Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridColumnCollection.cs / 1 / DataGridColumnCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Runtime.Remoting; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Collections; using System.Drawing.Design; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; ////// /// [ Editor("System.Windows.Forms.Design.DataGridColumnCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), ListBindable(false) ] public class GridColumnStylesCollection : BaseCollection, IList { CollectionChangeEventHandler onCollectionChanged; ArrayList items = new ArrayList(); DataGridTableStyle owner = null; private bool isDefault = false; // we have to implement IList for the Collection editor to work // ///Represents a collection of System.Windows.Forms.DataGridColumnStyle objects in the ////// control. /// int IList.Add(object value) { return this.Add((DataGridColumnStyle) value); } /// /// void IList.Clear() { this.Clear(); } /// /// bool IList.Contains(object value) { return items.Contains(value); } /// /// int IList.IndexOf(object value) { return items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { throw new NotSupportedException(); } /// /// void IList.Remove(object value) { this.Remove((DataGridColumnStyle)value); } /// /// void IList.RemoveAt(int index) { this.RemoveAt(index); } /// /// bool IList.IsFixedSize { get {return false;} } /// /// bool IList.IsReadOnly { get {return false;} } /// /// object IList.this[int index] { get { return items[index]; } set { throw new NotSupportedException(); } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get {return this.items.Count;} } /// /// bool ICollection.IsSynchronized { get {return false;} } /// /// object ICollection.SyncRoot { get {return this;} } /// /// IEnumerator IEnumerable.GetEnumerator() { return items.GetEnumerator(); } internal GridColumnStylesCollection(DataGridTableStyle table) { owner = table; } internal GridColumnStylesCollection(DataGridTableStyle table, bool isDefault) : this(table) { this.isDefault = isDefault; } /// /// /// protected override ArrayList List { get { return items; } } /* implemented in BaseCollection ///Gets the list of items in the collection. ////// ////// Gets the number of System.Windows.Forms.DataGridColumnStyle objects in the collection. /// ////// ////// The number of System.Windows.Forms.DataGridColumnStyle objects in the System.Windows.Forms.GridColumnsStyleCollection . /// ////// ////// The following example uses the ////// property to determine how many System.Windows.Forms.DataGridColumnStyle objects are in a System.Windows.Forms.GridColumnsStyleCollection, and uses that number to iterate through the /// collection. /// /// Private Sub PrintGridColumns() /// Dim colsCount As Integer /// colsCount = DataGrid1.GridColumns.Count /// Dim i As Integer /// For i = 0 to colsCount - 1 /// Debug.Print DataGrid1.GridColumns(i).GetType.ToString /// Next i /// End Sub ///
////// public override int Count { get { return items.Count; } } */ /// /// /// public DataGridColumnStyle this[int index] { get { return (DataGridColumnStyle)items[index]; } } ///Gets the System.Windows.Forms.DataGridColumnStyle at a specified index. ////// /// public DataGridColumnStyle this[string columnName] { // PM team has reviewed and decided on naming changes already [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] get { int itemCount = items.Count; for (int i = 0; i < itemCount; ++i) { DataGridColumnStyle column = (DataGridColumnStyle)items[i]; // NOTE: case-insensitive if (String.Equals(column.MappingName, columnName, StringComparison.OrdinalIgnoreCase)) return column; } return null; } } internal DataGridColumnStyle MapColumnStyleToPropertyName(string mappingName) { int itemCount = items.Count; for (int i = 0; i < itemCount; ++i) { DataGridColumnStyle column = (DataGridColumnStyle)items[i]; // NOTE: case-insensitive if (String.Equals(column.MappingName, mappingName, StringComparison.OrdinalIgnoreCase)) return column; } return null; } ///Gets the System.Windows.Forms.DataGridColumnStyle /// with the specified name. ////// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1043:UseIntegralOrStringArgumentForIndexers")] public DataGridColumnStyle this[PropertyDescriptor propertyDesciptor] { [ System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly") // already shipped ] get { int itemCount = items.Count; for (int i = 0; i < itemCount; ++i) { DataGridColumnStyle column = (DataGridColumnStyle)items[i]; if (propertyDesciptor.Equals(column.PropertyDescriptor)) return column; } return null; } } internal DataGridTableStyle DataGridTableStyle { get { return this.owner; } } ///Gets the System.Windows.Forms.DataGridColumnStyle associated with the /// specified ///. /// internal void CheckForMappingNameDuplicates(DataGridColumnStyle column) { if (String.IsNullOrEmpty(column.MappingName)) return; for (int i = 0; i < items.Count; i++) if ( ((DataGridColumnStyle)items[i]).MappingName.Equals(column.MappingName) && column != items[i]) throw new ArgumentException(SR.GetString(SR.DataGridColumnStyleDuplicateMappingName), "column"); } private void ColumnStyleMappingNameChanged(object sender, EventArgs pcea) { OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } private void ColumnStylePropDescChanged(object sender, EventArgs pcea) { OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, (DataGridColumnStyle) sender)); } ///Adds a System.Windows.Forms.DataGridColumnStyle to the System.Windows.Forms.GridColumnStylesCollection ////// /// public virtual int Add(DataGridColumnStyle column) { if (this.isDefault) { throw new ArgumentException(SR.GetString(SR.DataGridDefaultColumnCollectionChanged)); } CheckForMappingNameDuplicates(column); column.SetDataGridTableInColumn(owner, true); column.MappingNameChanged += new EventHandler(ColumnStyleMappingNameChanged); column.PropertyDescriptorChanged += new EventHandler(ColumnStylePropDescChanged); // columns which are not the default should have a default // width of DataGrid.PreferredColumnWidth if (this.DataGridTableStyle != null && column.Width == -1) column.width = this.DataGridTableStyle.PreferredColumnWidth; #if false column.AddOnPropertyChanged(owner.OnColumnChanged); #endif int index = items.Add(column); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, column)); return index; } ///[To be supplied.] ///public void AddRange(DataGridColumnStyle[] columns) { if (columns == null) { throw new ArgumentNullException("columns"); } for (int i = 0; i < columns.Length; i++) { Add(columns[i]); } } // the dataGrid will need to add default columns to a default // table when there is no match for the listName in the tableStyle internal void AddDefaultColumn(DataGridColumnStyle column) { #if DEBUG Debug.Assert(this.isDefault, "we should be calling this function only for default tables"); Debug.Assert(column.IsDefault, "we should be a default column"); #endif // DEBUG column.SetDataGridTableInColumn(owner, true); this.items.Add(column); } internal void ResetDefaultColumnCollection() { Debug.Assert(this.isDefault, "we should be calling this function only for default tables"); // unparent the edit controls for (int i = 0; i < Count; i++) { this[i].ReleaseHostedControl(); } // get rid of the old list and get a new empty list items.Clear(); } /// /// /// public event CollectionChangeEventHandler CollectionChanged { add { onCollectionChanged += value; } remove { onCollectionChanged -= value; } } ///Occurs when a change is made to the System.Windows.Forms.GridColumnStylesCollection. ////// /// public void Clear() { for (int i = 0; i < Count; i ++) { this[i].ReleaseHostedControl(); } items.Clear(); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///[To be supplied.] ////// /// public bool Contains(PropertyDescriptor propertyDescriptor) { return this[propertyDescriptor] != null; } ////// Gets a value