Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Generated / TextEffect.cs / 1 / TextEffect.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class TextEffect : Animatable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new TextEffect Clone() { return (TextEffect)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new TextEffect CloneCurrentValue() { return (TextEffect)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static bool ValidatePositionStartValue(object value) { // This resource needs to be notified on new values being set. if (!OnPositionStartChanging((int) value)) { return false; } return true; } private static bool ValidatePositionCountValue(object value) { // This resource needs to be notified on new values being set. if (!OnPositionCountChanging((int) value)) { return false; } return true; } #region Public Properties ////// Transform - Transform. Default value is null. /// Transform on the text with this effect /// public Transform Transform { get { return (Transform) GetValue(TransformProperty); } set { SetValueInternal(TransformProperty, value); } } ////// Clip - Geometry. Default value is null. /// Clip on the text with this effect /// public Geometry Clip { get { return (Geometry) GetValue(ClipProperty); } set { SetValueInternal(ClipProperty, value); } } ////// Foreground - Brush. Default value is null. /// Foreground for the text with this effect /// public Brush Foreground { get { return (Brush) GetValue(ForegroundProperty); } set { SetValueInternal(ForegroundProperty, value); } } ////// PositionStart - int. Default value is 0. /// The codepoint index of the start of the text effect /// public int PositionStart { get { return (int) GetValue(PositionStartProperty); } set { SetValueInternal(PositionStartProperty, value); } } ////// PositionCount - int. Default value is 0. /// The glyph or codepoint count, based on the graunularity /// public int PositionCount { get { return (int) GetValue(PositionCountProperty); } set { SetValueInternal(PositionCountProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new TextEffect(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the TextEffect.Transform property. /// public static readonly DependencyProperty TransformProperty; ////// The DependencyProperty for the TextEffect.Clip property. /// public static readonly DependencyProperty ClipProperty; ////// The DependencyProperty for the TextEffect.Foreground property. /// public static readonly DependencyProperty ForegroundProperty; ////// The DependencyProperty for the TextEffect.PositionStart property. /// public static readonly DependencyProperty PositionStartProperty; ////// The DependencyProperty for the TextEffect.PositionCount property. /// public static readonly DependencyProperty PositionCountProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const int c_PositionStart = 0; internal const int c_PositionCount = 0; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static TextEffect() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(TextEffect); TransformProperty = RegisterProperty("Transform", typeof(Transform), typeofThis, null, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ClipProperty = RegisterProperty("Clip", typeof(Geometry), typeofThis, null, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ForegroundProperty = RegisterProperty("Foreground", typeof(Brush), typeofThis, null, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); PositionStartProperty = RegisterProperty("PositionStart", typeof(int), typeofThis, 0, null, new ValidateValueCallback(ValidatePositionStartValue), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); PositionCountProperty = RegisterProperty("PositionCount", typeof(int), typeofThis, 0, null, new ValidateValueCallback(ValidatePositionCountValue), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class TextEffect : Animatable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new TextEffect Clone() { return (TextEffect)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new TextEffect CloneCurrentValue() { return (TextEffect)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static bool ValidatePositionStartValue(object value) { // This resource needs to be notified on new values being set. if (!OnPositionStartChanging((int) value)) { return false; } return true; } private static bool ValidatePositionCountValue(object value) { // This resource needs to be notified on new values being set. if (!OnPositionCountChanging((int) value)) { return false; } return true; } #region Public Properties ////// Transform - Transform. Default value is null. /// Transform on the text with this effect /// public Transform Transform { get { return (Transform) GetValue(TransformProperty); } set { SetValueInternal(TransformProperty, value); } } ////// Clip - Geometry. Default value is null. /// Clip on the text with this effect /// public Geometry Clip { get { return (Geometry) GetValue(ClipProperty); } set { SetValueInternal(ClipProperty, value); } } ////// Foreground - Brush. Default value is null. /// Foreground for the text with this effect /// public Brush Foreground { get { return (Brush) GetValue(ForegroundProperty); } set { SetValueInternal(ForegroundProperty, value); } } ////// PositionStart - int. Default value is 0. /// The codepoint index of the start of the text effect /// public int PositionStart { get { return (int) GetValue(PositionStartProperty); } set { SetValueInternal(PositionStartProperty, value); } } ////// PositionCount - int. Default value is 0. /// The glyph or codepoint count, based on the graunularity /// public int PositionCount { get { return (int) GetValue(PositionCountProperty); } set { SetValueInternal(PositionCountProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new TextEffect(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the TextEffect.Transform property. /// public static readonly DependencyProperty TransformProperty; ////// The DependencyProperty for the TextEffect.Clip property. /// public static readonly DependencyProperty ClipProperty; ////// The DependencyProperty for the TextEffect.Foreground property. /// public static readonly DependencyProperty ForegroundProperty; ////// The DependencyProperty for the TextEffect.PositionStart property. /// public static readonly DependencyProperty PositionStartProperty; ////// The DependencyProperty for the TextEffect.PositionCount property. /// public static readonly DependencyProperty PositionCountProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const int c_PositionStart = 0; internal const int c_PositionCount = 0; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static TextEffect() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // // Initializations Type typeofThis = typeof(TextEffect); TransformProperty = RegisterProperty("Transform", typeof(Transform), typeofThis, null, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ClipProperty = RegisterProperty("Clip", typeof(Geometry), typeofThis, null, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); ForegroundProperty = RegisterProperty("Foreground", typeof(Brush), typeofThis, null, null, null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); PositionStartProperty = RegisterProperty("PositionStart", typeof(int), typeofThis, 0, null, new ValidateValueCallback(ValidatePositionStartValue), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); PositionCountProperty = RegisterProperty("PositionCount", typeof(int), typeofThis, 0, null, new ValidateValueCallback(ValidatePositionCountValue), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
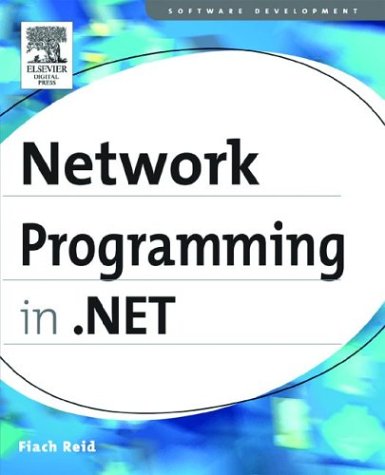
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ValidatingCollection.cs
- OletxDependentTransaction.cs
- ClientTarget.cs
- ConfigurationPropertyAttribute.cs
- DataGridViewMethods.cs
- PeerNodeAddress.cs
- GPRECT.cs
- PackUriHelper.cs
- StsCommunicationException.cs
- HttpStreamXmlDictionaryReader.cs
- FixedTextView.cs
- HiddenFieldPageStatePersister.cs
- InvalidCommandTreeException.cs
- TaiwanCalendar.cs
- SocketManager.cs
- CommentGlyph.cs
- EventSetter.cs
- XsdBuildProvider.cs
- ProcessHost.cs
- TraceXPathNavigator.cs
- RuntimeVariablesExpression.cs
- Missing.cs
- Debug.cs
- SessionStateSection.cs
- PeerToPeerException.cs
- Storyboard.cs
- RtfFormatStack.cs
- DecoderNLS.cs
- AutomationAttributeInfo.cs
- ObjectStateManager.cs
- SoapSchemaMember.cs
- FormsAuthentication.cs
- EdmProperty.cs
- EntityRecordInfo.cs
- PageVisual.cs
- DataGridPreparingCellForEditEventArgs.cs
- EngineSiteSapi.cs
- EditCommandColumn.cs
- BitmapPalettes.cs
- XmlSchemaImporter.cs
- RefreshResponseInfo.cs
- StylusButton.cs
- ListViewAutomationPeer.cs
- NonDualMessageSecurityOverHttp.cs
- LineGeometry.cs
- DocumentPageTextView.cs
- XPathQilFactory.cs
- BoundColumn.cs
- ListViewDataItem.cs
- BoundField.cs
- SelectionItemProviderWrapper.cs
- Padding.cs
- MobilePage.cs
- CommandManager.cs
- BitmapEffectInputConnector.cs
- AnchoredBlock.cs
- IPPacketInformation.cs
- HitTestParameters3D.cs
- FormsIdentity.cs
- SchemaLookupTable.cs
- BatchWriter.cs
- ColorConvertedBitmapExtension.cs
- ImagingCache.cs
- TransformGroup.cs
- URLIdentityPermission.cs
- DialogResultConverter.cs
- BezierSegment.cs
- ListControlDesigner.cs
- BaseResourcesBuildProvider.cs
- Type.cs
- RenderCapability.cs
- XmlBaseWriter.cs
- UriSectionReader.cs
- TextSearch.cs
- ClientUIRequest.cs
- ToolStripDropDownMenu.cs
- OutputBuffer.cs
- SharedDp.cs
- ProfileSettings.cs
- TabRenderer.cs
- ObjectDataSourceChooseMethodsPanel.cs
- ResourceDescriptionAttribute.cs
- FunctionCommandText.cs
- HttpCookie.cs
- ImageAnimator.cs
- MemberMaps.cs
- StrokeFIndices.cs
- XamlPoint3DCollectionSerializer.cs
- ProfileProvider.cs
- OperatingSystemVersionCheck.cs
- Duration.cs
- BasicExpressionVisitor.cs
- NestPullup.cs
- Drawing.cs
- RoleGroupCollection.cs
- ParallelTimeline.cs
- SymbolMethod.cs
- FilterQueryOptionExpression.cs
- EntityProviderFactory.cs
- ResourceExpressionBuilder.cs