Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / SQLTypes / SQLResource.cs / 1305376 / SQLResource.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SQLResource.cs // // Create by: JunFang // // Purpose: Implementation of utilities in COM+ SQL Types Library. // Includes interface INullable, exceptions SqlNullValueException // and SqlTruncateException, and SQLDebug class. // // Notes: // // History: // // 10/22/99 JunFang Created. // // @EndHeader@ //************************************************************************* namespace System.Data.SqlTypes { using System; using System.Data; using System.Globalization; internal sealed class SQLResource { private SQLResource() { /* prevent utility class from being insantiated*/ } internal static readonly String NullString = Res.GetString(Res.SqlMisc_NullString); internal static readonly String MessageString = Res.GetString(Res.SqlMisc_MessageString); internal static readonly String ArithOverflowMessage = Res.GetString(Res.SqlMisc_ArithOverflowMessage); internal static readonly String DivideByZeroMessage = Res.GetString(Res.SqlMisc_DivideByZeroMessage); internal static readonly String NullValueMessage = Res.GetString(Res.SqlMisc_NullValueMessage); internal static readonly String TruncationMessage = Res.GetString(Res.SqlMisc_TruncationMessage); internal static readonly String DateTimeOverflowMessage = Res.GetString(Res.SqlMisc_DateTimeOverflowMessage); internal static readonly String ConcatDiffCollationMessage = Res.GetString(Res.SqlMisc_ConcatDiffCollationMessage); internal static readonly String CompareDiffCollationMessage = Res.GetString(Res.SqlMisc_CompareDiffCollationMessage); internal static readonly String InvalidFlagMessage = Res.GetString(Res.SqlMisc_InvalidFlagMessage); internal static readonly String NumeToDecOverflowMessage = Res.GetString(Res.SqlMisc_NumeToDecOverflowMessage); internal static readonly String ConversionOverflowMessage = Res.GetString(Res.SqlMisc_ConversionOverflowMessage); internal static readonly String InvalidDateTimeMessage = Res.GetString(Res.SqlMisc_InvalidDateTimeMessage); internal static readonly String TimeZoneSpecifiedMessage = Res.GetString(Res.SqlMisc_TimeZoneSpecifiedMessage); internal static readonly String InvalidArraySizeMessage = Res.GetString(Res.SqlMisc_InvalidArraySizeMessage); internal static readonly String InvalidPrecScaleMessage = Res.GetString(Res.SqlMisc_InvalidPrecScaleMessage); internal static readonly String FormatMessage = Res.GetString(Res.SqlMisc_FormatMessage); internal static readonly String NotFilledMessage = Res.GetString(Res.SqlMisc_NotFilledMessage); internal static readonly String AlreadyFilledMessage = Res.GetString(Res.SqlMisc_AlreadyFilledMessage); internal static readonly String ClosedXmlReaderMessage = Res.GetString(Res.SqlMisc_ClosedXmlReaderMessage); internal static String InvalidOpStreamClosed(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamClosed, method); } internal static String InvalidOpStreamNonWritable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonWritable, method); } internal static String InvalidOpStreamNonReadable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonReadable, method); } internal static String InvalidOpStreamNonSeekable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonSeekable, method); } } // SqlResource } // namespace System // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SQLResource.cs // // Create by: JunFang // // Purpose: Implementation of utilities in COM+ SQL Types Library. // Includes interface INullable, exceptions SqlNullValueException // and SqlTruncateException, and SQLDebug class. // // Notes: // // History: // // 10/22/99 JunFang Created. // // @EndHeader@ //************************************************************************* namespace System.Data.SqlTypes { using System; using System.Data; using System.Globalization; internal sealed class SQLResource { private SQLResource() { /* prevent utility class from being insantiated*/ } internal static readonly String NullString = Res.GetString(Res.SqlMisc_NullString); internal static readonly String MessageString = Res.GetString(Res.SqlMisc_MessageString); internal static readonly String ArithOverflowMessage = Res.GetString(Res.SqlMisc_ArithOverflowMessage); internal static readonly String DivideByZeroMessage = Res.GetString(Res.SqlMisc_DivideByZeroMessage); internal static readonly String NullValueMessage = Res.GetString(Res.SqlMisc_NullValueMessage); internal static readonly String TruncationMessage = Res.GetString(Res.SqlMisc_TruncationMessage); internal static readonly String DateTimeOverflowMessage = Res.GetString(Res.SqlMisc_DateTimeOverflowMessage); internal static readonly String ConcatDiffCollationMessage = Res.GetString(Res.SqlMisc_ConcatDiffCollationMessage); internal static readonly String CompareDiffCollationMessage = Res.GetString(Res.SqlMisc_CompareDiffCollationMessage); internal static readonly String InvalidFlagMessage = Res.GetString(Res.SqlMisc_InvalidFlagMessage); internal static readonly String NumeToDecOverflowMessage = Res.GetString(Res.SqlMisc_NumeToDecOverflowMessage); internal static readonly String ConversionOverflowMessage = Res.GetString(Res.SqlMisc_ConversionOverflowMessage); internal static readonly String InvalidDateTimeMessage = Res.GetString(Res.SqlMisc_InvalidDateTimeMessage); internal static readonly String TimeZoneSpecifiedMessage = Res.GetString(Res.SqlMisc_TimeZoneSpecifiedMessage); internal static readonly String InvalidArraySizeMessage = Res.GetString(Res.SqlMisc_InvalidArraySizeMessage); internal static readonly String InvalidPrecScaleMessage = Res.GetString(Res.SqlMisc_InvalidPrecScaleMessage); internal static readonly String FormatMessage = Res.GetString(Res.SqlMisc_FormatMessage); internal static readonly String NotFilledMessage = Res.GetString(Res.SqlMisc_NotFilledMessage); internal static readonly String AlreadyFilledMessage = Res.GetString(Res.SqlMisc_AlreadyFilledMessage); internal static readonly String ClosedXmlReaderMessage = Res.GetString(Res.SqlMisc_ClosedXmlReaderMessage); internal static String InvalidOpStreamClosed(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamClosed, method); } internal static String InvalidOpStreamNonWritable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonWritable, method); } internal static String InvalidOpStreamNonReadable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonReadable, method); } internal static String InvalidOpStreamNonSeekable(String method) { return Res.GetString(Res.SqlMisc_InvalidOpStreamNonSeekable, method); } } // SqlResource } // namespace System // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
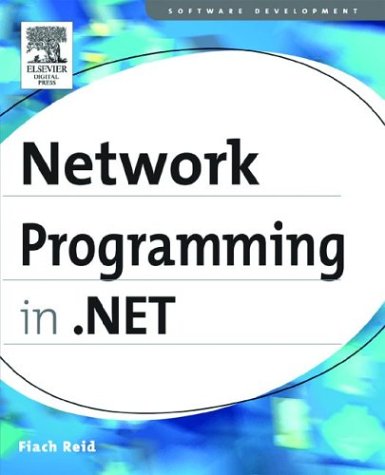
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlInputPassword.cs
- FileDetails.cs
- CodeThrowExceptionStatement.cs
- KnownBoxes.cs
- Cell.cs
- ProtocolsConfigurationHandler.cs
- IPipelineRuntime.cs
- _StreamFramer.cs
- ValueChangedEventManager.cs
- SubclassTypeValidatorAttribute.cs
- Application.cs
- DataSourceView.cs
- SchemaMapping.cs
- ThreadNeutralSemaphore.cs
- MissingSatelliteAssemblyException.cs
- JournalNavigationScope.cs
- Models.cs
- ClusterSafeNativeMethods.cs
- DataGridViewColumnCollection.cs
- GlyphShapingProperties.cs
- UpdatePanel.cs
- DocumentGrid.cs
- SiteIdentityPermission.cs
- MatrixConverter.cs
- SqlGatherProducedAliases.cs
- UrlUtility.cs
- Parser.cs
- BitVector32.cs
- TakeQueryOptionExpression.cs
- XmlSchemaRedefine.cs
- IOThreadScheduler.cs
- ObjectQuery.cs
- SimpleRecyclingCache.cs
- XmlQueryRuntime.cs
- ExpressionHelper.cs
- FileLogRecordStream.cs
- BookmarkTable.cs
- SQLByteStorage.cs
- Matrix3D.cs
- SqlProviderManifest.cs
- ComplusTypeValidator.cs
- WindowsBrush.cs
- ValidatingReaderNodeData.cs
- RoleGroup.cs
- EntityRecordInfo.cs
- SequentialUshortCollection.cs
- AccessorTable.cs
- SizeValueSerializer.cs
- CodeRegionDirective.cs
- SelectionUIService.cs
- DecoratedNameAttribute.cs
- IndexedEnumerable.cs
- CodePageEncoding.cs
- Collection.cs
- Pipe.cs
- ConvertEvent.cs
- DbFunctionCommandTree.cs
- MLangCodePageEncoding.cs
- TemplateBindingExtensionConverter.cs
- Comparer.cs
- ConnectionPoolManager.cs
- LicenseManager.cs
- BaseTemplateParser.cs
- LightweightCodeGenerator.cs
- DecoderExceptionFallback.cs
- DuplexClientBase.cs
- HttpListenerContext.cs
- ValidationRule.cs
- AttachmentCollection.cs
- CheckBoxField.cs
- TextElement.cs
- HttpApplicationFactory.cs
- ObjectIDGenerator.cs
- _NegotiateClient.cs
- RuntimeUtils.cs
- StylusButton.cs
- GreaterThan.cs
- CodeDirectionExpression.cs
- CodeCompiler.cs
- VectorCollectionConverter.cs
- ValidatingCollection.cs
- StreamReader.cs
- ParserHooks.cs
- XamlTypeMapper.cs
- DispatcherExceptionFilterEventArgs.cs
- EntityViewGenerationConstants.cs
- AsnEncodedData.cs
- ObjectStorage.cs
- DrawingBrush.cs
- BrushValueSerializer.cs
- SerialStream.cs
- MailBnfHelper.cs
- ApplicationDirectory.cs
- _Connection.cs
- FixedNode.cs
- FileClassifier.cs
- BoundingRectTracker.cs
- DocumentPaginator.cs
- Debug.cs
- SynchronizationContext.cs