indicating whether the System.Windows.Forms.GridColumnStylesCollection contains a System.Windows.Forms.DataGridColumnStyle associated with the /// specified ///. /// /// /// public bool Contains(DataGridColumnStyle column) { int index = items.IndexOf(column); return index != -1; } ////// Gets a value indicating whether the System.Windows.Forms.GridColumnsStyleCollection contains the specified System.Windows.Forms.DataGridColumnStyle. /// ////// /// public bool Contains(string name) { IEnumerator e = items.GetEnumerator(); while (e.MoveNext()) { DataGridColumnStyle column = (DataGridColumnStyle)e.Current; // NOTE: case-insensitive if (String.Compare(column.MappingName, name, true, CultureInfo.InvariantCulture) == 0) return true; } return false; } /* implemented at BaseCollection ////// Gets a value indicating whether the System.Windows.Forms.GridColumnsStyleCollection contains the System.Windows.Forms.DataGridColumnStyle with the specified name. /// ////// ////// Gets an enumerator for the System.Windows.Forms.GridColumnsStyleCollection. /// ////// ////// Gets an enumerator for the System.Windows.Forms.GridColumnsStyleCollection. /// ////// ////// An ////// that can be used to iterate through the collection. /// /// ////// The following example gets an ///that iterates through the System.Windows.Forms.GridColumnsStyleCollection. and prints the /// of each /// associated with the object. /// /// Private Sub EnumerateThroughGridColumns() /// Dim ie As System.Collections.IEnumerator /// Dim dgCol As DataGridColumn /// Set ie = DataGrid1.GridColumns.GetEnumerator /// Do While ie.GetNext = True /// Set dgCol = ie.GetObject /// Debug.Print dgCol.DataColumn.Caption /// Loop /// End Sub ///
////// /// /// public override IEnumerator GetEnumerator() { return items.GetEnumerator(); } /// /// /// ////// Gets an enumerator for the System.Windows.Forms.GridColumnsStyleCollection /// . /// ///A value that indicates if the enumerator can remove elements. /// ///, if removals are allowed; otherwise, . The default is . /// ////// An ///that can be used to iterate through the /// collection. /// /// An attempt was made to remove the System.Windows.Forms.DataGridColumnStyle through the ///object's method. Use the System.Windows.Forms.GridColumnsStyleCollection object's method instead. /// /// ////// Because this implementation doesn't support the removal /// of System.Windows.Forms.DataGridColumnStyle objects through the ////// class's method, you must use the class's /// method instead. /// /// ////// The following example gets an ///for that iterates through the System.Windows.Forms.GridColumnsStyleCollection. If a column in the collection is of type , it is deleted. /// /// Private Sub RemoveBoolColumns() /// Dim ie As System.Collections.IEnumerator /// Dim dgCol As DataGridColumn /// Set ie = DataGrid1.GridColumns.GetEnumerator(true) /// Do While ie.GetNext /// Set dgCol = ie.GetObject /// /// If dgCol.ToString = "DataGridBoolColumn" Then /// DataGrid1.GridColumns.Remove dgCol /// End If /// Loop /// End If ///
////// /// /// public override IEnumerator GetEnumerator(bool allowRemove) { if (!allowRemove) return GetEnumerator(); else throw new NotSupportedException(SR.GetString(SR.DataGridColumnCollectionGetEnumerator)); } */ /// /// /// public int IndexOf(DataGridColumnStyle element) { int itemCount = items.Count; for (int i = 0; i < itemCount; ++i) { DataGridColumnStyle column = (DataGridColumnStyle)items[i]; if (element == column) return i; } return -1; } ///Gets the index of a specified System.Windows.Forms.DataGridColumnStyle. ////// /// protected void OnCollectionChanged(CollectionChangeEventArgs e) { if (onCollectionChanged != null) onCollectionChanged(this, e); DataGrid grid = owner.DataGrid; if (grid != null) { grid.checkHierarchy = true; } } ///Raises the System.Windows.Forms.GridColumnsCollection.CollectionChanged event. ////// /// public void Remove(DataGridColumnStyle