Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / Win32Providers / MS / Internal / AutomationProxies / WindowsHyperlink.cs / 1305600 / WindowsHyperlink.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Windows Hyperlink Proxy // // History: // 07/01/2003 : a-jeanp Created //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.ComponentModel; using System.Text; using System.Collections; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows; using MS.Win32; namespace MS.Internal.AutomationProxies { // Implementation of the Hyperlink (SysLink) proxy. class WindowsHyperlink: ProxyHwnd { // ----------------------------------------------------- // // Constructors // // ----------------------------------------------------- #region Constructors WindowsHyperlink (IntPtr hwnd, ProxyFragment parent, int item) : base( hwnd, parent, item) { // Set the strings to return properly the properties. _cControlType = ControlType.Hyperlink; // support for events _createOnEvent = new WinEventTracker.ProxyRaiseEvents(RaiseEvents); } #endregion Constructors #region Proxy Create // Static Create method called by UIAutomation to create this proxy. //= 0 && GetLinkItem (HitTestInfo.item.iLink)) { return CreateHyperlinkItem (_linkItem, HitTestInfo.item.iLink); } return base.ElementProviderFromPoint (x, y); } // Returns an item corresponding to the focused element (if there is one), // or null otherwise. internal override ProxySimple GetFocus () { // // @ for (int iCurrentItem = 0; GetLinkItem(iCurrentItem); iCurrentItem++) { // If the item was focused... if (Misc.IsBitSet(_linkItem.state, NativeMethods.LIS_FOCUSED)) { return CreateHyperlinkItem(_linkItem, iCurrentItem); } } return null; } #endregion ProxyFragment Interface // ------------------------------------------------------ // // Private Methods // // ------------------------------------------------------ #region Private Methods // Copy creator for a link or link item. private ProxySimple CreateHyperlinkItem(UnsafeNativeMethods.LITEM linkItem, int index) { return new WindowsHyperlinkItem(_hwnd, this, index); } private bool GetLinkItem (int item) { if (item < 0) { return false; } // Set the members about which we care. _linkItem.mask = NativeMethods.LIF_ITEMINDEX | NativeMethods.LIF_STATE; _linkItem.iLink = item; _linkItem.state = 0; _linkItem.stateMask = NativeMethods.LIS_ENABLED; unsafe { fixed (UnsafeNativeMethods.LITEM* pLinkItem = &_linkItem) { return XSendMessage.XSend(_hwnd, NativeMethods.LM_GETITEM, IntPtr.Zero, new IntPtr(pLinkItem), sizeof(UnsafeNativeMethods.LITEM)); } } } private string RemoveHTMLAnchorTag(string text) { // If there are no anchor tag then it's ok just return it if (text.IndexOf("') { inAnchorMode = false; } } return new string(ach, 0, dest); } #endregion Private Methods // ----------------------------------------------------- // // Private Fields // // ------------------------------------------------------ #region Private Fields // Temporary variable used all over the proxy private UnsafeNativeMethods.LITEM _linkItem; #endregion Private Fields } // ----------------------------------------------------- // // WindowsHyperlinkItem Class // //----------------------------------------------------- // Implementation of the PAW WindowsHyperlinkItem (SysLink) proxy. class WindowsHyperlinkItem : ProxySimple, IInvokeProvider { // ----------------------------------------------------- // // Constructors // // ------------------------------------------------------ #region Constructors // Constructor. internal WindowsHyperlinkItem(IntPtr hwnd, ProxyFragment parent, int item) : base( hwnd, parent, item) { // Set the strings to return properly the properties. _cControlType = ControlType.Hyperlink; } #endregion Constructors //----------------------------------------------------- // // Patterns Implementation // //------------------------------------------------------ #region ProxySimple Interface // Returns a pattern interface if supported. internal override object GetPatternProvider(AutomationPattern iid) { return iid == InvokePattern.Pattern ? this : null; } // Sets the focus to this item. internal override bool