Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / IdleTimeoutMonitor.cs / 1 / IdleTimeoutMonitor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // check if there are active requests if (HostingEnvironment.BusyCount != 0) return; // check if enough time passed if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // check if debugger is attached if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdown(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Hosting; using System.Web.Util; internal class IdleTimeoutMonitor { private TimeSpan _idleTimeout; // the timeout value private DateTime _lastEvent; // idle since this time private Timer _timer; private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 30); // 30 secs internal IdleTimeoutMonitor(TimeSpan timeout) { _idleTimeout = timeout; _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); _lastEvent = DateTime.UtcNow; } internal void Stop() { // stop the timer if (_timer != null) { lock (this) { if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } } } } internal DateTime LastEvent { // thread-safe property get { DateTime t; lock (this) { t = _lastEvent; } return t; } set { lock (this) { _lastEvent = value; } } } private void TimerCompletionCallback(Object state) { // user idle timer to trim the free list of app instanced HttpApplicationFactory.TrimApplicationInstances(); // no idle timeout if (_idleTimeout == TimeSpan.MaxValue) return; // don't do idle timeout if already shutting down if (HostingEnvironment.ShutdownInitiated) return; // check if there are active requests if (HostingEnvironment.BusyCount != 0) return; // check if enough time passed if (DateTime.UtcNow <= LastEvent.Add(_idleTimeout)) return; // check if debugger is attached if (System.Diagnostics.Debugger.IsAttached) return; // shutdown HttpRuntime.SetShutdownReason(ApplicationShutdownReason.IdleTimeout, SR.GetString(SR.Hosting_Env_IdleTimeout)); HostingEnvironment.InitiateShutdown(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
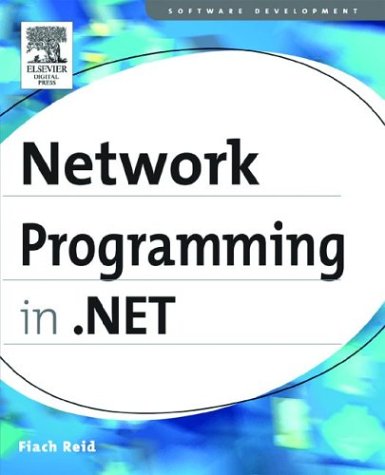
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OperationPickerDialog.cs
- Rotation3D.cs
- WindowsListView.cs
- ClickablePoint.cs
- DispatcherSynchronizationContext.cs
- ContainerControl.cs
- Action.cs
- InfiniteIntConverter.cs
- WinFormsUtils.cs
- Polyline.cs
- InterleavedZipPartStream.cs
- SchemaEntity.cs
- Convert.cs
- DocumentSequence.cs
- Button.cs
- Int32Rect.cs
- SegmentInfo.cs
- DefaultBinder.cs
- MasterPageParser.cs
- ZipIOExtraFieldZip64Element.cs
- HyperLink.cs
- WebPartUserCapability.cs
- ToolStripComboBox.cs
- ReadOnlyHierarchicalDataSourceView.cs
- MembershipSection.cs
- Buffer.cs
- SortQuery.cs
- ControlBuilder.cs
- AspNetSynchronizationContext.cs
- WebResourceAttribute.cs
- ServiceDiscoveryElement.cs
- MetadataWorkspace.cs
- FileSystemEnumerable.cs
- TraceHandlerErrorFormatter.cs
- NotifyIcon.cs
- AssociatedControlConverter.cs
- UInt16.cs
- MenuItem.cs
- DataGridTablesFactory.cs
- WebResourceUtil.cs
- ADMembershipUser.cs
- Invariant.cs
- GlobalEventManager.cs
- DbMetaDataCollectionNames.cs
- ListBindableAttribute.cs
- AnonymousIdentificationSection.cs
- StringArrayConverter.cs
- ListViewEditEventArgs.cs
- CssClassPropertyAttribute.cs
- ToolStripAdornerWindowService.cs
- UrlPropertyAttribute.cs
- WindowsContainer.cs
- UnmanagedMarshal.cs
- DocumentXPathNavigator.cs
- RouteData.cs
- BindingWorker.cs
- SafePointer.cs
- MissingSatelliteAssemblyException.cs
- LoginDesigner.cs
- Char.cs
- BuildManagerHost.cs
- EntityContainerEntitySetDefiningQuery.cs
- SafeFileHandle.cs
- OutOfProcStateClientManager.cs
- PropertyEmitter.cs
- IndentedTextWriter.cs
- ToolBarTray.cs
- NativeCppClassAttribute.cs
- TargetControlTypeAttribute.cs
- DrawingVisual.cs
- Bitmap.cs
- DiscoveryUtility.cs
- XmlAttribute.cs
- CaretElement.cs
- ParallelTimeline.cs
- AssemblyCache.cs
- XmlNodeList.cs
- DataSourceCache.cs
- EventLogLink.cs
- NestedContainer.cs
- DynamicValueConverter.cs
- GeometryCollection.cs
- WebSysDefaultValueAttribute.cs
- TimelineClockCollection.cs
- WebPartUserCapability.cs
- CfgSemanticTag.cs
- Table.cs
- ValidationPropertyAttribute.cs
- SpotLight.cs
- CompatibleComparer.cs
- EntityObject.cs
- RSAOAEPKeyExchangeFormatter.cs
- SqlReorderer.cs
- WindowsFont.cs
- SQLDateTimeStorage.cs
- _emptywebproxy.cs
- BinaryConverter.cs
- SqlDependencyUtils.cs
- AuthorizationSection.cs
- CompilerGlobalScopeAttribute.cs