column) { if (this.isDefault) { throw new ArgumentException(SR.GetString(SR.DataGridDefaultColumnCollectionChanged)); } int columnIndex = -1; int itemsCount = items.Count; for (int i = 0; i < itemsCount; ++i) if (items[i] == column) { columnIndex = i; break; } if (columnIndex == -1) throw new InvalidOperationException(SR.GetString(SR.DataGridColumnCollectionMissing)); else RemoveAt(columnIndex); } ///Removes the specified System.Windows.Forms.DataGridColumnStyle from the System.Windows.Forms.GridColumnsStyleCollection. ////// /// public void RemoveAt(int index) { if (this.isDefault) { throw new ArgumentException(SR.GetString(SR.DataGridDefaultColumnCollectionChanged)); } DataGridColumnStyle toRemove = (DataGridColumnStyle)items[index]; toRemove.SetDataGridTableInColumn(null, true); toRemove.MappingNameChanged -= new EventHandler(ColumnStyleMappingNameChanged); toRemove.PropertyDescriptorChanged -= new EventHandler(ColumnStylePropDescChanged); #if false toRemove.RemoveOnPropertyChange(owner.OnColumnChanged); #endif items.RemoveAt(index); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Remove, toRemove)); } ///Removes the System.Windows.Forms.DataGridColumnStyle with the specified index from the System.Windows.Forms.GridColumnsStyleCollection. ////// /// public void ResetPropertyDescriptors() { for (int i = 0; i < this.Count; i++) { this[i].PropertyDescriptor = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
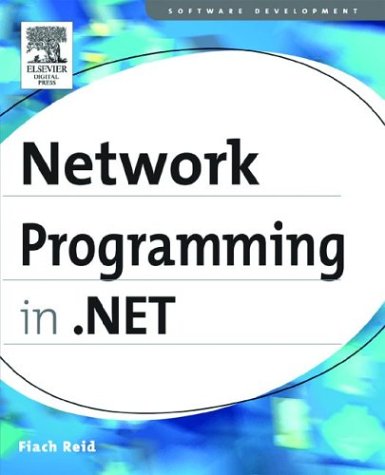
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlClientMetaDataCollectionNames.cs
- MDIWindowDialog.cs
- BooleanToVisibilityConverter.cs
- TextEditorCharacters.cs
- OnOperation.cs
- UniqueConstraint.cs
- Parsers.cs
- unsafenativemethodsother.cs
- HostingEnvironmentSection.cs
- FullTextState.cs
- RectangleHotSpot.cs
- ControlEvent.cs
- UnrecognizedPolicyAssertionElement.cs
- DocumentSchemaValidator.cs
- EventHandlerList.cs
- CaseInsensitiveOrdinalStringComparer.cs
- ParsedAttributeCollection.cs
- SuppressMergeCheckAttribute.cs
- VisualCollection.cs
- ResizingMessageFilter.cs
- SelectedDatesCollection.cs
- Convert.cs
- ValidationRuleCollection.cs
- StatusBarPanelClickEvent.cs
- CodeEntryPointMethod.cs
- NativeCompoundFileAPIs.cs
- IInstanceTable.cs
- CheckBoxBaseAdapter.cs
- ComponentTray.cs
- TextDecorations.cs
- LoginName.cs
- SmiMetaDataProperty.cs
- UnsupportedPolicyOptionsException.cs
- Dictionary.cs
- BindingExpressionBase.cs
- CodeIdentifiers.cs
- RenderDataDrawingContext.cs
- ProviderUtil.cs
- OleDbFactory.cs
- DynamicScriptObject.cs
- ElementNotAvailableException.cs
- StringInfo.cs
- DeleteHelper.cs
- DataGridCommandEventArgs.cs
- SimpleTableProvider.cs
- RequestCachePolicy.cs
- ButtonBaseAutomationPeer.cs
- Int64KeyFrameCollection.cs
- DrawItemEvent.cs
- DbParameterHelper.cs
- BlockUIContainer.cs
- CombinedGeometry.cs
- DataGridColumnHeader.cs
- DataGridTablesFactory.cs
- GeneralTransform3D.cs
- Item.cs
- NavigatorOutput.cs
- TextFormatterHost.cs
- XmlException.cs
- AutomationInteropProvider.cs
- mediaeventshelper.cs
- templategroup.cs
- ServiceReference.cs
- mansign.cs
- Pair.cs
- ProxyHelper.cs
- ResourceDictionaryCollection.cs
- LineGeometry.cs
- ICspAsymmetricAlgorithm.cs
- DataGridToolTip.cs
- ProfileBuildProvider.cs
- Range.cs
- StringExpressionSet.cs
- FreezableCollection.cs
- TextTreeInsertUndoUnit.cs
- DesignTimeVisibleAttribute.cs
- PenThread.cs
- streamingZipPartStream.cs
- XmlSchemaAll.cs
- RegularExpressionValidator.cs
- ToolStripRenderEventArgs.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- SafeNativeMethods.cs
- SynchronizationLockException.cs
- DataBinding.cs
- panel.cs
- OdbcCommand.cs
- WebPartsSection.cs
- WindowsSlider.cs
- AttachmentCollection.cs
- DbConvert.cs
- OutputCacheProfileCollection.cs
- NavigationProgressEventArgs.cs
- Listbox.cs
- EmbeddedMailObject.cs
- QueryableDataSourceHelper.cs
- _SslState.cs
- EnumBuilder.cs
- UpdateException.cs
- ReflectionTypeLoadException.cs