Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / ParallelTimeline.cs / 1305600 / ParallelTimeline.cs
//---------------------------------------------------------------------------- //// Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.ComponentModel; namespace System.Windows.Media.Animation { ////// This class represents a group of Timelines where the children /// become active according to the value of their Begin property rather /// than their specific order in the Children collection. Children /// are also able to overlap and run in parallel with each other. /// public partial class ParallelTimeline : TimelineGroup { #region Constructors ////// Creates a ParallelTimeline with default properties. /// public ParallelTimeline() : base() { } ////// Creates a ParallelTimeline with the specified BeginTime. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime) : base(beginTime) { } ////// Creates a ParallelTimeline with the specified begin time and duration. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// /// /// The simple Duration of this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime, Duration duration) : base(beginTime, duration) { } ////// Creates a ParallelTimeline with the specified BeginTime, Duration and RepeatBehavior. /// /// /// The scheduled BeginTime for this ParallelTimeline. /// /// /// The simple Duration of this ParallelTimeline. /// /// /// The RepeatBehavior for this ParallelTimeline. /// public ParallelTimeline(TimeSpan? beginTime, Duration duration, RepeatBehavior repeatBehavior) : base(beginTime, duration, repeatBehavior) { } #endregion #region Methods ////// Return the duration from a specific clock /// /// /// The Clock whose natural duration is desired. /// ////// A Duration quantity representing the natural duration. /// protected override Duration GetNaturalDurationCore(Clock clock) { Duration simpleDuration = TimeSpan.Zero; ClockGroup clockGroup = clock as ClockGroup; if (clockGroup != null) { Listchildren = clockGroup.InternalChildren; // The container ends when all of its children have ended at least // one of their active periods. if (children != null) { bool hasChildWithUnresolvedDuration = false; for (int childIndex = 0; childIndex < children.Count; childIndex++) { Duration childEndOfActivePeriod = children[childIndex].EndOfActivePeriod; if (childEndOfActivePeriod == Duration.Forever) { // If we have even one child with a duration of forever // our resolved duration will also be forever. It doesn't // matter if other children have unresolved durations. return Duration.Forever; } else if (childEndOfActivePeriod == Duration.Automatic) { hasChildWithUnresolvedDuration = true; } else if (childEndOfActivePeriod > simpleDuration) { simpleDuration = childEndOfActivePeriod; } } // We've iterated through all our children. We know that at this // point none of them have a duration of Forever or we would have // returned already. If any of them still have unresolved // durations then our duration is also still unresolved and we // will return automatic. Otherwise, we'll fall out of the 'if' // block and return the simpleDuration as our final resolved // duration. if (hasChildWithUnresolvedDuration) { return Duration.Automatic; } } } return simpleDuration; } #endregion #region SlipBehavior Property /// /// SlipBehavior Property /// public static readonly DependencyProperty SlipBehaviorProperty = DependencyProperty.Register( "SlipBehavior", typeof(SlipBehavior), typeof(ParallelTimeline), new PropertyMetadata( SlipBehavior.Grow, new PropertyChangedCallback(ParallelTimeline_PropertyChangedFunction)), new ValidateValueCallback(ValidateSlipBehavior)); private static bool ValidateSlipBehavior(object value) { return TimeEnumHelper.IsValidSlipBehavior((SlipBehavior)value); } ////// Returns the SlipBehavior for this ClockGroup /// [DefaultValue(SlipBehavior.Grow)] public SlipBehavior SlipBehavior { get { return (SlipBehavior)GetValue(SlipBehaviorProperty); } set { SetValue(SlipBehaviorProperty, value); } } internal static void ParallelTimeline_PropertyChangedFunction(DependencyObject d, DependencyPropertyChangedEventArgs e) { ((ParallelTimeline)d).PropertyChanged(e.Property); } #endregion // SlipBehavior Property } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
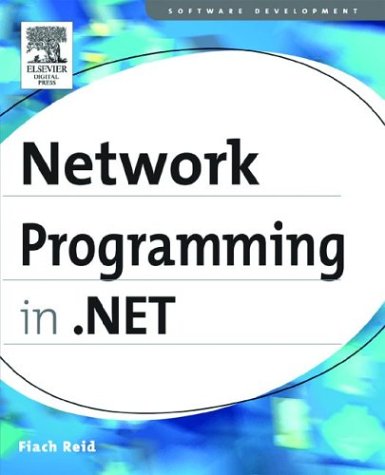
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LicFileLicenseProvider.cs
- ADMembershipUser.cs
- WizardForm.cs
- XmlDataDocument.cs
- BasicExpandProvider.cs
- AssemblySettingAttributes.cs
- PrintController.cs
- DataGridViewRowCollection.cs
- Latin1Encoding.cs
- DecimalFormatter.cs
- SQLGuid.cs
- RegexMatchCollection.cs
- AmbientProperties.cs
- NotSupportedException.cs
- CqlGenerator.cs
- EndpointDiscoveryElement.cs
- UseLicense.cs
- HttpHandlersSection.cs
- FreezableCollection.cs
- ExpressionBuilderContext.cs
- DataGridHelper.cs
- ScalarType.cs
- XmlHierarchicalDataSourceView.cs
- StatusBarPanelClickEvent.cs
- SingleQueryOperator.cs
- SctClaimDictionary.cs
- AddInServer.cs
- ByteRangeDownloader.cs
- FontStretch.cs
- DesignTimeValidationFeature.cs
- SyntaxCheck.cs
- ColumnResult.cs
- SoapExtensionTypeElement.cs
- ExpressionNode.cs
- ClientBuildManager.cs
- DocumentApplicationJournalEntry.cs
- ResourceIDHelper.cs
- ViewStateException.cs
- TextWriter.cs
- ScopedKnownTypes.cs
- BuildProviderCollection.cs
- Button.cs
- SecurityTokenSerializer.cs
- PageThemeParser.cs
- WindowsStatusBar.cs
- DataGridViewSortCompareEventArgs.cs
- ContainerUtilities.cs
- ServiceModelExtensionElement.cs
- Menu.cs
- Rules.cs
- ResourceProviderFactory.cs
- CssTextWriter.cs
- PointAnimationClockResource.cs
- Group.cs
- Stack.cs
- SetStoryboardSpeedRatio.cs
- MetadataCache.cs
- Utils.cs
- CrossSiteScriptingValidation.cs
- XPathParser.cs
- LayoutSettings.cs
- MenuItemBinding.cs
- AlphaSortedEnumConverter.cs
- WSSecurityOneDotOneReceiveSecurityHeader.cs
- TripleDESCryptoServiceProvider.cs
- AnonymousIdentificationModule.cs
- ToolBar.cs
- EndpointDiscoveryMetadata11.cs
- AssemblyBuilder.cs
- QueryBranchOp.cs
- ObjectKeyFrameCollection.cs
- PenThreadWorker.cs
- TypeConverterHelper.cs
- DataTableReader.cs
- ModuleConfigurationInfo.cs
- BigInt.cs
- AnimationClock.cs
- PeerName.cs
- NativeMethods.cs
- QueryOperationResponseOfT.cs
- SoapFault.cs
- Rule.cs
- MatrixIndependentAnimationStorage.cs
- XhtmlBasicCalendarAdapter.cs
- WindowManager.cs
- DATA_BLOB.cs
- MembershipUser.cs
- QilExpression.cs
- AnnotationMap.cs
- Literal.cs
- CodeActivityMetadata.cs
- ProfileManager.cs
- GeneralTransform3DGroup.cs
- wgx_commands.cs
- Item.cs
- WebEventTraceProvider.cs
- XmlSiteMapProvider.cs
- FixedDocument.cs
- DateTimeOffset.cs
- RequiredFieldValidator.cs