwnerType == typeof(ApplicationCommands)) { return ApplicationCommands.LoadDefaultGestureFromResource(_commandId); } else if(OwnerType == typeof(NavigationCommands)) { return NavigationCommands.LoadDefaultGestureFromResource(_commandId); } else if(OwnerType == typeof(MediaCommands)) { return MediaCommands.LoadDefaultGestureFromResource(_commandId); } else if(OwnerType == typeof(ComponentCommands)) { return ComponentCommands.LoadDefaultGestureFromResource(_commandId); } return new InputGestureCollection(); } ////// Rights Management Enabledness /// Will be set by Rights Management code. /// internal bool IsBlockedByRM { [SecurityCritical] get { return ReadPrivateFlag(PrivateFlags.IsBlockedByRM); } [UIPermissionAttribute(SecurityAction.LinkDemand, Unrestricted = true)] [SecurityCritical] set { WritePrivateFlag(PrivateFlags.IsBlockedByRM, value); } } /// /// Critical: Calls WritePrivateFlag /// TreatAsSafe: Setting IsBlockedByRM is a critical operation. Setting AreInputGesturesDelayLoaded isn't. /// internal bool AreInputGesturesDelayLoaded { get { return ReadPrivateFlag(PrivateFlags.AreInputGesturesDelayLoaded); } [SecurityCritical, SecurityTreatAsSafe] set { WritePrivateFlag(PrivateFlags.AreInputGesturesDelayLoaded, value); } } #endregion #region Implementation private static IInputElement FilterInputElement(IInputElement elem) { // We only support UIElement, ContentElement, and UIElement3D if ((elem != null) && InputElement.IsValid(elem)) { return elem; } return null; } /// /// /// /// ////// /// Critical: This code takes in a trusted bit which can be used to cause elevations for paste /// [SecurityCritical] private bool CanExecuteImpl(object parameter, IInputElement target, bool trusted, out bool continueRouting) { // If blocked by rights-management fall through and return false if ((target != null) && !IsBlockedByRM) { // Raise the Preview Event, check the Handled value, and raise the regular event. CanExecuteRoutedEventArgs args = new CanExecuteRoutedEventArgs(this, parameter); args.RoutedEvent = CommandManager.PreviewCanExecuteEvent; CriticalCanExecuteWrapper(parameter, target, trusted, args); if (!args.Handled) { args.RoutedEvent = CommandManager.CanExecuteEvent; CriticalCanExecuteWrapper(parameter, target, trusted, args); } continueRouting = args.ContinueRouting; return args.CanExecute; } else { continueRouting = false; return false; } } ////// Critical: This code takes in a trusted bit which can be used to cause elevations for paste /// [SecurityCritical] private void CriticalCanExecuteWrapper(object parameter, IInputElement target, bool trusted, CanExecuteRoutedEventArgs args) { // This cast is ok since we are already testing for UIElement, ContentElement, or UIElement3D // both of which derive from DO DependencyObject targetAsDO = (DependencyObject)target; if (InputElement.IsUIElement(targetAsDO)) { ((UIElement)targetAsDO).RaiseEvent(args, trusted); } else if (InputElement.IsContentElement(targetAsDO)) { ((ContentElement)targetAsDO).RaiseEvent(args, trusted); } else if (InputElement.IsUIElement3D(targetAsDO)) { ((UIElement3D)targetAsDO).RaiseEvent(args, trusted); } } ////// Critical - Calls ExecuteImpl, which sets the user initiated bit on a command, which is used /// for security purposes later. It is important to validate /// the callers of this, and the implementation to make sure /// that we only call MarkAsUserInitiated in the correct cases. /// [SecurityCritical] internal bool ExecuteCore(object parameter, IInputElement target, bool userInitiated) { if (target == null) { target = FilterInputElement(Keyboard.FocusedElement); } return ExecuteImpl(parameter, target, userInitiated); } ////// Critical - sets the user initiated bit on a command, which is used /// for security purposes later. It is important to validate /// the callers of this, and the implementation to make sure /// that we only call MarkAsUserInitiated in the correct cases. /// [SecurityCritical] private bool ExecuteImpl(object parameter, IInputElement target, bool userInitiated) { // If blocked by rights-management fall through and return false if ((target != null) && !IsBlockedByRM) { UIElement targetUIElement = target as UIElement; ContentElement targetAsContentElement = null; UIElement3D targetAsUIElement3D = null; // Raise the Preview Event and check for Handled value, and // Raise the regular ExecuteEvent. ExecutedRoutedEventArgs args = new ExecutedRoutedEventArgs(this, parameter); args.RoutedEvent = CommandManager.PreviewExecutedEvent; if (targetUIElement != null) { targetUIElement.RaiseEvent(args, userInitiated); } else { targetAsContentElement = target as ContentElement; if (targetAsContentElement != null) { targetAsContentElement.RaiseEvent(args, userInitiated); } else { targetAsUIElement3D = target as UIElement3D; if (targetAsUIElement3D != null) { targetAsUIElement3D.RaiseEvent(args, userInitiated); } } } if (!args.Handled) { args.RoutedEvent = CommandManager.ExecutedEvent; if (targetUIElement != null) { targetUIElement.RaiseEvent(args, userInitiated); } else if (targetAsContentElement != null) { targetAsContentElement.RaiseEvent(args, userInitiated); } else if (targetAsUIElement3D != null) { targetAsUIElement3D.RaiseEvent(args, userInitiated); } } return args.Handled; } return false; } #endregion #region PrivateMethods ////// Critical - Accesses _flags. Setting IsBlockedByRM is a critical operation. Setting other flags should be fine. /// [SecurityCritical] private void WritePrivateFlag(PrivateFlags bit, bool value) { if (value) { _flags.Value |= bit; } else { _flags.Value &= ~bit; } } private bool ReadPrivateFlag(PrivateFlags bit) { return (_flags.Value & bit) != 0; } #endregion PrivateMethods #region Data private string _name; ////// Critical : Holds the value to IsBlockedByRM which should not be settable from PartialTrust. /// private MS.Internal.SecurityCriticalDataForSet_flags; private enum PrivateFlags : byte { IsBlockedByRM = 0x01, AreInputGesturesDelayLoaded = 0x02 } private Type _ownerType; private InputGestureCollection _inputGestureCollection; private byte _commandId; //Note that this is NOT a global command identifier. It is specific to the owning type. //it represents one of the CommandID enums defined by each of the command types and will be cast to one of them when used. #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
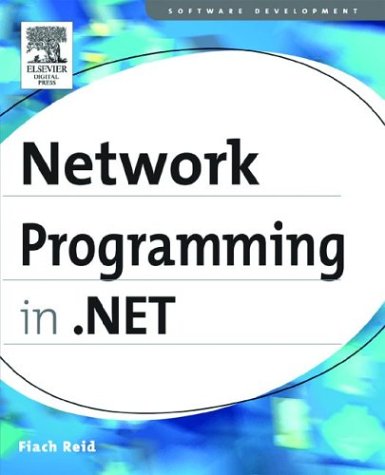
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectNavigationPropertyMapping.cs
- FormatterServices.cs
- RuntimeEnvironment.cs
- TreeViewDesigner.cs
- ToolStripArrowRenderEventArgs.cs
- RenderingEventArgs.cs
- _FtpControlStream.cs
- BitmapMetadataBlob.cs
- CacheMemory.cs
- WithStatement.cs
- CriticalExceptions.cs
- WebBaseEventKeyComparer.cs
- TimelineGroup.cs
- MetaDataInfo.cs
- ChooseAction.cs
- _SslStream.cs
- ZipIOFileItemStream.cs
- ModelItemCollectionImpl.cs
- ContextStack.cs
- EventLogPermissionAttribute.cs
- DataBoundControlHelper.cs
- ScriptingAuthenticationServiceSection.cs
- ExpressionEditorSheet.cs
- Condition.cs
- MailBnfHelper.cs
- ToolStripGrip.cs
- SqlCacheDependency.cs
- TextSimpleMarkerProperties.cs
- HttpException.cs
- BrowserCapabilitiesCodeGenerator.cs
- UnsafeNativeMethods.cs
- BamlRecordReader.cs
- PageCache.cs
- BrowsableAttribute.cs
- XPathDocumentNavigator.cs
- TdsParameterSetter.cs
- StylusPointProperties.cs
- DiagnosticTrace.cs
- SqlDependencyListener.cs
- PageCatalogPart.cs
- ProjectionCamera.cs
- ComponentManagerBroker.cs
- DSASignatureFormatter.cs
- ValidationSummary.cs
- CompletedAsyncResult.cs
- WebPartMinimizeVerb.cs
- InfoCardRSACryptoProvider.cs
- UniqueEventHelper.cs
- DataBindEngine.cs
- WebPartExportVerb.cs
- FontCollection.cs
- HandlerWithFactory.cs
- ComboBoxHelper.cs
- TypeProvider.cs
- SafeEventLogWriteHandle.cs
- ExpandCollapsePattern.cs
- SiteMapDataSource.cs
- FlowDocumentFormatter.cs
- ProxyWebPartManager.cs
- SQLMoneyStorage.cs
- TabletDevice.cs
- SimplePropertyEntry.cs
- ActivityAction.cs
- securitycriticaldata.cs
- WebPartPersonalization.cs
- HelloMessageApril2005.cs
- BitmapImage.cs
- DataTableCollection.cs
- HttpApplicationStateWrapper.cs
- NativeMethods.cs
- _Connection.cs
- DataGridViewComboBoxCell.cs
- ListViewItem.cs
- DoubleAnimation.cs
- SafeNativeMethods.cs
- GeometryCollection.cs
- ReadOnlyCollectionBuilder.cs
- IgnoreDeviceFilterElement.cs
- Package.cs
- SamlSubjectStatement.cs
- TrailingSpaceComparer.cs
- CryptoProvider.cs
- HttpMethodAttribute.cs
- ConfigurationValidatorBase.cs
- ValidationRuleCollection.cs
- DataGridViewCellCancelEventArgs.cs
- DesignerSerializationOptionsAttribute.cs
- ValidationPropertyAttribute.cs
- XmlAutoDetectWriter.cs
- SudsWriter.cs
- StringDictionaryWithComparer.cs
- ProfileGroupSettingsCollection.cs
- SspiSafeHandles.cs
- EncryptedKeyIdentifierClause.cs
- InterleavedZipPartStream.cs
- XmlElementList.cs
- AsnEncodedData.cs
- Base64Encoding.cs
- RegexCapture.cs
- DecryptRequest.cs