SetFocus() { // // Send the link an LM_SETITEM message. // // Allocate a local LITEM struct. UnsafeNativeMethods.LITEM linkItem = new UnsafeNativeMethods.LITEM(); // Fill in the coordinates about which we care. linkItem.mask = NativeMethods.LIF_ITEMINDEX | NativeMethods.LIF_STATE; linkItem.iLink = _item; linkItem.stateMask = NativeMethods.LIS_FOCUSED; linkItem.state = NativeMethods.LIS_FOCUSED; unsafe { // Send the LM_SETITEM message. return XSendMessage.XSend(_hwnd, NativeMethods.LM_SETITEM, IntPtr.Zero, new IntPtr(&linkItem), Marshal.SizeOf(linkItem.GetType())); } } //Gets the localized name internal override string LocalizedName { get { // Cannot get the associated with each each hyperlink (within ) return ""; } } #endregion ProxySimple Interface #region Invoke Pattern // Same as clicking on an hyperlink void IInvokeProvider.Invoke() { // Check that button can be clicked. // // @ // Make sure that the control is enabled if (!SafeNativeMethods.IsWindowEnabled(_hwnd)) { throw new ElementNotEnabledException(); } if (!SafeNativeMethods.IsWindowVisible(_hwnd)) { throw new InvalidOperationException(SR.Get(SRID.OperationCannotBePerformed)); } // // Get the bounding rect for the window. // NativeMethods.Win32Rect BoundingRect = NativeMethods.Win32Rect.Empty; if (!Misc.GetWindowRect(_hwnd, ref BoundingRect)) { return; } // // All we really need here are the height and the width, // so we don't even need to translate between screen // and client coordinates. // int width = BoundingRect.right - BoundingRect.left; int height = BoundingRect.bottom - BoundingRect.top; // // Determine the point to click. // // @ for (int Resolution = 10; Resolution > 0; --Resolution) { for (int x = 1; x <= width; x += Resolution) { for (int y = 1; y <= height; y += Resolution) { // // Send the link an LM_HITTEST message. // // Allocate a local hit test info struct. UnsafeNativeMethods.LHITTESTINFO HitTestInfo = new UnsafeNativeMethods.LHITTESTINFO(); // Fill in the coordinates that we want to check. HitTestInfo.pt.x = x; HitTestInfo.pt.y = y; // Fill in index and state info. HitTestInfo.item.mask = NativeMethods.LIF_ITEMINDEX | NativeMethods.LIF_STATE; HitTestInfo.item.iLink = 0; HitTestInfo.item.stateMask = NativeMethods.LIS_ENABLED; HitTestInfo.item.state = 0; bool bGetItemResult; unsafe { // Send the LM_HITTEST message. bGetItemResult = XSendMessage.XSend(_hwnd, NativeMethods.LM_HITTEST, IntPtr.Zero, new IntPtr(&HitTestInfo), Marshal.SizeOf(HitTestInfo.GetType())); } if (bGetItemResult == true && HitTestInfo.item.iLink == _item) { // // N.B. [SEdmison]: // This multiplication is essentially just // a left shift by one word's width; in // Win32 I'd just use my trusty MAKELONG macro, // but C# doesn't give me that option. // Misc.ProxySendMessage(_hwnd, NativeMethods.WM_LBUTTONDOWN, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); Misc.ProxySendMessage(_hwnd, NativeMethods.WM_LBUTTONUP, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return; } } } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Windows Hyperlink Proxy // // History: // 07/01/2003 : a-jeanp Created //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.ComponentModel; using System.Text; using System.Collections; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows; using MS.Win32; namespace MS.Internal.AutomationProxies { // Implementation of the Hyperlink (SysLink) proxy. class WindowsHyperlink: ProxyHwnd { // ----------------------------------------------------- // // Constructors // // ----------------------------------------------------- #region Constructors WindowsHyperlink (IntPtr hwnd, ProxyFragment parent, int item) : base( hwnd, parent, item) { // Set the strings to return properly the properties. _cControlType = ControlType.Hyperlink; // support for events _createOnEvent = new WinEventTracker.ProxyRaiseEvents(RaiseEvents); } #endregion Constructors #region Proxy Create // Static Create method called by UIAutomation to create this proxy. //= 0 && GetLinkItem (HitTestInfo.item.iLink)) { return CreateHyperlinkItem (_linkItem, HitTestInfo.item.iLink); } return base.ElementProviderFromPoint (x, y); } // Returns an item corresponding to the focused element (if there is one), // or null otherwise. internal override ProxySimple GetFocus () { // // @ for (int iCurrentItem = 0; GetLinkItem(iCurrentItem); iCurrentItem++) { // If the item was focused... if (Misc.IsBitSet(_linkItem.state, NativeMethods.LIS_FOCUSED)) { return CreateHyperlinkItem(_linkItem, iCurrentItem); } } return null; } #endregion ProxyFragment Interface // ------------------------------------------------------ // // Private Methods // // ------------------------------------------------------ #region Private Methods // Copy creator for a link or link item. private ProxySimple CreateHyperlinkItem(UnsafeNativeMethods.LITEM linkItem, int index) { return new WindowsHyperlinkItem(_hwnd, this, index); } private bool GetLinkItem (int item) { if (item < 0) { return false; } // Set the members about which we care. _linkItem.mask = NativeMethods.LIF_ITEMINDEX | NativeMethods.LIF_STATE; _linkItem.iLink = item; _linkItem.state = 0; _linkItem.stateMask = NativeMethods.LIS_ENABLED; unsafe { fixed (UnsafeNativeMethods.LITEM* pLinkItem = &_linkItem) { return XSendMessage.XSend(_hwnd, NativeMethods.LM_GETITEM, IntPtr.Zero, new IntPtr(pLinkItem), sizeof(UnsafeNativeMethods.LITEM)); } } } private string RemoveHTMLAnchorTag(string text) { // If there are no anchor tag then it's ok just return it if (text.IndexOf("') { inAnchorMode = false; } } return new string(ach, 0, dest); } #endregion Private Methods // ----------------------------------------------------- // // Private Fields // // ------------------------------------------------------ #region Private Fields // Temporary variable used all over the proxy private UnsafeNativeMethods.LITEM _linkItem; #endregion Private Fields } // ----------------------------------------------------- // // WindowsHyperlinkItem Class // //----------------------------------------------------- // Implementation of the PAW WindowsHyperlinkItem (SysLink) proxy. class WindowsHyperlinkItem : ProxySimple, IInvokeProvider { // ----------------------------------------------------- // // Constructors // // ------------------------------------------------------ #region Constructors // Constructor. internal WindowsHyperlinkItem(IntPtr hwnd, ProxyFragment parent, int item) : base( hwnd, parent, item) { // Set the strings to return properly the properties. _cControlType = ControlType.Hyperlink; } #endregion Constructors //----------------------------------------------------- // // Patterns Implementation // //------------------------------------------------------ #region ProxySimple Interface // Returns a pattern interface if supported. internal override object GetPatternProvider(AutomationPattern iid) { return iid == InvokePattern.Pattern ? this : null; } // Sets the focus to this item. internal override bool SetFocus() { // // Send the link an LM_SETITEM message. // // Allocate a local LITEM struct. UnsafeNativeMethods.LITEM linkItem = new UnsafeNativeMethods.LITEM(); // Fill in the coordinates about which we care. linkItem.mask = NativeMethods.LIF_ITEMINDEX | NativeMethods.LIF_STATE; linkItem.iLink = _item; linkItem.stateMask = NativeMethods.LIS_FOCUSED; linkItem.state = NativeMethods.LIS_FOCUSED; unsafe { // Send the LM_SETITEM message. return XSendMessage.XSend(_hwnd, NativeMethods.LM_SETITEM, IntPtr.Zero, new IntPtr(&linkItem), Marshal.SizeOf(linkItem.GetType())); } } //Gets the localized name internal override string LocalizedName { get { // Cannot get the associated with each each hyperlink (within ) return ""; } } #endregion ProxySimple Interface #region Invoke Pattern // Same as clicking on an hyperlink void IInvokeProvider.Invoke() { // Check that button can be clicked. // // @ // Make sure that the control is enabled if (!SafeNativeMethods.IsWindowEnabled(_hwnd)) { throw new ElementNotEnabledException(); } if (!SafeNativeMethods.IsWindowVisible(_hwnd)) { throw new InvalidOperationException(SR.Get(SRID.OperationCannotBePerformed)); } // // Get the bounding rect for the window. // NativeMethods.Win32Rect BoundingRect = NativeMethods.Win32Rect.Empty; if (!Misc.GetWindowRect(_hwnd, ref BoundingRect)) { return; } // // All we really need here are the height and the width, // so we don't even need to translate between screen // and client coordinates. // int width = BoundingRect.right - BoundingRect.left; int height = BoundingRect.bottom - BoundingRect.top; // // Determine the point to click. // // @ for (int Resolution = 10; Resolution > 0; --Resolution) { for (int x = 1; x <= width; x += Resolution) { for (int y = 1; y <= height; y += Resolution) { // // Send the link an LM_HITTEST message. // // Allocate a local hit test info struct. UnsafeNativeMethods.LHITTESTINFO HitTestInfo = new UnsafeNativeMethods.LHITTESTINFO(); // Fill in the coordinates that we want to check. HitTestInfo.pt.x = x; HitTestInfo.pt.y = y; // Fill in index and state info. HitTestInfo.item.mask = NativeMethods.LIF_ITEMINDEX | NativeMethods.LIF_STATE; HitTestInfo.item.iLink = 0; HitTestInfo.item.stateMask = NativeMethods.LIS_ENABLED; HitTestInfo.item.state = 0; bool bGetItemResult; unsafe { // Send the LM_HITTEST message. bGetItemResult = XSendMessage.XSend(_hwnd, NativeMethods.LM_HITTEST, IntPtr.Zero, new IntPtr(&HitTestInfo), Marshal.SizeOf(HitTestInfo.GetType())); } if (bGetItemResult == true && HitTestInfo.item.iLink == _item) { // // N.B. [SEdmison]: // This multiplication is essentially just // a left shift by one word's width; in // Win32 I'd just use my trusty MAKELONG macro, // but C# doesn't give me that option. // Misc.ProxySendMessage(_hwnd, NativeMethods.WM_LBUTTONDOWN, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); Misc.ProxySendMessage(_hwnd, NativeMethods.WM_LBUTTONUP, IntPtr.Zero, NativeMethods.Util.MAKELPARAM(x, y)); return; } } } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
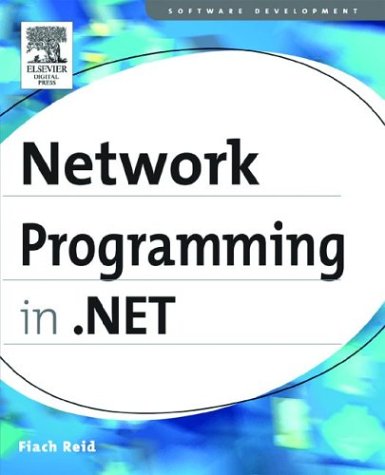
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ApplicationActivator.cs
- ListViewInsertEventArgs.cs
- MetadataItemEmitter.cs
- Attribute.cs
- GridViewAutomationPeer.cs
- ConfigurationElementProperty.cs
- ContractListAdapter.cs
- RunWorkerCompletedEventArgs.cs
- ListBoxAutomationPeer.cs
- BindingManagerDataErrorEventArgs.cs
- FormView.cs
- CheckBoxStandardAdapter.cs
- _BufferOffsetSize.cs
- DefaultWorkflowSchedulerService.cs
- CommandPlan.cs
- FillRuleValidation.cs
- ExpressionBuilderContext.cs
- WebPartConnection.cs
- XmlWrappingReader.cs
- DataTrigger.cs
- followingquery.cs
- DateTimePicker.cs
- ProfileParameter.cs
- WhiteSpaceTrimStringConverter.cs
- MetadataSet.cs
- PingReply.cs
- HtmlHead.cs
- SerializationEventsCache.cs
- PeerTransportSecuritySettings.cs
- UniqueIdentifierService.cs
- Rectangle.cs
- Table.cs
- ConnectionOrientedTransportBindingElement.cs
- ListenUriMode.cs
- DoubleLink.cs
- ControlLocalizer.cs
- SystemIcons.cs
- ObjectQueryProvider.cs
- Helper.cs
- ExpressionBinding.cs
- RewritingValidator.cs
- FileSystemWatcher.cs
- ManagementInstaller.cs
- ObjectStateEntry.cs
- StringConcat.cs
- SqlDataSourceQueryEditor.cs
- StrokeNodeData.cs
- MetadataCacheItem.cs
- _TransmitFileOverlappedAsyncResult.cs
- RequestQueryProcessor.cs
- DescendantQuery.cs
- ResourceDisplayNameAttribute.cs
- DiscreteKeyFrames.cs
- CommandExpr.cs
- FunctionMappingTranslator.cs
- AudioFileOut.cs
- Scripts.cs
- Container.cs
- XmlILModule.cs
- ErrorLog.cs
- AdCreatedEventArgs.cs
- ToolStripGripRenderEventArgs.cs
- WinEventHandler.cs
- MatrixAnimationUsingPath.cs
- ProgramPublisher.cs
- DateTimePicker.cs
- SqlDataSourceConfigureFilterForm.cs
- ManualResetEvent.cs
- ContentIterators.cs
- DataServiceRequestArgs.cs
- CodeObjectCreateExpression.cs
- XmlSchemaRedefine.cs
- BindingList.cs
- BaseTreeIterator.cs
- AnnotationResource.cs
- MappedMetaModel.cs
- FormView.cs
- WriteTimeStream.cs
- FlowLayout.cs
- XmlSchemaInclude.cs
- OdbcConnectionFactory.cs
- TheQuery.cs
- ListViewDataItem.cs
- FormsAuthenticationEventArgs.cs
- KeySplineConverter.cs
- VisualCollection.cs
- TableLayoutSettingsTypeConverter.cs
- XsltLibrary.cs
- WeakReferenceList.cs
- WebBrowsableAttribute.cs
- TaskDesigner.cs
- FixedNode.cs
- TrustLevelCollection.cs
- UriTemplateTrieNode.cs
- OracleTransaction.cs
- ConfigUtil.cs
- XhtmlCssHandler.cs
- DataColumn.cs
- MetadataWorkspace.cs
- WriterOutput.cs